Angular multi search filtering
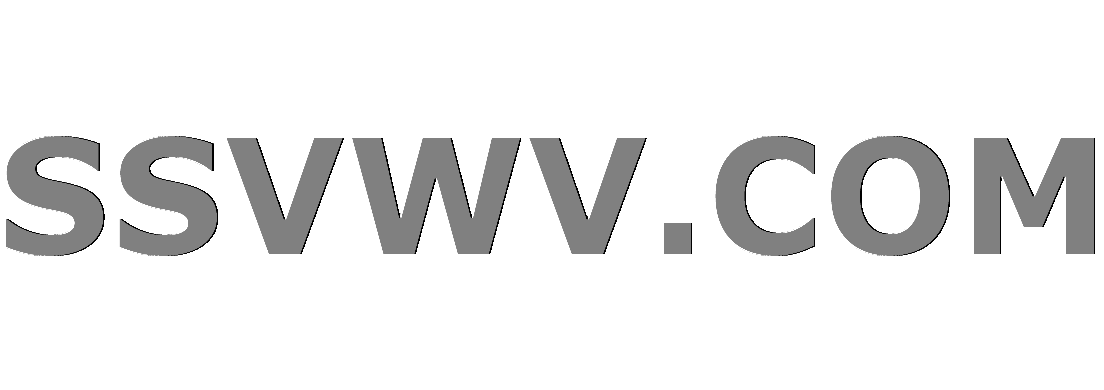
Multi tool use
up vote
0
down vote
favorite
I am building a multi search filter using angular 2. I can filter based on multiple filters using check boxes. The filters are applied perfectly based on what manufacturer, model, colour is selected.
I am looping through my array cars to draw my filters using *ngFor="let filters of filtersToDisplay" but i don't want to loop through the group of filters which are being selected, So i can apply more filters from the same group i.e manufacturer?
Here is my example: https://stackblitz.com/edit/angular-tenpga?file=src%2Fapp%2Fapp.component.ts
I use ng-for directive to build the filters see below:
<div style="padding:5px;" *ngFor="let filters of filtersToDisplay">
<input id="chkManufacturer" name="manufacturer" value="
{{filters.manufacturer}}" (change)="change($event)" type="checkbox" />
{{filters.manufacturer}}
</div>
<br />
<div style="padding:5px;" *ngFor="let filters of filtersToDisplay">
<input id="chkModel" name="model" (change)="change($event)" value="
{{filters.model}}" type="checkbox" />{{filters.model}}
</div>
<br />
<div style="padding:5px;" *ngFor="let filters of filtersToDisplay">
<input id="chkColour" name="colour" (change)="change($event)" value="
{{filters.colour}}" type="checkbox" />{{filters.colour}}
</div>
<table>
<thead>
<tr>
<th>Manufacturer</th>
<th>Model</th>
<th>Range</th>
<th>Price</th>
<th>Fuel</th>
<th>Year</th>
<th>Transmisson</th>
</tr>
</thead>
<tbody>
<ng-container *ngFor="let car of carsToDisplay">
<tr>
<td>{{car.manufacturer}}</td>
<td>{{car.model}}</td>
<td>{{car.range}}</td>
<td>{{car.price | currency:'GBP':true:'1.3-3'}}</td>
<td>{{car.fuel}}</td>
<td>{{car.year}}</td>
<td>{{car.transmisson}}</td>
</tr>
</ng-container>
<tr *ngIf="!cars || cars.length==0">
<td colspan="7">No cars to display</td>
</tr>
</tbody>
</table>
Here is my array of cars and change event when a check box is selected
Here is TS file:
selectedValue = ;
filterArr = ;
cars = [
{ code: 'car101', manufacturer: 'BMW', model: '3 Series', range: '320d M sport', price: 20000, fuel: 'Diesel', year: 2018, transmisson: 'Manual', colour: 'White'},
{ code: 'car102', manufacturer: 'Honda', model: 'Civic', range: 'Type R', price: 30000, fuel: 'Petrol', year: 2017, transmisson: 'Automatic', colour: 'Black'},
{ code: 'car103', manufacturer: 'Mercedes', model: 'A Class', range: 'AMG SPort', price: 50000, fuel: 'Petrol', year: 2018, transmisson: 'Automatic', colour: 'Red'},
{ code: 'car104', manufacturer: 'Audi', model: 'A3', range: 'TFSI Quattro S Line', price: 20000, fuel: 'Diesel', year: 2018, transmisson: 'Automatic', colour: 'Grey'},
{ code: 'car105', manufacturer: 'Ford', model: 'Focus', range: 'ST', price: 50000, fuel: 'Petrol', year: 2016, transmisson: 'Automatic', colour: 'Black'},
]
carsToDisplay = this.cars;
filtersToDisplay = this.cars;
change(e) {
if (e.target.checked) {
if (!this.filterArr.hasOwnProperty(e.srcElement.name))
this.filterArr[e.srcElement.name] = [e.target.value];
else
this.filterArr[e.srcElement.name].push(e.target.value);
this.carsToDisplay = multiFilter(this.cars, this.filterArr);
