Tables with rounded corners
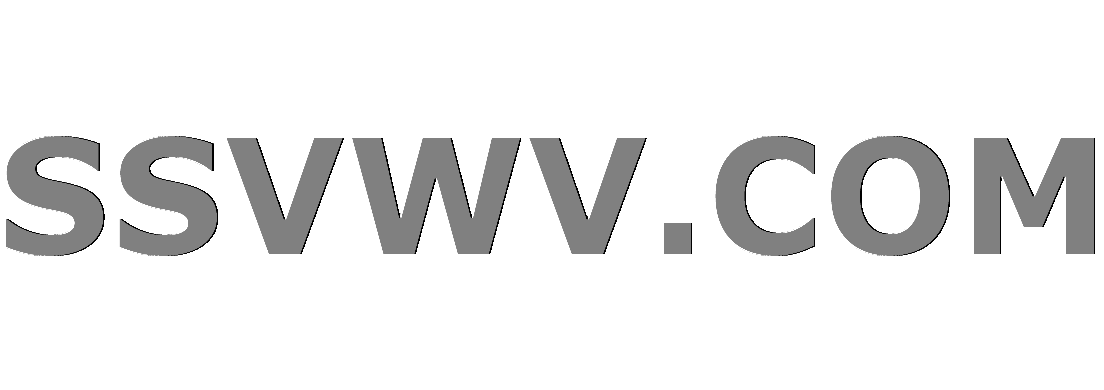
Multi tool use
I was wondering what would be the best approach for creating tables in a pdf that have rounded corners using the iTextSharp 5.x+ library.
itextsharp
add a comment |
I was wondering what would be the best approach for creating tables in a pdf that have rounded corners using the iTextSharp 5.x+ library.
itextsharp
add a comment |
I was wondering what would be the best approach for creating tables in a pdf that have rounded corners using the iTextSharp 5.x+ library.
itextsharp
I was wondering what would be the best approach for creating tables in a pdf that have rounded corners using the iTextSharp 5.x+ library.
itextsharp
itextsharp
edited Apr 16 '13 at 17:52


Bobrovsky
8,6751758105
8,6751758105
asked Mar 31 '10 at 23:33
Rick Make
2561514
2561514
add a comment |
add a comment |
4 Answers
4
active
oldest
votes
If you have iTextSharp source code, add the following to PdfContentByte
class:
/// <summary>
/// Enumuration for defining corners you want rounded.
/// </summary>
[Flags()]
public enum Corners {None = 0,All=15,Top=3,Bottom=12, TopLeft = 1, TopRight=2, BottomLeft=4, BottomRight=8};
/// <summary>
/// Adds a round rectangle to the current path, with rounded conrners as specified by roundCorners.
/// </summary>
/// <param name="x"></param>
/// <param name="y"></param>
/// <param name="w"></param>
/// <param name="h"></param>
/// <param name="r"></param>
/// <param name="roundCorners"></param>
public void RoundRectangle(float x, float y, float w, float h, float r,Corners roundCorners)
{
if (w < 0)
{
x += w;
w = -w;
}
if (h < 0)
{
y += h;
h = -h;
}
if (r < 0)
r = -r;
float b = 0.4477f;
if((roundCorners & Corners.BottomLeft) == Corners.BottomLeft)
MoveTo(x + r, y);
else
MoveTo(x, y);
if ((roundCorners & Corners.BottomRight) == Corners.BottomRight)
{
LineTo(x + w - r, y);
CurveTo(x + w - r * b, y, x + w, y + r * b, x + w, y + r);
}
else
LineTo(x + w, y);
if ((roundCorners & Corners.TopRight ) == Corners.TopRight)
{
LineTo(x + w, y + h - r);
CurveTo(x + w, y + h - r * b, x + w - r * b, y + h, x + w - r, y + h);
}
else
LineTo(x + w, y + h);
if ((roundCorners & Corners.TopLeft) == Corners.TopLeft)
{
LineTo(x + r, y + h);
CurveTo(x + r * b, y + h, x, y + h - r * b, x, y + h - r);
}
else
LineTo(x , y + h);
if ((roundCorners & Corners.BottomLeft) == Corners.BottomLeft)
{
LineTo(x, y + r);
CurveTo(x, y + r * b, x + r * b, y, x + r, y);
}else
LineTo(x, y);
}
Next step is to implment IPdfPCellEvent
interface:
public class myCellEvent : IPdfPCellEvent
{
#region members
PdfContentByte.Corners _roundedCorners;
float _roundness;
#endregion
#region ctor
public myCellEvent()
:this( PdfContentByte.Corners.All,2f)
{
}
public myCellEvent(PdfContentByte.Corners roundedCorners)
: this(roundedCorners, 2f)
{
}
public myCellEvent(PdfContentByte.Corners roundedCorners,float roundness)
{
_roundedCorners = roundedCorners;
_roundness = roundness;
}
#endregion
#region IPdfPCellEvent Members
public void CellLayout(PdfPCell cell, Rectangle position, PdfContentByte canvases)
{
PdfContentByte cb = canvases[PdfPTable.LINECANVAS];
cb.RoundRectangle(position.Left, position.Bottom, position.Right - position.Left, position.Top - position.Bottom, this._roundness, this._roundedCorners);
cb.SetColorStroke(new BaseColor(System.Drawing.Color.Black));
cb.SetColorFill(new BaseColor(System.Drawing.Color.Beige));
cb.FillStroke();
cb.Stroke();
}
#endregion
}
Then you round what ever corners you want to produce the rounded table of choice:
PdfPTable table = new PdfPTable(1);
PdfPCell cell = new PdfPCell(new Phrase(10, atext, f2));
cell.Border = 0;
cell.Padding = 5f;
cell.CellEvent = new myCellEvent(PdfContentByte.Corners.Top,2f);
table.AddCell(cell);
If you like the answer ... give it a vote :)
That is great for individual cells but what about the entire table? How could implement TableCell Events to accomplish this.
