Send json array data from html to Flask
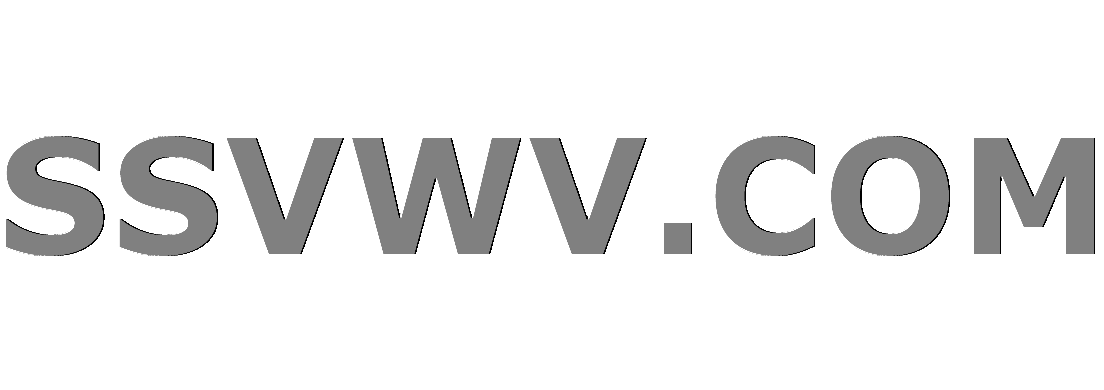
Multi tool use
up vote
0
down vote
favorite
I have a variable, called images, in my frontend holding a handful of images selected by the user.
The following:
JSON.stringify(images)
would then return
["image1", "image2", "image3"]
if the user selected the first three images.
I want to POST this array to Flask, make flask do some manipulation and then return the images in a new route.
My jQuery script for the HTTP post looks as follows:
$("document").ready(function() {
$("#btn1").click(function(){
$.ajax({
type: 'POST',
contentType: 'application/json',
url: "{{ url_for('filter') }}",
dataType : 'json',
data : JSON.stringify(images)
})
})
})
The route responsible for the manipulation looks as follows:
@app.route("/filter", methods=["POST", "GET"])
def filter():
if request.method=="POST":
result=request.get_json()
## Do some manipulation to result
return render_template("result.html",result=result)
My problem is that the data retrieved by Flask is empty.
jquery ajax flask
add a comment |
up vote
0
down vote
favorite
I have a variable, called images, in my frontend holding a handful of images selected by the user.
The following:
JSON.stringify(images)
would then return
["image1", "image2", "image3"]
if the user selected the first three images.
I want to POST this array to Flask, make flask do some manipulation and then return the images in a new route.
My jQuery script for the HTTP post looks as follows:
$("document").ready(function() {
$("#btn1").click(function(){
$.ajax({
type: 'POST',
contentType: 'application/json',
url: "{{ url_for('filter') }}",
dataType : 'json',
data : JSON.stringify(images)
})
})
})
The route responsible for the manipulation looks as follows:
@app.route("/filter", methods=["POST", "GET"])
def filter():
if request.method=="POST":
result=request.get_json()
## Do some manipulation to result
return render_template("result.html",result=result)
My problem is that the data retrieved by Flask is empty.
jquery ajax flask
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I have a variable, called images, in my frontend holding a handful of images selected by the user.
The following:
JSON.stringify(images)
would then return
["image1", "image2", "image3"]
if the user selected the first three images.
I want to POST this array to Flask, make flask do some manipulation and then return the images in a new route.
My jQuery script for the HTTP post looks as follows:
$("document").ready(function() {
$("#btn1").click(function(){
$.ajax({
type: 'POST',
contentType: 'application/json',
url: "{{ url_for('filter') }}",
dataType : 'json',
data : JSON.stringify(images)
})
})
})
The route responsible for the manipulation looks as follows:
@app.route("/filter", methods=["POST", "GET"])
def filter():
if request.method=="POST":
result=request.get_json()
## Do some manipulation to result
return render_template("result.html",result=result)
My problem is that the data retrieved by Flask is empty.
jquery ajax flask
I have a variable, called images, in my frontend holding a handful of images selected by the user.
The following:
JSON.stringify(images)
would then return
["image1", "image2", "image3"]
if the user selected the first three images.
I want to POST this array to Flask, make flask do some manipulation and then return the images in a new route.
My jQuery script for the HTTP post looks as follows:
$("document").ready(function() {
$("#btn1").click(function(){
$.ajax({
type: 'POST',
contentType: 'application/json',
url: "{{ url_for('filter') }}",
dataType : 'json',
data : JSON.stringify(images)
})
})
})
The route responsible for the manipulation looks as follows:
@app.route("/filter", methods=["POST", "GET"])
def filter():
if request.method=="POST":
result=request.get_json()
## Do some manipulation to result
return render_template("result.html",result=result)
My problem is that the data retrieved by Flask is empty.
jquery ajax flask
jquery ajax flask
asked Nov 21 at 18:48
Nicolai Iversen
454
454
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
up vote
0
down vote
accepted
The dataType for the response expected from the Flask app server was configured to be JSON
.
dataType: 'json',
That is not the right dataType as the Flask server returns HTML in it's response and jQuery runs into error trying to parse it into a JavaScript object.
return render_template("result.html", result=result)
JSON response can be returned from the server and handle appropriately in the success handler.
from flask import jsonify
#...
return jsonify(result)
Or the dataType
for the response set to html
(for this, you can insert the returned markup piece into HTML document).
