D3 Multi Line Chart Not Displaying Properly With X Axis Value
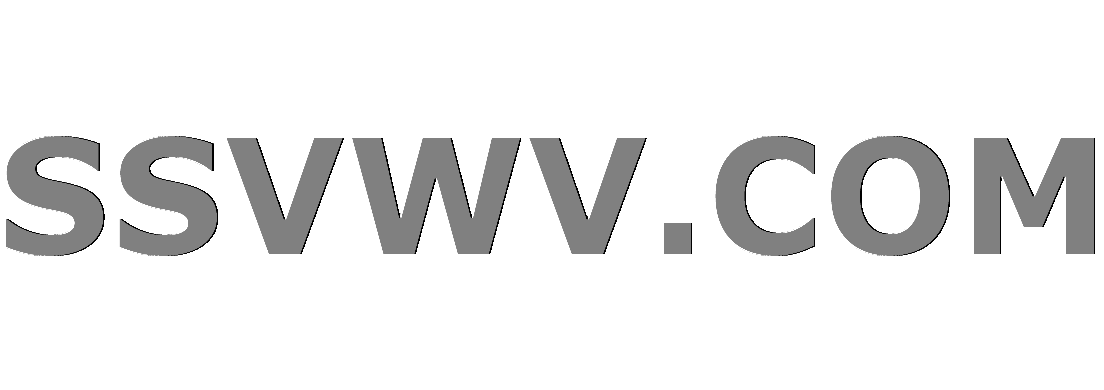
Multi tool use
I have implemented a D3v3 Multi-line chart in my application. As I'm quite new to D3 charts I looked for some online resources and came up with this. Initially, I had to display Years on X-axis and it was working properly. This fiddle shows it (https://jsfiddle.net/yasirunilan/tz57wc29/13/). Then according to a new requirement I need to show the data distribution with months in X-Axis, The difference is the X-axis doesn't always start with the month of January. As given in the Dataset it might start from October of a year to October of a year to September of Next Year. In that case, I faced a problem in displaying the line and X-Axis values in the chart. Can someone provide direction for this problem?
Fiddle: https://jsfiddle.net/yasirunilan/v160sr4z/10/
var data = [{
name: "USA",
values: [{
date: "October",
price: "100"
},
{
date: "November",
price: "110"
},
{
date: "December",
price: "145"
},
{
date: "January",
price: "241"
},
{
date: "February",
price: "101"
},
{
date: "March",
price: "90"
},
{
date: "April",
price: "120"
},
{
date: "May",
price: "135"
},
{
date: "June",
price: "99"
},
{
date: "July",
price: "105"
},
{
date: "August",
price: "101"
},
{
date: "September",
price: "106"
}
]
},
{
name: "UK",
values: [{
date: "October",
price: "130"
},
{
date: "November",
price: "120"
},
{
date: "December",
price: "115"
},
{
date: "January",
price: "220"
},
{
date: "February",
price: "100"
},
{
date: "March",
price: "140"
},
{
date: "April",
price: "90"
},
{
date: "May",
price: "235"
},
{
date: "June",
price: "160"
},
{
date: "July",
price: "86"
},
{
date: "August",
price: "201"
},
{
date: "September",
price: "140"
}
]
}
];
const margin = 80;
const width = 1000 - 2 * margin;
const height = 550 - 2 * margin;
var duration = 250;
var lineOpacity = "0.25";
var lineOpacityHover = "0.85";
var otherLinesOpacityHover = "0.1";
var lineStroke = "1.5px";
var lineStrokeHover = "2.5px";
var circleOpacity = '0.85';
var circleOpacityOnLineHover = "0.25"
var circleRadius = 3;
var circleRadiusHover = 6;
/* Format Data */
var parseDate = d3.time.format("%B");
data.forEach(function(d) {
d.values.forEach(function(d) {
d.date = parseDate.parse(d.date);
d.price = +d.price;
});
});
/* Scale */
var xScale = d3.time.scale()
.domain(d3.extent(data[0].values, d => d.date))
.range([0, width - margin]);
var yScale = d3.scale.linear()
.domain([0, d3.max(data[0].values, d => d.price)])
.range([height - margin, 0]);
// var color = d3.scale.ordinal(d3.schemeCategory10);
var color = d3.scale.category10();
/* Add SVG */
var svg = d3.select("svg")
.attr("width", (width + margin) + "px")
.attr("height", (height + margin) + "px")
.append('g')
.attr("transform", `translate(${margin}, ${margin})`);
/* Add line into SVG */
var line = d3.svg.line()
.x(d => xScale(d.date))
.y(d => yScale(d.price));
let lines = svg.append('g')
.attr('class', 'lines');
lines.selectAll('.line-group')
.data(data).enter()
.append('g')
.attr('class', 'line-group')
.on("mouseover", function(d, i) {
