Numpy question regarding accessing elements
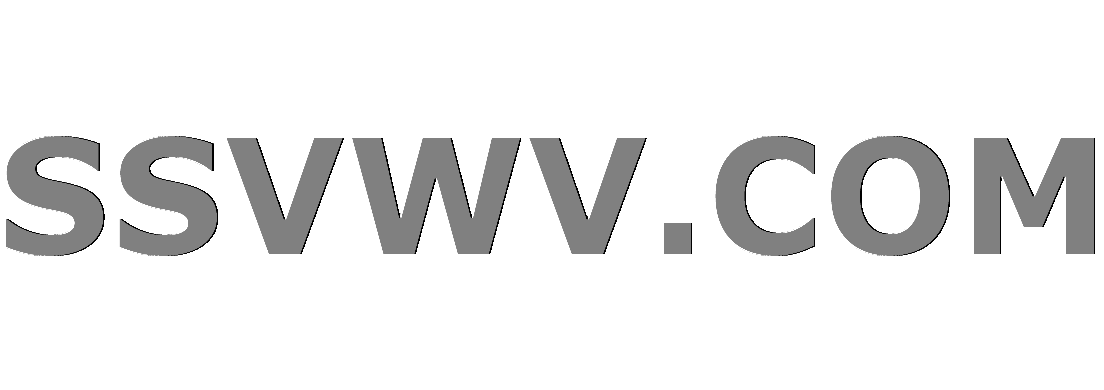
Multi tool use
up vote
0
down vote
favorite
So I have created an array from 3 nested lists (atleast I think it is an array from 3 lists), and I want to access the three diagonal elements in it. I have the array created, but how do I access the three diagonal elements in it?
from numpy import *
test1 = arange(27).reshape(3,3,3)
test1
Result:
array([[[ 0, 1, 2],
[ 3, 4, 5],
[ 6, 7, 8]],
[[ 9, 10, 11],
[12, 13, 14],
[15, 16, 17]],
[[18, 19, 20],
[21, 22, 23],
[24, 25, 26]]])
python numpy
add a comment |
up vote
0
down vote
favorite
So I have created an array from 3 nested lists (atleast I think it is an array from 3 lists), and I want to access the three diagonal elements in it. I have the array created, but how do I access the three diagonal elements in it?
from numpy import *
test1 = arange(27).reshape(3,3,3)
test1
Result:
array([[[ 0, 1, 2],
[ 3, 4, 5],
[ 6, 7, 8]],
[[ 9, 10, 11],
[12, 13, 14],
[15, 16, 17]],
[[18, 19, 20],
[21, 22, 23],
[24, 25, 26]]])
python numpy
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
So I have created an array from 3 nested lists (atleast I think it is an array from 3 lists), and I want to access the three diagonal elements in it. I have the array created, but how do I access the three diagonal elements in it?
from numpy import *
test1 = arange(27).reshape(3,3,3)
test1
Result:
array([[[ 0, 1, 2],
[ 3, 4, 5],
[ 6, 7, 8]],
[[ 9, 10, 11],
[12, 13, 14],
[15, 16, 17]],
[[18, 19, 20],
[21, 22, 23],
[24, 25, 26]]])
python numpy
So I have created an array from 3 nested lists (atleast I think it is an array from 3 lists), and I want to access the three diagonal elements in it. I have the array created, but how do I access the three diagonal elements in it?
from numpy import *
test1 = arange(27).reshape(3,3,3)
test1
Result:
array([[[ 0, 1, 2],
[ 3, 4, 5],
[ 6, 7, 8]],
[[ 9, 10, 11],
[12, 13, 14],
[15, 16, 17]],
[[18, 19, 20],
[21, 22, 23],
[24, 25, 26]]])
python numpy
python numpy
edited Nov 22 at 2:24
asked Nov 22 at 1:58
blargh
12
12
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
up vote
0
down vote
Here is a list comprehension approach:
>>> [np.diagonal(i) for i in test1]
[array([0, 4, 8]), array([ 9, 13, 17]), array([18, 22, 26])]
Sorry I had to re edit my code, and the np.diag(test1) does not work now : (
– blargh
Nov 22 at 2:24
add a comment |
up vote
0
down vote
There are several ways to achieve your goal. Here, I will highlight the use of a boolean mask.
First create the boolean 3x3 identity matrix : i.e. the diagonal is True whilst 2. every off diagonal entry is False.
