Access to encapsulated anonymous function
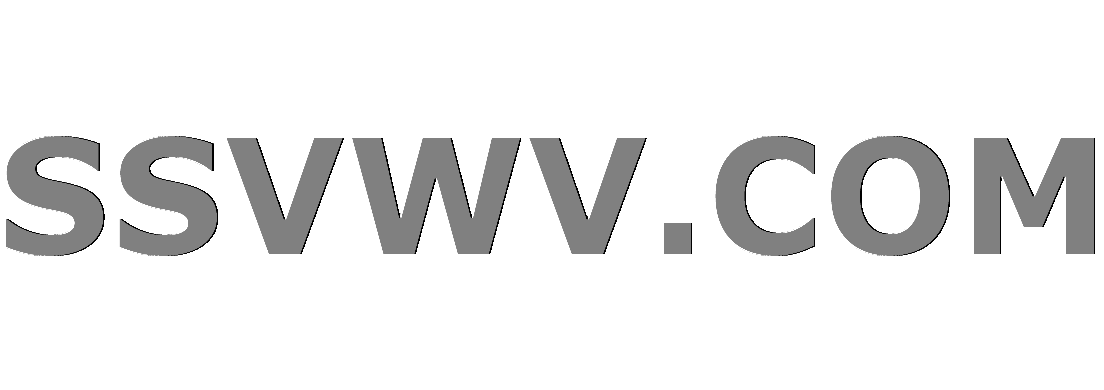
Multi tool use
up vote
0
down vote
favorite
I have this code
(function(data){
this.init=function(){
console.log("Hello world");
};
this.init();
});
I wan to call the anonymous function from external code, without self execution. Can anyone help whit this?
javascript
|
show 3 more comments
up vote
0
down vote
favorite
I have this code
(function(data){
this.init=function(){
console.log("Hello world");
};
this.init();
});
I wan to call the anonymous function from external code, without self execution. Can anyone help whit this?
javascript
1
You can't do that as that is the point of an anonymous function.
– Scott Marcus
Nov 22 at 15:38
You have to assign it to a variable for example,var myFunc = (function(data){...})
– lenilsondc
Nov 22 at 15:39
You want to call self executing function without self executing? Maybe use named function instead and when need auto execution simply call it?
– Justinas
Nov 22 at 15:39
Maybe you should assing the function to a globally accessible object (like window or document) and then you could access it from there?
– Marcelo Myara
Nov 22 at 15:40
@lenilsondc That wouldn't work. You'd need to remove the outer parenthesis, because with them the expression returns nothing.
– Scott Marcus
Nov 22 at 15:43
|
show 3 more comments
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I have this code
(function(data){
this.init=function(){
console.log("Hello world");
};
this.init();
});
I wan to call the anonymous function from external code, without self execution. Can anyone help whit this?
javascript
I have this code
(function(data){
this.init=function(){
console.log("Hello world");
};
this.init();
});
I wan to call the anonymous function from external code, without self execution. Can anyone help whit this?
javascript
javascript
edited Nov 22 at 15:37


Scott Marcus
38.3k51936
38.3k51936
asked Nov 22 at 15:36
Mcruz
1
1
1
You can't do that as that is the point of an anonymous function.
– Scott Marcus
Nov 22 at 15:38
You have to assign it to a variable for example,var myFunc = (function(data){...})
– lenilsondc
Nov 22 at 15:39
You want to call self executing function without self executing? Maybe use named function instead and when need auto execution simply call it?
– Justinas
Nov 22 at 15:39
Maybe you should assing the function to a globally accessible object (like window or document) and then you could access it from there?
– Marcelo Myara
Nov 22 at 15:40
@lenilsondc That wouldn't work. You'd need to remove the outer parenthesis, because with them the expression returns nothing.
– Scott Marcus
Nov 22 at 15:43
|
show 3 more comments
1
You can't do that as that is the point of an anonymous function.
– Scott Marcus
Nov 22 at 15:38
You have to assign it to a variable for example,var myFunc = (function(data){...})
– lenilsondc
Nov 22 at 15:39
You want to call self executing function without self executing? Maybe use named function instead and when need auto execution simply call it?
– Justinas
Nov 22 at 15:39
Maybe you should assing the function to a globally accessible object (like window or document) and then you could access it from there?
– Marcelo Myara
Nov 22 at 15:40
@lenilsondc That wouldn't work. You'd need to remove the outer parenthesis, because with them the expression returns nothing.
– Scott Marcus
Nov 22 at 15:43
1
1
You can't do that as that is the point of an anonymous function.
