Maximum product of 13 adjacent numbers of a 1000-digit number
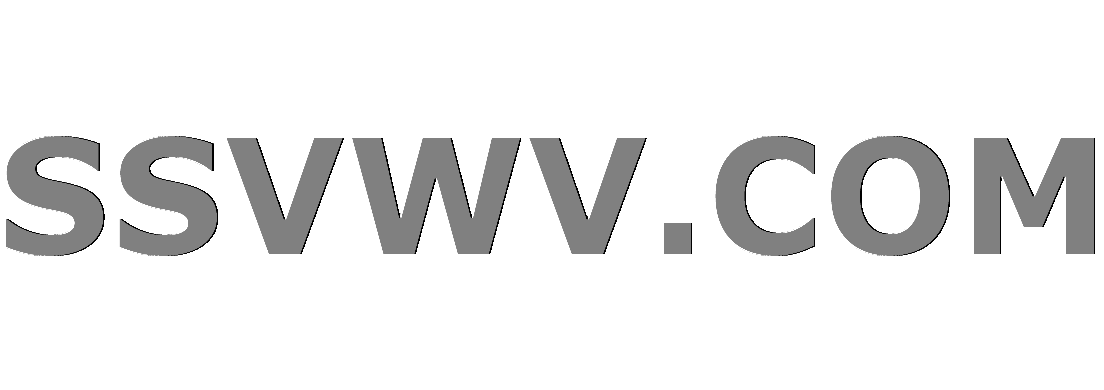
Multi tool use
I have to find the largest product of 13 adjacent numbers of a 1000-digit number below. My code for the problem is as follows:
#include <stdio.h>
int main()
{
char arr[1000] =
"731671765313306249192251196744265747423553491949349698352031277450"
"632623957831801698480186947885184385861560789112949495459501737958"
"331952853208805511125406987471585238630507156932909632952274430435"
"576689664895044524452316173185640309871112172238311362229893423380"
"308135336276614282806444486645238749303589072962904915604407723907"
"138105158593079608667017242712188399879790879227492190169972088809"
"377665727333001053367881220235421809751254540594752243525849077116"
"705560136048395864467063244157221553975369781797784617406495514929"
"086256932197846862248283972241375657056057490261407972968652414535"
"100474821663704844031998900088952434506585412275886668811642717147"
"992444292823086346567481391912316282458617866458359124566529476545"
"682848912883142607690042242190226710556263211111093705442175069416"
"589604080719840385096245544436298123098787992724428490918884580156"
"166097919133875499200524063689912560717606058861164671094050775410"
"022569831552000559357297257163626956188267042825248360082325753042"
"0752963450";
int i, j;
long int max;
max = 0;
long int s = 1;
for (i = 0; i < 988; i++) {
int a = 0;
for (j = 1; j <= 13; j++) {
printf("%c", arr[i + a]);
s = s * arr[i + a];
a++;
}
printf("%c%d", '=', s);
printf("n");
if (s > max) {
max = s;
}
}
printf("nMaximum product is %d", max);
getchar();
}
Some outputs are zero even if none of the input is zero. The second output happens to be negative. The answers don't even match. Any help is appreciated.
c
|
show 3 more comments
I have to find the largest product of 13 adjacent numbers of a 1000-digit number below. My code for the problem is as follows:
#include <stdio.h>
int main()
{
char arr[1000] =
"731671765313306249192251196744265747423553491949349698352031277450"
"632623957831801698480186947885184385861560789112949495459501737958"
"331952853208805511125406987471585238630507156932909632952274430435"
"576689664895044524452316173185640309871112172238311362229893423380"
"308135336276614282806444486645238749303589072962904915604407723907"
"138105158593079608667017242712188399879790879227492190169972088809"
"377665727333001053367881220235421809751254540594752243525849077116"
"705560136048395864467063244157221553975369781797784617406495514929"
"086256932197846862248283972241375657056057490261407972968652414535"
"100474821663704844031998900088952434506585412275886668811642717147"
"992444292823086346567481391912316282458617866458359124566529476545"
"682848912883142607690042242190226710556263211111093705442175069416"
"589604080719840385096245544436298123098787992724428490918884580156"
"166097919133875499200524063689912560717606058861164671094050775410"
"022569831552000559357297257163626956188267042825248360082325753042"
"0752963450";
int i, j;
long int max;
max = 0;
long int s = 1;
for (i = 0; i < 988; i++) {
int a = 0;
for (j = 1; j <= 13; j++) {
printf("%c", arr[i + a]);
s = s * arr[i + a];
a++;
}
printf("%c%d", '=', s);
printf("n");
if (s > max) {
max = s;
}
}
printf("nMaximum product is %d", max);
getchar();
}
Some outputs are zero even if none of the input is zero. The second output happens to be negative. The answers don't even match. Any help is appreciated.