svg.append("text")
.attr("class", "title-text")
.style("fill", color(i))
.text(d.name)
.attr("text-anchor", "middle")
.attr("x", (width - margin) / 2)
.attr("y", 5);
})
.on("mouseout", function(d) {
svg.select(".title-text").remove();
})
.append('path')
.attr('class', 'line')
.attr('d', d => line(d.values))
.style('stroke', (d, i) => color(i))
.style('opacity', lineOpacity)
.on("mouseover", function(d) {
d3.selectAll('.line')
.style('opacity', otherLinesOpacityHover);
d3.selectAll('.circle')
.style('opacity', circleOpacityOnLineHover);
d3.select(this)
.style('opacity', lineOpacityHover)
.style("stroke-width", lineStrokeHover)
.style("cursor", "pointer");
})
.on("mouseout", function(d) {
d3.selectAll(".line")
.style('opacity', lineOpacity);
d3.selectAll('.circle')
.style('opacity', circleOpacity);
d3.select(this)
.style("stroke-width", lineStroke)
.style("cursor", "none");
});
/* Add circles in the line */
lines.selectAll("circle-group")
.data(data).enter()
.append("g")
.style("fill", (d, i) => color(i))
.selectAll("circle")
.data(d => d.values).enter()
.append("g")
.attr("class", "circle")
.on("mouseover", function(d) {
d3.select(this)
.style("cursor", "pointer")
.append("text")
.attr("class", "text")
.text(`${d.price}`)
.attr("x", d => xScale(d.date) + 5)
.attr("y", d => yScale(d.price) - 10);
})
.on("mouseout", function(d) {
d3.select(this)
.style("cursor", "none")
.transition()
.duration(duration)
.selectAll(".text").remove();
})
.append("circle")
.attr("cx", d => xScale(d.date))
.attr("cy", d => yScale(d.price))
.attr("r", circleRadius)
.style('opacity', circleOpacity)
.on("mouseover", function(d) {
d3.select(this)
.transition()
.duration(duration)
.attr("r", circleRadiusHover);
})
.on("mouseout", function(d) {
d3.select(this)
.transition()
.duration(duration)
.attr("r", circleRadius);
});
var xAxis = d3.svg.axis().scale(xScale)
.orient("bottom").tickFormat(d3.time.format("%B")).tickSize(1);
var yAxis = d3.svg.axis().scale(yScale)
.orient("left").tickSize(1);
svg.append("g")
.attr("class", "x axis")
.attr("transform", `translate(0, ${height-margin})`)
.call(xAxis.ticks(d3.time.year));
svg.append("g")
.attr("class", "y axis")
.call(yAxis)
.append('text')
.attr("y", 15)
.attr("transform", "rotate(-90)")
.attr("fill", "#000")
.attr('text-anchor', 'middle')
.text("No. of Employees");
javascript d3.js linechart xscale
add a comment |
I have implemented a D3v3 Multi-line chart in my application. As I'm quite new to D3 charts I looked for some online resources and came up with this. Initially, I had to display Years on X-axis and it was working properly. This fiddle shows it (https://jsfiddle.net/yasirunilan/tz57wc29/13/). Then according to a new requirement I need to show the data distribution with months in X-Axis, The difference is the X-axis doesn't always start with the month of January. As given in the Dataset it might start from October of a year to October of a year to September of Next Year. In that case, I faced a problem in displaying the line and X-Axis values in the chart. Can someone provide direction for this problem?
