Can't change value of class
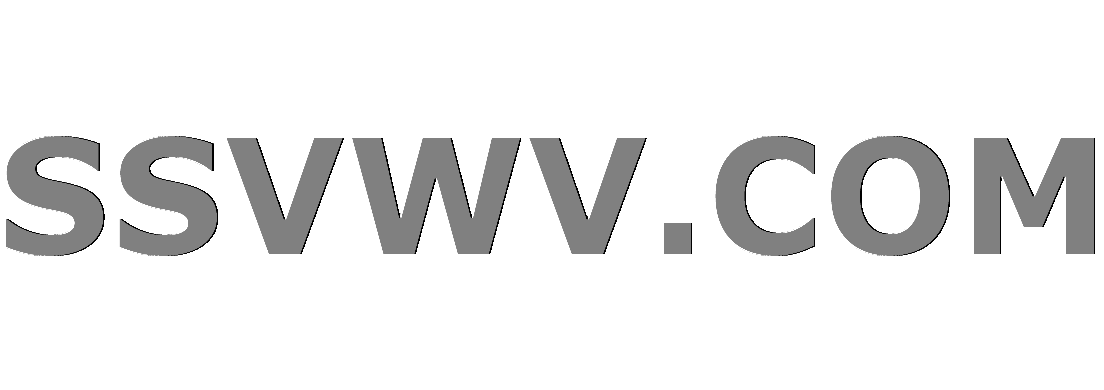
Multi tool use
up vote
1
down vote
favorite
I have the following Python code:
class Characters():
default_player_hp = 100.0
default_enemy_hp = 100.0
class Player:
def __init__(self):
self.player_health = Characters().default_player_hp
def update(self):
self.player_health = Characters().default_player_hp
class Enemy:
def __init__(self):
self.enemy_health = Characters().default_enemy_hp
def update(self):
self.enemy_health = Characters().default_enemy_hp
characters = Characters()
player = characters.Player()
while (True):
characters.default_enemy_hp = round(characters.default_enemy_hp * float(input()) / 10, 3)
enemy = characters.Enemy()
enemy.update()
print(characters.default_enemy_hp, enemy.enemy_health)
The problem is it doesn't change enemy_hp variable (always prints [default_enemy_hp] 100.0).
python
add a comment |
up vote
1
down vote
favorite
I have the following Python code:
class Characters():
default_player_hp = 100.0
default_enemy_hp = 100.0
class Player:
def __init__(self):
self.player_health = Characters().default_player_hp
def update(self):
self.player_health = Characters().default_player_hp
class Enemy:
def __init__(self):
self.enemy_health = Characters().default_enemy_hp
def update(self):
self.enemy_health = Characters().default_enemy_hp
characters = Characters()
player = characters.Player()
while (True):
characters.default_enemy_hp = round(characters.default_enemy_hp * float(input()) / 10, 3)
enemy = characters.Enemy()
enemy.update()
print(characters.default_enemy_hp, enemy.enemy_health)
The problem is it doesn't change enemy_hp variable (always prints [default_enemy_hp] 100.0).
python
3
Why are the classes nested, anyway?
– timgeb
Nov 21 at 18:54
Because why not? Anyway, it doesn't change anything. I've tried.
– Shiney
Nov 21 at 18:58
1
I think you're confusing class variables and instance variables. Try reading digitalocean.com/community/tutorials/…
– Fred Larson
Nov 21 at 19:21
add a comment |
up vote
1
down vote
favorite
up vote
1
down vote
favorite
I have the following Python code:
class Characters():
default_player_hp = 100.0
default_enemy_hp = 100.0
class Player:
def __init__(self):
self.player_health = Characters().default_player_hp
def update(self):
self.player_health = Characters().default_player_hp
class Enemy:
def __init__(self):
self.enemy_health = Characters().default_enemy_hp
def update(self):
self.enemy_health = Characters().default_enemy_hp
characters = Characters()
player = characters.Player()
while (True):
characters.default_enemy_hp = round(characters.default_enemy_hp * float(input()) / 10, 3)
enemy = characters.Enemy()
enemy.update()
print(characters.default_enemy_hp, enemy.enemy_health)
The problem is it doesn't change enemy_hp variable (always prints [default_enemy_hp] 100.0).