this.filtersToDisplay = Filter(this.cars, this.filterArr);
}
else
{
if (this.filterArr[e.srcElement.name]) {
let index =
this.filterArr[e.srcElement.name].indexOf(e.target.value)
this.filterArr[e.srcElement.name].splice(index, 1)
}
removeEmptyObjects(this.filterArr);
this.carsToDisplay = multiFilter(this.cars, this.filterArr);
this.filtersToDisplay = Filter(this.cars, this.filterArr);
}
function removeEmptyObjects(obj) {
for (var propName in obj) {
if (obj[propName] === null || obj[propName] === undefined ||
obj[propName].length == 0) {
delete obj[propName];
}
}
}
function multiFilter(array, filters) {
if (Object.keys(filters))
return array.filter(o =>
Object.keys(filters).every(k =>
.concat(filters[k]).some(v => o[k].includes(v))));
}
function Filter(array, filters) {
console.log(Object.keys(filters));
if (Object.keys(filters))
return array.filter(o =>
Object.keys(filters).every(k =>
.concat(filters[k]).some(v => o[k].includes(v))));
}
}
Hope this makes sense? I am very new to angular so would appreciate the best way to solve this.
javascript jquery angular typescript filtering
add a comment |
up vote
0
down vote
favorite
I am building a multi search filter using angular 2. I can filter based on multiple filters using check boxes. The filters are applied perfectly based on what manufacturer, model, colour is selected.
I am looping through my array cars to draw my filters using *ngFor="let filters of filtersToDisplay" but i don't want to loop through the group of filters which are being selected, So i can apply more filters from the same group i.e manufacturer?
Here is my example: https://stackblitz.com/edit/angular-tenpga?file=src%2Fapp%2Fapp.component.ts
I use ng-for directive to build the filters see below:
<div style="padding:5px;" *ngFor="let filters of filtersToDisplay">
<input id="chkManufacturer" name="manufacturer" value="
{{filters.manufacturer}}" (change)="change($event)" type="checkbox" />
{{filters.manufacturer}}
</div>
<br />
<div style="padding:5px;" *ngFor="let filters of filtersToDisplay">
<input id="chkModel" name="model" (change)="change($event)" value="
{{filters.model}}" type="checkbox" />{{filters.model}}
</div>
<br />
<div style="padding:5px;" *ngFor="let filters of filtersToDisplay">
<input id="chkColour" name="colour" (change)="change($event)" value="
{{filters.colour}}" type="checkbox" />{{filters.colour}}
</div>
<table>
<thead>
<tr>
<th>Manufacturer</th>
<th>Model</th>
<th>Range</th>
<th>Price</th>
<th>Fuel</th>
<th>Year</th>
<th>Transmisson</th>
</tr>
</thead>
<tbody>
<ng-container *ngFor="let car of carsToDisplay">
<tr>
<td>{{car.manufacturer}}</td>
<td>{{car.model}}</td>
<td>{{car.range}}</td>
<td>{{car.price | currency:'GBP':true:'1.3-3'}}</td>
<td>{{car.fuel}}</td>
<td>{{car.year}}</td>
<td>{{car.transmisson}}</td>
</tr>
</ng-container>
<tr *ngIf="!cars || cars.length==0">
<td colspan="7">No cars to display</td>
</tr>
</tbody>
</table>
Here is my array of cars and change event when a check box is selected
Here is TS file:
selectedValue = ;
filterArr = ;
cars = [