Then overlay the boolean mask over your original ndarray to get the diagonals.
import numpy as np
test1 = np.arange(27).reshape(3,3,3)
>>> diag = np.eye(3, dtype=bool)
>>> test1[:, diag]
array([[ 0, 4, 8],
[ 9, 13, 17],
[18, 22, 26]])
As you can see, this gives a 2d array where each row is the corresponding diagonal of the zeroth, first and second
2d array in your 3d array.
As an aside, avoid import *
, it is the cause of many a headache because if destroys the namespace abstraction you
have. In the above example, what if numpy had a diag
function or variable defined? same if you import another package after numpy and it happens to have it's own arange
function, you will looes numpy's arange function.
Prefer explicit imports to star imports.
I see! Thank you and I will def keep the asterisk in mind. I have a follow up question, what is a constant array?
– blargh
Nov 22 at 3:39
Please accept the solution if it answers your question. A constant array is an array that is constant i.e. in a mathematical context it can be an array of coefficients where the coefficients are constant.
– Xero Smith
Nov 22 at 3:46
add a comment |
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
Here is a list comprehension approach:
>>> [np.diagonal(i) for i in test1]
[array([0, 4, 8]), array([ 9, 13, 17]), array([18, 22, 26])]
Sorry I had to re edit my code, and the np.diag(test1) does not work now : (
– blargh
Nov 22 at 2:24
add a comment |
up vote
0
down vote
Here is a list comprehension approach:
>>> [np.diagonal(i) for i in test1]
[array([0, 4, 8]), array([ 9, 13, 17]), array([18, 22, 26])]
Sorry I had to re edit my code, and the np.diag(test1) does not work now : (
– blargh
Nov 22 at 2:24
add a comment |
up vote
0
down vote
up vote
0
down vote
Here is a list comprehension approach:
>>> [np.diagonal(i) for i in test1]
[array([0, 4, 8]), array([ 9, 13, 17]), array([18, 22, 26])]
Here is a list comprehension approach:
>>> [np.diagonal(i) for i in test1]
[array([0, 4, 8]), array([ 9, 13, 17]), array([18, 22, 26])]
edited Nov 22 at 2:43
answered Nov 22 at 2:10


Siong Thye Goh
859312
859312
Sorry I had to re edit my code, and the np.diag(test1) does not work now : (
– blargh
Nov 22 at 2:24
add a comment |
Sorry I had to re edit my code, and the np.diag(test1) does not work now : (
– blargh
Nov 22 at 2:24
Sorry I had to re edit my code, and the np.diag(test1) does not work now : (
– blargh
Nov 22 at 2:24
Sorry I had to re edit my code, and the np.diag(test1) does not work now : (
– blargh
Nov 22 at 2:24
add a comment |
up vote
0
down vote
There are several ways to achieve your goal. Here, I will highlight the use of a boolean mask.
First create the boolean 3x3 identity matrix : i.e. the diagonal is True whilst 2. every off diagonal entry is False.
Then overlay the boolean mask over your original ndarray to get the diagonals.
import numpy as np
test1 = np.arange(27).reshape(3,3,3)
>>> diag = np.eye(3, dtype=bool)
>>> test1[:, diag]
array([[ 0, 4, 8],
[ 9, 13, 17],
[18, 22, 26]])
As you can see, this gives a 2d array where each row is the corresponding diagonal of the zeroth, first and second
2d array in your 3d array.
As an aside, avoid import *
, it is the cause of many a headache because if destroys the namespace abstraction you
have. In the above example, what if numpy had a diag
function or variable defined? same if you import another package after numpy and it happens to have it's own arange
function, you will looes numpy's arange function.
Prefer explicit imports to star imports.
I see! Thank you and I will def keep the asterisk in mind. I have a follow up question, what is a constant array?
– blargh
Nov 22 at 3:39
Please accept the solution if it answers your question. A constant array is an array that is constant i.e. in a mathematical context it can be an array of coefficients where the coefficients are constant.
– Xero Smith
Nov 22 at 3:46
add a comment |
up vote
0
down vote
There are several ways to achieve your goal. Here, I will highlight the use of a boolean mask.
First create the boolean 3x3 identity matrix : i.e. the diagonal is True whilst 2. every off diagonal entry is False.