– Rick Make
Apr 29 '10 at 17:53
1
Adding another table with one cell that contains my target table works. Thanks again for your answer MK.
– Rick Make
Apr 29 '10 at 18:10
1
I'am glad that you have found the answer, another approach would be by rounding one corner of each table corner cell (TopLeft, TopRight, BottomLeft and BottomRight).
– MK.
May 2 '10 at 6:56
1
Nice answer MK! Helped me out a lot! :)
– Inigoesdr
Dec 9 '10 at 20:27
@Inigoesdr It's nice to READ that :)
– MK.
Dec 16 '10 at 15:42
add a comment |
There's no way to create an iTextSharp PdfPTable
with rounded corners but what you could do is draw a table border with rounded corners using the PdfContentByte.RoundRectangle()
method.
Here's a snippet that demonstrates this:
Document doc = new Document();
using (FileStream fs = new FileStream("RoundRectangle Demo.pdf", FileMode.Create)) {
PdfWriter writer = PdfWriter.GetInstance(doc, fs);
doc.Open();
PdfContentByte cb = writer.DirectContent;
// Bottom left coordinates x & y, followed by width, height and radius of corners.
cb.RoundRectangle(100f, 500f, 200f, 200f, 2f);
cb.Stroke();
doc.Close();
}
This snippet is a condensed version of code I found in the article iTextSharp - Drawing shapes and Graphics on www.mikesdotnetting.com. You'll want to take a close look at his other articles if you haven't already.
1
That worked great. Do you know how I could find the positioning of the table so that I can accurately draw the rounded corners.
– Rick Make
Apr 3 '10 at 21:04
Simplest possible answer
– hdoghmen
Jun 17 '15 at 19:34
add a comment |
I assume that if you are wanting a table with rounded corners, you're looking for some flashy (or at least pretty) formatting on your table. If that is the case, I would recommend turning off all the borders in your iTextSharp table, and using a graphic image as the background on your page. You can then make the graphic background as intricate and fancy as you want (shading, gradients, rounded corners, etc.) with very little trouble. Then, just line up your table with the background image.
If your data format will change often, you will not want to use this approach.
add a comment |
Private Function pdfDIF() As Byte()
Dim celevent As New PdfPCellEvent
Dim tableImportes As New PdfPTable(1)
tableImportes.SetTotalWidth({10 * dpixcm})
Dim cellImportes = New PdfPCell(New Phrase("IMPORTE", COURIER9Bold))
cellImportes.BorderWidth = 0
cellImportes.HorizontalAlignment = Element.ALIGN_CENTER
cellImportes.CellEvent = celevent
tableImportes.AddCell(cellImportes)
end function
Public Class PdfPCellEvent
Implements IPdfPCellEvent
Public Sub CellLayout(cell As iTextSharp.text.pdf.PdfPCell, position As iTextSharp.text.Rectangle, canvases() As iTextSharp.text.pdf.PdfContentByte) Implements iTextSharp.text.pdf.IPdfPCellEve`enter code here`nt.CellLayout
Dim cb As PdfContentByte = canvases(PdfPTable.LINECANVAS)
cb.RoundRectangle(position.Left,position.Bottom,position.Width,position.Height,10)
cb.SetLineWidth(1)
cb.SetCMYKColorStrokeF(0.0F, 0.0F, 0.0F, 1.0F)
cb.Stroke()
End Sub
End Class
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f2557217%2ftables-with-rounded-corners%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
4 Answers
4
active
oldest
votes
4 Answers
4
active
oldest
votes
active
oldest
votes
active
oldest
votes
If you have iTextSharp source code, add the following to PdfContentByte
class:
/// <summary>
/// Enumuration for defining corners you want rounded.
/// </summary>
[Flags()]
public enum Corners {None = 0,All=15,Top=3,Bottom=12, TopLeft = 1, TopRight=2, BottomLeft=4, BottomRight=8};
/// <summary>
/// Adds a round rectangle to the current path, with rounded conrners as specified by roundCorners.