{ code: 'car101', manufacturer: 'BMW', model: '3 Series', range: '320d M sport', price: 20000, fuel: 'Diesel', year: 2018, transmisson: 'Manual', colour: 'White'},
{ code: 'car102', manufacturer: 'Honda', model: 'Civic', range: 'Type R', price: 30000, fuel: 'Petrol', year: 2017, transmisson: 'Automatic', colour: 'Black'},
{ code: 'car103', manufacturer: 'Mercedes', model: 'A Class', range: 'AMG SPort', price: 50000, fuel: 'Petrol', year: 2018, transmisson: 'Automatic', colour: 'Red'},
{ code: 'car104', manufacturer: 'Audi', model: 'A3', range: 'TFSI Quattro S Line', price: 20000, fuel: 'Diesel', year: 2018, transmisson: 'Automatic', colour: 'Grey'},
{ code: 'car105', manufacturer: 'Ford', model: 'Focus', range: 'ST', price: 50000, fuel: 'Petrol', year: 2016, transmisson: 'Automatic', colour: 'Black'},
]
carsToDisplay = this.cars;
filtersToDisplay = this.cars;
change(e) {
if (e.target.checked) {
if (!this.filterArr.hasOwnProperty(e.srcElement.name))
this.filterArr[e.srcElement.name] = [e.target.value];
else
this.filterArr[e.srcElement.name].push(e.target.value);
this.carsToDisplay = multiFilter(this.cars, this.filterArr);
this.filtersToDisplay = Filter(this.cars, this.filterArr);
}
else
{
if (this.filterArr[e.srcElement.name]) {
let index =
this.filterArr[e.srcElement.name].indexOf(e.target.value)
this.filterArr[e.srcElement.name].splice(index, 1)
}
removeEmptyObjects(this.filterArr);
this.carsToDisplay = multiFilter(this.cars, this.filterArr);
this.filtersToDisplay = Filter(this.cars, this.filterArr);
}
function removeEmptyObjects(obj) {
for (var propName in obj) {
if (obj[propName] === null || obj[propName] === undefined ||
obj[propName].length == 0) {
delete obj[propName];
}
}
}
function multiFilter(array, filters) {
if (Object.keys(filters))
return array.filter(o =>
Object.keys(filters).every(k =>
.concat(filters[k]).some(v => o[k].includes(v))));
}
function Filter(array, filters) {
console.log(Object.keys(filters));
if (Object.keys(filters))
return array.filter(o =>
Object.keys(filters).every(k =>
.concat(filters[k]).some(v => o[k].includes(v))));
}
}
Hope this makes sense? I am very new to angular so would appreciate the best way to solve this.
javascript jquery angular typescript filtering
Welcome to StackOverflow! Please provide a Minimal, Complete, and Verifiable example of what you've tried so far so we can reproduce your issue.
– Joseph Cho
Nov 22 at 17:52
1
I have edited my question hope it is more clear now. Thanks
– hamz123
Nov 23 at 14:41
Hey @hamz123 can you recreate this in stackblitz.io ? Use comments to explain the problem :-)
– Yoeri
Nov 23 at 14:46
Hey yes here is my example: stackblitz.com/edit/….
– hamz123
Nov 23 at 15:59
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I am building a multi search filter using angular 2. I can filter based on multiple filters using check boxes. The filters are applied perfectly based on what manufacturer, model, colour is selected.
I am looping through my array cars to draw my filters using *ngFor="let filters of filtersToDisplay" but i don't want to loop through the group of filters which are being selected, So i can apply more filters from the same group i.e manufacturer?