– Scott Marcus
Nov 22 at 15:38
You can't do that as that is the point of an anonymous function.
– Scott Marcus
Nov 22 at 15:38
You have to assign it to a variable for example,
var myFunc = (function(data){...})
– lenilsondc
Nov 22 at 15:39
You have to assign it to a variable for example,
var myFunc = (function(data){...})
– lenilsondc
Nov 22 at 15:39
You want to call self executing function without self executing? Maybe use named function instead and when need auto execution simply call it?
– Justinas
Nov 22 at 15:39
You want to call self executing function without self executing? Maybe use named function instead and when need auto execution simply call it?
– Justinas
Nov 22 at 15:39
Maybe you should assing the function to a globally accessible object (like window or document) and then you could access it from there?
– Marcelo Myara
Nov 22 at 15:40
Maybe you should assing the function to a globally accessible object (like window or document) and then you could access it from there?
– Marcelo Myara
Nov 22 at 15:40
@lenilsondc That wouldn't work. You'd need to remove the outer parenthesis, because with them the expression returns nothing.
– Scott Marcus
Nov 22 at 15:43
@lenilsondc That wouldn't work. You'd need to remove the outer parenthesis, because with them the expression returns nothing.
– Scott Marcus
Nov 22 at 15:43
|
show 3 more comments
1 Answer
1
active
oldest
votes
up vote
0
down vote
You would have to attribute the anonymous function to a variable in order to get a reference to it and then be able to call it like the code bellow:
var myFunc = (function(data){...})
However, assuming you don't have control over the javascript to which you are trying to do it, you would have to evaluate the code as described by MDN in this chapter and build the previous expression.
UGLY CODING PRACTICES ALERT: this practice I'm about to propose is not at all a good practice, evaluating javascript code at runtime is not good and should be avoided unless it is extremely necessary. Having said that, and you still think you need to do this, follow the instructions bellow (and suit your self).
First, you have to do an HTTP request to your javascript file and get its contents. Then, you can create an expression and evaluate it like bellow supplying the code obtained from the request (myCodeFromHttpRequest
).
eval('var myFunc = ' + myCodeFromHttpRequest);
myFunc();
This way you somehow mock what you would be trying to do if you had control over the code.
But, using eval
is bad practice, as MDN suggests, you can evaluate it to a function instead, so that it will create an isolated scope preventing javascript to do the global check for new variables and preventing your code from injecing insecure garbage on the global scope as you are adding a new piece of code on the running program.
var myFunc = Function('return ' + myCodeFromHttpRequest)();
myFunc();
That is basically creating a function
that returns your anonymous function and self executing it to produce the value and assign it to the myFunc
var.
You final solution would be something like this:
you can use
$.ajax
or a different HTTP client if you don't supportfetch
fetch('my-script.js').then(async(res) => {
var myCodeFromHttpRequest = await res.text();
var myFunc = Function('return ' + myCodeFromHttpRequest)()
myFunc();
});
Working snippet:
// fetch('my-script.js').then(async(res) => {
var myCodeFromHttpRequest = //await res.text();
// mocked code in order to simulate the request
'(function(data){' +
' this.init=function(){' +
' console.log("Hello world");' +
' };' +
' this.init();' +
'});';
var myFunc = Function('return ' + myCodeFromHttpRequest)()
myFunc();
//});
Thanks so much, but i guess is not a good practise :/ i'll try in other way
– Mcruz
Nov 23 at 19:11
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
You would have to attribute the anonymous function to a variable in order to get a reference to it and then be able to call it like the code bellow:
var myFunc = (function(data){...})
However, assuming you don't have control over the javascript to which you are trying to do it, you would have to evaluate the code as described by MDN in this chapter and build the previous expression.
UGLY CODING PRACTICES ALERT: this practice I'm about to propose is not at all a good practice, evaluating javascript code at runtime is not good and should be avoided unless it is extremely necessary. Having said that, and you still think you need to do this, follow the instructions bellow (and suit your self).
First, you have to do an HTTP request to your javascript file and get its contents. Then, you can create an expression and evaluate it like bellow supplying the code obtained from the request (myCodeFromHttpRequest
).
eval('var myFunc = ' + myCodeFromHttpRequest);
myFunc();
This way you somehow mock what you would be trying to do if you had control over the code.
But, using eval
is bad practice, as MDN suggests, you can evaluate it to a function instead, so that it will create an isolated scope preventing javascript to do the global check for new variables and preventing your code from injecing insecure garbage on the global scope as you are adding a new piece of code on the running program.
var myFunc = Function('return ' + myCodeFromHttpRequest)();
myFunc();
That is basically creating a function
that returns your anonymous function and self executing it to produce the value and assign it to the myFunc
var.
