Self Updating App Won't Install Update Anymore
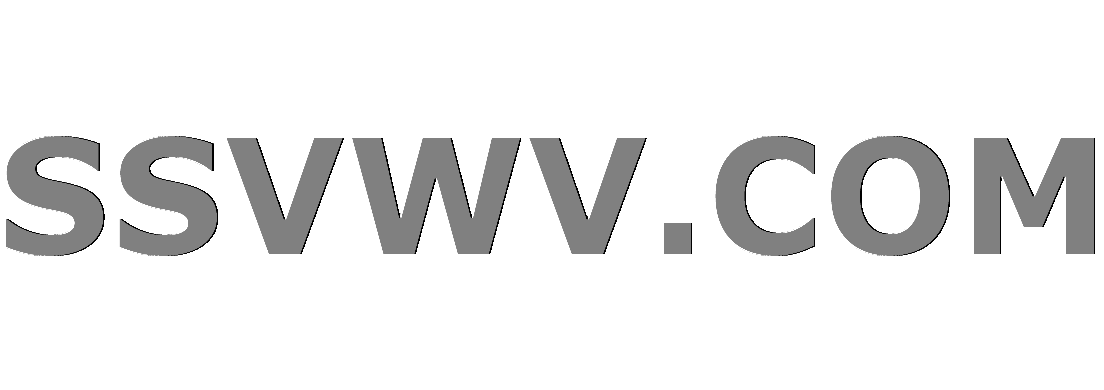
Multi tool use
up vote
0
down vote
favorite
I've got a private app for my organization that is not in Google Play Store (it is classified by Android as "Unknown Source"). Up until Android 8 (Oreo), it could successfully contact the server, determine if the running version was different from the one on the server, and download and install the update if a new version was available, with very little interaction from the user. The following is the code that was working prior to Android 8:
String apkURLStr = "http://myserver.com/MyApp.apk";
if (!currentAppVersion.equals(appVersion)) {
UpdateApp atualizaApp = new UpdateApp();
atualizaApp.setContext(getApplicationContext());
atualizaApp.execute(apkURLStr);
}
public class UpdateApp extends AsyncTask<String, Void, Void> {
private ProgressDialog dialog = new ProgressDialog(MyActivity.this);
private Context context;
private String saveResult;
public void setContext(Context contextf) {
context = contextf;
}
@Override
protected Void doInBackground(String... arg0) {
try {
// download and save the new apk file
URL url = new URL(arg0[0]);
HttpURLConnection c = (HttpURLConnection) url.openConnection();
c.setRequestMethod("GET");
c.setDoOutput(true);
c.connect();
String PATH = Environment.getExternalStorageDirectory() + "/download/";
File file = new File(PATH);
file.mkdirs();
File outputFile = new File(file, "MyApp.apk");
if (outputFile.exists()) {
outputFile.delete();
}
FileOutputStream fos = new FileOutputStream(outputFile);
InputStream is = c.getInputStream();
byte buffer = new byte[1024];
int len1 = 0;
while ((len1 = is.read(buffer)) != -1) {
fos.write(buffer, 0, len1);
}
fos.close();
is.close();
saveResult = "";
// initiate view of new apk so user can choose to install it
Intent intent = new Intent(Intent.ACTION_VIEW);
intent.setDataAndType(Uri.fromFile(new File(Environment.getExternalStorageDirectory() + "/download/" + "MyApp.apk")), "application/vnd.android.package-archive");
intent.setFlags(Intent.FLAG_ACTIVITY_NEW_TASK);
context.startActivity(intent);
}
catch (Exception e) {
saveResult = "Error occurred while downloading update. Please login again to retry download.";
}
return null;
}
@Override
protected void onPostExecute(Void result) {
GeneralUtils.dismissWithCheck(this.dialog);
if (saveResult.length() > 0) {
displayAlertMessage(saveResult);
}
super.onPostExecute(result);
}
@Override
protected void onPreExecute() {
super.onPreExecute();
if (this.dialog != null) {
this.dialog.setMessage("Downloading Update. Please wait..");
this.dialog.show();
}
}
}
After Android 8, this code is no longer working. The download completes, the "for your security, your phone is not allowed to install unknown apps from this source dialog displays, I go to Settings to toggle "Allow from this source" on, I touch the INSTALL button, the install starts and then it ends unsuccessfully with the nondescript error "App Not Installed" as seen below.
