Flow requires type annotation of props in HOC
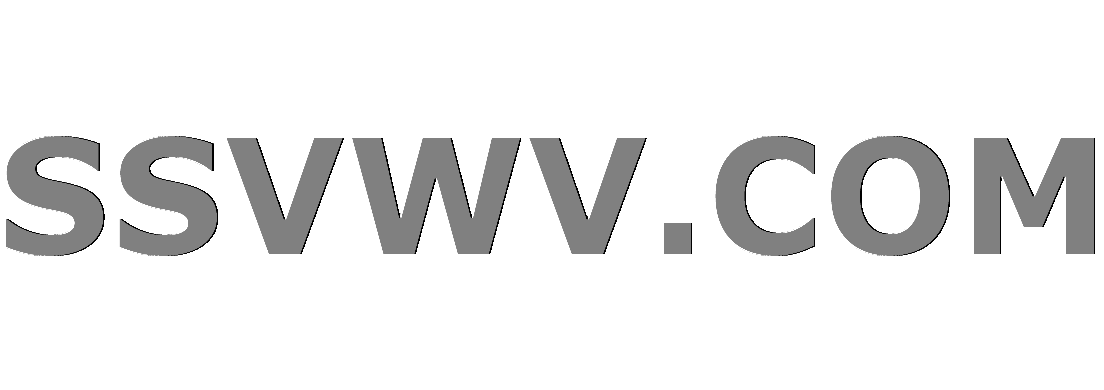
Multi tool use
up vote
2
down vote
favorite
I'm struggling with types of injected props by HOC.
It's just simple, in App Component, there are two props: title
and message
.
However title
is provided by HOC.
Here's the code for this:
/* @flow */
import React, { Component } from 'react';
import type { ComponentType } from 'react';
interface AppProps {
title: string;
message: string;
}
class App extends Component<AppProps, {}> {
static defaultProps = {
message: 'Helloworld'
}
render() {
return (
<div>
<div>Title: {this.props.title}</div>
<div>Message: {this.props.message}</div>
</div>
);
}
}
function injectProp<Props: {}>(
Component: ComponentType<{ title: string } & Props>
): ComponentType<Props> {
return function EnhancedComponent(props: Props) {
return <Component title="Hello" {...props} />;
}
};
export default injectProp(App);
It seems fine, however when I run the flow, it fails with
Missing type annotation for Props.
32| export default injectProp(App);
So I tried this, but no luck:
export default injectProp<AppProps>(App);
Now I got bunch of error messages.
> babel-react-webpack-flow-boilerplate@1.0.0 flow F:devwebproptype-test
> flow
Error ----------------------------------------------------------------------------------- src/js/components/App.js:32:16
Cannot compare boolean [1] to statics of `App` [2].
src/js/components/App.js:32:16
32| export default injectProp<AppProps>(App);
^^^^^^^^^^^^^^^^^^^ [1]
References:
src/js/components/App.js:10:7
10| class App extends Component<AppProps, {}> {
^^^ [2]
Error ----------------------------------------------------------------------------------- src/js/components/App.js:32:27
Cannot reference type `AppProps` [1] from a value position.
src/js/components/App.js:32:27
32| export default injectProp<AppProps>(App);
^^^^^^^^
References:
src/js/components/App.js:5:11
5| interface AppProps {
^^^^^^^^ [1]
Error --------------------------------------------------------------------------------------------- src/js/index.js:17:1
Cannot call `ReactDOM.render` because boolean [1] is not a React component.
src/js/index.js:17:1
17| ReactDOM.render(<App message="A" />, entryEl);
^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^
References:
src/js/components/App.js:32:16
32| export default injectProp<AppProps>(App);
^^^^^^^^^^^^^^^^^^^^^^^^^ [1]
Error -------------------------------------------------------------------------------------------- src/js/index.js:17:17
Cannot call `ReactDOM.render` with `<App />` bound to `element` because boolean [1] is incompatible with string [2] in
type argument `ElementType` [3].
