Parsing PDF file using Apache PDFBox to get outlines
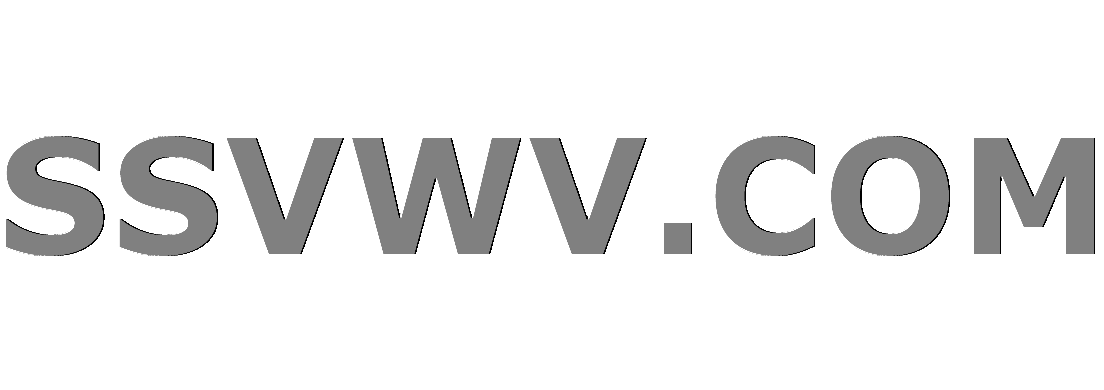
Multi tool use
up vote
0
down vote
favorite
Now I can use the PDFBox to extract the outlines from PDF, but some PDF can get the outlines, others can't.
Every PDF has outlines and when I open a pdf use pdf read tool, I can click an outline to a certain page.
Here is my code:
public static void main(String args) {
try {
PDDocument document = PDDocument.load(new File(filePath));
PDDocumentOutline outline = document.getDocumentCatalog().getDocumentOutline();
getOutlines(document, outline, "");
document.close();
} catch (InvalidPasswordException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
public static void getOutlines(PDDocument document, PDOutlineNode bookmark, String indentation) throws IOException{
PDOutlineItem current = bookmark.getFirstChild();
while (current != null) {
PDPage currentPage = current.findDestinationPage(document);
Integer pageNumber = document.getDocumentCatalog().getPages().indexOf(currentPage) + 1;
System.out.println(current.getTitle() + "-------->" + pageNumber);
getOutlines(document, current, indentation);
current = current.getNextSibling();
}
}
pdf pdfbox
|
show 7 more comments
up vote
0
down vote
favorite
Now I can use the PDFBox to extract the outlines from PDF, but some PDF can get the outlines, others can't.
Every PDF has outlines and when I open a pdf use pdf read tool, I can click an outline to a certain page.
Here is my code:
public static void main(String args) {
try {
PDDocument document = PDDocument.load(new File(filePath));
PDDocumentOutline outline = document.getDocumentCatalog().getDocumentOutline();
getOutlines(document, outline, "");
document.close();
} catch (InvalidPasswordException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
public static void getOutlines(PDDocument document, PDOutlineNode bookmark, String indentation) throws IOException{
PDOutlineItem current = bookmark.getFirstChild();
while (current != null) {
PDPage currentPage = current.findDestinationPage(document);
Integer pageNumber = document.getDocumentCatalog().getPages().indexOf(currentPage) + 1;
System.out.println(current.getTitle() + "-------->" + pageNumber);
getOutlines(document, current, indentation);
current = current.getNextSibling();
}
}
pdf pdfbox
1
If I understand you correctly, your code works for some files and not for others. Thus, it would be helpful if you shared an example file for which it does not work.
– mkl
Nov 22 at 7:08
1
"Every PDF has outlines" - no.
– Tilman Hausherr
Nov 22 at 7:22
@mkl the PDF have outlines but can parse it and I want to know the structure of a PDF file from this link
– th000
Nov 22 at 7:27
@TilmanHausherr I means the PDFs I want to parse have outlines.
– th000
Nov 22 at 7:28
Your link requires individual permission.
– Tilman Hausherr
Nov 22 at 7:32
|
show 7 more comments
up vote
0
down vote
favorite
up vote
0
down vote
favorite
Now I can use the PDFBox to extract the outlines from PDF, but some PDF can get the outlines, others can't.
Every PDF has outlines and when I open a pdf use pdf read tool, I can click an outline to a certain page.