Fiddle: https://jsfiddle.net/yasirunilan/v160sr4z/10/
var data = [{
name: "USA",
values: [{
date: "October",
price: "100"
},
{
date: "November",
price: "110"
},
{
date: "December",
price: "145"
},
{
date: "January",
price: "241"
},
{
date: "February",
price: "101"
},
{
date: "March",
price: "90"
},
{
date: "April",
price: "120"
},
{
date: "May",
price: "135"
},
{
date: "June",
price: "99"
},
{
date: "July",
price: "105"
},
{
date: "August",
price: "101"
},
{
date: "September",
price: "106"
}
]
},
{
name: "UK",
values: [{
date: "October",
price: "130"
},
{
date: "November",
price: "120"
},
{
date: "December",
price: "115"
},
{
date: "January",
price: "220"
},
{
date: "February",
price: "100"
},
{
date: "March",
price: "140"
},
{
date: "April",
price: "90"
},
{
date: "May",
price: "235"
},
{
date: "June",
price: "160"
},
{
date: "July",
price: "86"
},
{
date: "August",
price: "201"
},
{
date: "September",
price: "140"
}
]
}
];
const margin = 80;
const width = 1000 - 2 * margin;
const height = 550 - 2 * margin;
var duration = 250;
var lineOpacity = "0.25";
var lineOpacityHover = "0.85";
var otherLinesOpacityHover = "0.1";
var lineStroke = "1.5px";
var lineStrokeHover = "2.5px";
var circleOpacity = '0.85';
var circleOpacityOnLineHover = "0.25"
var circleRadius = 3;
var circleRadiusHover = 6;
/* Format Data */
var parseDate = d3.time.format("%B");
data.forEach(function(d) {
d.values.forEach(function(d) {
d.date = parseDate.parse(d.date);
d.price = +d.price;
});
});
/* Scale */
var xScale = d3.time.scale()
.domain(d3.extent(data[0].values, d => d.date))
.range([0, width - margin]);
var yScale = d3.scale.linear()
.domain([0, d3.max(data[0].values, d => d.price)])
.range([height - margin, 0]);
// var color = d3.scale.ordinal(d3.schemeCategory10);
var color = d3.scale.category10();
/* Add SVG */
var svg = d3.select("svg")
.attr("width", (width + margin) + "px")
.attr("height", (height + margin) + "px")
.append('g')
.attr("transform", `translate(${margin}, ${margin})`);
/* Add line into SVG */
var line = d3.svg.line()
.x(d => xScale(d.date))
.y(d => yScale(d.price));
let lines = svg.append('g')
.attr('class', 'lines');
lines.selectAll('.line-group')
.data(data).enter()
.append('g')
.attr('class', 'line-group')
.on("mouseover", function(d, i) {
svg.append("text")
.attr("class", "title-text")
.style("fill", color(i))
.text(d.name)
.attr("text-anchor", "middle")
.attr("x", (width - margin) / 2)
.attr("y", 5);
})
.on("mouseout", function(d) {
svg.select(".title-text").remove();
})
.append('path')
.attr('class', 'line')
.attr('d', d => line(d.values))
.style('stroke', (d, i) => color(i))
.style('opacity', lineOpacity)
.on("mouseover", function(d) {
d3.selectAll('.line')
.style('opacity', otherLinesOpacityHover);
d3.selectAll('.circle')
.style('opacity', circleOpacityOnLineHover);
d3.select(this)
.style('opacity', lineOpacityHover)
.style("stroke-width", lineStrokeHover)
.style("cursor", "pointer");
})
.on("mouseout", function(d) {
d3.selectAll(".line")
.style('opacity', lineOpacity);
d3.selectAll('.circle')
.style('opacity', circleOpacity);
d3.select(this)
.style("stroke-width", lineStroke)
.style("cursor", "none");
});
/* Add circles in the line */
lines.selectAll("circle-group")
.data(data).enter()
.append("g")
.style("fill", (d, i) => color(i))
.selectAll("circle")
.data(d => d.values).enter()
.append("g")
.attr("class", "circle")
.on("mouseover", function(d) {
d3.select(this)
.style("cursor", "pointer")
.append("text")
.attr("class", "text")
.text(`${d.price}`)
.attr("x", d => xScale(d.date) + 5)