src/js/index.js:17:17
17| ReactDOM.render(<App message="A" />, entryEl);
^^^^^^^^^^^^^^^^^^^
References:
src/js/components/App.js:32:16
32| export default injectProp<AppProps>(App);
^^^^^^^^^^^^^^^^^^^^^^^^^ [1]
C:UsersUserAppDataLocalTempflowflowlib_6db8195react.js:159:5
159| | string
^^^^^^ [2]
C:UsersUserAppDataLocalTempflowflowlib_6db8195react.js:167:29
167| declare type React$Element<+ElementType: React$ElementType> = {|
^^^^^^^^^^^ [3]
Error -------------------------------------------------------------------------------------------- src/js/index.js:17:17
Cannot create `App` element because boolean [1] is not a React component.
src/js/index.js:17:17
17| ReactDOM.render(<App message="A" />, entryEl);
^^^^^^^^^^^^^^^^^^^
References:
src/js/components/App.js:32:16
32| export default injectProp<AppProps>(App);
^^^^^^^^^^^^^^^^^^^^^^^^^ [1]
Found 5 errors
Only showing the most relevant union/intersection branches.
To see all branches, re-run Flow with --show-all-branches
Using flow-bin@0.69.0 and React@16.3.0. What am I missing? Why keep Flow complains about type annotation that doesn't needed?
reactjs flowtype high-order-component
add a comment |
up vote
2
down vote
favorite
I'm struggling with types of injected props by HOC.
It's just simple, in App Component, there are two props: title
and message
.
However title
is provided by HOC.
Here's the code for this:
/* @flow */
import React, { Component } from 'react';
import type { ComponentType } from 'react';
interface AppProps {
title: string;
message: string;
}
class App extends Component<AppProps, {}> {
static defaultProps = {
message: 'Helloworld'
}
render() {
return (
<div>
<div>Title: {this.props.title}</div>
<div>Message: {this.props.message}</div>
</div>
);
}
}
function injectProp<Props: {}>(
Component: ComponentType<{ title: string } & Props>
): ComponentType<Props> {
return function EnhancedComponent(props: Props) {
return <Component title="Hello" {...props} />;
}
};
export default injectProp(App);
It seems fine, however when I run the flow, it fails with
Missing type annotation for Props.
32| export default injectProp(App);
So I tried this, but no luck:
export default injectProp<AppProps>(App);
Now I got bunch of error messages.
> babel-react-webpack-flow-boilerplate@1.0.0 flow F:devwebproptype-test
> flow
Error ----------------------------------------------------------------------------------- src/js/components/App.js:32:16
Cannot compare boolean [1] to statics of `App` [2].
src/js/components/App.js:32:16
32| export default injectProp<AppProps>(App);
^^^^^^^^^^^^^^^^^^^ [1]
References:
src/js/components/App.js:10:7
10| class App extends Component<AppProps, {}> {
^^^ [2]
Error ----------------------------------------------------------------------------------- src/js/components/App.js:32:27
Cannot reference type `AppProps` [1] from a value position.
src/js/components/App.js:32:27
32| export default injectProp<AppProps>(App);
^^^^^^^^
References:
src/js/components/App.js:5:11
5| interface AppProps {
^^^^^^^^ [1]
Error --------------------------------------------------------------------------------------------- src/js/index.js:17:1
Cannot call `ReactDOM.render` because boolean [1] is not a React component.
src/js/index.js:17:1
17| ReactDOM.render(<App message="A" />, entryEl);
^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^
References:
src/js/components/App.js:32:16
32| export default injectProp<AppProps>(App);
^^^^^^^^^^^^^^^^^^^^^^^^^ [1]
Error -------------------------------------------------------------------------------------------- src/js/index.js:17:17
Cannot call `ReactDOM.render` with `<App />` bound to `element` because boolean [1] is incompatible with string [2] in
type argument `ElementType` [3].