(https://imgur.com/a/0lm7Kvp)
(https://imgur.com/a/0lm7Kvp)
(https://imgur.com/a/0lm7Kvp)
(https://imgur.com/a/0lm7Kvp)
I've tried portions of the solution in this post How to manage installation from Unknown Sources in Android Oreo? but I cannot target Android 26 (version 8), as some of my users do not have devices running Android 8 yet. I did test this solution by targeting 26, but even when the user toggles unknown sources on for the apk, the installation is still unsuccessful.
My initial problem is that my app contains the code above, so there is no way that it will be able to update itself, since that code no longer works in Android 8, no matter what settings regarding unknown sources are toggled. I will have to ask my users to uninstall the app, and install the new version from the server. The second problem is that I need to modify the code for the new version, so that in the future, it can update itself. My question is, what code, permissions, or device settings changes must be made to have my app successfully update itself in the future?
BTW, uninstalling the current version of the app, and linking to the apk file for the new version in Chrome, which downloads it and initiates the install, works successfully.
Changes I have tried, that were unsuccessful:
adding this to the manifest:
<uses-permission android:name="android.permission.REQUEST_INSTALL_PACKAGES"/>
linking to the apk location in Chrome to see if it would initiate the download/install because then the apk file would be in the official "downloads" folder and possibly cause install to work
using FileProvider to install the package:
Uri apkUri = FileProvider.getUriForFile(MyActivity.this, "myapp.provider", outputFile);
Intent intent = new Intent(Intent.ACTION_INSTALL_PACKAGE);
intent.setDataAndType(apkUri, "application/vnd.android.package-archive");
intent.setFlags(Intent.FLAG_GRANT_READ_URI_PERMISSION);
MyActivity.this.startActivity(intent);
Specifically requesting permission to Manifest.permission.REQUEST_INSTALL_PACKAGES using ActivityCompat.shouldShowRequestPermissionRationale. In this case, the permission was not asked of the user, since the result of the method call was false. Even with the android.permission.REQUEST_INSTALL_PACKAGES permission in the manifest, the result of calling ContextCompat.checkSelfPermission was not PackageManager.PERMISSION_GRANTED.
Generating the signed apk in both V1 (Jar Signature) and V2 (Full APK Signature)
Various results, either with zero visible feedback on the device, or with errors similar to the one described above were produced by the steps outlined above, all of which with no success.
There are so many posts regarding this situation, all of which contained no success when I tried them, either in total or piecemeal. The app cannot be put in Google Play Store, as it should not be visible to the public or even stored in a cloud for security reasons, so I need a solution of the "unknown sources" variety that works.
Any suggestions/solutions would be greatly appreciated.