/// </summary>
/// <param name="x"></param>
/// <param name="y"></param>
/// <param name="w"></param>
/// <param name="h"></param>
/// <param name="r"></param>
/// <param name="roundCorners"></param>
public void RoundRectangle(float x, float y, float w, float h, float r,Corners roundCorners)
{
if (w < 0)
{
x += w;
w = -w;
}
if (h < 0)
{
y += h;
h = -h;
}
if (r < 0)
r = -r;
float b = 0.4477f;
if((roundCorners & Corners.BottomLeft) == Corners.BottomLeft)
MoveTo(x + r, y);
else
MoveTo(x, y);
if ((roundCorners & Corners.BottomRight) == Corners.BottomRight)
{
LineTo(x + w - r, y);
CurveTo(x + w - r * b, y, x + w, y + r * b, x + w, y + r);
}
else
LineTo(x + w, y);
if ((roundCorners & Corners.TopRight ) == Corners.TopRight)
{
LineTo(x + w, y + h - r);
CurveTo(x + w, y + h - r * b, x + w - r * b, y + h, x + w - r, y + h);
}
else
LineTo(x + w, y + h);
if ((roundCorners & Corners.TopLeft) == Corners.TopLeft)
{
LineTo(x + r, y + h);
CurveTo(x + r * b, y + h, x, y + h - r * b, x, y + h - r);
}
else
LineTo(x , y + h);
if ((roundCorners & Corners.BottomLeft) == Corners.BottomLeft)
{
LineTo(x, y + r);
CurveTo(x, y + r * b, x + r * b, y, x + r, y);
}else
LineTo(x, y);
}
Next step is to implment IPdfPCellEvent
interface:
public class myCellEvent : IPdfPCellEvent
{
#region members
PdfContentByte.Corners _roundedCorners;
float _roundness;
#endregion
#region ctor
public myCellEvent()
:this( PdfContentByte.Corners.All,2f)
{
}
public myCellEvent(PdfContentByte.Corners roundedCorners)
: this(roundedCorners, 2f)
{
}
public myCellEvent(PdfContentByte.Corners roundedCorners,float roundness)
{
_roundedCorners = roundedCorners;
_roundness = roundness;
}
#endregion
#region IPdfPCellEvent Members
public void CellLayout(PdfPCell cell, Rectangle position, PdfContentByte canvases)
{
PdfContentByte cb = canvases[PdfPTable.LINECANVAS];
cb.RoundRectangle(position.Left, position.Bottom, position.Right - position.Left, position.Top - position.Bottom, this._roundness, this._roundedCorners);
cb.SetColorStroke(new BaseColor(System.Drawing.Color.Black));
cb.SetColorFill(new BaseColor(System.Drawing.Color.Beige));
cb.FillStroke();
cb.Stroke();
}
#endregion
}
Then you round what ever corners you want to produce the rounded table of choice:
PdfPTable table = new PdfPTable(1);
PdfPCell cell = new PdfPCell(new Phrase(10, atext, f2));
cell.Border = 0;
cell.Padding = 5f;
cell.CellEvent = new myCellEvent(PdfContentByte.Corners.Top,2f);
table.AddCell(cell);
If you like the answer ... give it a vote :)
That is great for individual cells but what about the entire table? How could implement TableCell Events to accomplish this.
– Rick Make
Apr 29 '10 at 17:53
1
Adding another table with one cell that contains my target table works. Thanks again for your answer MK.
– Rick Make
Apr 29 '10 at 18:10
1
I'am glad that you have found the answer, another approach would be by rounding one corner of each table corner cell (TopLeft, TopRight, BottomLeft and BottomRight).
– MK.
May 2 '10 at 6:56
1
Nice answer MK! Helped me out a lot! :)
– Inigoesdr
Dec 9 '10 at 20:27
@Inigoesdr It's nice to READ that :)
– MK.
Dec 16 '10 at 15:42
add a comment |
If you have iTextSharp source code, add the following to PdfContentByte
class:
/// <summary>
/// Enumuration for defining corners you want rounded.
/// </summary>
[Flags()]
public enum Corners {None = 0,All=15,Top=3,Bottom=12, TopLeft = 1, TopRight=2, BottomLeft=4, BottomRight=8};
/// <summary>
/// Adds a round rectangle to the current path, with rounded conrners as specified by roundCorners.