Okay, so I tried both, but it is still like jQuery does not parse any data to Flask. Setting dataType='json' and return jsonify(result), returns null in the /filter route
– Nicolai Iversen
Nov 21 at 19:59
I'd verify the value ofimages
inJSON.stringify(images)
. I don't see a reason forrequest.get_json()
to beNone
– Oluwafemi Sule
Nov 21 at 20:23
Okay yes, you were right
– Nicolai Iversen
Nov 21 at 21:16
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
accepted
The dataType for the response expected from the Flask app server was configured to be JSON
.
dataType: 'json',
That is not the right dataType as the Flask server returns HTML in it's response and jQuery runs into error trying to parse it into a JavaScript object.
return render_template("result.html", result=result)
JSON response can be returned from the server and handle appropriately in the success handler.
from flask import jsonify
#...
return jsonify(result)
Or the dataType
for the response set to html
(for this, you can insert the returned markup piece into HTML document).
Okay, so I tried both, but it is still like jQuery does not parse any data to Flask. Setting dataType='json' and return jsonify(result), returns null in the /filter route
– Nicolai Iversen
Nov 21 at 19:59
I'd verify the value ofimages
inJSON.stringify(images)
. I don't see a reason forrequest.get_json()
to beNone
– Oluwafemi Sule
Nov 21 at 20:23
Okay yes, you were right
– Nicolai Iversen
Nov 21 at 21:16
add a comment |
up vote
0
down vote
accepted
The dataType for the response expected from the Flask app server was configured to be JSON
.
dataType: 'json',
That is not the right dataType as the Flask server returns HTML in it's response and jQuery runs into error trying to parse it into a JavaScript object.
return render_template("result.html", result=result)
JSON response can be returned from the server and handle appropriately in the success handler.
from flask import jsonify
#...
return jsonify(result)
Or the dataType
for the response set to html
(for this, you can insert the returned markup piece into HTML document).
Okay, so I tried both, but it is still like jQuery does not parse any data to Flask. Setting dataType='json' and return jsonify(result), returns null in the /filter route
– Nicolai Iversen
Nov 21 at 19:59
I'd verify the value ofimages
inJSON.stringify(images)
. I don't see a reason forrequest.get_json()
to beNone
– Oluwafemi Sule
Nov 21 at 20:23
Okay yes, you were right
– Nicolai Iversen
Nov 21 at 21:16
add a comment |
up vote
0
down vote
accepted
up vote
0
down vote
accepted
The dataType for the response expected from the Flask app server was configured to be JSON
.
dataType: 'json',
That is not the right dataType as the Flask server returns HTML in it's response and jQuery runs into error trying to parse it into a JavaScript object.
return render_template("result.html", result=result)
JSON response can be returned from the server and handle appropriately in the success handler.
from flask import jsonify
#...
return jsonify(result)
Or the dataType
for the response set to html
(for this, you can insert the returned markup piece into HTML document).
The dataType for the response expected from the Flask app server was configured to be JSON
.
dataType: 'json',
That is not the right dataType as the Flask server returns HTML in it's response and jQuery runs into error trying to parse it into a JavaScript object.
return render_template("result.html", result=result)
JSON response can be returned from the server and handle appropriately in the success handler.
from flask import jsonify
#...
return jsonify(result)
Or the dataType
for the response set to html
(for this, you can insert the returned markup piece into HTML document).
answered Nov 21 at 19:39


Oluwafemi Sule
10.2k1330
10.2k1330
Okay, so I tried both, but it is still like jQuery does not parse any data to Flask. Setting dataType='json' and return jsonify(result), returns null in the /filter route
– Nicolai Iversen
Nov 21 at 19:59
I'd verify the value ofimages
inJSON.stringify(images)
. I don't see a reason forrequest.get_json()
to beNone
– Oluwafemi Sule
Nov 21 at 20:23
Okay yes, you were right
– Nicolai Iversen
Nov 21 at 21:16
add a comment |
Okay, so I tried both, but it is still like jQuery does not parse any data to Flask. Setting dataType='json' and return jsonify(result), returns null in the /filter route
– Nicolai Iversen
Nov 21 at 19:59
I'd verify the value ofimages
inJSON.stringify(images)
. I don't see a reason forrequest.get_json()
to beNone
– Oluwafemi Sule
Nov 21 at 20:23
Okay yes, you were right
– Nicolai Iversen
Nov 21 at 21:16
Okay, so I tried both, but it is still like jQuery does not parse any data to Flask. Setting dataType='json' and return jsonify(result), returns null in the /filter route
– Nicolai Iversen
Nov 21 at 19:59
Okay, so I tried both, but it is still like jQuery does not parse any data to Flask. Setting dataType='json' and return jsonify(result), returns null in the /filter route
– Nicolai Iversen
Nov 21 at 19:59
I'd verify the value of
images
in JSON.stringify(images)
. I don't see a reason for request.get_json()
to be None
– Oluwafemi Sule
Nov 21 at 20:23
I'd verify the value of
images
in JSON.stringify(images)
. I don't see a reason for request.get_json()
to be None
– Oluwafemi Sule
Nov 21 at 20:23
Okay yes, you were right
– Nicolai Iversen
Nov 21 at 21:16
Okay yes, you were right
– Nicolai Iversen
Nov 21 at 21:16
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53418733%2fsend-json-array-data-from-html-to-flask%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
7sCzA E,IHymH