Problem in understanding three.js coordinate and axes system
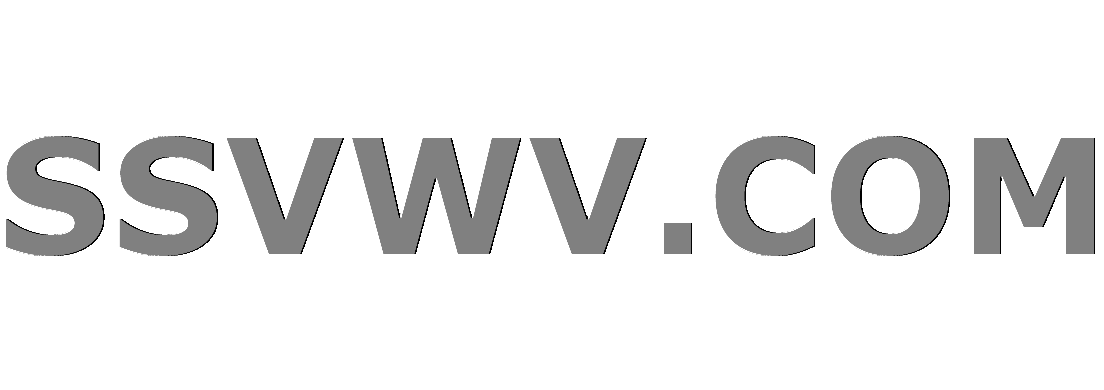
Multi tool use
I have following code:
// coordinate values
var x1 = -815723.5125568421;
var y1 = 20538442.534868136;
var z1 = -17.439584224846456;
var x2 = -815723.5125568421;
var y2 = 20538443.164575472;
var z2 = -16.620415776398275;
// make a rectangular face parallel to y-z plane
var dummySquare = new THREE.Geometry();
dummySquare.vertices.push(new THREE.Vector3(x1,y1,z1));
dummySquare.vertices.push(new THREE.Vector3(x1,y1,z2));
dummySquare.vertices.push(new THREE.Vector3(x2,y2,z1));
dummySquare.vertices.push(new THREE.Vector3(x2,y2,z2));
dummySquare.faces.push(new THREE.Face3(0,1,2));
dummySquare.faces.push(new THREE.Face3(1,2,3));
var dummySquareMaterial = new THREE.MeshBasicMaterial( { color: "#0000FF", side: THREE.DoubleSide } );
var dummySquareMesh = new THREE.Mesh(dummySquare, dummySquareMaterial);
So, I am making a rectangular face parallel to y-z plane.
During debugging I observe following:
vertices: Array(4)
0: p {x: -815723.5125568421, y: 20538442.534868136, z:
-17.439584224846456}
1: p {x: -815723.5125568421, y: 20538442.534868136, z:
-16.620415776398275}
2: p {x: -815723.5125568421, y: 20538443.164575472, z:
-17.439584224846456}
3: p {x: -815723.5125568421, y: 20538443.164575472, z:
-16.620415776398275}
position: p {x: 0, y: 0, z: 0}
So vertices are as expected. But position is at (0,0,0). I expected position to be mid point of the plane defined by above four vertices.
What is missing here in my understanding?
Another observation is as follows.
I make two faces just like above(same vertices).
For one of the two faces, I determine centre of geometry, move geometry it to origin(translate by negative of centre), create a mesh with it which then I move back to original position:
var face = new THREE.Geometry();
....add vertices as code snippet above
var faceCentre = new THREE.Vector3();
face.boundingBox.getCenter(faceCentre );
face.translate(-faceCentre .x,-faceCentre .y,-faceCentre .z);
//make mesh
var faceMaterial = new THREE.MeshBasicMaterial( { color: "#FF0000", side:
THREE.DoubleSide } );
var faceMesh= new THREE.Mesh(face, faceMaterial);
// move mesh back by setting its position to original centre of face
faceMesh.position.x = faceCentre .x;
faceMesh.position.y = faceCentre .y;
faceMesh.position.z = faceCentre .z;
Unmoved face has same vertices as for face above, as expected.
But other face has now totally different vertices, even though both are displayed at same position and in same orientation.