Here is my example: https://stackblitz.com/edit/angular-tenpga?file=src%2Fapp%2Fapp.component.ts
I use ng-for directive to build the filters see below:
<div style="padding:5px;" *ngFor="let filters of filtersToDisplay">
<input id="chkManufacturer" name="manufacturer" value="
{{filters.manufacturer}}" (change)="change($event)" type="checkbox" />
{{filters.manufacturer}}
</div>
<br />
<div style="padding:5px;" *ngFor="let filters of filtersToDisplay">
<input id="chkModel" name="model" (change)="change($event)" value="
{{filters.model}}" type="checkbox" />{{filters.model}}
</div>
<br />
<div style="padding:5px;" *ngFor="let filters of filtersToDisplay">
<input id="chkColour" name="colour" (change)="change($event)" value="
{{filters.colour}}" type="checkbox" />{{filters.colour}}
</div>
<table>
<thead>
<tr>
<th>Manufacturer</th>
<th>Model</th>
<th>Range</th>
<th>Price</th>
<th>Fuel</th>
<th>Year</th>
<th>Transmisson</th>
</tr>
</thead>
<tbody>
<ng-container *ngFor="let car of carsToDisplay">
<tr>
<td>{{car.manufacturer}}</td>
<td>{{car.model}}</td>
<td>{{car.range}}</td>
<td>{{car.price | currency:'GBP':true:'1.3-3'}}</td>
<td>{{car.fuel}}</td>
<td>{{car.year}}</td>
<td>{{car.transmisson}}</td>
</tr>
</ng-container>
<tr *ngIf="!cars || cars.length==0">
<td colspan="7">No cars to display</td>
</tr>
</tbody>
</table>
Here is my array of cars and change event when a check box is selected
Here is TS file:
selectedValue = ;
filterArr = ;
cars = [
{ code: 'car101', manufacturer: 'BMW', model: '3 Series', range: '320d M sport', price: 20000, fuel: 'Diesel', year: 2018, transmisson: 'Manual', colour: 'White'},
{ code: 'car102', manufacturer: 'Honda', model: 'Civic', range: 'Type R', price: 30000, fuel: 'Petrol', year: 2017, transmisson: 'Automatic', colour: 'Black'},
{ code: 'car103', manufacturer: 'Mercedes', model: 'A Class', range: 'AMG SPort', price: 50000, fuel: 'Petrol', year: 2018, transmisson: 'Automatic', colour: 'Red'},
{ code: 'car104', manufacturer: 'Audi', model: 'A3', range: 'TFSI Quattro S Line', price: 20000, fuel: 'Diesel', year: 2018, transmisson: 'Automatic', colour: 'Grey'},
{ code: 'car105', manufacturer: 'Ford', model: 'Focus', range: 'ST', price: 50000, fuel: 'Petrol', year: 2016, transmisson: 'Automatic', colour: 'Black'},
]
carsToDisplay = this.cars;
filtersToDisplay = this.cars;
change(e) {
if (e.target.checked) {
if (!this.filterArr.hasOwnProperty(e.srcElement.name))
this.filterArr[e.srcElement.name] = [e.target.value];
else
this.filterArr[e.srcElement.name].push(e.target.value);
this.carsToDisplay = multiFilter(this.cars, this.filterArr);
this.filtersToDisplay = Filter(this.cars, this.filterArr);
}
else
{
if (this.filterArr[e.srcElement.name]) {
let index =
this.filterArr[e.srcElement.name].indexOf(e.target.value)
this.filterArr[e.srcElement.name].splice(index, 1)
}
removeEmptyObjects(this.filterArr);
this.carsToDisplay = multiFilter(this.cars, this.filterArr);
this.filtersToDisplay = Filter(this.cars, this.filterArr);
}
function removeEmptyObjects(obj) {
for (var propName in obj) {
if (obj[propName] === null || obj[propName] === undefined ||
obj[propName].length == 0) {
delete obj[propName];
}
}
}
function multiFilter(array, filters) {
if (Object.keys(filters))
return array.filter(o =>
Object.keys(filters).every(k =>
.concat(filters[k]).some(v => o[k].includes(v))));
}
function Filter(array, filters) {
console.log(Object.keys(filters));
if (Object.keys(filters))
return array.filter(o =>
Object.keys(filters).every(k =>
.concat(filters[k]).some(v => o[k].includes(v))));
}
}
Hope this makes sense? I am very new to angular so would appreciate the best way to solve this.
javascript jquery angular typescript filtering
I am building a multi search filter using angular 2. I can filter based on multiple filters using check boxes. The filters are applied perfectly based on what manufacturer, model, colour is selected.