Here is my code:
public static void main(String args) {
try {
PDDocument document = PDDocument.load(new File(filePath));
PDDocumentOutline outline = document.getDocumentCatalog().getDocumentOutline();
getOutlines(document, outline, "");
document.close();
} catch (InvalidPasswordException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
public static void getOutlines(PDDocument document, PDOutlineNode bookmark, String indentation) throws IOException{
PDOutlineItem current = bookmark.getFirstChild();
while (current != null) {
PDPage currentPage = current.findDestinationPage(document);
Integer pageNumber = document.getDocumentCatalog().getPages().indexOf(currentPage) + 1;
System.out.println(current.getTitle() + "-------->" + pageNumber);
getOutlines(document, current, indentation);
current = current.getNextSibling();
}
}
pdf pdfbox
Now I can use the PDFBox to extract the outlines from PDF, but some PDF can get the outlines, others can't.
Every PDF has outlines and when I open a pdf use pdf read tool, I can click an outline to a certain page.
Here is my code:
public static void main(String args) {
try {
PDDocument document = PDDocument.load(new File(filePath));
PDDocumentOutline outline = document.getDocumentCatalog().getDocumentOutline();
getOutlines(document, outline, "");
document.close();
} catch (InvalidPasswordException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
public static void getOutlines(PDDocument document, PDOutlineNode bookmark, String indentation) throws IOException{
PDOutlineItem current = bookmark.getFirstChild();
while (current != null) {
PDPage currentPage = current.findDestinationPage(document);
Integer pageNumber = document.getDocumentCatalog().getPages().indexOf(currentPage) + 1;
System.out.println(current.getTitle() + "-------->" + pageNumber);
getOutlines(document, current, indentation);
current = current.getNextSibling();
}
}
pdf pdfbox
pdf pdfbox
edited Nov 22 at 3:36
asked Nov 22 at 3:25
th000
336
336
1
If I understand you correctly, your code works for some files and not for others. Thus, it would be helpful if you shared an example file for which it does not work.
– mkl
Nov 22 at 7:08
1
"Every PDF has outlines" - no.
– Tilman Hausherr
Nov 22 at 7:22
@mkl the PDF have outlines but can parse it and I want to know the structure of a PDF file from this link
– th000
Nov 22 at 7:27
@TilmanHausherr I means the PDFs I want to parse have outlines.
– th000
Nov 22 at 7:28
Your link requires individual permission.
– Tilman Hausherr
Nov 22 at 7:32
|
show 7 more comments
1
If I understand you correctly, your code works for some files and not for others. Thus, it would be helpful if you shared an example file for which it does not work.
– mkl
Nov 22 at 7:08
1
"Every PDF has outlines" - no.
– Tilman Hausherr
Nov 22 at 7:22
@mkl the PDF have outlines but can parse it and I want to know the structure of a PDF file from this link
– th000
Nov 22 at 7:27
@TilmanHausherr I means the PDFs I want to parse have outlines.
– th000
Nov 22 at 7:28
Your link requires individual permission.
– Tilman Hausherr
Nov 22 at 7:32
1
1
If I understand you correctly, your code works for some files and not for others. Thus, it would be helpful if you shared an example file for which it does not work.
– mkl
Nov 22 at 7:08
If I understand you correctly, your code works for some files and not for others. Thus, it would be helpful if you shared an example file for which it does not work.
– mkl
Nov 22 at 7:08
1
1
"Every PDF has outlines" - no.
– Tilman Hausherr
Nov 22 at 7:22
"Every PDF has outlines" - no.
– Tilman Hausherr
Nov 22 at 7:22
@mkl the PDF have outlines but can parse it and I want to know the structure of a PDF file from this link
– th000
Nov 22 at 7:27
@mkl the PDF have outlines but can parse it and I want to know the structure of a PDF file from this link
– th000
Nov 22 at 7:27
@TilmanHausherr I means the PDFs I want to parse have outlines.
– th000
Nov 22 at 7:28
@TilmanHausherr I means the PDFs I want to parse have outlines.
– th000
Nov 22 at 7:28
Your link requires individual permission.
– Tilman Hausherr
Nov 22 at 7:32
Your link requires individual permission.
– Tilman Hausherr
Nov 22 at 7:32
|
show 7 more comments
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53423430%2fparsing-pdf-file-using-apache-pdfbox-to-get-outlines%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
HKPeE 1rTu1UM,j j6KFVf7IEy,Jc8YXUHHbweEE0E73YC,Hq3,iiwoDZpF6a7lFIbj3umanKsC43jmtI
1
If I understand you correctly, your code works for some files and not for others. Thus, it would be helpful if you shared an example file for which it does not work.
– mkl
Nov 22 at 7:08
1
"Every PDF has outlines" - no.
– Tilman Hausherr
Nov 22 at 7:22
@mkl the PDF have outlines but can parse it and I want to know the structure of a PDF file from this link
– th000
Nov 22 at 7:27
@TilmanHausherr I means the PDFs I want to parse have outlines.
– th000
Nov 22 at 7:28
Your link requires individual permission.
– Tilman Hausherr
Nov 22 at 7:32