Why this difference in vertices?
three.js
add a comment |
I have following code:
// coordinate values
var x1 = -815723.5125568421;
var y1 = 20538442.534868136;
var z1 = -17.439584224846456;
var x2 = -815723.5125568421;
var y2 = 20538443.164575472;
var z2 = -16.620415776398275;
// make a rectangular face parallel to y-z plane
var dummySquare = new THREE.Geometry();
dummySquare.vertices.push(new THREE.Vector3(x1,y1,z1));
dummySquare.vertices.push(new THREE.Vector3(x1,y1,z2));
dummySquare.vertices.push(new THREE.Vector3(x2,y2,z1));
dummySquare.vertices.push(new THREE.Vector3(x2,y2,z2));
dummySquare.faces.push(new THREE.Face3(0,1,2));
dummySquare.faces.push(new THREE.Face3(1,2,3));
var dummySquareMaterial = new THREE.MeshBasicMaterial( { color: "#0000FF", side: THREE.DoubleSide } );
var dummySquareMesh = new THREE.Mesh(dummySquare, dummySquareMaterial);
So, I am making a rectangular face parallel to y-z plane.
During debugging I observe following:
vertices: Array(4)
0: p {x: -815723.5125568421, y: 20538442.534868136, z:
-17.439584224846456}
1: p {x: -815723.5125568421, y: 20538442.534868136, z:
-16.620415776398275}
2: p {x: -815723.5125568421, y: 20538443.164575472, z:
-17.439584224846456}
3: p {x: -815723.5125568421, y: 20538443.164575472, z:
-16.620415776398275}
position: p {x: 0, y: 0, z: 0}
So vertices are as expected. But position is at (0,0,0). I expected position to be mid point of the plane defined by above four vertices.
What is missing here in my understanding?
Another observation is as follows.
I make two faces just like above(same vertices).
For one of the two faces, I determine centre of geometry, move geometry it to origin(translate by negative of centre), create a mesh with it which then I move back to original position:
var face = new THREE.Geometry();
....add vertices as code snippet above
var faceCentre = new THREE.Vector3();
face.boundingBox.getCenter(faceCentre );
face.translate(-faceCentre .x,-faceCentre .y,-faceCentre .z);
//make mesh
var faceMaterial = new THREE.MeshBasicMaterial( { color: "#FF0000", side:
THREE.DoubleSide } );
var faceMesh= new THREE.Mesh(face, faceMaterial);
// move mesh back by setting its position to original centre of face
faceMesh.position.x = faceCentre .x;
faceMesh.position.y = faceCentre .y;
faceMesh.position.z = faceCentre .z;
Unmoved face has same vertices as for face above, as expected.
But other face has now totally different vertices, even though both are displayed at same position and in same orientation.
Why this difference in vertices?
three.js
add a comment |
I have following code:
// coordinate values
var x1 = -815723.5125568421;
var y1 = 20538442.534868136;
var z1 = -17.439584224846456;
var x2 = -815723.5125568421;
var y2 = 20538443.164575472;
var z2 = -16.620415776398275;
// make a rectangular face parallel to y-z plane
var dummySquare = new THREE.Geometry();
dummySquare.vertices.push(new THREE.Vector3(x1,y1,z1));
dummySquare.vertices.push(new THREE.Vector3(x1,y1,z2));
dummySquare.vertices.push(new THREE.Vector3(x2,y2,z1));
dummySquare.vertices.push(new THREE.Vector3(x2,y2,z2));
dummySquare.faces.push(new THREE.Face3(0,1,2));
dummySquare.faces.push(new THREE.Face3(1,2,3));
var dummySquareMaterial = new THREE.MeshBasicMaterial( { color: "#0000FF", side: THREE.DoubleSide } );
var dummySquareMesh = new THREE.Mesh(dummySquare, dummySquareMaterial);
So, I am making a rectangular face parallel to y-z plane.
During debugging I observe following:
vertices: Array(4)
0: p {x: -815723.5125568421, y: 20538442.534868136, z:
-17.439584224846456}
1: p {x: -815723.5125568421, y: 20538442.534868136, z:
-16.620415776398275}
2: p {x: -815723.5125568421, y: 20538443.164575472, z:
-17.439584224846456}
3: p {x: -815723.5125568421, y: 20538443.164575472, z:
-16.620415776398275}
position: p {x: 0, y: 0, z: 0}
So vertices are as expected. But position is at (0,0,0). I expected position to be mid point of the plane defined by above four vertices.
What is missing here in my understanding?
Another observation is as follows.
I make two faces just like above(same vertices).
For one of the two faces, I determine centre of geometry, move geometry it to origin(translate by negative of centre), create a mesh with it which then I move back to original position:
var face = new THREE.Geometry();
....add vertices as code snippet above
var faceCentre = new THREE.Vector3();
face.boundingBox.getCenter(faceCentre );
face.translate(-faceCentre .x,-faceCentre .y,-faceCentre .z);
//make mesh
var faceMaterial = new THREE.MeshBasicMaterial( { color: "#FF0000", side:
THREE.DoubleSide } );
var faceMesh= new THREE.Mesh(face, faceMaterial);
// move mesh back by setting its position to original centre of face
faceMesh.position.x = faceCentre .x;
faceMesh.position.y = faceCentre .y;
faceMesh.position.z = faceCentre .z;
Unmoved face has same vertices as for face above, as expected.