java

add a comment |
up vote
0
down vote
favorite
I've got a private app for my organization that is not in Google Play Store (it is classified by Android as "Unknown Source"). Up until Android 8 (Oreo), it could successfully contact the server, determine if the running version was different from the one on the server, and download and install the update if a new version was available, with very little interaction from the user. The following is the code that was working prior to Android 8:
String apkURLStr = "http://myserver.com/MyApp.apk";
if (!currentAppVersion.equals(appVersion)) {
UpdateApp atualizaApp = new UpdateApp();
atualizaApp.setContext(getApplicationContext());
atualizaApp.execute(apkURLStr);
}
public class UpdateApp extends AsyncTask<String, Void, Void> {
private ProgressDialog dialog = new ProgressDialog(MyActivity.this);
private Context context;
private String saveResult;
public void setContext(Context contextf) {
context = contextf;
}
@Override
protected Void doInBackground(String... arg0) {
try {
// download and save the new apk file
URL url = new URL(arg0[0]);
HttpURLConnection c = (HttpURLConnection) url.openConnection();
c.setRequestMethod("GET");
c.setDoOutput(true);
c.connect();
String PATH = Environment.getExternalStorageDirectory() + "/download/";
File file = new File(PATH);
file.mkdirs();
File outputFile = new File(file, "MyApp.apk");
if (outputFile.exists()) {
outputFile.delete();
}
FileOutputStream fos = new FileOutputStream(outputFile);
InputStream is = c.getInputStream();
byte buffer = new byte[1024];
int len1 = 0;
while ((len1 = is.read(buffer)) != -1) {
fos.write(buffer, 0, len1);
}
fos.close();
is.close();
saveResult = "";
// initiate view of new apk so user can choose to install it
Intent intent = new Intent(Intent.ACTION_VIEW);
intent.setDataAndType(Uri.fromFile(new File(Environment.getExternalStorageDirectory() + "/download/" + "MyApp.apk")), "application/vnd.android.package-archive");
intent.setFlags(Intent.FLAG_ACTIVITY_NEW_TASK);
context.startActivity(intent);
}
catch (Exception e) {
saveResult = "Error occurred while downloading update. Please login again to retry download.";
}
return null;
}
@Override
protected void onPostExecute(Void result) {
GeneralUtils.dismissWithCheck(this.dialog);
if (saveResult.length() > 0) {
displayAlertMessage(saveResult);
}
super.onPostExecute(result);
}
@Override
protected void onPreExecute() {
super.onPreExecute();
if (this.dialog != null) {
this.dialog.setMessage("Downloading Update. Please wait..");
this.dialog.show();
}
}
}
After Android 8, this code is no longer working. The download completes, the "for your security, your phone is not allowed to install unknown apps from this source dialog displays, I go to Settings to toggle "Allow from this source" on, I touch the INSTALL button, the install starts and then it ends unsuccessfully with the nondescript error "App Not Installed" as seen below.
(https://imgur.com/a/0lm7Kvp)
(https://imgur.com/a/0lm7Kvp)
(https://imgur.com/a/0lm7Kvp)
(https://imgur.com/a/0lm7Kvp)
I've tried portions of the solution in this post How to manage installation from Unknown Sources in Android Oreo? but I cannot target Android 26 (version 8), as some of my users do not have devices running Android 8 yet. I did test this solution by targeting 26, but even when the user toggles unknown sources on for the apk, the installation is still unsuccessful.
My initial problem is that my app contains the code above, so there is no way that it will be able to update itself, since that code no longer works in Android 8, no matter what settings regarding unknown sources are toggled. I will have to ask my users to uninstall the app, and install the new version from the server. The second problem is that I need to modify the code for the new version, so that in the future, it can update itself. My question is, what code, permissions, or device settings changes must be made to have my app successfully update itself in the future?
BTW, uninstalling the current version of the app, and linking to the apk file for the new version in Chrome, which downloads it and initiates the install, works successfully.
Changes I have tried, that were unsuccessful:
adding this to the manifest:
<uses-permission android:name="android.permission.REQUEST_INSTALL_PACKAGES"/>
linking to the apk location in Chrome to see if it would initiate the download/install because then the apk file would be in the official "downloads" folder and possibly cause install to work
using FileProvider to install the package:
Uri apkUri = FileProvider.getUriForFile(MyActivity.this, "myapp.provider", outputFile);
Intent intent = new Intent(Intent.ACTION_INSTALL_PACKAGE);
intent.setDataAndType(apkUri, "application/vnd.android.package-archive");
intent.setFlags(Intent.FLAG_GRANT_READ_URI_PERMISSION);
MyActivity.this.startActivity(intent);
Specifically requesting permission to Manifest.permission.REQUEST_INSTALL_PACKAGES using ActivityCompat.shouldShowRequestPermissionRationale. In this case, the permission was not asked of the user, since the result of the method call was false. Even with the android.permission.REQUEST_INSTALL_PACKAGES permission in the manifest, the result of calling ContextCompat.checkSelfPermission was not PackageManager.PERMISSION_GRANTED.