c
2
There are zeros in your string. Why do you say that none of the input is 0?
– P.W
Nov 23 '18 at 5:14
looks like Project Euler problem 8
– phuclv
Nov 23 '18 at 5:31
1
@paddy I'm not acquainted with that accumulator ring buffer method ,would you please elaborate ?
– Nehal Samee
Nov 23 '18 at 5:46
1
It's just a common dynamic programming technique. You have a 13-value array containing the last 13 values that you have processed, and you also have a single value containing the product of all those values. When you add a new value to this array, you will be replacing the oldest value. If you divide that out of your product, then the product is now only the most recent 12 values. You then take the new one, multiply it into your product variable, and replace the oldest one in the array. If you encounter a zero, you reset everything, as if you were filling from the beginning.
– paddy
Nov 23 '18 at 10:20
1
Here, I knocked up an example just for fun: ideone.com/MKdNnL
– paddy
Nov 23 '18 at 10:40
|
show 3 more comments
I have to find the largest product of 13 adjacent numbers of a 1000-digit number below. My code for the problem is as follows:
#include <stdio.h>
int main()
{
char arr[1000] =
"731671765313306249192251196744265747423553491949349698352031277450"
"632623957831801698480186947885184385861560789112949495459501737958"
"331952853208805511125406987471585238630507156932909632952274430435"
"576689664895044524452316173185640309871112172238311362229893423380"
"308135336276614282806444486645238749303589072962904915604407723907"
"138105158593079608667017242712188399879790879227492190169972088809"
"377665727333001053367881220235421809751254540594752243525849077116"
"705560136048395864467063244157221553975369781797784617406495514929"
"086256932197846862248283972241375657056057490261407972968652414535"
"100474821663704844031998900088952434506585412275886668811642717147"
"992444292823086346567481391912316282458617866458359124566529476545"
"682848912883142607690042242190226710556263211111093705442175069416"
"589604080719840385096245544436298123098787992724428490918884580156"
"166097919133875499200524063689912560717606058861164671094050775410"
"022569831552000559357297257163626956188267042825248360082325753042"
"0752963450";
int i, j;
long int max;
max = 0;
long int s = 1;
for (i = 0; i < 988; i++) {
int a = 0;
for (j = 1; j <= 13; j++) {
printf("%c", arr[i + a]);
s = s * arr[i + a];
a++;
}
printf("%c%d", '=', s);
printf("n");
if (s > max) {
max = s;
}
}
printf("nMaximum product is %d", max);
getchar();
}
Some outputs are zero even if none of the input is zero. The second output happens to be negative. The answers don't even match. Any help is appreciated.