src/js/index.js:17:17
17| ReactDOM.render(<App message="A" />, entryEl);
^^^^^^^^^^^^^^^^^^^
References:
src/js/components/App.js:32:16
32| export default injectProp<AppProps>(App);
^^^^^^^^^^^^^^^^^^^^^^^^^ [1]
C:UsersUserAppDataLocalTempflowflowlib_6db8195react.js:159:5
159| | string
^^^^^^ [2]
C:UsersUserAppDataLocalTempflowflowlib_6db8195react.js:167:29
167| declare type React$Element<+ElementType: React$ElementType> = {|
^^^^^^^^^^^ [3]
Error -------------------------------------------------------------------------------------------- src/js/index.js:17:17
Cannot create `App` element because boolean [1] is not a React component.
src/js/index.js:17:17
17| ReactDOM.render(<App message="A" />, entryEl);
^^^^^^^^^^^^^^^^^^^
References:
src/js/components/App.js:32:16
32| export default injectProp<AppProps>(App);
^^^^^^^^^^^^^^^^^^^^^^^^^ [1]
Found 5 errors
Only showing the most relevant union/intersection branches.
To see all branches, re-run Flow with --show-all-branches
Using flow-bin@0.69.0 and React@16.3.0. What am I missing? Why keep Flow complains about type annotation that doesn't needed?
reactjs flowtype high-order-component
how do you import App intoindex.js
?
– Alex
Nov 25 at 19:28
@Alex I just import App from 'App'; something like this.
– modernator
Nov 26 at 15:03
add a comment |
up vote
2
down vote
favorite
up vote
2
down vote
favorite
I'm struggling with types of injected props by HOC.
It's just simple, in App Component, there are two props: title
and message
.
However title
is provided by HOC.
Here's the code for this:
/* @flow */
import React, { Component } from 'react';
import type { ComponentType } from 'react';
interface AppProps {
title: string;
message: string;
}
class App extends Component<AppProps, {}> {
static defaultProps = {
message: 'Helloworld'
}
render() {
return (
<div>
<div>Title: {this.props.title}</div>
<div>Message: {this.props.message}</div>
</div>
);
}
}
function injectProp<Props: {}>(
Component: ComponentType<{ title: string } & Props>
): ComponentType<Props> {
return function EnhancedComponent(props: Props) {
return <Component title="Hello" {...props} />;
}
};
export default injectProp(App);
It seems fine, however when I run the flow, it fails with
Missing type annotation for Props.
32| export default injectProp(App);
So I tried this, but no luck:
export default injectProp<AppProps>(App);
Now I got bunch of error messages.
> babel-react-webpack-flow-boilerplate@1.0.0 flow F:devwebproptype-test
> flow
Error ----------------------------------------------------------------------------------- src/js/components/App.js:32:16
Cannot compare boolean [1] to statics of `App` [2].
src/js/components/App.js:32:16
32| export default injectProp<AppProps>(App);
^^^^^^^^^^^^^^^^^^^ [1]
References:
src/js/components/App.js:10:7
10| class App extends Component<AppProps, {}> {
^^^ [2]
Error ----------------------------------------------------------------------------------- src/js/components/App.js:32:27
Cannot reference type `AppProps` [1] from a value position.
src/js/components/App.js:32:27
32| export default injectProp<AppProps>(App);
^^^^^^^^
References:
src/js/components/App.js:5:11
5| interface AppProps {
^^^^^^^^ [1]
Error --------------------------------------------------------------------------------------------- src/js/index.js:17:1
Cannot call `ReactDOM.render` because boolean [1] is not a React component.
src/js/index.js:17:1
17| ReactDOM.render(<App message="A" />, entryEl);
^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^
References:
src/js/components/App.js:32:16
32| export default injectProp<AppProps>(App);
^^^^^^^^^^^^^^^^^^^^^^^^^ [1]
Error -------------------------------------------------------------------------------------------- src/js/index.js:17:17
Cannot call `ReactDOM.render` with `<App />` bound to `element` because boolean [1] is incompatible with string [2] in
type argument `ElementType` [3].