Generating the signed apk in both V1 (Jar Signature) and V2 (Full APK Signature)
Various results, either with zero visible feedback on the device, or with errors similar to the one described above were produced by the steps outlined above, all of which with no success.
There are so many posts regarding this situation, all of which contained no success when I tried them, either in total or piecemeal. The app cannot be put in Google Play Store, as it should not be visible to the public or even stored in a cloud for security reasons, so I need a solution of the "unknown sources" variety that works.
Any suggestions/solutions would be greatly appreciated.
java

Sounds like the APK you're getting from the server is malformed somehow. Are you compiling the app in the normal way?
– Sub 6 Resources
Nov 21 at 18:56
"but I cannot target Android 26 (version 8), as some of my users do not have devices running Android 8 yet" setting atargetSdkVersion
of 26 or higher does not prevent you from having aminSdkVersion
that is lower than 26. That's why they are separate settings. "My question is, what code, permissions, or device settings changes must be made to have my app successfully update itself in the future?" -- that's covered in the SO question that you linked to. "using FileProvider to install the package" -- that is definitely a requirement.
– CommonsWare
Nov 21 at 22:20
"Specifically requesting permission to Manifest.permission.REQUEST_INSTALL_PACKAGES using ActivityCompat.shouldShowRequestPermissionRationale" -- that is not how you request runtime permissions. AndREQUEST_INSTALL_PACKAGES
does not work that way anyway. Its use is covered in the SO question that you linked to, leading the user to the proper Settings screen for this. Overall, though, a Minimal, Complete, and Verifiable example showing what you tried and what your specific results were would increase the chances that somebody can help you.
– CommonsWare
Nov 21 at 22:24
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I've got a private app for my organization that is not in Google Play Store (it is classified by Android as "Unknown Source"). Up until Android 8 (Oreo), it could successfully contact the server, determine if the running version was different from the one on the server, and download and install the update if a new version was available, with very little interaction from the user. The following is the code that was working prior to Android 8:
String apkURLStr = "http://myserver.com/MyApp.apk";
if (!currentAppVersion.equals(appVersion)) {
UpdateApp atualizaApp = new UpdateApp();
atualizaApp.setContext(getApplicationContext());
atualizaApp.execute(apkURLStr);
}
public class UpdateApp extends AsyncTask<String, Void, Void> {
private ProgressDialog dialog = new ProgressDialog(MyActivity.this);
private Context context;
private String saveResult;
public void setContext(Context contextf) {
context = contextf;
}
@Override
protected Void doInBackground(String... arg0) {
try {
// download and save the new apk file
URL url = new URL(arg0[0]);
HttpURLConnection c = (HttpURLConnection) url.openConnection();
c.setRequestMethod("GET");
c.setDoOutput(true);
c.connect();
String PATH = Environment.getExternalStorageDirectory() + "/download/";
File file = new File(PATH);
file.mkdirs();
File outputFile = new File(file, "MyApp.apk");
if (outputFile.exists()) {
outputFile.delete();
}
FileOutputStream fos = new FileOutputStream(outputFile);
InputStream is = c.getInputStream();
byte buffer = new byte[1024];
int len1 = 0;
while ((len1 = is.read(buffer)) != -1) {
fos.write(buffer, 0, len1);
}
fos.close();
is.close();
saveResult = "";
// initiate view of new apk so user can choose to install it
Intent intent = new Intent(Intent.ACTION_VIEW);
intent.setDataAndType(Uri.fromFile(new File(Environment.getExternalStorageDirectory() + "/download/" + "MyApp.apk")), "application/vnd.android.package-archive");
intent.setFlags(Intent.FLAG_ACTIVITY_NEW_TASK);
context.startActivity(intent);
}
catch (Exception e) {
saveResult = "Error occurred while downloading update. Please login again to retry download.";
}
return null;
}
@Override
protected void onPostExecute(Void result) {
GeneralUtils.dismissWithCheck(this.dialog);
if (saveResult.length() > 0) {
displayAlertMessage(saveResult);
}
super.onPostExecute(result);
}
@Override
protected void onPreExecute() {
super.onPreExecute();
if (this.dialog != null) {
this.dialog.setMessage("Downloading Update. Please wait..");
this.dialog.show();
}
}
}
After Android 8, this code is no longer working. The download completes, the "for your security, your phone is not allowed to install unknown apps from this source dialog displays, I go to Settings to toggle "Allow from this source" on, I touch the INSTALL button, the install starts and then it ends unsuccessfully with the nondescript error "App Not Installed" as seen below.