.attr("y", d => yScale(d.price) - 10);
})
.on("mouseout", function(d) {
d3.select(this)
.style("cursor", "none")
.transition()
.duration(duration)
.selectAll(".text").remove();
})
.append("circle")
.attr("cx", d => xScale(d.date))
.attr("cy", d => yScale(d.price))
.attr("r", circleRadius)
.style('opacity', circleOpacity)
.on("mouseover", function(d) {
d3.select(this)
.transition()
.duration(duration)
.attr("r", circleRadiusHover);
})
.on("mouseout", function(d) {
d3.select(this)
.transition()
.duration(duration)
.attr("r", circleRadius);
});
var xAxis = d3.svg.axis().scale(xScale)
.orient("bottom").tickFormat(d3.time.format("%B")).tickSize(1);
var yAxis = d3.svg.axis().scale(yScale)
.orient("left").tickSize(1);
svg.append("g")
.attr("class", "x axis")
.attr("transform", `translate(0, ${height-margin})`)
.call(xAxis.ticks(d3.time.year));
svg.append("g")
.attr("class", "y axis")
.call(yAxis)
.append('text')
.attr("y", 15)
.attr("transform", "rotate(-90)")
.attr("fill", "#000")
.attr('text-anchor', 'middle')
.text("No. of Employees");
javascript d3.js linechart xscale
add a comment |
I have implemented a D3v3 Multi-line chart in my application. As I'm quite new to D3 charts I looked for some online resources and came up with this. Initially, I had to display Years on X-axis and it was working properly. This fiddle shows it (https://jsfiddle.net/yasirunilan/tz57wc29/13/). Then according to a new requirement I need to show the data distribution with months in X-Axis, The difference is the X-axis doesn't always start with the month of January. As given in the Dataset it might start from October of a year to October of a year to September of Next Year. In that case, I faced a problem in displaying the line and X-Axis values in the chart. Can someone provide direction for this problem?
Fiddle: https://jsfiddle.net/yasirunilan/v160sr4z/10/
var data = [{
name: "USA",
values: [{
date: "October",
price: "100"
},
{
date: "November",
price: "110"
},
{
date: "December",
price: "145"
},
{
date: "January",
price: "241"
},
{
date: "February",
price: "101"
},
{
date: "March",
price: "90"
},
{
date: "April",
price: "120"
},
{
date: "May",
price: "135"
},
{
date: "June",
price: "99"
},
{
date: "July",
price: "105"
},
{
date: "August",
price: "101"
},
{
date: "September",
price: "106"
}
]
},
{
name: "UK",
values: [{
date: "October",
price: "130"
},
{
date: "November",
price: "120"
},
{
date: "December",
price: "115"
},
{
date: "January",
price: "220"
},
{
date: "February",
price: "100"
},
{
date: "March",
price: "140"
},
{
date: "April",
price: "90"
},
{
date: "May",
price: "235"
},
{
date: "June",
price: "160"
},
{
date: "July",
price: "86"
},
{
date: "August",
price: "201"
},
{
date: "September",
price: "140"
}
]
}
];
const margin = 80;
const width = 1000 - 2 * margin;
const height = 550 - 2 * margin;
var duration = 250;
var lineOpacity = "0.25";
var lineOpacityHover = "0.85";
var otherLinesOpacityHover = "0.1";
var lineStroke = "1.5px";
var lineStrokeHover = "2.5px";
var circleOpacity = '0.85';
var circleOpacityOnLineHover = "0.25"
var circleRadius = 3;
var circleRadiusHover = 6;
/* Format Data */
var parseDate = d3.time.format("%B");
data.forEach(function(d) {
d.values.forEach(function(d) {
d.date = parseDate.parse(d.date);
d.price = +d.price;
});
});
/* Scale */
var xScale = d3.time.scale()
.domain(d3.extent(data[0].values, d => d.date))
.range([0, width - margin]);
var yScale = d3.scale.linear()
.domain([0, d3.max(data[0].values, d => d.price)])
.range([height - margin, 0]);
// var color = d3.scale.ordinal(d3.schemeCategory10);