src/js/index.js:17:17
17| ReactDOM.render(<App message="A" />, entryEl);
^^^^^^^^^^^^^^^^^^^
References:
src/js/components/App.js:32:16
32| export default injectProp<AppProps>(App);
^^^^^^^^^^^^^^^^^^^^^^^^^ [1]
C:UsersUserAppDataLocalTempflowflowlib_6db8195react.js:159:5
159| | string
^^^^^^ [2]
C:UsersUserAppDataLocalTempflowflowlib_6db8195react.js:167:29
167| declare type React$Element<+ElementType: React$ElementType> = {|
^^^^^^^^^^^ [3]
Error -------------------------------------------------------------------------------------------- src/js/index.js:17:17
Cannot create `App` element because boolean [1] is not a React component.
src/js/index.js:17:17
17| ReactDOM.render(<App message="A" />, entryEl);
^^^^^^^^^^^^^^^^^^^
References:
src/js/components/App.js:32:16
32| export default injectProp<AppProps>(App);
^^^^^^^^^^^^^^^^^^^^^^^^^ [1]
Found 5 errors
Only showing the most relevant union/intersection branches.
To see all branches, re-run Flow with --show-all-branches
Using flow-bin@0.69.0 and React@16.3.0. What am I missing? Why keep Flow complains about type annotation that doesn't needed?
reactjs flowtype high-order-component
I'm struggling with types of injected props by HOC.
It's just simple, in App Component, there are two props: title
and message
.
However title
is provided by HOC.
Here's the code for this:
/* @flow */
import React, { Component } from 'react';
import type { ComponentType } from 'react';
interface AppProps {
title: string;
message: string;
}
class App extends Component<AppProps, {}> {
static defaultProps = {
message: 'Helloworld'
}
render() {
return (
<div>
<div>Title: {this.props.title}</div>
<div>Message: {this.props.message}</div>
</div>
);
}
}
function injectProp<Props: {}>(
Component: ComponentType<{ title: string } & Props>
): ComponentType<Props> {
return function EnhancedComponent(props: Props) {
return <Component title="Hello" {...props} />;
}
};
export default injectProp(App);
It seems fine, however when I run the flow, it fails with
Missing type annotation for Props.
32| export default injectProp(App);
So I tried this, but no luck:
export default injectProp<AppProps>(App);
Now I got bunch of error messages.
> babel-react-webpack-flow-boilerplate@1.0.0 flow F:devwebproptype-test
> flow
Error ----------------------------------------------------------------------------------- src/js/components/App.js:32:16
Cannot compare boolean [1] to statics of `App` [2].
src/js/components/App.js:32:16
32| export default injectProp<AppProps>(App);
^^^^^^^^^^^^^^^^^^^ [1]
References:
src/js/components/App.js:10:7
10| class App extends Component<AppProps, {}> {
^^^ [2]
Error ----------------------------------------------------------------------------------- src/js/components/App.js:32:27
Cannot reference type `AppProps` [1] from a value position.
src/js/components/App.js:32:27
32| export default injectProp<AppProps>(App);
^^^^^^^^
References:
src/js/components/App.js:5:11
5| interface AppProps {
^^^^^^^^ [1]
Error --------------------------------------------------------------------------------------------- src/js/index.js:17:1
Cannot call `ReactDOM.render` because boolean [1] is not a React component.
src/js/index.js:17:1
17| ReactDOM.render(<App message="A" />, entryEl);
^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^
References:
src/js/components/App.js:32:16
32| export default injectProp<AppProps>(App);
^^^^^^^^^^^^^^^^^^^^^^^^^ [1]
Error -------------------------------------------------------------------------------------------- src/js/index.js:17:17
Cannot call `ReactDOM.render` with `<App />` bound to `element` because boolean [1] is incompatible with string [2] in
type argument `ElementType` [3].