(https://imgur.com/a/0lm7Kvp)
(https://imgur.com/a/0lm7Kvp)
(https://imgur.com/a/0lm7Kvp)
(https://imgur.com/a/0lm7Kvp)
I've tried portions of the solution in this post How to manage installation from Unknown Sources in Android Oreo? but I cannot target Android 26 (version 8), as some of my users do not have devices running Android 8 yet. I did test this solution by targeting 26, but even when the user toggles unknown sources on for the apk, the installation is still unsuccessful.
My initial problem is that my app contains the code above, so there is no way that it will be able to update itself, since that code no longer works in Android 8, no matter what settings regarding unknown sources are toggled. I will have to ask my users to uninstall the app, and install the new version from the server. The second problem is that I need to modify the code for the new version, so that in the future, it can update itself. My question is, what code, permissions, or device settings changes must be made to have my app successfully update itself in the future?
BTW, uninstalling the current version of the app, and linking to the apk file for the new version in Chrome, which downloads it and initiates the install, works successfully.
Changes I have tried, that were unsuccessful:
adding this to the manifest:
<uses-permission android:name="android.permission.REQUEST_INSTALL_PACKAGES"/>
linking to the apk location in Chrome to see if it would initiate the download/install because then the apk file would be in the official "downloads" folder and possibly cause install to work
using FileProvider to install the package:
Uri apkUri = FileProvider.getUriForFile(MyActivity.this, "myapp.provider", outputFile);
Intent intent = new Intent(Intent.ACTION_INSTALL_PACKAGE);
intent.setDataAndType(apkUri, "application/vnd.android.package-archive");
intent.setFlags(Intent.FLAG_GRANT_READ_URI_PERMISSION);
MyActivity.this.startActivity(intent);
Specifically requesting permission to Manifest.permission.REQUEST_INSTALL_PACKAGES using ActivityCompat.shouldShowRequestPermissionRationale. In this case, the permission was not asked of the user, since the result of the method call was false. Even with the android.permission.REQUEST_INSTALL_PACKAGES permission in the manifest, the result of calling ContextCompat.checkSelfPermission was not PackageManager.PERMISSION_GRANTED.
Generating the signed apk in both V1 (Jar Signature) and V2 (Full APK Signature)
Various results, either with zero visible feedback on the device, or with errors similar to the one described above were produced by the steps outlined above, all of which with no success.
There are so many posts regarding this situation, all of which contained no success when I tried them, either in total or piecemeal. The app cannot be put in Google Play Store, as it should not be visible to the public or even stored in a cloud for security reasons, so I need a solution of the "unknown sources" variety that works.