You final solution would be something like this:
you can use
$.ajax
or a different HTTP client if you don't supportfetch
fetch('my-script.js').then(async(res) => {
var myCodeFromHttpRequest = await res.text();
var myFunc = Function('return ' + myCodeFromHttpRequest)()
myFunc();
});
Working snippet:
// fetch('my-script.js').then(async(res) => {
var myCodeFromHttpRequest = //await res.text();
// mocked code in order to simulate the request
'(function(data){' +
' this.init=function(){' +
' console.log("Hello world");' +
' };' +
' this.init();' +
'});';
var myFunc = Function('return ' + myCodeFromHttpRequest)()
myFunc();
//});
Thanks so much, but i guess is not a good practise :/ i'll try in other way
– Mcruz
Nov 23 at 19:11
add a comment |
up vote
0
down vote
You would have to attribute the anonymous function to a variable in order to get a reference to it and then be able to call it like the code bellow:
var myFunc = (function(data){...})
However, assuming you don't have control over the javascript to which you are trying to do it, you would have to evaluate the code as described by MDN in this chapter and build the previous expression.
UGLY CODING PRACTICES ALERT: this practice I'm about to propose is not at all a good practice, evaluating javascript code at runtime is not good and should be avoided unless it is extremely necessary. Having said that, and you still think you need to do this, follow the instructions bellow (and suit your self).
First, you have to do an HTTP request to your javascript file and get its contents. Then, you can create an expression and evaluate it like bellow supplying the code obtained from the request (myCodeFromHttpRequest
).
eval('var myFunc = ' + myCodeFromHttpRequest);
myFunc();
This way you somehow mock what you would be trying to do if you had control over the code.
But, using eval
is bad practice, as MDN suggests, you can evaluate it to a function instead, so that it will create an isolated scope preventing javascript to do the global check for new variables and preventing your code from injecing insecure garbage on the global scope as you are adding a new piece of code on the running program.
var myFunc = Function('return ' + myCodeFromHttpRequest)();
myFunc();
That is basically creating a function
that returns your anonymous function and self executing it to produce the value and assign it to the myFunc
var.
You final solution would be something like this:
you can use
$.ajax
or a different HTTP client if you don't supportfetch
fetch('my-script.js').then(async(res) => {
var myCodeFromHttpRequest = await res.text();
var myFunc = Function('return ' + myCodeFromHttpRequest)()
myFunc();
});
Working snippet:
// fetch('my-script.js').then(async(res) => {
var myCodeFromHttpRequest = //await res.text();
// mocked code in order to simulate the request
'(function(data){' +
' this.init=function(){' +
' console.log("Hello world");' +
' };' +
' this.init();' +
'});';
var myFunc = Function('return ' + myCodeFromHttpRequest)()
myFunc();
//});
Thanks so much, but i guess is not a good practise :/ i'll try in other way
– Mcruz
Nov 23 at 19:11
add a comment |
up vote
0
down vote
up vote
0
down vote
You would have to attribute the anonymous function to a variable in order to get a reference to it and then be able to call it like the code bellow:
var myFunc = (function(data){...})
However, assuming you don't have control over the javascript to which you are trying to do it, you would have to evaluate the code as described by MDN in this chapter and build the previous expression.
UGLY CODING PRACTICES ALERT: this practice I'm about to propose is not at all a good practice, evaluating javascript code at runtime is not good and should be avoided unless it is extremely necessary. Having said that, and you still think you need to do this, follow the instructions bellow (and suit your self).
First, you have to do an HTTP request to your javascript file and get its contents. Then, you can create an expression and evaluate it like bellow supplying the code obtained from the request (myCodeFromHttpRequest
).
eval('var myFunc = ' + myCodeFromHttpRequest);
myFunc();
This way you somehow mock what you would be trying to do if you had control over the code.
But, using eval
is bad practice, as MDN suggests, you can evaluate it to a function instead, so that it will create an isolated scope preventing javascript to do the global check for new variables and preventing your code from injecing insecure garbage on the global scope as you are adding a new piece of code on the running program.
var myFunc = Function('return ' + myCodeFromHttpRequest)();
myFunc();
That is basically creating a function
that returns your anonymous function and self executing it to produce the value and assign it to the myFunc
var.