src/js/index.js:17:17
17| ReactDOM.render(<App message="A" />, entryEl);
^^^^^^^^^^^^^^^^^^^
References:
src/js/components/App.js:32:16
32| export default injectProp<AppProps>(App);
^^^^^^^^^^^^^^^^^^^^^^^^^ [1]
C:UsersUserAppDataLocalTempflowflowlib_6db8195react.js:159:5
159| | string
^^^^^^ [2]
C:UsersUserAppDataLocalTempflowflowlib_6db8195react.js:167:29
167| declare type React$Element<+ElementType: React$ElementType> = {|
^^^^^^^^^^^ [3]
Error -------------------------------------------------------------------------------------------- src/js/index.js:17:17
Cannot create `App` element because boolean [1] is not a React component.
src/js/index.js:17:17
17| ReactDOM.render(<App message="A" />, entryEl);
^^^^^^^^^^^^^^^^^^^
References:
src/js/components/App.js:32:16
32| export default injectProp<AppProps>(App);
^^^^^^^^^^^^^^^^^^^^^^^^^ [1]
Found 5 errors
Only showing the most relevant union/intersection branches.
To see all branches, re-run Flow with --show-all-branches
Using flow-bin@0.69.0 and React@16.3.0. What am I missing? Why keep Flow complains about type annotation that doesn't needed?
reactjs flowtype high-order-component
reactjs flowtype high-order-component
edited Nov 25 at 19:29


Alex
3,125621
3,125621
asked Nov 22 at 17:22
modernator
1,24032448
1,24032448
how do you import App intoindex.js
?
– Alex
Nov 25 at 19:28
@Alex I just import App from 'App'; something like this.
– modernator
Nov 26 at 15:03
add a comment |
how do you import App intoindex.js
?
– Alex
Nov 25 at 19:28
@Alex I just import App from 'App'; something like this.
– modernator
Nov 26 at 15:03
how do you import App into
index.js
?– Alex
Nov 25 at 19:28
how do you import App into
index.js
?– Alex
Nov 25 at 19:28
@Alex I just import App from 'App'; something like this.
– modernator
Nov 26 at 15:03
@Alex I just import App from 'App'; something like this.
– modernator
Nov 26 at 15:03
add a comment |
1 Answer
1
active
oldest
votes
up vote
0
down vote
accepted
This problem will resolve by export module like this:
export default injectProp<AppProps>(App);
And update to latest version(0.86.0) will works.
I made repo for this:
https://github.com/rico345100/hoc-prop-types-test
Might be helpful to people who has same issue like me.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53435785%2fflow-requires-type-annotation-of-props-in-hoc%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
accepted
This problem will resolve by export module like this:
export default injectProp<AppProps>(App);
And update to latest version(0.86.0) will works.
I made repo for this:
https://github.com/rico345100/hoc-prop-types-test
Might be helpful to people who has same issue like me.
add a comment |
up vote
0
down vote
accepted
This problem will resolve by export module like this:
export default injectProp<AppProps>(App);
And update to latest version(0.86.0) will works.
I made repo for this:
https://github.com/rico345100/hoc-prop-types-test
Might be helpful to people who has same issue like me.
add a comment |
up vote
0
down vote
accepted
up vote
0
down vote
accepted
This problem will resolve by export module like this:
export default injectProp<AppProps>(App);
And update to latest version(0.86.0) will works.
I made repo for this:
https://github.com/rico345100/hoc-prop-types-test
Might be helpful to people who has same issue like me.
This problem will resolve by export module like this:
export default injectProp<AppProps>(App);
And update to latest version(0.86.0) will works.
I made repo for this:
https://github.com/rico345100/hoc-prop-types-test
Might be helpful to people who has same issue like me.
answered Nov 26 at 15:04
modernator
1,24032448
1,24032448
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53435785%2fflow-requires-type-annotation-of-props-in-hoc%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
rugyyrq2oBEF,NZzs f U MJZiyfs6zCAR
how do you import App into
index.js
?– Alex
Nov 25 at 19:28
@Alex I just import App from 'App'; something like this.
– modernator
Nov 26 at 15:03