var color = d3.scale.category10();
/* Add SVG */
var svg = d3.select("svg")
.attr("width", (width + margin) + "px")
.attr("height", (height + margin) + "px")
.append('g')
.attr("transform", `translate(${margin}, ${margin})`);
/* Add line into SVG */
var line = d3.svg.line()
.x(d => xScale(d.date))
.y(d => yScale(d.price));
let lines = svg.append('g')
.attr('class', 'lines');
lines.selectAll('.line-group')
.data(data).enter()
.append('g')
.attr('class', 'line-group')
.on("mouseover", function(d, i) {
svg.append("text")
.attr("class", "title-text")
.style("fill", color(i))
.text(d.name)
.attr("text-anchor", "middle")
.attr("x", (width - margin) / 2)
.attr("y", 5);
})
.on("mouseout", function(d) {
svg.select(".title-text").remove();
})
.append('path')
.attr('class', 'line')
.attr('d', d => line(d.values))
.style('stroke', (d, i) => color(i))
.style('opacity', lineOpacity)
.on("mouseover", function(d) {
d3.selectAll('.line')
.style('opacity', otherLinesOpacityHover);
d3.selectAll('.circle')
.style('opacity', circleOpacityOnLineHover);
d3.select(this)
.style('opacity', lineOpacityHover)
.style("stroke-width", lineStrokeHover)
.style("cursor", "pointer");
})
.on("mouseout", function(d) {
d3.selectAll(".line")
.style('opacity', lineOpacity);
d3.selectAll('.circle')
.style('opacity', circleOpacity);
d3.select(this)
.style("stroke-width", lineStroke)
.style("cursor", "none");
});
/* Add circles in the line */
lines.selectAll("circle-group")
.data(data).enter()
.append("g")
.style("fill", (d, i) => color(i))
.selectAll("circle")
.data(d => d.values).enter()
.append("g")
.attr("class", "circle")
.on("mouseover", function(d) {
d3.select(this)
.style("cursor", "pointer")
.append("text")
.attr("class", "text")
.text(`${d.price}`)
.attr("x", d => xScale(d.date) + 5)
.attr("y", d => yScale(d.price) - 10);
})
.on("mouseout", function(d) {
d3.select(this)
.style("cursor", "none")
.transition()
.duration(duration)
.selectAll(".text").remove();
})
.append("circle")
.attr("cx", d => xScale(d.date))
.attr("cy", d => yScale(d.price))
.attr("r", circleRadius)
.style('opacity', circleOpacity)
.on("mouseover", function(d) {
d3.select(this)
.transition()
.duration(duration)
.attr("r", circleRadiusHover);
})
.on("mouseout", function(d) {
d3.select(this)
.transition()
.duration(duration)
.attr("r", circleRadius);
});
var xAxis = d3.svg.axis().scale(xScale)
.orient("bottom").tickFormat(d3.time.format("%B")).tickSize(1);
var yAxis = d3.svg.axis().scale(yScale)
.orient("left").tickSize(1);
svg.append("g")
.attr("class", "x axis")
.attr("transform", `translate(0, ${height-margin})`)
.call(xAxis.ticks(d3.time.year));
svg.append("g")
.attr("class", "y axis")
.call(yAxis)
.append('text')
.attr("y", 15)
.attr("transform", "rotate(-90)")
.attr("fill", "#000")
.attr('text-anchor', 'middle')
.text("No. of Employees");
javascript d3.js linechart xscale
I have implemented a D3v3 Multi-line chart in my application. As I'm quite new to D3 charts I looked for some online resources and came up with this. Initially, I had to display Years on X-axis and it was working properly. This fiddle shows it (https://jsfiddle.net/yasirunilan/tz57wc29/13/). Then according to a new requirement I need to show the data distribution with months in X-Axis, The difference is the X-axis doesn't always start with the month of January. As given in the Dataset it might start from October of a year to October of a year to September of Next Year. In that case, I faced a problem in displaying the line and X-Axis values in the chart. Can someone provide direction for this problem?