But other face has now totally different vertices, even though both are displayed at same position and in same orientation.
Why this difference in vertices?
three.js
I have following code:
// coordinate values
var x1 = -815723.5125568421;
var y1 = 20538442.534868136;
var z1 = -17.439584224846456;
var x2 = -815723.5125568421;
var y2 = 20538443.164575472;
var z2 = -16.620415776398275;
// make a rectangular face parallel to y-z plane
var dummySquare = new THREE.Geometry();
dummySquare.vertices.push(new THREE.Vector3(x1,y1,z1));
dummySquare.vertices.push(new THREE.Vector3(x1,y1,z2));
dummySquare.vertices.push(new THREE.Vector3(x2,y2,z1));
dummySquare.vertices.push(new THREE.Vector3(x2,y2,z2));
dummySquare.faces.push(new THREE.Face3(0,1,2));
dummySquare.faces.push(new THREE.Face3(1,2,3));
var dummySquareMaterial = new THREE.MeshBasicMaterial( { color: "#0000FF", side: THREE.DoubleSide } );
var dummySquareMesh = new THREE.Mesh(dummySquare, dummySquareMaterial);
So, I am making a rectangular face parallel to y-z plane.
During debugging I observe following:
vertices: Array(4)
0: p {x: -815723.5125568421, y: 20538442.534868136, z:
-17.439584224846456}
1: p {x: -815723.5125568421, y: 20538442.534868136, z:
-16.620415776398275}
2: p {x: -815723.5125568421, y: 20538443.164575472, z:
-17.439584224846456}
3: p {x: -815723.5125568421, y: 20538443.164575472, z:
-16.620415776398275}
position: p {x: 0, y: 0, z: 0}
So vertices are as expected. But position is at (0,0,0). I expected position to be mid point of the plane defined by above four vertices.
What is missing here in my understanding?
Another observation is as follows.
I make two faces just like above(same vertices).
For one of the two faces, I determine centre of geometry, move geometry it to origin(translate by negative of centre), create a mesh with it which then I move back to original position:
var face = new THREE.Geometry();
....add vertices as code snippet above
var faceCentre = new THREE.Vector3();
face.boundingBox.getCenter(faceCentre );
face.translate(-faceCentre .x,-faceCentre .y,-faceCentre .z);
//make mesh
var faceMaterial = new THREE.MeshBasicMaterial( { color: "#FF0000", side:
THREE.DoubleSide } );
var faceMesh= new THREE.Mesh(face, faceMaterial);
// move mesh back by setting its position to original centre of face
faceMesh.position.x = faceCentre .x;
faceMesh.position.y = faceCentre .y;
faceMesh.position.z = faceCentre .z;
Unmoved face has same vertices as for face above, as expected.
But other face has now totally different vertices, even though both are displayed at same position and in same orientation.
Why this difference in vertices?
three.js
three.js
asked Nov 22 at 17:30
Mapper
915
915
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
THREE.js uses a hierarchical representation of objects and their translation. In particular, the .position
of an Object3D
is not generally, as you say you expected, its middle point in world space, but it can be viewed as a variable that stores the current translation of the object. It is called its local position. This translation is (0,0,0)
on default.
So when you define an object by the vertices of its geometry, the object's vertices will render at these positions. However, if you .translate()
it by a factor (dx, dy, dz)
, then a vertex (vx, vy, vz)
will render at position (vx+dx, vy+dy, vz+dz)
.
Similarly, other transformations are also stored as members of the object. The vertices of the geometry do not change when an object is transformed, but instead the object keeps track of its current local transformations, which are applied, typically as a series of matrix multiplications, to the vertices. Using this logic, you can define a tree of objects inside each other, which have local transformations in relation to its parent, which in turn may be transformed in relation to its parent etc. This sort of representation proves very useful for slightly more complicated scenes.
This should explain your results. For example, in your first test, you are successfully creating an object exactly where you want it, but its position
is still (0,0,0) because it has undergone no transformations.
Which coordinate system are vertices expressed? I made a cube as:
– Mapper
Nov 23 at 19:27
When you define a geometry, its vertices are expressed in a local coordinate system. When you place an object directly in your scene, at position p, then a vertex v in your object will be rendered on position p+v. I.e., the local coordinate system of your object is translated by p. In particular, the mesh does not change, only the transformations that the object keeps track of do
– Berthur
Nov 23 at 22:04
Thanks for this reply. So it means while vertices are expressed in object's local coordinate system(v), while object's position(p) is expressed in global coordinate system. So in global coordinate system, a vertice becomes p+v(basic vector algebra rule). Thanks a lot.