You final solution would be something like this:
you can use
$.ajax
or a different HTTP client if you don't supportfetch
fetch('my-script.js').then(async(res) => {
var myCodeFromHttpRequest = await res.text();
var myFunc = Function('return ' + myCodeFromHttpRequest)()
myFunc();
});
Working snippet:
// fetch('my-script.js').then(async(res) => {
var myCodeFromHttpRequest = //await res.text();
// mocked code in order to simulate the request
'(function(data){' +
' this.init=function(){' +
' console.log("Hello world");' +
' };' +
' this.init();' +
'});';
var myFunc = Function('return ' + myCodeFromHttpRequest)()
myFunc();
//});
You would have to attribute the anonymous function to a variable in order to get a reference to it and then be able to call it like the code bellow:
var myFunc = (function(data){...})
However, assuming you don't have control over the javascript to which you are trying to do it, you would have to evaluate the code as described by MDN in this chapter and build the previous expression.
UGLY CODING PRACTICES ALERT: this practice I'm about to propose is not at all a good practice, evaluating javascript code at runtime is not good and should be avoided unless it is extremely necessary. Having said that, and you still think you need to do this, follow the instructions bellow (and suit your self).
First, you have to do an HTTP request to your javascript file and get its contents. Then, you can create an expression and evaluate it like bellow supplying the code obtained from the request (myCodeFromHttpRequest
).
eval('var myFunc = ' + myCodeFromHttpRequest);
myFunc();
This way you somehow mock what you would be trying to do if you had control over the code.
But, using eval
is bad practice, as MDN suggests, you can evaluate it to a function instead, so that it will create an isolated scope preventing javascript to do the global check for new variables and preventing your code from injecing insecure garbage on the global scope as you are adding a new piece of code on the running program.
var myFunc = Function('return ' + myCodeFromHttpRequest)();
myFunc();
That is basically creating a function
that returns your anonymous function and self executing it to produce the value and assign it to the myFunc
var.
You final solution would be something like this:
you can use
$.ajax
or a different HTTP client if you don't supportfetch
fetch('my-script.js').then(async(res) => {
var myCodeFromHttpRequest = await res.text();
var myFunc = Function('return ' + myCodeFromHttpRequest)()
myFunc();
});
Working snippet:
// fetch('my-script.js').then(async(res) => {
var myCodeFromHttpRequest = //await res.text();
// mocked code in order to simulate the request
'(function(data){' +
' this.init=function(){' +
' console.log("Hello world");' +
' };' +
' this.init();' +
'});';
var myFunc = Function('return ' + myCodeFromHttpRequest)()
myFunc();
//});
// fetch('my-script.js').then(async(res) => {
var myCodeFromHttpRequest = //await res.text();
// mocked code in order to simulate the request
'(function(data){' +
' this.init=function(){' +
' console.log("Hello world");' +
' };' +
' this.init();' +
'});';
var myFunc = Function('return ' + myCodeFromHttpRequest)()
myFunc();
//});
// fetch('my-script.js').then(async(res) => {
var myCodeFromHttpRequest = //await res.text();
// mocked code in order to simulate the request
'(function(data){' +
' this.init=function(){' +
' console.log("Hello world");' +
' };' +
' this.init();' +
'});';
var myFunc = Function('return ' + myCodeFromHttpRequest)()
myFunc();
//});
answered Nov 22 at 18:14


lenilsondc
6,90211030
6,90211030
Thanks so much, but i guess is not a good practise :/ i'll try in other way
– Mcruz
Nov 23 at 19:11
add a comment |
Thanks so much, but i guess is not a good practise :/ i'll try in other way
– Mcruz
Nov 23 at 19:11
Thanks so much, but i guess is not a good practise :/ i'll try in other way
– Mcruz
Nov 23 at 19:11
Thanks so much, but i guess is not a good practise :/ i'll try in other way
– Mcruz
Nov 23 at 19:11
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53434243%2faccess-to-encapsulated-anonymous-function%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
UJqz,9,U9e fT4T8eHDjJyqAGDT6dDFxF QTqj3CF8crTRaH,11r2,RUQT
1
You can't do that as that is the point of an anonymous function.
– Scott Marcus
Nov 22 at 15:38
You have to assign it to a variable for example,
var myFunc = (function(data){...})
– lenilsondc
Nov 22 at 15:39
You want to call self executing function without self executing? Maybe use named function instead and when need auto execution simply call it?
– Justinas
Nov 22 at 15:39
Maybe you should assing the function to a globally accessible object (like window or document) and then you could access it from there?
– Marcelo Myara
Nov 22 at 15:40
@lenilsondc That wouldn't work. You'd need to remove the outer parenthesis, because with them the expression returns nothing.
– Scott Marcus
Nov 22 at 15:43