Fiddle: https://jsfiddle.net/yasirunilan/v160sr4z/10/
var data = [{
name: "USA",
values: [{
date: "October",
price: "100"
},
{
date: "November",
price: "110"
},
{
date: "December",
price: "145"
},
{
date: "January",
price: "241"
},
{
date: "February",
price: "101"
},
{
date: "March",
price: "90"
},
{
date: "April",
price: "120"
},
{
date: "May",
price: "135"
},
{
date: "June",
price: "99"
},
{
date: "July",
price: "105"
},
{
date: "August",
price: "101"
},
{
date: "September",
price: "106"
}
]
},
{
name: "UK",
values: [{
date: "October",
price: "130"
},
{
date: "November",
price: "120"
},
{
date: "December",
price: "115"
},
{
date: "January",
price: "220"
},
{
date: "February",
price: "100"
},
{
date: "March",
price: "140"
},
{
date: "April",
price: "90"
},
{
date: "May",
price: "235"
},
{
date: "June",
price: "160"
},
{
date: "July",
price: "86"
},
{
date: "August",
price: "201"
},
{
date: "September",
price: "140"
}
]
}
];
const margin = 80;
const width = 1000 - 2 * margin;
const height = 550 - 2 * margin;
var duration = 250;
var lineOpacity = "0.25";
var lineOpacityHover = "0.85";
var otherLinesOpacityHover = "0.1";
var lineStroke = "1.5px";
var lineStrokeHover = "2.5px";
var circleOpacity = '0.85';
var circleOpacityOnLineHover = "0.25"
var circleRadius = 3;
var circleRadiusHover = 6;
/* Format Data */
var parseDate = d3.time.format("%B");
data.forEach(function(d) {
d.values.forEach(function(d) {
d.date = parseDate.parse(d.date);
d.price = +d.price;
});
});
/* Scale */
var xScale = d3.time.scale()
.domain(d3.extent(data[0].values, d => d.date))
.range([0, width - margin]);
var yScale = d3.scale.linear()
.domain([0, d3.max(data[0].values, d => d.price)])
.range([height - margin, 0]);
// var color = d3.scale.ordinal(d3.schemeCategory10);
var color = d3.scale.category10();
/* Add SVG */
var svg = d3.select("svg")
.attr("width", (width + margin) + "px")
.attr("height", (height + margin) + "px")
.append('g')
.attr("transform", `translate(${margin}, ${margin})`);
/* Add line into SVG */
var line = d3.svg.line()
.x(d => xScale(d.date))
.y(d => yScale(d.price));
let lines = svg.append('g')
.attr('class', 'lines');
lines.selectAll('.line-group')
.data(data).enter()
.append('g')
.attr('class', 'line-group')
.on("mouseover", function(d, i) {
svg.append("text")
.attr("class", "title-text")
.style("fill", color(i))
.text(d.name)
.attr("text-anchor", "middle")
.attr("x", (width - margin) / 2)
.attr("y", 5);
})
.on("mouseout", function(d) {
svg.select(".title-text").remove();
})
.append('path')
.attr('class', 'line')
.attr('d', d => line(d.values))
.style('stroke', (d, i) => color(i))
.style('opacity', lineOpacity)
.on("mouseover", function(d) {
d3.selectAll('.line')
.style('opacity', otherLinesOpacityHover);
d3.selectAll('.circle')
.style('opacity', circleOpacityOnLineHover);
d3.select(this)
.style('opacity', lineOpacityHover)
.style("stroke-width", lineStrokeHover)
.style("cursor", "pointer");
})
.on("mouseout", function(d) {
d3.selectAll(".line")
.style('opacity', lineOpacity);
d3.selectAll('.circle')
.style('opacity', circleOpacity);
d3.select(this)
.style("stroke-width", lineStroke)
.style("cursor", "none");
});
/* Add circles in the line */
lines.selectAll("circle-group")
.data(data).enter()
.append("g")
.style("fill", (d, i) => color(i))
.selectAll("circle")
.data(d => d.values).enter()
.append("g")
.attr("class", "circle")
.on("mouseover", function(d) {
d3.select(this)
.style("cursor", "pointer")
.append("text")
.attr("class", "text")
.text(`${d.price}`)
.attr("x", d => xScale(d.date) + 5)
.attr("y", d => yScale(d.price) - 10);
})
.on("mouseout", function(d) {
d3.select(this)
.style("cursor", "none")
.transition()
.duration(duration)
.selectAll(".text").remove();