/// </summary>
/// <param name="x"></param>
/// <param name="y"></param>
/// <param name="w"></param>
/// <param name="h"></param>
/// <param name="r"></param>
/// <param name="roundCorners"></param>
public void RoundRectangle(float x, float y, float w, float h, float r,Corners roundCorners)
{
if (w < 0)
{
x += w;
w = -w;
}
if (h < 0)
{
y += h;
h = -h;
}
if (r < 0)
r = -r;
float b = 0.4477f;
if((roundCorners & Corners.BottomLeft) == Corners.BottomLeft)
MoveTo(x + r, y);
else
MoveTo(x, y);
if ((roundCorners & Corners.BottomRight) == Corners.BottomRight)
{
LineTo(x + w - r, y);
CurveTo(x + w - r * b, y, x + w, y + r * b, x + w, y + r);
}
else
LineTo(x + w, y);
if ((roundCorners & Corners.TopRight ) == Corners.TopRight)
{
LineTo(x + w, y + h - r);
CurveTo(x + w, y + h - r * b, x + w - r * b, y + h, x + w - r, y + h);
}
else
LineTo(x + w, y + h);
if ((roundCorners & Corners.TopLeft) == Corners.TopLeft)
{
LineTo(x + r, y + h);
CurveTo(x + r * b, y + h, x, y + h - r * b, x, y + h - r);
}
else
LineTo(x , y + h);
if ((roundCorners & Corners.BottomLeft) == Corners.BottomLeft)
{
LineTo(x, y + r);
CurveTo(x, y + r * b, x + r * b, y, x + r, y);
}else
LineTo(x, y);
}
Next step is to implment IPdfPCellEvent
interface:
public class myCellEvent : IPdfPCellEvent
{
#region members
PdfContentByte.Corners _roundedCorners;
float _roundness;
#endregion
#region ctor
public myCellEvent()
:this( PdfContentByte.Corners.All,2f)
{
}
public myCellEvent(PdfContentByte.Corners roundedCorners)
: this(roundedCorners, 2f)
{
}
public myCellEvent(PdfContentByte.Corners roundedCorners,float roundness)
{
_roundedCorners = roundedCorners;
_roundness = roundness;
}
#endregion
#region IPdfPCellEvent Members
public void CellLayout(PdfPCell cell, Rectangle position, PdfContentByte canvases)
{
PdfContentByte cb = canvases[PdfPTable.LINECANVAS];
cb.RoundRectangle(position.Left, position.Bottom, position.Right - position.Left, position.Top - position.Bottom, this._roundness, this._roundedCorners);
cb.SetColorStroke(new BaseColor(System.Drawing.Color.Black));
cb.SetColorFill(new BaseColor(System.Drawing.Color.Beige));
cb.FillStroke();
cb.Stroke();
}
#endregion
}
Then you round what ever corners you want to produce the rounded table of choice:
PdfPTable table = new PdfPTable(1);
PdfPCell cell = new PdfPCell(new Phrase(10, atext, f2));
cell.Border = 0;
cell.Padding = 5f;
cell.CellEvent = new myCellEvent(PdfContentByte.Corners.Top,2f);
table.AddCell(cell);
If you like the answer ... give it a vote :)
That is great for individual cells but what about the entire table? How could implement TableCell Events to accomplish this.
– Rick Make
Apr 29 '10 at 17:53
1
Adding another table with one cell that contains my target table works. Thanks again for your answer MK.
– Rick Make
Apr 29 '10 at 18:10
1
I'am glad that you have found the answer, another approach would be by rounding one corner of each table corner cell (TopLeft, TopRight, BottomLeft and BottomRight).
– MK.
May 2 '10 at 6:56
1
Nice answer MK! Helped me out a lot! :)
– Inigoesdr
Dec 9 '10 at 20:27
@Inigoesdr It's nice to READ that :)
– MK.
Dec 16 '10 at 15:42
add a comment |
If you have iTextSharp source code, add the following to PdfContentByte
class:
/// <summary>
/// Enumuration for defining corners you want rounded.
/// </summary>
[Flags()]
public enum Corners {None = 0,All=15,Top=3,Bottom=12, TopLeft = 1, TopRight=2, BottomLeft=4, BottomRight=8};
/// <summary>
/// Adds a round rectangle to the current path, with rounded conrners as specified by roundCorners.
/// </summary>
/// <param name="x"></param>
/// <param name="y"></param>
/// <param name="w"></param>
/// <param name="h"></param>
/// <param name="r"></param>
/// <param name="roundCorners"></param>
public void RoundRectangle(float x, float y, float w, float h, float r,Corners roundCorners)
{
if (w < 0)
{
x += w;
w = -w;
}
if (h < 0)
{
y += h;
h = -h;
}
if (r < 0)
r = -r;
float b = 0.4477f;
if((roundCorners & Corners.BottomLeft) == Corners.BottomLeft)
MoveTo(x + r, y);
else
MoveTo(x, y);
if ((roundCorners & Corners.BottomRight) == Corners.BottomRight)
{
LineTo(x + w - r, y);
CurveTo(x + w - r * b, y, x + w, y + r * b, x + w, y + r);
}
else
LineTo(x + w, y);
if ((roundCorners & Corners.TopRight ) == Corners.TopRight)
{
LineTo(x + w, y + h - r);
CurveTo(x + w, y + h - r * b, x + w - r * b, y + h, x + w - r, y + h);
}
else
LineTo(x + w, y + h);
if ((roundCorners & Corners.TopLeft) == Corners.TopLeft)
{
LineTo(x + r, y + h);