Any suggestions/solutions would be greatly appreciated.
java

I've got a private app for my organization that is not in Google Play Store (it is classified by Android as "Unknown Source"). Up until Android 8 (Oreo), it could successfully contact the server, determine if the running version was different from the one on the server, and download and install the update if a new version was available, with very little interaction from the user. The following is the code that was working prior to Android 8:
String apkURLStr = "http://myserver.com/MyApp.apk";
if (!currentAppVersion.equals(appVersion)) {
UpdateApp atualizaApp = new UpdateApp();
atualizaApp.setContext(getApplicationContext());
atualizaApp.execute(apkURLStr);
}
public class UpdateApp extends AsyncTask<String, Void, Void> {
private ProgressDialog dialog = new ProgressDialog(MyActivity.this);
private Context context;
private String saveResult;
public void setContext(Context contextf) {
context = contextf;
}
@Override
protected Void doInBackground(String... arg0) {
try {
// download and save the new apk file
URL url = new URL(arg0[0]);
HttpURLConnection c = (HttpURLConnection) url.openConnection();
c.setRequestMethod("GET");
c.setDoOutput(true);
c.connect();
String PATH = Environment.getExternalStorageDirectory() + "/download/";
File file = new File(PATH);
file.mkdirs();
File outputFile = new File(file, "MyApp.apk");
if (outputFile.exists()) {
outputFile.delete();
}
FileOutputStream fos = new FileOutputStream(outputFile);
InputStream is = c.getInputStream();
byte buffer = new byte[1024];
int len1 = 0;
while ((len1 = is.read(buffer)) != -1) {
fos.write(buffer, 0, len1);
}
fos.close();
is.close();
saveResult = "";
// initiate view of new apk so user can choose to install it
Intent intent = new Intent(Intent.ACTION_VIEW);
intent.setDataAndType(Uri.fromFile(new File(Environment.getExternalStorageDirectory() + "/download/" + "MyApp.apk")), "application/vnd.android.package-archive");
intent.setFlags(Intent.FLAG_ACTIVITY_NEW_TASK);
context.startActivity(intent);
}
catch (Exception e) {
saveResult = "Error occurred while downloading update. Please login again to retry download.";
}
return null;
}
@Override
protected void onPostExecute(Void result) {
GeneralUtils.dismissWithCheck(this.dialog);
if (saveResult.length() > 0) {
displayAlertMessage(saveResult);
}
super.onPostExecute(result);
}
@Override
protected void onPreExecute() {
super.onPreExecute();
if (this.dialog != null) {
this.dialog.setMessage("Downloading Update. Please wait..");
this.dialog.show();
}
}
}
After Android 8, this code is no longer working. The download completes, the "for your security, your phone is not allowed to install unknown apps from this source dialog displays, I go to Settings to toggle "Allow from this source" on, I touch the INSTALL button, the install starts and then it ends unsuccessfully with the nondescript error "App Not Installed" as seen below.
(https://imgur.com/a/0lm7Kvp)
(https://imgur.com/a/0lm7Kvp)
(https://imgur.com/a/0lm7Kvp)
(https://imgur.com/a/0lm7Kvp)
I've tried portions of the solution in this post How to manage installation from Unknown Sources in Android Oreo? but I cannot target Android 26 (version 8), as some of my users do not have devices running Android 8 yet. I did test this solution by targeting 26, but even when the user toggles unknown sources on for the apk, the installation is still unsuccessful.
My initial problem is that my app contains the code above, so there is no way that it will be able to update itself, since that code no longer works in Android 8, no matter what settings regarding unknown sources are toggled. I will have to ask my users to uninstall the app, and install the new version from the server. The second problem is that I need to modify the code for the new version, so that in the future, it can update itself. My question is, what code, permissions, or device settings changes must be made to have my app successfully update itself in the future?
BTW, uninstalling the current version of the app, and linking to the apk file for the new version in Chrome, which downloads it and initiates the install, works successfully.