python
I have the following Python code:
class Characters():
default_player_hp = 100.0
default_enemy_hp = 100.0
class Player:
def __init__(self):
self.player_health = Characters().default_player_hp
def update(self):
self.player_health = Characters().default_player_hp
class Enemy:
def __init__(self):
self.enemy_health = Characters().default_enemy_hp
def update(self):
self.enemy_health = Characters().default_enemy_hp
characters = Characters()
player = characters.Player()
while (True):
characters.default_enemy_hp = round(characters.default_enemy_hp * float(input()) / 10, 3)
enemy = characters.Enemy()
enemy.update()
print(characters.default_enemy_hp, enemy.enemy_health)
The problem is it doesn't change enemy_hp variable (always prints [default_enemy_hp] 100.0).
python
python
asked Nov 21 at 18:51
Shiney
63
63
3
Why are the classes nested, anyway?
– timgeb
Nov 21 at 18:54
Because why not? Anyway, it doesn't change anything. I've tried.
– Shiney
Nov 21 at 18:58
1
I think you're confusing class variables and instance variables. Try reading digitalocean.com/community/tutorials/…
– Fred Larson
Nov 21 at 19:21
add a comment |
3
Why are the classes nested, anyway?
– timgeb
Nov 21 at 18:54
Because why not? Anyway, it doesn't change anything. I've tried.
– Shiney
Nov 21 at 18:58
1
I think you're confusing class variables and instance variables. Try reading digitalocean.com/community/tutorials/…
– Fred Larson
Nov 21 at 19:21
3
3
Why are the classes nested, anyway?
– timgeb
Nov 21 at 18:54
Why are the classes nested, anyway?
– timgeb
Nov 21 at 18:54
Because why not? Anyway, it doesn't change anything. I've tried.
– Shiney
Nov 21 at 18:58
Because why not? Anyway, it doesn't change anything. I've tried.
– Shiney
Nov 21 at 18:58
1
1
I think you're confusing class variables and instance variables. Try reading digitalocean.com/community/tutorials/…
– Fred Larson
Nov 21 at 19:21
I think you're confusing class variables and instance variables. Try reading digitalocean.com/community/tutorials/…
– Fred Larson
Nov 21 at 19:21
add a comment |
1 Answer
1
active
oldest
votes
up vote
0
down vote
This snippet:
def update(self):
self.enemy_health = Characters().default_enemy_hp
makes a new (anonymous) Character
object, and sets the current enemy_health
attribute of the given Enemy
object to 100
. That's because new Character
's always start with 100
health.
This:
characters.default_enemy_hp = round(characters.default_enemy_hp * float(input()) / 10, 3)
is therefore irrelevant, as enemy.update()
will create a new Character
object (it will not use the Character
object modified above), and just use that new Character
object's health (i.e. 100
).
If you want to actually update the health, don't work so hard, and just pass in a value and be done with it:
def update(self, new_health):
self.enemy_health = new_health
Usage:
enemy.update(new_health=15) # or new_health=round(float(input()) / 10, 3), whatever you want.
print(enemy.enemy_health) # outputs 15, as desired
HTH.
P.S. You've got other issues with your code, mostly having to do with creating new Character
objects (similar problems to the update
issue you've asked about), but this answer only focuses on why update
is not working as expected.
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
This snippet:
def update(self):
self.enemy_health = Characters().default_enemy_hp
makes a new (anonymous) Character
object, and sets the current enemy_health
attribute of the given Enemy
object to 100
. That's because new Character
's always start with 100
health.
This:
characters.default_enemy_hp = round(characters.default_enemy_hp * float(input()) / 10, 3)
is therefore irrelevant, as enemy.update()
will create a new Character
object (it will not use the Character
object modified above), and just use that new Character
object's health (i.e. 100
).