})
.append("circle")
.attr("cx", d => xScale(d.date))
.attr("cy", d => yScale(d.price))
.attr("r", circleRadius)
.style('opacity', circleOpacity)
.on("mouseover", function(d) {
d3.select(this)
.transition()
.duration(duration)
.attr("r", circleRadiusHover);
})
.on("mouseout", function(d) {
d3.select(this)
.transition()
.duration(duration)
.attr("r", circleRadius);
});
var xAxis = d3.svg.axis().scale(xScale)
.orient("bottom").tickFormat(d3.time.format("%B")).tickSize(1);
var yAxis = d3.svg.axis().scale(yScale)
.orient("left").tickSize(1);
svg.append("g")
.attr("class", "x axis")
.attr("transform", `translate(0, ${height-margin})`)
.call(xAxis.ticks(d3.time.year));
svg.append("g")
.attr("class", "y axis")
.call(yAxis)
.append('text')
.attr("y", 15)
.attr("transform", "rotate(-90)")
.attr("fill", "#000")
.attr('text-anchor', 'middle')
.text("No. of Employees");
javascript d3.js linechart xscale
javascript d3.js linechart xscale
asked Nov 23 '18 at 11:55
Yasiru NilanYasiru Nilan
8810
8810
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
One possible solution is to add years to date
field like this
{
date: "2010-October",
price: "100"
}
If you are not going to display the year, it may be arbitrary, just make sure, that after December it increases by 1.
Then replace var parseDate = d3.time.format("%B");
with var parseDate = d3.time.format("%Y-%B");
Also replace .call(xAxis.ticks(d3.time.year));
with .call(xAxis.ticks(d3.time.months));
Here is the fiddle: https://jsfiddle.net/p68z0bqo/
Edit: If you can't add years manually, you can try to do this automatically like this:
var parseDate = d3.time.format("%Y-%B");
data.forEach(function(d) {
var year = 2000;
d.values.forEach(function(d) {
if (d.date === 'January') year++;
d.date = parseDate.parse(year.toString() + '-' + d.date);
d.price = +d.price;
});
});
This code only works if all months are present (there are no gaps) and if January is represented exactly as "January"
(i.e. it has the same case).
can't we use " date: "October" ", instead of "date: "2010-October" ",
– Yasiru Nilan
Nov 23 '18 at 12:53
Modified answer in response to your comment
– Yaroslav Sergienko
Nov 23 '18 at 13:07
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53446272%2fd3-multi-line-chart-not-displaying-properly-with-x-axis-value%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
One possible solution is to add years to date
field like this
{
date: "2010-October",
price: "100"
}
If you are not going to display the year, it may be arbitrary, just make sure, that after December it increases by 1.
Then replace var parseDate = d3.time.format("%B");
with var parseDate = d3.time.format("%Y-%B");
Also replace .call(xAxis.ticks(d3.time.year));
with .call(xAxis.ticks(d3.time.months));
Here is the fiddle: https://jsfiddle.net/p68z0bqo/
Edit: If you can't add years manually, you can try to do this automatically like this:
var parseDate = d3.time.format("%Y-%B");
data.forEach(function(d) {
var year = 2000;
d.values.forEach(function(d) {
if (d.date === 'January') year++;
d.date = parseDate.parse(year.toString() + '-' + d.date);
d.price = +d.price;
});
});
This code only works if all months are present (there are no gaps) and if January is represented exactly as "January"
(i.e. it has the same case).
can't we use " date: "October" ", instead of "date: "2010-October" ",
– Yasiru Nilan
Nov 23 '18 at 12:53
Modified answer in response to your comment
– Yaroslav Sergienko
Nov 23 '18 at 13:07
add a comment |
One possible solution is to add years to date
field like this
{
date: "2010-October",
price: "100"
}
If you are not going to display the year, it may be arbitrary, just make sure, that after December it increases by 1.