c
I have to find the largest product of 13 adjacent numbers of a 1000-digit number below. My code for the problem is as follows:
#include <stdio.h>
int main()
{
char arr[1000] =
"731671765313306249192251196744265747423553491949349698352031277450"
"632623957831801698480186947885184385861560789112949495459501737958"
"331952853208805511125406987471585238630507156932909632952274430435"
"576689664895044524452316173185640309871112172238311362229893423380"
"308135336276614282806444486645238749303589072962904915604407723907"
"138105158593079608667017242712188399879790879227492190169972088809"
"377665727333001053367881220235421809751254540594752243525849077116"
"705560136048395864467063244157221553975369781797784617406495514929"
"086256932197846862248283972241375657056057490261407972968652414535"
"100474821663704844031998900088952434506585412275886668811642717147"
"992444292823086346567481391912316282458617866458359124566529476545"
"682848912883142607690042242190226710556263211111093705442175069416"
"589604080719840385096245544436298123098787992724428490918884580156"
"166097919133875499200524063689912560717606058861164671094050775410"
"022569831552000559357297257163626956188267042825248360082325753042"
"0752963450";
int i, j;
long int max;
max = 0;
long int s = 1;
for (i = 0; i < 988; i++) {
int a = 0;
for (j = 1; j <= 13; j++) {
printf("%c", arr[i + a]);
s = s * arr[i + a];
a++;
}
printf("%c%d", '=', s);
printf("n");
if (s > max) {
max = s;
}
}
printf("nMaximum product is %d", max);
getchar();
}
Some outputs are zero even if none of the input is zero. The second output happens to be negative. The answers don't even match. Any help is appreciated.
c
c
edited Nov 23 '18 at 5:11


Swordfish
1
1
asked Nov 23 '18 at 5:02


Nehal Samee
1066
1066
2
There are zeros in your string. Why do you say that none of the input is 0?
– P.W
Nov 23 '18 at 5:14
looks like Project Euler problem 8
– phuclv
Nov 23 '18 at 5:31
1
@paddy I'm not acquainted with that accumulator ring buffer method ,would you please elaborate ?
– Nehal Samee
Nov 23 '18 at 5:46
1
It's just a common dynamic programming technique. You have a 13-value array containing the last 13 values that you have processed, and you also have a single value containing the product of all those values. When you add a new value to this array, you will be replacing the oldest value. If you divide that out of your product, then the product is now only the most recent 12 values. You then take the new one, multiply it into your product variable, and replace the oldest one in the array. If you encounter a zero, you reset everything, as if you were filling from the beginning.
– paddy
Nov 23 '18 at 10:20
1
Here, I knocked up an example just for fun: ideone.com/MKdNnL
– paddy
Nov 23 '18 at 10:40
|
show 3 more comments
2
There are zeros in your string. Why do you say that none of the input is 0?
– P.W
Nov 23 '18 at 5:14
looks like Project Euler problem 8
– phuclv
Nov 23 '18 at 5:31
1
@paddy I'm not acquainted with that accumulator ring buffer method ,would you please elaborate ?
– Nehal Samee
Nov 23 '18 at 5:46
1
It's just a common dynamic programming technique. You have a 13-value array containing the last 13 values that you have processed, and you also have a single value containing the product of all those values. When you add a new value to this array, you will be replacing the oldest value. If you divide that out of your product, then the product is now only the most recent 12 values. You then take the new one, multiply it into your product variable, and replace the oldest one in the array. If you encounter a zero, you reset everything, as if you were filling from the beginning.
– paddy
Nov 23 '18 at 10:20
1
Here, I knocked up an example just for fun: ideone.com/MKdNnL
– paddy
Nov 23 '18 at 10:40
2
2
There are zeros in your string. Why do you say that none of the input is 0?
– P.W
Nov 23 '18 at 5:14
There are zeros in your string. Why do you say that none of the input is 0?
– P.W
Nov 23 '18 at 5:14
looks like Project Euler problem 8
– phuclv
Nov 23 '18 at 5:31
looks like Project Euler problem 8
– phuclv
Nov 23 '18 at 5:31
1
1
@paddy I'm not acquainted with that accumulator ring buffer method ,would you please elaborate ?
– Nehal Samee
Nov 23 '18 at 5:46
@paddy I'm not acquainted with that accumulator ring buffer method ,would you please elaborate ?
– Nehal Samee
Nov 23 '18 at 5:46
1
1
It's just a common dynamic programming technique. You have a 13-value array containing the last 13 values that you have processed, and you also have a single value containing the product of all those values. When you add a new value to this array, you will be replacing the oldest value. If you divide that out of your product, then the product is now only the most recent 12 values. You then take the new one, multiply it into your product variable, and replace the oldest one in the array. If you encounter a zero, you reset everything, as if you were filling from the beginning.