Changes I have tried, that were unsuccessful:
adding this to the manifest:
<uses-permission android:name="android.permission.REQUEST_INSTALL_PACKAGES"/>
linking to the apk location in Chrome to see if it would initiate the download/install because then the apk file would be in the official "downloads" folder and possibly cause install to work
using FileProvider to install the package:
Uri apkUri = FileProvider.getUriForFile(MyActivity.this, "myapp.provider", outputFile);
Intent intent = new Intent(Intent.ACTION_INSTALL_PACKAGE);
intent.setDataAndType(apkUri, "application/vnd.android.package-archive");
intent.setFlags(Intent.FLAG_GRANT_READ_URI_PERMISSION);
MyActivity.this.startActivity(intent);
Specifically requesting permission to Manifest.permission.REQUEST_INSTALL_PACKAGES using ActivityCompat.shouldShowRequestPermissionRationale. In this case, the permission was not asked of the user, since the result of the method call was false. Even with the android.permission.REQUEST_INSTALL_PACKAGES permission in the manifest, the result of calling ContextCompat.checkSelfPermission was not PackageManager.PERMISSION_GRANTED.
Generating the signed apk in both V1 (Jar Signature) and V2 (Full APK Signature)
Various results, either with zero visible feedback on the device, or with errors similar to the one described above were produced by the steps outlined above, all of which with no success.
There are so many posts regarding this situation, all of which contained no success when I tried them, either in total or piecemeal. The app cannot be put in Google Play Store, as it should not be visible to the public or even stored in a cloud for security reasons, so I need a solution of the "unknown sources" variety that works.
Any suggestions/solutions would be greatly appreciated.
java

java

edited Nov 21 at 18:52
asked Nov 21 at 18:47


dpspae03
114
114
Sounds like the APK you're getting from the server is malformed somehow. Are you compiling the app in the normal way?
– Sub 6 Resources
Nov 21 at 18:56
"but I cannot target Android 26 (version 8), as some of my users do not have devices running Android 8 yet" setting atargetSdkVersion
of 26 or higher does not prevent you from having aminSdkVersion
that is lower than 26. That's why they are separate settings. "My question is, what code, permissions, or device settings changes must be made to have my app successfully update itself in the future?" -- that's covered in the SO question that you linked to. "using FileProvider to install the package" -- that is definitely a requirement.
– CommonsWare
Nov 21 at 22:20
"Specifically requesting permission to Manifest.permission.REQUEST_INSTALL_PACKAGES using ActivityCompat.shouldShowRequestPermissionRationale" -- that is not how you request runtime permissions. AndREQUEST_INSTALL_PACKAGES
does not work that way anyway. Its use is covered in the SO question that you linked to, leading the user to the proper Settings screen for this. Overall, though, a Minimal, Complete, and Verifiable example showing what you tried and what your specific results were would increase the chances that somebody can help you.
– CommonsWare
Nov 21 at 22:24
add a comment |
Sounds like the APK you're getting from the server is malformed somehow. Are you compiling the app in the normal way?
– Sub 6 Resources
Nov 21 at 18:56
"but I cannot target Android 26 (version 8), as some of my users do not have devices running Android 8 yet" setting atargetSdkVersion
of 26 or higher does not prevent you from having aminSdkVersion
that is lower than 26. That's why they are separate settings. "My question is, what code, permissions, or device settings changes must be made to have my app successfully update itself in the future?" -- that's covered in the SO question that you linked to. "using FileProvider to install the package" -- that is definitely a requirement.
– CommonsWare
Nov 21 at 22:20
"Specifically requesting permission to Manifest.permission.REQUEST_INSTALL_PACKAGES using ActivityCompat.shouldShowRequestPermissionRationale" -- that is not how you request runtime permissions. AndREQUEST_INSTALL_PACKAGES
does not work that way anyway. Its use is covered in the SO question that you linked to, leading the user to the proper Settings screen for this. Overall, though, a Minimal, Complete, and Verifiable example showing what you tried and what your specific results were would increase the chances that somebody can help you.
