Caesar Cipher Frequency Analysis in Java
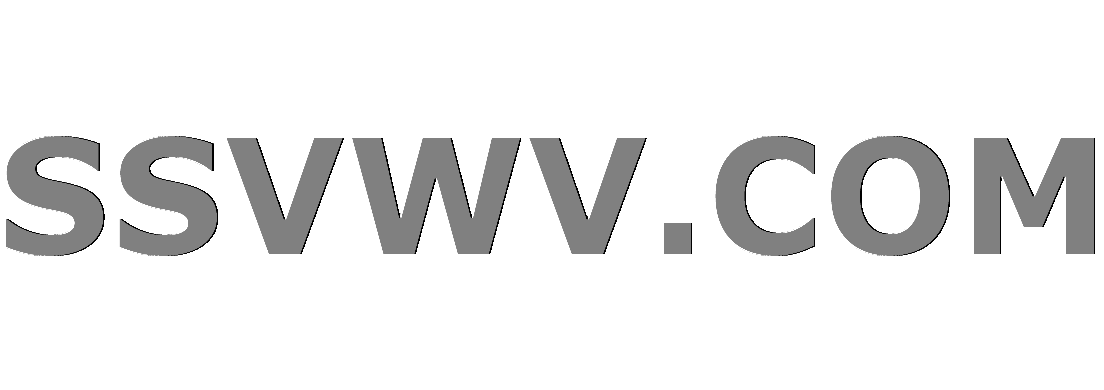
Multi tool use
up vote
0
down vote
favorite
Caesar Cipher using Frequency Analysis** in Java: this is my code for the decode part:
public static String decode (String code){
int key=0;
final int ALPHABET_SIZE = 26;
int freqs = new int[ALPHABET_SIZE];
for (int l=0;l<freqs.length;l++){
freqs[l]=0;
}
for (int k=0;k<code.length();k++){
if (code.charAt(k)>='a' && code.charAt(k)<='z'){
freqs[code.charAt(k)-'a']++;
}
}
int biggest = 0;
for (int t=0;t<freqs.length;t++){
if (freqs[t]>biggest){
biggest= t;
}
}
if (biggest<4){
key = (biggest + 26 - ('e'+'a'));
}
else{
key = biggest + 'a' - 'e';
}
return (decode(code,key));
}
I cannot use maps, imports, lists, or add a key, I do know that the most freq letter is E but I do not know how to implement it in a different function. I would appreciate a more elegant solution, thank you. ** Frequency Analysis
java caesar-cipher frequency-analysis
add a comment |
up vote
0
down vote
favorite
Caesar Cipher using Frequency Analysis** in Java: this is my code for the decode part:
public static String decode (String code){
int key=0;
final int ALPHABET_SIZE = 26;
int freqs = new int[ALPHABET_SIZE];
for (int l=0;l<freqs.length;l++){
freqs[l]=0;
}
for (int k=0;k<code.length();k++){
if (code.charAt(k)>='a' && code.charAt(k)<='z'){
freqs[code.charAt(k)-'a']++;
}
}
int biggest = 0;
for (int t=0;t<freqs.length;t++){
if (freqs[t]>biggest){
biggest= t;
}
}
if (biggest<4){
key = (biggest + 26 - ('e'+'a'));
}
else{
key = biggest + 'a' - 'e';
}
return (decode(code,key));
}
I cannot use maps, imports, lists, or add a key, I do know that the most freq letter is E but I do not know how to implement it in a different function. I would appreciate a more elegant solution, thank you. ** Frequency Analysis
java caesar-cipher frequency-analysis
A more elegant solution would use all the Java objects you have it's an oriented object language so a better solution would use it
– azro
Nov 22 at 15:21
Letter frequencies: ETAOINSHRDLU... though [space] is more frequent than E.