– paddy
Nov 23 '18 at 10:20
It's just a common dynamic programming technique. You have a 13-value array containing the last 13 values that you have processed, and you also have a single value containing the product of all those values. When you add a new value to this array, you will be replacing the oldest value. If you divide that out of your product, then the product is now only the most recent 12 values. You then take the new one, multiply it into your product variable, and replace the oldest one in the array. If you encounter a zero, you reset everything, as if you were filling from the beginning.
– paddy
Nov 23 '18 at 10:20
1
1
Here, I knocked up an example just for fun: ideone.com/MKdNnL
– paddy
Nov 23 '18 at 10:40
Here, I knocked up an example just for fun: ideone.com/MKdNnL
– paddy
Nov 23 '18 at 10:40
|
show 3 more comments
2 Answers
2
active
oldest
votes
Many set of 13 digits in your char
array arr
contains zeroes and that is why the multiplication of these sets will result in 0.
There are a couple of issues with your code:
- You are using
%d
instead of%ld
to printlong int
. Using the wrong conversion specifier will result in undefined behaviour.
If any argument is not the correct type for the corresponding conversion specification, the behavior is undefined.
- You are not converting the ASCII value of the digit into its actual value before multiplication. (ASCII value of '0' is 48). This results in integer overflow and is the cause for negative values to be printed.
So the statement:
s = s * arr[i + a];
should be changed to:
s = s * (arr[i + a] - '0');
- You are also not resetting the product
s
to 1 at the beginning of the innerfor
loop and because of this, you end up multiplying values from the results of different sets of 13.
After making these changes, you can see the live demo here.
I got it already though ...but how do I write it in the answer section ?
– Nehal Samee
Nov 23 '18 at 5:47
@NehalSamee: You mean the answer section here on SO?
– P.W
Nov 23 '18 at 5:48
No...In PE ...how do I write the two answers ...If I write in format 2×3×4 ...×0 I don't have enough spaces then .
– Nehal Samee
Nov 23 '18 at 5:49
1
@NehalSamee: I am not familiar with PE. But you only have to write answer that is working. No need for two answers.
– P.W
Nov 23 '18 at 5:52
Note, I followed the live demo link and found it did not match this answer. I took the liberty of fixing it, and also corrected an off-by-one error in the outer loop, and made the inner loop more standard (zero-based indexing) even though for some reason there is a second variable used to count the offset.
– paddy
Nov 23 '18 at 11:01
|
show 1 more comment
There are a few issues to tackle in this code:
Clean up spacing and variable names (an edit by another user helped resolve this issue). Remove redundant variables like
a
, whichj
could easily represent by iterating from 0 to 12 rather than 1 to 13. This seems cosmetic but will make it easier for you to understand your program state, so it's actually critical.Numerical overflow: As with all PE problems, you'll be dealing with extremely large numbers which may overflow the capacity of the
long int
datatype (231 - 1). Useunsigned long long
to store yourmax
ands
(which I'd callproduct
) variables. Print the result with%llu
.Convert
char
s toint
s:arr[i+j] - '0';
so that you're multiplying the actual numbers the chars represent rather than their ASCII values (which are 48 higher).s
(reallyproduct
) is not reset on each iteration of the inner loop, so you're taking the product of the entire 1000-sized input (or trying to, until yourint
s start to overflow).
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53440887%2fmaximum-product-of-13-adjacent-numbers-of-a-1000-digit-number%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
Many set of 13 digits in your char
array arr
contains zeroes and that is why the multiplication of these sets will result in 0.
There are a couple of issues with your code:
- You are using
%d
instead of%ld
to printlong int
. Using the wrong conversion specifier will result in undefined behaviour.
If any argument is not the correct type for the corresponding conversion specification, the behavior is undefined.
- You are not converting the ASCII value of the digit into its actual value before multiplication. (ASCII value of '0' is 48). This results in integer overflow and is the cause for negative values to be printed.
So the statement:
s = s * arr[i + a];
should be changed to:
s = s * (arr[i + a] - '0');
- You are also not resetting the product
s
to 1 at the beginning of the innerfor
loop and because of this, you end up multiplying values from the results of different sets of 13.
After making these changes, you can see the live demo here.
I got it already though ...but how do I write it in the answer section ?