I am looping through my array cars to draw my filters using *ngFor="let filters of filtersToDisplay" but i don't want to loop through the group of filters which are being selected, So i can apply more filters from the same group i.e manufacturer?
Here is my example: https://stackblitz.com/edit/angular-tenpga?file=src%2Fapp%2Fapp.component.ts
I use ng-for directive to build the filters see below:
<div style="padding:5px;" *ngFor="let filters of filtersToDisplay">
<input id="chkManufacturer" name="manufacturer" value="
{{filters.manufacturer}}" (change)="change($event)" type="checkbox" />
{{filters.manufacturer}}
</div>
<br />
<div style="padding:5px;" *ngFor="let filters of filtersToDisplay">
<input id="chkModel" name="model" (change)="change($event)" value="
{{filters.model}}" type="checkbox" />{{filters.model}}
</div>
<br />
<div style="padding:5px;" *ngFor="let filters of filtersToDisplay">
<input id="chkColour" name="colour" (change)="change($event)" value="
{{filters.colour}}" type="checkbox" />{{filters.colour}}
</div>
<table>
<thead>
<tr>
<th>Manufacturer</th>
<th>Model</th>
<th>Range</th>
<th>Price</th>
<th>Fuel</th>
<th>Year</th>
<th>Transmisson</th>
</tr>
</thead>
<tbody>
<ng-container *ngFor="let car of carsToDisplay">
<tr>
<td>{{car.manufacturer}}</td>
<td>{{car.model}}</td>
<td>{{car.range}}</td>
<td>{{car.price | currency:'GBP':true:'1.3-3'}}</td>
<td>{{car.fuel}}</td>
<td>{{car.year}}</td>
<td>{{car.transmisson}}</td>
</tr>
</ng-container>
<tr *ngIf="!cars || cars.length==0">
<td colspan="7">No cars to display</td>
</tr>
</tbody>
</table>
Here is my array of cars and change event when a check box is selected
Here is TS file:
selectedValue = ;
filterArr = ;
cars = [
{ code: 'car101', manufacturer: 'BMW', model: '3 Series', range: '320d M sport', price: 20000, fuel: 'Diesel', year: 2018, transmisson: 'Manual', colour: 'White'},
{ code: 'car102', manufacturer: 'Honda', model: 'Civic', range: 'Type R', price: 30000, fuel: 'Petrol', year: 2017, transmisson: 'Automatic', colour: 'Black'},
{ code: 'car103', manufacturer: 'Mercedes', model: 'A Class', range: 'AMG SPort', price: 50000, fuel: 'Petrol', year: 2018, transmisson: 'Automatic', colour: 'Red'},
{ code: 'car104', manufacturer: 'Audi', model: 'A3', range: 'TFSI Quattro S Line', price: 20000, fuel: 'Diesel', year: 2018, transmisson: 'Automatic', colour: 'Grey'},
{ code: 'car105', manufacturer: 'Ford', model: 'Focus', range: 'ST', price: 50000, fuel: 'Petrol', year: 2016, transmisson: 'Automatic', colour: 'Black'},
]
carsToDisplay = this.cars;
filtersToDisplay = this.cars;
change(e) {
if (e.target.checked) {
if (!this.filterArr.hasOwnProperty(e.srcElement.name))
this.filterArr[e.srcElement.name] = [e.target.value];
else
this.filterArr[e.srcElement.name].push(e.target.value);
this.carsToDisplay = multiFilter(this.cars, this.filterArr);
this.filtersToDisplay = Filter(this.cars, this.filterArr);
}
else
{
if (this.filterArr[e.srcElement.name]) {
let index =
this.filterArr[e.srcElement.name].indexOf(e.target.value)
this.filterArr[e.srcElement.name].splice(index, 1)
}
removeEmptyObjects(this.filterArr);
this.carsToDisplay = multiFilter(this.cars, this.filterArr);
this.filtersToDisplay = Filter(this.cars, this.filterArr);
}
function removeEmptyObjects(obj) {
for (var propName in obj) {
if (obj[propName] === null || obj[propName] === undefined ||
obj[propName].length == 0) {
delete obj[propName];
}
}
}
function multiFilter(array, filters) {
if (Object.keys(filters))
return array.filter(o =>
Object.keys(filters).every(k =>
.concat(filters[k]).some(v => o[k].includes(v))));
}
function Filter(array, filters) {
console.log(Object.keys(filters));
if (Object.keys(filters))
return array.filter(o =>
Object.keys(filters).every(k =>
.concat(filters[k]).some(v => o[k].includes(v))));
}
}
Hope this makes sense? I am very new to angular so would appreciate the best way to solve this.