– CommonsWare
Nov 21 at 22:24
Sounds like the APK you're getting from the server is malformed somehow. Are you compiling the app in the normal way?
– Sub 6 Resources
Nov 21 at 18:56
Sounds like the APK you're getting from the server is malformed somehow. Are you compiling the app in the normal way?
– Sub 6 Resources
Nov 21 at 18:56
"but I cannot target Android 26 (version 8), as some of my users do not have devices running Android 8 yet" setting a
targetSdkVersion
of 26 or higher does not prevent you from having a minSdkVersion
that is lower than 26. That's why they are separate settings. "My question is, what code, permissions, or device settings changes must be made to have my app successfully update itself in the future?" -- that's covered in the SO question that you linked to. "using FileProvider to install the package" -- that is definitely a requirement.– CommonsWare
Nov 21 at 22:20
"but I cannot target Android 26 (version 8), as some of my users do not have devices running Android 8 yet" setting a
targetSdkVersion
of 26 or higher does not prevent you from having a minSdkVersion
that is lower than 26. That's why they are separate settings. "My question is, what code, permissions, or device settings changes must be made to have my app successfully update itself in the future?" -- that's covered in the SO question that you linked to. "using FileProvider to install the package" -- that is definitely a requirement.– CommonsWare
Nov 21 at 22:20
"Specifically requesting permission to Manifest.permission.REQUEST_INSTALL_PACKAGES using ActivityCompat.shouldShowRequestPermissionRationale" -- that is not how you request runtime permissions. And
REQUEST_INSTALL_PACKAGES
does not work that way anyway. Its use is covered in the SO question that you linked to, leading the user to the proper Settings screen for this. Overall, though, a Minimal, Complete, and Verifiable example showing what you tried and what your specific results were would increase the chances that somebody can help you.– CommonsWare
Nov 21 at 22:24
"Specifically requesting permission to Manifest.permission.REQUEST_INSTALL_PACKAGES using ActivityCompat.shouldShowRequestPermissionRationale" -- that is not how you request runtime permissions. And
REQUEST_INSTALL_PACKAGES
does not work that way anyway. Its use is covered in the SO question that you linked to, leading the user to the proper Settings screen for this. Overall, though, a Minimal, Complete, and Verifiable example showing what you tried and what your specific results were would increase the chances that somebody can help you.– CommonsWare
Nov 21 at 22:24
add a comment |
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53418723%2fself-updating-app-wont-install-update-anymore%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
29Y86RF48KzVTUCYtwdj8K6q9KmlJ2K3J OxFADEsHZrfw3K rx,cg0Fj,CS 7ZNoo
Sounds like the APK you're getting from the server is malformed somehow. Are you compiling the app in the normal way?
– Sub 6 Resources
Nov 21 at 18:56
"but I cannot target Android 26 (version 8), as some of my users do not have devices running Android 8 yet" setting a
targetSdkVersion
of 26 or higher does not prevent you from having aminSdkVersion
that is lower than 26. That's why they are separate settings. "My question is, what code, permissions, or device settings changes must be made to have my app successfully update itself in the future?" -- that's covered in the SO question that you linked to. "using FileProvider to install the package" -- that is definitely a requirement.– CommonsWare
Nov 21 at 22:20
"Specifically requesting permission to Manifest.permission.REQUEST_INSTALL_PACKAGES using ActivityCompat.shouldShowRequestPermissionRationale" -- that is not how you request runtime permissions. And
REQUEST_INSTALL_PACKAGES
does not work that way anyway. Its use is covered in the SO question that you linked to, leading the user to the proper Settings screen for this. Overall, though, a Minimal, Complete, and Verifiable example showing what you tried and what your specific results were would increase the chances that somebody can help you.– CommonsWare
Nov 21 at 22:24