CurveTo(x + r * b, y + h, x, y + h - r * b, x, y + h - r);
}
else
LineTo(x , y + h);
if ((roundCorners & Corners.BottomLeft) == Corners.BottomLeft)
{
LineTo(x, y + r);
CurveTo(x, y + r * b, x + r * b, y, x + r, y);
}else
LineTo(x, y);
}
Next step is to implment IPdfPCellEvent
interface:
public class myCellEvent : IPdfPCellEvent
{
#region members
PdfContentByte.Corners _roundedCorners;
float _roundness;
#endregion
#region ctor
public myCellEvent()
:this( PdfContentByte.Corners.All,2f)
{
}
public myCellEvent(PdfContentByte.Corners roundedCorners)
: this(roundedCorners, 2f)
{
}
public myCellEvent(PdfContentByte.Corners roundedCorners,float roundness)
{
_roundedCorners = roundedCorners;
_roundness = roundness;
}
#endregion
#region IPdfPCellEvent Members
public void CellLayout(PdfPCell cell, Rectangle position, PdfContentByte canvases)
{
PdfContentByte cb = canvases[PdfPTable.LINECANVAS];
cb.RoundRectangle(position.Left, position.Bottom, position.Right - position.Left, position.Top - position.Bottom, this._roundness, this._roundedCorners);
cb.SetColorStroke(new BaseColor(System.Drawing.Color.Black));
cb.SetColorFill(new BaseColor(System.Drawing.Color.Beige));
cb.FillStroke();
cb.Stroke();
}
#endregion
}
Then you round what ever corners you want to produce the rounded table of choice:
PdfPTable table = new PdfPTable(1);
PdfPCell cell = new PdfPCell(new Phrase(10, atext, f2));
cell.Border = 0;
cell.Padding = 5f;
cell.CellEvent = new myCellEvent(PdfContentByte.Corners.Top,2f);
table.AddCell(cell);
If you like the answer ... give it a vote :)
If you have iTextSharp source code, add the following to PdfContentByte
class:
/// <summary>
/// Enumuration for defining corners you want rounded.
/// </summary>
[Flags()]
public enum Corners {None = 0,All=15,Top=3,Bottom=12, TopLeft = 1, TopRight=2, BottomLeft=4, BottomRight=8};
/// <summary>
/// Adds a round rectangle to the current path, with rounded conrners as specified by roundCorners.
/// </summary>
/// <param name="x"></param>
/// <param name="y"></param>
/// <param name="w"></param>
/// <param name="h"></param>
/// <param name="r"></param>
/// <param name="roundCorners"></param>
public void RoundRectangle(float x, float y, float w, float h, float r,Corners roundCorners)
{
if (w < 0)
{
x += w;
w = -w;
}
if (h < 0)
{
y += h;
h = -h;
}
if (r < 0)
r = -r;
float b = 0.4477f;
if((roundCorners & Corners.BottomLeft) == Corners.BottomLeft)
MoveTo(x + r, y);
else
MoveTo(x, y);
if ((roundCorners & Corners.BottomRight) == Corners.BottomRight)
{
LineTo(x + w - r, y);
CurveTo(x + w - r * b, y, x + w, y + r * b, x + w, y + r);
}
else
LineTo(x + w, y);
if ((roundCorners & Corners.TopRight ) == Corners.TopRight)
{
LineTo(x + w, y + h - r);
CurveTo(x + w, y + h - r * b, x + w - r * b, y + h, x + w - r, y + h);
}
else
LineTo(x + w, y + h);
if ((roundCorners & Corners.TopLeft) == Corners.TopLeft)
{
LineTo(x + r, y + h);
CurveTo(x + r * b, y + h, x, y + h - r * b, x, y + h - r);
}
else
LineTo(x , y + h);
if ((roundCorners & Corners.BottomLeft) == Corners.BottomLeft)
{
LineTo(x, y + r);
CurveTo(x, y + r * b, x + r * b, y, x + r, y);
}else
LineTo(x, y);
}
Next step is to implment IPdfPCellEvent
interface:
public class myCellEvent : IPdfPCellEvent
{
#region members
PdfContentByte.Corners _roundedCorners;
float _roundness;
#endregion
#region ctor
public myCellEvent()
:this( PdfContentByte.Corners.All,2f)
{
}
public myCellEvent(PdfContentByte.Corners roundedCorners)
: this(roundedCorners, 2f)
{
}
public myCellEvent(PdfContentByte.Corners roundedCorners,float roundness)
{
_roundedCorners = roundedCorners;
_roundness = roundness;
}
#endregion
#region IPdfPCellEvent Members
public void CellLayout(PdfPCell cell, Rectangle position, PdfContentByte canvases)
{
PdfContentByte cb = canvases[PdfPTable.LINECANVAS];
cb.RoundRectangle(position.Left, position.Bottom, position.Right - position.Left, position.Top - position.Bottom, this._roundness, this._roundedCorners);
cb.SetColorStroke(new BaseColor(System.Drawing.Color.Black));
cb.SetColorFill(new BaseColor(System.Drawing.Color.Beige));
cb.FillStroke();
cb.Stroke();
}
#endregion
}
Then you round what ever corners you want to produce the rounded table of choice:
PdfPTable table = new PdfPTable(1);
PdfPCell cell = new PdfPCell(new Phrase(10, atext, f2));
cell.Border = 0;
cell.Padding = 5f;
cell.CellEvent = new myCellEvent(PdfContentByte.Corners.Top,2f);
table.AddCell(cell);
If you like the answer ... give it a vote :)
answered Apr 28 '10 at 15:58
MK.