– rossum
Nov 22 at 16:23
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
Caesar Cipher using Frequency Analysis** in Java: this is my code for the decode part:
public static String decode (String code){
int key=0;
final int ALPHABET_SIZE = 26;
int freqs = new int[ALPHABET_SIZE];
for (int l=0;l<freqs.length;l++){
freqs[l]=0;
}
for (int k=0;k<code.length();k++){
if (code.charAt(k)>='a' && code.charAt(k)<='z'){
freqs[code.charAt(k)-'a']++;
}
}
int biggest = 0;
for (int t=0;t<freqs.length;t++){
if (freqs[t]>biggest){
biggest= t;
}
}
if (biggest<4){
key = (biggest + 26 - ('e'+'a'));
}
else{
key = biggest + 'a' - 'e';
}
return (decode(code,key));
}
I cannot use maps, imports, lists, or add a key, I do know that the most freq letter is E but I do not know how to implement it in a different function. I would appreciate a more elegant solution, thank you. ** Frequency Analysis
java caesar-cipher frequency-analysis
Caesar Cipher using Frequency Analysis** in Java: this is my code for the decode part:
public static String decode (String code){
int key=0;
final int ALPHABET_SIZE = 26;
int freqs = new int[ALPHABET_SIZE];
for (int l=0;l<freqs.length;l++){
freqs[l]=0;
}
for (int k=0;k<code.length();k++){
if (code.charAt(k)>='a' && code.charAt(k)<='z'){
freqs[code.charAt(k)-'a']++;
}
}
int biggest = 0;
for (int t=0;t<freqs.length;t++){
if (freqs[t]>biggest){
biggest= t;
}
}
if (biggest<4){
key = (biggest + 26 - ('e'+'a'));
}
else{
key = biggest + 'a' - 'e';
}
return (decode(code,key));
}
I cannot use maps, imports, lists, or add a key, I do know that the most freq letter is E but I do not know how to implement it in a different function. I would appreciate a more elegant solution, thank you. ** Frequency Analysis
java caesar-cipher frequency-analysis
java caesar-cipher frequency-analysis
asked Nov 22 at 15:10
Yuki1112
586
586
A more elegant solution would use all the Java objects you have it's an oriented object language so a better solution would use it
– azro
Nov 22 at 15:21
Letter frequencies: ETAOINSHRDLU... though [space] is more frequent than E.
– rossum
Nov 22 at 16:23
add a comment |
A more elegant solution would use all the Java objects you have it's an oriented object language so a better solution would use it
– azro
Nov 22 at 15:21
Letter frequencies: ETAOINSHRDLU... though [space] is more frequent than E.
– rossum
Nov 22 at 16:23
A more elegant solution would use all the Java objects you have it's an oriented object language so a better solution would use it
– azro
Nov 22 at 15:21
A more elegant solution would use all the Java objects you have it's an oriented object language so a better solution would use it
– azro
Nov 22 at 15:21
Letter frequencies: ETAOINSHRDLU... though [space] is more frequent than E.
– rossum
Nov 22 at 16:23
Letter frequencies: ETAOINSHRDLU... though [space] is more frequent than E.
– rossum
Nov 22 at 16:23
add a comment |
1 Answer
1
active
oldest
votes
up vote
0
down vote
I am not too sure what your definition of "elegant" is, but your solution looks fine in general.