Then overlay the boolean mask over your original ndarray to get the diagonals.
import numpy as np
test1 = np.arange(27).reshape(3,3,3)
>>> diag = np.eye(3, dtype=bool)
>>> test1[:, diag]
array([[ 0, 4, 8],
[ 9, 13, 17],
[18, 22, 26]])
As you can see, this gives a 2d array where each row is the corresponding diagonal of the zeroth, first and second
2d array in your 3d array.
As an aside, avoid import *
, it is the cause of many a headache because if destroys the namespace abstraction you
have. In the above example, what if numpy had a diag
function or variable defined? same if you import another package after numpy and it happens to have it's own arange
function, you will looes numpy's arange function.
Prefer explicit imports to star imports.
I see! Thank you and I will def keep the asterisk in mind. I have a follow up question, what is a constant array?
– blargh
Nov 22 at 3:39
Please accept the solution if it answers your question. A constant array is an array that is constant i.e. in a mathematical context it can be an array of coefficients where the coefficients are constant.
– Xero Smith
Nov 22 at 3:46
add a comment |
up vote
0
down vote
up vote
0
down vote
There are several ways to achieve your goal. Here, I will highlight the use of a boolean mask.
First create the boolean 3x3 identity matrix : i.e. the diagonal is True whilst 2. every off diagonal entry is False.
Then overlay the boolean mask over your original ndarray to get the diagonals.
import numpy as np
test1 = np.arange(27).reshape(3,3,3)
>>> diag = np.eye(3, dtype=bool)
>>> test1[:, diag]
array([[ 0, 4, 8],
[ 9, 13, 17],
[18, 22, 26]])
As you can see, this gives a 2d array where each row is the corresponding diagonal of the zeroth, first and second
2d array in your 3d array.
As an aside, avoid import *
, it is the cause of many a headache because if destroys the namespace abstraction you
have. In the above example, what if numpy had a diag
function or variable defined? same if you import another package after numpy and it happens to have it's own arange
function, you will looes numpy's arange function.
Prefer explicit imports to star imports.
There are several ways to achieve your goal. Here, I will highlight the use of a boolean mask.
First create the boolean 3x3 identity matrix : i.e. the diagonal is True whilst 2. every off diagonal entry is False.
Then overlay the boolean mask over your original ndarray to get the diagonals.
import numpy as np
test1 = np.arange(27).reshape(3,3,3)
>>> diag = np.eye(3, dtype=bool)
>>> test1[:, diag]
array([[ 0, 4, 8],
[ 9, 13, 17],
[18, 22, 26]])
As you can see, this gives a 2d array where each row is the corresponding diagonal of the zeroth, first and second
2d array in your 3d array.
As an aside, avoid import *
, it is the cause of many a headache because if destroys the namespace abstraction you
have. In the above example, what if numpy had a diag
function or variable defined? same if you import another package after numpy and it happens to have it's own arange
function, you will looes numpy's arange function.
Prefer explicit imports to star imports.
answered Nov 22 at 3:06


Xero Smith
8061513
8061513
I see! Thank you and I will def keep the asterisk in mind. I have a follow up question, what is a constant array?
– blargh
Nov 22 at 3:39
Please accept the solution if it answers your question. A constant array is an array that is constant i.e. in a mathematical context it can be an array of coefficients where the coefficients are constant.
– Xero Smith
Nov 22 at 3:46
add a comment |
I see! Thank you and I will def keep the asterisk in mind. I have a follow up question, what is a constant array?
– blargh
Nov 22 at 3:39
Please accept the solution if it answers your question. A constant array is an array that is constant i.e. in a mathematical context it can be an array of coefficients where the coefficients are constant.
– Xero Smith
Nov 22 at 3:46
I see! Thank you and I will def keep the asterisk in mind. I have a follow up question, what is a constant array?
– blargh
Nov 22 at 3:39
I see! Thank you and I will def keep the asterisk in mind. I have a follow up question, what is a constant array?
– blargh
Nov 22 at 3:39
Please accept the solution if it answers your question. A constant array is an array that is constant i.e. in a mathematical context it can be an array of coefficients where the coefficients are constant.
– Xero Smith
Nov 22 at 3:46
Please accept the solution if it answers your question. A constant array is an array that is constant i.e. in a mathematical context it can be an array of coefficients where the coefficients are constant.
– Xero Smith
Nov 22 at 3:46
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53422875%2fnumpy-question-regarding-accessing-elements%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
V2kcTDp4OTAKAP uObTK,DZUUCViXGGT6z