Then replace var parseDate = d3.time.format("%B");
with var parseDate = d3.time.format("%Y-%B");
Also replace .call(xAxis.ticks(d3.time.year));
with .call(xAxis.ticks(d3.time.months));
Here is the fiddle: https://jsfiddle.net/p68z0bqo/
Edit: If you can't add years manually, you can try to do this automatically like this:
var parseDate = d3.time.format("%Y-%B");
data.forEach(function(d) {
var year = 2000;
d.values.forEach(function(d) {
if (d.date === 'January') year++;
d.date = parseDate.parse(year.toString() + '-' + d.date);
d.price = +d.price;
});
});
This code only works if all months are present (there are no gaps) and if January is represented exactly as "January"
(i.e. it has the same case).
can't we use " date: "October" ", instead of "date: "2010-October" ",
– Yasiru Nilan
Nov 23 '18 at 12:53
Modified answer in response to your comment
– Yaroslav Sergienko
Nov 23 '18 at 13:07
add a comment |
One possible solution is to add years to date
field like this
{
date: "2010-October",
price: "100"
}
If you are not going to display the year, it may be arbitrary, just make sure, that after December it increases by 1.
Then replace var parseDate = d3.time.format("%B");
with var parseDate = d3.time.format("%Y-%B");
Also replace .call(xAxis.ticks(d3.time.year));
with .call(xAxis.ticks(d3.time.months));
Here is the fiddle: https://jsfiddle.net/p68z0bqo/
Edit: If you can't add years manually, you can try to do this automatically like this:
var parseDate = d3.time.format("%Y-%B");
data.forEach(function(d) {
var year = 2000;
d.values.forEach(function(d) {
if (d.date === 'January') year++;
d.date = parseDate.parse(year.toString() + '-' + d.date);
d.price = +d.price;
});
});
This code only works if all months are present (there are no gaps) and if January is represented exactly as "January"
(i.e. it has the same case).
One possible solution is to add years to date
field like this
{
date: "2010-October",
price: "100"
}
If you are not going to display the year, it may be arbitrary, just make sure, that after December it increases by 1.
Then replace var parseDate = d3.time.format("%B");
with var parseDate = d3.time.format("%Y-%B");
Also replace .call(xAxis.ticks(d3.time.year));
with .call(xAxis.ticks(d3.time.months));
Here is the fiddle: https://jsfiddle.net/p68z0bqo/
Edit: If you can't add years manually, you can try to do this automatically like this:
var parseDate = d3.time.format("%Y-%B");
data.forEach(function(d) {
var year = 2000;
d.values.forEach(function(d) {
if (d.date === 'January') year++;
d.date = parseDate.parse(year.toString() + '-' + d.date);
d.price = +d.price;
});
});
This code only works if all months are present (there are no gaps) and if January is represented exactly as "January"
(i.e. it has the same case).
edited Nov 23 '18 at 13:07
answered Nov 23 '18 at 12:26


Yaroslav SergienkoYaroslav Sergienko
40016
40016
can't we use " date: "October" ", instead of "date: "2010-October" ",
– Yasiru Nilan
Nov 23 '18 at 12:53
Modified answer in response to your comment
– Yaroslav Sergienko
Nov 23 '18 at 13:07
add a comment |
can't we use " date: "October" ", instead of "date: "2010-October" ",
– Yasiru Nilan
Nov 23 '18 at 12:53
Modified answer in response to your comment
– Yaroslav Sergienko
Nov 23 '18 at 13:07
can't we use " date: "October" ", instead of "date: "2010-October" ",
– Yasiru Nilan
Nov 23 '18 at 12:53
can't we use " date: "October" ", instead of "date: "2010-October" ",
– Yasiru Nilan
Nov 23 '18 at 12:53
Modified answer in response to your comment
– Yaroslav Sergienko
Nov 23 '18 at 13:07
Modified answer in response to your comment
– Yaroslav Sergienko
Nov 23 '18 at 13:07
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53446272%2fd3-multi-line-chart-not-displaying-properly-with-x-axis-value%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
FBP7UJ8BP6,sVsJ DvJ99euQzX,iG5xMUq3ZXGTGIJWB