If you want to actually update the health, don't work so hard, and just pass in a value and be done with it:
def update(self, new_health):
self.enemy_health = new_health
Usage:
enemy.update(new_health=15) # or new_health=round(float(input()) / 10, 3), whatever you want.
print(enemy.enemy_health) # outputs 15, as desired
HTH.
P.S. You've got other issues with your code, mostly having to do with creating new Character
objects (similar problems to the update
issue you've asked about), but this answer only focuses on why update
is not working as expected.
add a comment |
up vote
0
down vote
This snippet:
def update(self):
self.enemy_health = Characters().default_enemy_hp
makes a new (anonymous) Character
object, and sets the current enemy_health
attribute of the given Enemy
object to 100
. That's because new Character
's always start with 100
health.
This:
characters.default_enemy_hp = round(characters.default_enemy_hp * float(input()) / 10, 3)
is therefore irrelevant, as enemy.update()
will create a new Character
object (it will not use the Character
object modified above), and just use that new Character
object's health (i.e. 100
).
If you want to actually update the health, don't work so hard, and just pass in a value and be done with it:
def update(self, new_health):
self.enemy_health = new_health
Usage:
enemy.update(new_health=15) # or new_health=round(float(input()) / 10, 3), whatever you want.
print(enemy.enemy_health) # outputs 15, as desired
HTH.
P.S. You've got other issues with your code, mostly having to do with creating new Character
objects (similar problems to the update
issue you've asked about), but this answer only focuses on why update
is not working as expected.
add a comment |
up vote
0
down vote
up vote
0
down vote
This snippet:
def update(self):
self.enemy_health = Characters().default_enemy_hp
makes a new (anonymous) Character
object, and sets the current enemy_health
attribute of the given Enemy
object to 100
. That's because new Character
's always start with 100
health.
This:
characters.default_enemy_hp = round(characters.default_enemy_hp * float(input()) / 10, 3)
is therefore irrelevant, as enemy.update()
will create a new Character
object (it will not use the Character
object modified above), and just use that new Character
object's health (i.e. 100
).
If you want to actually update the health, don't work so hard, and just pass in a value and be done with it:
def update(self, new_health):
self.enemy_health = new_health
Usage:
enemy.update(new_health=15) # or new_health=round(float(input()) / 10, 3), whatever you want.
print(enemy.enemy_health) # outputs 15, as desired
HTH.
P.S. You've got other issues with your code, mostly having to do with creating new Character
objects (similar problems to the update
issue you've asked about), but this answer only focuses on why update
is not working as expected.
This snippet:
def update(self):
self.enemy_health = Characters().default_enemy_hp
makes a new (anonymous) Character
object, and sets the current enemy_health
attribute of the given Enemy
object to 100
. That's because new Character
's always start with 100
health.
This:
characters.default_enemy_hp = round(characters.default_enemy_hp * float(input()) / 10, 3)
is therefore irrelevant, as enemy.update()
will create a new Character
object (it will not use the Character
object modified above), and just use that new Character
object's health (i.e. 100
).
If you want to actually update the health, don't work so hard, and just pass in a value and be done with it:
def update(self, new_health):
self.enemy_health = new_health
Usage:
enemy.update(new_health=15) # or new_health=round(float(input()) / 10, 3), whatever you want.
print(enemy.enemy_health) # outputs 15, as desired
HTH.
P.S. You've got other issues with your code, mostly having to do with creating new Character
objects (similar problems to the update
issue you've asked about), but this answer only focuses on why update
is not working as expected.
answered Nov 21 at 19:20
Matt Messersmith
5,64521729
5,64521729
add a comment |
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53418790%2fcant-change-value-of-class%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
4dCzhyxsKhVsd86dX0 IGcIug3EcO1EOgZ 4pAW kmg,MH1xP
3
Why are the classes nested, anyway?
– timgeb
Nov 21 at 18:54
Because why not? Anyway, it doesn't change anything. I've tried.
– Shiney
Nov 21 at 18:58
1
I think you're confusing class variables and instance variables. Try reading digitalocean.com/community/tutorials/…
– Fred Larson
Nov 21 at 19:21