A few comments:
You don't actually have to manually initialise your array to 0 at the start since Java will do that for you
Looking for the letter with the highest frequency can be done while you are counting the characters
For example, the for
loop can become:
int highestFreq = 0;
int highestFreqIdx = -1;
for (int k=0; k < code.length(); k++) {
if (code.charAt(k) >= 'a' && code.charAt(k) <= 'z') {
int count = freqs[code.charAt(k)-'a']++;
if (count > highestFreq) {
highestFreq = count;
highestFreqIdx = k;
}
}
}
- Calculating the key can be simplified
If I am not wrong, your key is the number of positions that the letter with the highest frequency is away from 'e'. In this case, you can simply do this:
key = biggest - ('e' - 'a');
So your code becomes:
public static String decode (String code){
int key = 0;
final int ALPHABET_SIZE = 26;
int freqs = new int[ALPHABET_SIZE];
int highestFreq = 0;
int highestFreqIdx = -1;
for (int k=0; k < code.length(); k++) {
if (code.charAt(k) >= 'a' && code.charAt(k) <= 'z') {
int count = freqs[code.charAt(k)-'a']++;
if (count > highestFreq) {
highestFreq = count;
highestFreqIdx = k;
}
}
}
key = highestFreqIdx - ('e' - 'a'); // Can also directly use 4 instead of 'e' - 'a'
return (decode(code, key));
}
That said, your code will only work for messages that really have the letter 'e' as the most common letter...
P/S Such code review questions may be better off in this Stack Exchange site for code reviews instead
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
I am not too sure what your definition of "elegant" is, but your solution looks fine in general.
A few comments:
You don't actually have to manually initialise your array to 0 at the start since Java will do that for you
Looking for the letter with the highest frequency can be done while you are counting the characters
For example, the for
loop can become:
int highestFreq = 0;
int highestFreqIdx = -1;
for (int k=0; k < code.length(); k++) {
if (code.charAt(k) >= 'a' && code.charAt(k) <= 'z') {
int count = freqs[code.charAt(k)-'a']++;
if (count > highestFreq) {
highestFreq = count;
highestFreqIdx = k;
}
}
}
- Calculating the key can be simplified
If I am not wrong, your key is the number of positions that the letter with the highest frequency is away from 'e'. In this case, you can simply do this:
key = biggest - ('e' - 'a');
So your code becomes:
public static String decode (String code){
int key = 0;
final int ALPHABET_SIZE = 26;
int freqs = new int[ALPHABET_SIZE];
int highestFreq = 0;
int highestFreqIdx = -1;
for (int k=0; k < code.length(); k++) {
if (code.charAt(k) >= 'a' && code.charAt(k) <= 'z') {
int count = freqs[code.charAt(k)-'a']++;
if (count > highestFreq) {
highestFreq = count;
highestFreqIdx = k;
}
}
}
key = highestFreqIdx - ('e' - 'a'); // Can also directly use 4 instead of 'e' - 'a'
return (decode(code, key));
}
That said, your code will only work for messages that really have the letter 'e' as the most common letter...
P/S Such code review questions may be better off in this Stack Exchange site for code reviews instead
add a comment |
up vote
0
down vote
I am not too sure what your definition of "elegant" is, but your solution looks fine in general.
A few comments:
You don't actually have to manually initialise your array to 0 at the start since Java will do that for you
Looking for the letter with the highest frequency can be done while you are counting the characters
For example, the for
loop can become:
int highestFreq = 0;
int highestFreqIdx = -1;
for (int k=0; k < code.length(); k++) {
if (code.charAt(k) >= 'a' && code.charAt(k) <= 'z') {
int count = freqs[code.charAt(k)-'a']++;
if (count > highestFreq) {
highestFreq = count;
highestFreqIdx = k;
}
}
}
- Calculating the key can be simplified
If I am not wrong, your key is the number of positions that the letter with the highest frequency is away from 'e'. In this case, you can simply do this:
key = biggest - ('e' - 'a');
So your code becomes:
public static String decode (String code){
int key = 0;
final int ALPHABET_SIZE = 26;
int freqs = new int[ALPHABET_SIZE];
int highestFreq = 0;
int highestFreqIdx = -1;
for (int k=0; k < code.length(); k++) {
if (code.charAt(k) >= 'a' && code.charAt(k) <= 'z') {
int count = freqs[code.charAt(k)-'a']++;
if (count > highestFreq) {
highestFreq = count;
highestFreqIdx = k;
}
}
}
key = highestFreqIdx - ('e' - 'a'); // Can also directly use 4 instead of 'e' - 'a'
return (decode(code, key));
}
That said, your code will only work for messages that really have the letter 'e' as the most common letter...