– Nehal Samee
Nov 23 '18 at 5:47
@NehalSamee: You mean the answer section here on SO?
– P.W
Nov 23 '18 at 5:48
No...In PE ...how do I write the two answers ...If I write in format 2×3×4 ...×0 I don't have enough spaces then .
– Nehal Samee
Nov 23 '18 at 5:49
1
@NehalSamee: I am not familiar with PE. But you only have to write answer that is working. No need for two answers.
– P.W
Nov 23 '18 at 5:52
Note, I followed the live demo link and found it did not match this answer. I took the liberty of fixing it, and also corrected an off-by-one error in the outer loop, and made the inner loop more standard (zero-based indexing) even though for some reason there is a second variable used to count the offset.
– paddy
Nov 23 '18 at 11:01
|
show 1 more comment
Many set of 13 digits in your char
array arr
contains zeroes and that is why the multiplication of these sets will result in 0.
There are a couple of issues with your code:
- You are using
%d
instead of%ld
to printlong int
. Using the wrong conversion specifier will result in undefined behaviour.
If any argument is not the correct type for the corresponding conversion specification, the behavior is undefined.
- You are not converting the ASCII value of the digit into its actual value before multiplication. (ASCII value of '0' is 48). This results in integer overflow and is the cause for negative values to be printed.
So the statement:
s = s * arr[i + a];
should be changed to:
s = s * (arr[i + a] - '0');
- You are also not resetting the product
s
to 1 at the beginning of the innerfor
loop and because of this, you end up multiplying values from the results of different sets of 13.
After making these changes, you can see the live demo here.
I got it already though ...but how do I write it in the answer section ?
– Nehal Samee
Nov 23 '18 at 5:47
@NehalSamee: You mean the answer section here on SO?
– P.W
Nov 23 '18 at 5:48
No...In PE ...how do I write the two answers ...If I write in format 2×3×4 ...×0 I don't have enough spaces then .
– Nehal Samee
Nov 23 '18 at 5:49
1
@NehalSamee: I am not familiar with PE. But you only have to write answer that is working. No need for two answers.
– P.W
Nov 23 '18 at 5:52
Note, I followed the live demo link and found it did not match this answer. I took the liberty of fixing it, and also corrected an off-by-one error in the outer loop, and made the inner loop more standard (zero-based indexing) even though for some reason there is a second variable used to count the offset.
– paddy
Nov 23 '18 at 11:01
|
show 1 more comment
Many set of 13 digits in your char
array arr
contains zeroes and that is why the multiplication of these sets will result in 0.
There are a couple of issues with your code:
- You are using
%d
instead of%ld
to printlong int
. Using the wrong conversion specifier will result in undefined behaviour.
If any argument is not the correct type for the corresponding conversion specification, the behavior is undefined.
- You are not converting the ASCII value of the digit into its actual value before multiplication. (ASCII value of '0' is 48). This results in integer overflow and is the cause for negative values to be printed.
So the statement:
s = s * arr[i + a];
should be changed to:
s = s * (arr[i + a] - '0');
- You are also not resetting the product
s
to 1 at the beginning of the innerfor
loop and because of this, you end up multiplying values from the results of different sets of 13.
After making these changes, you can see the live demo here.
Many set of 13 digits in your char
array arr
contains zeroes and that is why the multiplication of these sets will result in 0.
There are a couple of issues with your code:
- You are using
%d
instead of%ld
to printlong int
. Using the wrong conversion specifier will result in undefined behaviour.
If any argument is not the correct type for the corresponding conversion specification, the behavior is undefined.
- You are not converting the ASCII value of the digit into its actual value before multiplication. (ASCII value of '0' is 48). This results in integer overflow and is the cause for negative values to be printed.
So the statement:
s = s * arr[i + a];
should be changed to:
s = s * (arr[i + a] - '0');
- You are also not resetting the product
s
to 1 at the beginning of the innerfor
loop and because of this, you end up multiplying values from the results of different sets of 13.
After making these changes, you can see the live demo here.
edited Nov 23 '18 at 10:55


paddy
42.5k53076
42.5k53076
answered Nov 23 '18 at 5:27
P.W
10.9k3742
10.9k3742
I got it already though ...but how do I write it in the answer section ?