4,62911635
4,62911635
That is great for individual cells but what about the entire table? How could implement TableCell Events to accomplish this.
– Rick Make
Apr 29 '10 at 17:53
1
Adding another table with one cell that contains my target table works. Thanks again for your answer MK.
– Rick Make
Apr 29 '10 at 18:10
1
I'am glad that you have found the answer, another approach would be by rounding one corner of each table corner cell (TopLeft, TopRight, BottomLeft and BottomRight).
– MK.
May 2 '10 at 6:56
1
Nice answer MK! Helped me out a lot! :)
– Inigoesdr
Dec 9 '10 at 20:27
@Inigoesdr It's nice to READ that :)
– MK.
Dec 16 '10 at 15:42
add a comment |
That is great for individual cells but what about the entire table? How could implement TableCell Events to accomplish this.
– Rick Make
Apr 29 '10 at 17:53
1
Adding another table with one cell that contains my target table works. Thanks again for your answer MK.
– Rick Make
Apr 29 '10 at 18:10
1
I'am glad that you have found the answer, another approach would be by rounding one corner of each table corner cell (TopLeft, TopRight, BottomLeft and BottomRight).
– MK.
May 2 '10 at 6:56
1
Nice answer MK! Helped me out a lot! :)
– Inigoesdr
Dec 9 '10 at 20:27
@Inigoesdr It's nice to READ that :)
– MK.
Dec 16 '10 at 15:42
That is great for individual cells but what about the entire table? How could implement TableCell Events to accomplish this.
– Rick Make
Apr 29 '10 at 17:53
That is great for individual cells but what about the entire table? How could implement TableCell Events to accomplish this.
– Rick Make
Apr 29 '10 at 17:53
1
1
Adding another table with one cell that contains my target table works. Thanks again for your answer MK.
– Rick Make
Apr 29 '10 at 18:10
Adding another table with one cell that contains my target table works. Thanks again for your answer MK.
– Rick Make
Apr 29 '10 at 18:10
1
1
I'am glad that you have found the answer, another approach would be by rounding one corner of each table corner cell (TopLeft, TopRight, BottomLeft and BottomRight).
– MK.
May 2 '10 at 6:56
I'am glad that you have found the answer, another approach would be by rounding one corner of each table corner cell (TopLeft, TopRight, BottomLeft and BottomRight).
– MK.
May 2 '10 at 6:56
1
1
Nice answer MK! Helped me out a lot! :)
– Inigoesdr
Dec 9 '10 at 20:27
Nice answer MK! Helped me out a lot! :)
– Inigoesdr
Dec 9 '10 at 20:27
@Inigoesdr It's nice to READ that :)
– MK.
Dec 16 '10 at 15:42
@Inigoesdr It's nice to READ that :)
– MK.
Dec 16 '10 at 15:42
add a comment |
There's no way to create an iTextSharp PdfPTable
with rounded corners but what you could do is draw a table border with rounded corners using the PdfContentByte.RoundRectangle()
method.
Here's a snippet that demonstrates this:
Document doc = new Document();
using (FileStream fs = new FileStream("RoundRectangle Demo.pdf", FileMode.Create)) {
PdfWriter writer = PdfWriter.GetInstance(doc, fs);
doc.Open();
PdfContentByte cb = writer.DirectContent;
// Bottom left coordinates x & y, followed by width, height and radius of corners.
cb.RoundRectangle(100f, 500f, 200f, 200f, 2f);
cb.Stroke();
doc.Close();
}
This snippet is a condensed version of code I found in the article iTextSharp - Drawing shapes and Graphics on www.mikesdotnetting.com. You'll want to take a close look at his other articles if you haven't already.
1
That worked great. Do you know how I could find the positioning of the table so that I can accurately draw the rounded corners.
– Rick Make
Apr 3 '10 at 21:04
Simplest possible answer
– hdoghmen
Jun 17 '15 at 19:34
add a comment |
There's no way to create an iTextSharp PdfPTable
with rounded corners but what you could do is draw a table border with rounded corners using the PdfContentByte.RoundRectangle()
method.
Here's a snippet that demonstrates this:
Document doc = new Document();
using (FileStream fs = new FileStream("RoundRectangle Demo.pdf", FileMode.Create)) {
PdfWriter writer = PdfWriter.GetInstance(doc, fs);
doc.Open();
PdfContentByte cb = writer.DirectContent;
// Bottom left coordinates x & y, followed by width, height and radius of corners.
cb.RoundRectangle(100f, 500f, 200f, 200f, 2f);
cb.Stroke();
doc.Close();
}
This snippet is a condensed version of code I found in the article iTextSharp - Drawing shapes and Graphics on www.mikesdotnetting.com. You'll want to take a close look at his other articles if you haven't already.