P/S Such code review questions may be better off in this Stack Exchange site for code reviews instead
add a comment |
up vote
0
down vote
up vote
0
down vote
I am not too sure what your definition of "elegant" is, but your solution looks fine in general.
A few comments:
You don't actually have to manually initialise your array to 0 at the start since Java will do that for you
Looking for the letter with the highest frequency can be done while you are counting the characters
For example, the for
loop can become:
int highestFreq = 0;
int highestFreqIdx = -1;
for (int k=0; k < code.length(); k++) {
if (code.charAt(k) >= 'a' && code.charAt(k) <= 'z') {
int count = freqs[code.charAt(k)-'a']++;
if (count > highestFreq) {
highestFreq = count;
highestFreqIdx = k;
}
}
}
- Calculating the key can be simplified
If I am not wrong, your key is the number of positions that the letter with the highest frequency is away from 'e'. In this case, you can simply do this:
key = biggest - ('e' - 'a');
So your code becomes:
public static String decode (String code){
int key = 0;
final int ALPHABET_SIZE = 26;
int freqs = new int[ALPHABET_SIZE];
int highestFreq = 0;
int highestFreqIdx = -1;
for (int k=0; k < code.length(); k++) {
if (code.charAt(k) >= 'a' && code.charAt(k) <= 'z') {
int count = freqs[code.charAt(k)-'a']++;
if (count > highestFreq) {
highestFreq = count;
highestFreqIdx = k;
}
}
}
key = highestFreqIdx - ('e' - 'a'); // Can also directly use 4 instead of 'e' - 'a'
return (decode(code, key));
}
That said, your code will only work for messages that really have the letter 'e' as the most common letter...
P/S Such code review questions may be better off in this Stack Exchange site for code reviews instead
I am not too sure what your definition of "elegant" is, but your solution looks fine in general.
A few comments:
You don't actually have to manually initialise your array to 0 at the start since Java will do that for you
Looking for the letter with the highest frequency can be done while you are counting the characters
For example, the for
loop can become:
int highestFreq = 0;
int highestFreqIdx = -1;
for (int k=0; k < code.length(); k++) {
if (code.charAt(k) >= 'a' && code.charAt(k) <= 'z') {
int count = freqs[code.charAt(k)-'a']++;
if (count > highestFreq) {
highestFreq = count;
highestFreqIdx = k;
}
}
}
- Calculating the key can be simplified
If I am not wrong, your key is the number of positions that the letter with the highest frequency is away from 'e'. In this case, you can simply do this:
key = biggest - ('e' - 'a');
So your code becomes:
public static String decode (String code){
int key = 0;
final int ALPHABET_SIZE = 26;
int freqs = new int[ALPHABET_SIZE];
int highestFreq = 0;
int highestFreqIdx = -1;
for (int k=0; k < code.length(); k++) {
if (code.charAt(k) >= 'a' && code.charAt(k) <= 'z') {
int count = freqs[code.charAt(k)-'a']++;
if (count > highestFreq) {
highestFreq = count;
highestFreqIdx = k;
}
}
}
key = highestFreqIdx - ('e' - 'a'); // Can also directly use 4 instead of 'e' - 'a'
return (decode(code, key));
}
That said, your code will only work for messages that really have the letter 'e' as the most common letter...
P/S Such code review questions may be better off in this Stack Exchange site for code reviews instead
answered Nov 22 at 16:13
umop apisdn
342513
342513
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53433812%2fcaesar-cipher-frequency-analysis-in-java%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
tRPJ,kKh1zscbk Zm KItjBA9VR,wQpUJ6Bk,E
A more elegant solution would use all the Java objects you have it's an oriented object language so a better solution would use it
– azro
Nov 22 at 15:21
Letter frequencies: ETAOINSHRDLU... though [space] is more frequent than E.
– rossum
Nov 22 at 16:23