– Nehal Samee
Nov 23 '18 at 5:47
@NehalSamee: You mean the answer section here on SO?
– P.W
Nov 23 '18 at 5:48
No...In PE ...how do I write the two answers ...If I write in format 2×3×4 ...×0 I don't have enough spaces then .
– Nehal Samee
Nov 23 '18 at 5:49
1
@NehalSamee: I am not familiar with PE. But you only have to write answer that is working. No need for two answers.
– P.W
Nov 23 '18 at 5:52
Note, I followed the live demo link and found it did not match this answer. I took the liberty of fixing it, and also corrected an off-by-one error in the outer loop, and made the inner loop more standard (zero-based indexing) even though for some reason there is a second variable used to count the offset.
– paddy
Nov 23 '18 at 11:01
|
show 1 more comment
I got it already though ...but how do I write it in the answer section ?
– Nehal Samee
Nov 23 '18 at 5:47
@NehalSamee: You mean the answer section here on SO?
– P.W
Nov 23 '18 at 5:48
No...In PE ...how do I write the two answers ...If I write in format 2×3×4 ...×0 I don't have enough spaces then .
– Nehal Samee
Nov 23 '18 at 5:49
1
@NehalSamee: I am not familiar with PE. But you only have to write answer that is working. No need for two answers.
– P.W
Nov 23 '18 at 5:52
Note, I followed the live demo link and found it did not match this answer. I took the liberty of fixing it, and also corrected an off-by-one error in the outer loop, and made the inner loop more standard (zero-based indexing) even though for some reason there is a second variable used to count the offset.
– paddy
Nov 23 '18 at 11:01
I got it already though ...but how do I write it in the answer section ?
– Nehal Samee
Nov 23 '18 at 5:47
I got it already though ...but how do I write it in the answer section ?
– Nehal Samee
Nov 23 '18 at 5:47
@NehalSamee: You mean the answer section here on SO?
– P.W
Nov 23 '18 at 5:48
@NehalSamee: You mean the answer section here on SO?
– P.W
Nov 23 '18 at 5:48
No...In PE ...how do I write the two answers ...If I write in format 2×3×4 ...×0 I don't have enough spaces then .
– Nehal Samee
Nov 23 '18 at 5:49
No...In PE ...how do I write the two answers ...If I write in format 2×3×4 ...×0 I don't have enough spaces then .
– Nehal Samee
Nov 23 '18 at 5:49
1
1
@NehalSamee: I am not familiar with PE. But you only have to write answer that is working. No need for two answers.
– P.W
Nov 23 '18 at 5:52
@NehalSamee: I am not familiar with PE. But you only have to write answer that is working. No need for two answers.
– P.W
Nov 23 '18 at 5:52
Note, I followed the live demo link and found it did not match this answer. I took the liberty of fixing it, and also corrected an off-by-one error in the outer loop, and made the inner loop more standard (zero-based indexing) even though for some reason there is a second variable used to count the offset.
– paddy
Nov 23 '18 at 11:01
Note, I followed the live demo link and found it did not match this answer. I took the liberty of fixing it, and also corrected an off-by-one error in the outer loop, and made the inner loop more standard (zero-based indexing) even though for some reason there is a second variable used to count the offset.
– paddy
Nov 23 '18 at 11:01
|
show 1 more comment
There are a few issues to tackle in this code:
Clean up spacing and variable names (an edit by another user helped resolve this issue). Remove redundant variables like
a
, whichj
could easily represent by iterating from 0 to 12 rather than 1 to 13. This seems cosmetic but will make it easier for you to understand your program state, so it's actually critical.Numerical overflow: As with all PE problems, you'll be dealing with extremely large numbers which may overflow the capacity of the
long int
datatype (231 - 1). Useunsigned long long
to store yourmax
ands
(which I'd callproduct
) variables. Print the result with%llu
.Convert
char
s toint
s:arr[i+j] - '0';
so that you're multiplying the actual numbers the chars represent rather than their ASCII values (which are 48 higher).s
(reallyproduct
) is not reset on each iteration of the inner loop, so you're taking the product of the entire 1000-sized input (or trying to, until yourint
s start to overflow).