1
That worked great. Do you know how I could find the positioning of the table so that I can accurately draw the rounded corners.
– Rick Make
Apr 3 '10 at 21:04
Simplest possible answer
– hdoghmen
Jun 17 '15 at 19:34
add a comment |
There's no way to create an iTextSharp PdfPTable
with rounded corners but what you could do is draw a table border with rounded corners using the PdfContentByte.RoundRectangle()
method.
Here's a snippet that demonstrates this:
Document doc = new Document();
using (FileStream fs = new FileStream("RoundRectangle Demo.pdf", FileMode.Create)) {
PdfWriter writer = PdfWriter.GetInstance(doc, fs);
doc.Open();
PdfContentByte cb = writer.DirectContent;
// Bottom left coordinates x & y, followed by width, height and radius of corners.
cb.RoundRectangle(100f, 500f, 200f, 200f, 2f);
cb.Stroke();
doc.Close();
}
This snippet is a condensed version of code I found in the article iTextSharp - Drawing shapes and Graphics on www.mikesdotnetting.com. You'll want to take a close look at his other articles if you haven't already.
There's no way to create an iTextSharp PdfPTable
with rounded corners but what you could do is draw a table border with rounded corners using the PdfContentByte.RoundRectangle()
method.
Here's a snippet that demonstrates this:
Document doc = new Document();
using (FileStream fs = new FileStream("RoundRectangle Demo.pdf", FileMode.Create)) {
PdfWriter writer = PdfWriter.GetInstance(doc, fs);
doc.Open();
PdfContentByte cb = writer.DirectContent;
// Bottom left coordinates x & y, followed by width, height and radius of corners.
cb.RoundRectangle(100f, 500f, 200f, 200f, 2f);
cb.Stroke();
doc.Close();
}
This snippet is a condensed version of code I found in the article iTextSharp - Drawing shapes and Graphics on www.mikesdotnetting.com. You'll want to take a close look at his other articles if you haven't already.
answered Apr 2 '10 at 5:53
Jay Riggs
46.5k8114133
46.5k8114133
1
That worked great. Do you know how I could find the positioning of the table so that I can accurately draw the rounded corners.
– Rick Make
Apr 3 '10 at 21:04
Simplest possible answer
– hdoghmen
Jun 17 '15 at 19:34
add a comment |
1
That worked great. Do you know how I could find the positioning of the table so that I can accurately draw the rounded corners.
– Rick Make
Apr 3 '10 at 21:04
Simplest possible answer
– hdoghmen
Jun 17 '15 at 19:34
1
1
That worked great. Do you know how I could find the positioning of the table so that I can accurately draw the rounded corners.
– Rick Make
Apr 3 '10 at 21:04
That worked great. Do you know how I could find the positioning of the table so that I can accurately draw the rounded corners.
– Rick Make
Apr 3 '10 at 21:04
Simplest possible answer
– hdoghmen
Jun 17 '15 at 19:34
Simplest possible answer
– hdoghmen
Jun 17 '15 at 19:34
add a comment |
I assume that if you are wanting a table with rounded corners, you're looking for some flashy (or at least pretty) formatting on your table. If that is the case, I would recommend turning off all the borders in your iTextSharp table, and using a graphic image as the background on your page. You can then make the graphic background as intricate and fancy as you want (shading, gradients, rounded corners, etc.) with very little trouble. Then, just line up your table with the background image.
If your data format will change often, you will not want to use this approach.
add a comment |
I assume that if you are wanting a table with rounded corners, you're looking for some flashy (or at least pretty) formatting on your table. If that is the case, I would recommend turning off all the borders in your iTextSharp table, and using a graphic image as the background on your page. You can then make the graphic background as intricate and fancy as you want (shading, gradients, rounded corners, etc.) with very little trouble. Then, just line up your table with the background image.
If your data format will change often, you will not want to use this approach.
add a comment |
I assume that if you are wanting a table with rounded corners, you're looking for some flashy (or at least pretty) formatting on your table. If that is the case, I would recommend turning off all the borders in your iTextSharp table, and using a graphic image as the background on your page. You can then make the graphic background as intricate and fancy as you want (shading, gradients, rounded corners, etc.) with very little trouble. Then, just line up your table with the background image.
If your data format will change often, you will not want to use this approach.
I assume that if you are wanting a table with rounded corners, you're looking for some flashy (or at least pretty) formatting on your table. If that is the case, I would recommend turning off all the borders in your iTextSharp table, and using a graphic image as the background on your page. You can then make the graphic background as intricate and fancy as you want (shading, gradients, rounded corners, etc.) with very little trouble. Then, just line up your table with the background image.