javascript jquery angular typescript filtering
javascript jquery angular typescript filtering
edited Nov 27 at 11:54
asked Nov 22 at 17:18
hamz123
61
61
Welcome to StackOverflow! Please provide a Minimal, Complete, and Verifiable example of what you've tried so far so we can reproduce your issue.
– Joseph Cho
Nov 22 at 17:52
1
I have edited my question hope it is more clear now. Thanks
– hamz123
Nov 23 at 14:41
Hey @hamz123 can you recreate this in stackblitz.io ? Use comments to explain the problem :-)
– Yoeri
Nov 23 at 14:46
Hey yes here is my example: stackblitz.com/edit/….
– hamz123
Nov 23 at 15:59
add a comment |
Welcome to StackOverflow! Please provide a Minimal, Complete, and Verifiable example of what you've tried so far so we can reproduce your issue.
– Joseph Cho
Nov 22 at 17:52
1
I have edited my question hope it is more clear now. Thanks
– hamz123
Nov 23 at 14:41
Hey @hamz123 can you recreate this in stackblitz.io ? Use comments to explain the problem :-)
– Yoeri
Nov 23 at 14:46
Hey yes here is my example: stackblitz.com/edit/….
– hamz123
Nov 23 at 15:59
Welcome to StackOverflow! Please provide a Minimal, Complete, and Verifiable example of what you've tried so far so we can reproduce your issue.
– Joseph Cho
Nov 22 at 17:52
Welcome to StackOverflow! Please provide a Minimal, Complete, and Verifiable example of what you've tried so far so we can reproduce your issue.
– Joseph Cho
Nov 22 at 17:52
1
1
I have edited my question hope it is more clear now. Thanks
– hamz123
Nov 23 at 14:41
I have edited my question hope it is more clear now. Thanks
– hamz123
Nov 23 at 14:41
Hey @hamz123 can you recreate this in stackblitz.io ? Use comments to explain the problem :-)
– Yoeri
Nov 23 at 14:46
Hey @hamz123 can you recreate this in stackblitz.io ? Use comments to explain the problem :-)
– Yoeri
Nov 23 at 14:46
Hey yes here is my example: stackblitz.com/edit/….
– hamz123
Nov 23 at 15:59
Hey yes here is my example: stackblitz.com/edit/….
– hamz123
Nov 23 at 15:59
add a comment |
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53435726%2fangular-multi-search-filtering%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53435726%2fangular-multi-search-filtering%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
S 1YPwfw3yORPiJ6,nmZRmNPTiiJ 5d99z3 XjE0,W3FNA,2nadUVNOuvE,iu0g9HDaO2kFvSb1FOD i6R0IZNuG
Welcome to StackOverflow! Please provide a Minimal, Complete, and Verifiable example of what you've tried so far so we can reproduce your issue.
– Joseph Cho
Nov 22 at 17:52
1
I have edited my question hope it is more clear now. Thanks
– hamz123
Nov 23 at 14:41
Hey @hamz123 can you recreate this in stackblitz.io ? Use comments to explain the problem :-)
– Yoeri
Nov 23 at 14:46
Hey yes here is my example: stackblitz.com/edit/….
– hamz123
Nov 23 at 15:59