add a comment |
There are a few issues to tackle in this code:
Clean up spacing and variable names (an edit by another user helped resolve this issue). Remove redundant variables like
a
, whichj
could easily represent by iterating from 0 to 12 rather than 1 to 13. This seems cosmetic but will make it easier for you to understand your program state, so it's actually critical.Numerical overflow: As with all PE problems, you'll be dealing with extremely large numbers which may overflow the capacity of the
long int
datatype (231 - 1). Useunsigned long long
to store yourmax
ands
(which I'd callproduct
) variables. Print the result with%llu
.Convert
char
s toint
s:arr[i+j] - '0';
so that you're multiplying the actual numbers the chars represent rather than their ASCII values (which are 48 higher).s
(reallyproduct
) is not reset on each iteration of the inner loop, so you're taking the product of the entire 1000-sized input (or trying to, until yourint
s start to overflow).
add a comment |
There are a few issues to tackle in this code:
Clean up spacing and variable names (an edit by another user helped resolve this issue). Remove redundant variables like
a
, whichj
could easily represent by iterating from 0 to 12 rather than 1 to 13. This seems cosmetic but will make it easier for you to understand your program state, so it's actually critical.Numerical overflow: As with all PE problems, you'll be dealing with extremely large numbers which may overflow the capacity of the
long int
datatype (231 - 1). Useunsigned long long
to store yourmax
ands
(which I'd callproduct
) variables. Print the result with%llu
.Convert
char
s toint
s:arr[i+j] - '0';
so that you're multiplying the actual numbers the chars represent rather than their ASCII values (which are 48 higher).s
(reallyproduct
) is not reset on each iteration of the inner loop, so you're taking the product of the entire 1000-sized input (or trying to, until yourint
s start to overflow).
There are a few issues to tackle in this code:
Clean up spacing and variable names (an edit by another user helped resolve this issue). Remove redundant variables like
a
, whichj
could easily represent by iterating from 0 to 12 rather than 1 to 13. This seems cosmetic but will make it easier for you to understand your program state, so it's actually critical.Numerical overflow: As with all PE problems, you'll be dealing with extremely large numbers which may overflow the capacity of the
long int
datatype (231 - 1). Useunsigned long long
to store yourmax
ands
(which I'd callproduct
) variables. Print the result with%llu
.Convert
char
s toint
s:arr[i+j] - '0';
so that you're multiplying the actual numbers the chars represent rather than their ASCII values (which are 48 higher).s
(reallyproduct
) is not reset on each iteration of the inner loop, so you're taking the product of the entire 1000-sized input (or trying to, until yourint
s start to overflow).
edited Nov 23 '18 at 5:48
answered Nov 23 '18 at 5:30
ggorlen
6,4413825
6,4413825
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53440887%2fmaximum-product-of-13-adjacent-numbers-of-a-1000-digit-number%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
l 0nkE,8OeYq,CG,07mkhq5Ifmoe,84D0SPw AC9asuDkMoOudN00MRHG7gDqrv IR 35mlFO,e aOV9Qwe
2
There are zeros in your string. Why do you say that none of the input is 0?
– P.W
Nov 23 '18 at 5:14
looks like Project Euler problem 8
– phuclv
Nov 23 '18 at 5:31
1
@paddy I'm not acquainted with that accumulator ring buffer method ,would you please elaborate ?
– Nehal Samee
Nov 23 '18 at 5:46
1
It's just a common dynamic programming technique. You have a 13-value array containing the last 13 values that you have processed, and you also have a single value containing the product of all those values. When you add a new value to this array, you will be replacing the oldest value. If you divide that out of your product, then the product is now only the most recent 12 values. You then take the new one, multiply it into your product variable, and replace the oldest one in the array. If you encounter a zero, you reset everything, as if you were filling from the beginning.
– paddy
Nov 23 '18 at 10:20
1
Here, I knocked up an example just for fun: ideone.com/MKdNnL
– paddy
Nov 23 '18 at 10:40