If your data format will change often, you will not want to use this approach.
answered Apr 1 '10 at 13:59


Stewbob
14.7k85193
14.7k85193
add a comment |
add a comment |
Private Function pdfDIF() As Byte()
Dim celevent As New PdfPCellEvent
Dim tableImportes As New PdfPTable(1)
tableImportes.SetTotalWidth({10 * dpixcm})
Dim cellImportes = New PdfPCell(New Phrase("IMPORTE", COURIER9Bold))
cellImportes.BorderWidth = 0
cellImportes.HorizontalAlignment = Element.ALIGN_CENTER
cellImportes.CellEvent = celevent
tableImportes.AddCell(cellImportes)
end function
Public Class PdfPCellEvent
Implements IPdfPCellEvent
Public Sub CellLayout(cell As iTextSharp.text.pdf.PdfPCell, position As iTextSharp.text.Rectangle, canvases() As iTextSharp.text.pdf.PdfContentByte) Implements iTextSharp.text.pdf.IPdfPCellEve`enter code here`nt.CellLayout
Dim cb As PdfContentByte = canvases(PdfPTable.LINECANVAS)
cb.RoundRectangle(position.Left,position.Bottom,position.Width,position.Height,10)
cb.SetLineWidth(1)
cb.SetCMYKColorStrokeF(0.0F, 0.0F, 0.0F, 1.0F)
cb.Stroke()
End Sub
End Class
add a comment |
Private Function pdfDIF() As Byte()
Dim celevent As New PdfPCellEvent
Dim tableImportes As New PdfPTable(1)
tableImportes.SetTotalWidth({10 * dpixcm})
Dim cellImportes = New PdfPCell(New Phrase("IMPORTE", COURIER9Bold))
cellImportes.BorderWidth = 0
cellImportes.HorizontalAlignment = Element.ALIGN_CENTER
cellImportes.CellEvent = celevent
tableImportes.AddCell(cellImportes)
end function
Public Class PdfPCellEvent
Implements IPdfPCellEvent
Public Sub CellLayout(cell As iTextSharp.text.pdf.PdfPCell, position As iTextSharp.text.Rectangle, canvases() As iTextSharp.text.pdf.PdfContentByte) Implements iTextSharp.text.pdf.IPdfPCellEve`enter code here`nt.CellLayout
Dim cb As PdfContentByte = canvases(PdfPTable.LINECANVAS)
cb.RoundRectangle(position.Left,position.Bottom,position.Width,position.Height,10)
cb.SetLineWidth(1)
cb.SetCMYKColorStrokeF(0.0F, 0.0F, 0.0F, 1.0F)
cb.Stroke()
End Sub
End Class
add a comment |
Private Function pdfDIF() As Byte()
Dim celevent As New PdfPCellEvent
Dim tableImportes As New PdfPTable(1)
tableImportes.SetTotalWidth({10 * dpixcm})
Dim cellImportes = New PdfPCell(New Phrase("IMPORTE", COURIER9Bold))
cellImportes.BorderWidth = 0
cellImportes.HorizontalAlignment = Element.ALIGN_CENTER
cellImportes.CellEvent = celevent
tableImportes.AddCell(cellImportes)
end function
Public Class PdfPCellEvent
Implements IPdfPCellEvent
Public Sub CellLayout(cell As iTextSharp.text.pdf.PdfPCell, position As iTextSharp.text.Rectangle, canvases() As iTextSharp.text.pdf.PdfContentByte) Implements iTextSharp.text.pdf.IPdfPCellEve`enter code here`nt.CellLayout
Dim cb As PdfContentByte = canvases(PdfPTable.LINECANVAS)
cb.RoundRectangle(position.Left,position.Bottom,position.Width,position.Height,10)
cb.SetLineWidth(1)
cb.SetCMYKColorStrokeF(0.0F, 0.0F, 0.0F, 1.0F)
cb.Stroke()
End Sub
End Class
Private Function pdfDIF() As Byte()
Dim celevent As New PdfPCellEvent
Dim tableImportes As New PdfPTable(1)
tableImportes.SetTotalWidth({10 * dpixcm})
Dim cellImportes = New PdfPCell(New Phrase("IMPORTE", COURIER9Bold))
cellImportes.BorderWidth = 0
cellImportes.HorizontalAlignment = Element.ALIGN_CENTER
cellImportes.CellEvent = celevent
tableImportes.AddCell(cellImportes)
end function
Public Class PdfPCellEvent
Implements IPdfPCellEvent
Public Sub CellLayout(cell As iTextSharp.text.pdf.PdfPCell, position As iTextSharp.text.Rectangle, canvases() As iTextSharp.text.pdf.PdfContentByte) Implements iTextSharp.text.pdf.IPdfPCellEve`enter code here`nt.CellLayout
Dim cb As PdfContentByte = canvases(PdfPTable.LINECANVAS)
cb.RoundRectangle(position.Left,position.Bottom,position.Width,position.Height,10)
cb.SetLineWidth(1)
cb.SetCMYKColorStrokeF(0.0F, 0.0F, 0.0F, 1.0F)
cb.Stroke()
End Sub
End Class
answered Nov 23 at 0:16
felu
1
1
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f2557217%2ftables-with-rounded-corners%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
JLt5BfYhOqMiqcmBx ZVgw8MYfP6Z3,P,DvJwy 1QYlY4E,vH8zvTYiGD2j35QK8aiPS8lmFnTpQW pkLGCpPkKGbx