– Mapper
Nov 24 at 3:12
I have doubt though. When I do geometry.translate(), why doesn't it change object.position? Shouldn't an object's position be determined by its vertices value?
– Mapper
Nov 24 at 3:19
Ah, I think the main source for confusion here is this: A geometry in THREE.js does not represent an object in the scene. It represents a shape of some sort. You can create a geometry and then assign that same geometry to several objects, all placed in the scene under different translations (or other transformations, e.g. rotation or scale). The underlying geometry will be the same, but the different "copies" may have undergone different transformations.
– Berthur
Nov 24 at 4:30
|
show 3 more comments
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53435879%2fproblem-in-understanding-three-js-coordinate-and-axes-system%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
THREE.js uses a hierarchical representation of objects and their translation. In particular, the .position
of an Object3D
is not generally, as you say you expected, its middle point in world space, but it can be viewed as a variable that stores the current translation of the object. It is called its local position. This translation is (0,0,0)
on default.
So when you define an object by the vertices of its geometry, the object's vertices will render at these positions. However, if you .translate()
it by a factor (dx, dy, dz)
, then a vertex (vx, vy, vz)
will render at position (vx+dx, vy+dy, vz+dz)
.
Similarly, other transformations are also stored as members of the object. The vertices of the geometry do not change when an object is transformed, but instead the object keeps track of its current local transformations, which are applied, typically as a series of matrix multiplications, to the vertices. Using this logic, you can define a tree of objects inside each other, which have local transformations in relation to its parent, which in turn may be transformed in relation to its parent etc. This sort of representation proves very useful for slightly more complicated scenes.
This should explain your results. For example, in your first test, you are successfully creating an object exactly where you want it, but its position
is still (0,0,0) because it has undergone no transformations.
Which coordinate system are vertices expressed? I made a cube as:
– Mapper
Nov 23 at 19:27
When you define a geometry, its vertices are expressed in a local coordinate system. When you place an object directly in your scene, at position p, then a vertex v in your object will be rendered on position p+v. I.e., the local coordinate system of your object is translated by p. In particular, the mesh does not change, only the transformations that the object keeps track of do
– Berthur
Nov 23 at 22:04
Thanks for this reply. So it means while vertices are expressed in object's local coordinate system(v), while object's position(p) is expressed in global coordinate system. So in global coordinate system, a vertice becomes p+v(basic vector algebra rule). Thanks a lot.
– Mapper
Nov 24 at 3:12
I have doubt though. When I do geometry.translate(), why doesn't it change object.position? Shouldn't an object's position be determined by its vertices value?
– Mapper
Nov 24 at 3:19
Ah, I think the main source for confusion here is this: A geometry in THREE.js does not represent an object in the scene. It represents a shape of some sort. You can create a geometry and then assign that same geometry to several objects, all placed in the scene under different translations (or other transformations, e.g. rotation or scale). The underlying geometry will be the same, but the different "copies" may have undergone different transformations.
– Berthur
Nov 24 at 4:30
|
show 3 more comments
THREE.js uses a hierarchical representation of objects and their translation. In particular, the .position
of an Object3D
is not generally, as you say you expected, its middle point in world space, but it can be viewed as a variable that stores the current translation of the object. It is called its local position. This translation is (0,0,0)
on default.
So when you define an object by the vertices of its geometry, the object's vertices will render at these positions. However, if you .translate()
it by a factor (dx, dy, dz)
, then a vertex (vx, vy, vz)
will render at position (vx+dx, vy+dy, vz+dz)
.
Similarly, other transformations are also stored as members of the object. The vertices of the geometry do not change when an object is transformed, but instead the object keeps track of its current local transformations, which are applied, typically as a series of matrix multiplications, to the vertices. Using this logic, you can define a tree of objects inside each other, which have local transformations in relation to its parent, which in turn may be transformed in relation to its parent etc. This sort of representation proves very useful for slightly more complicated scenes.
This should explain your results. For example, in your first test, you are successfully creating an object exactly where you want it, but its position
is still (0,0,0) because it has undergone no transformations.
Which coordinate system are vertices expressed? I made a cube as:
– Mapper
Nov 23 at 19:27
When you define a geometry, its vertices are expressed in a local coordinate system. When you place an object directly in your scene, at position p, then a vertex v in your object will be rendered on position p+v. I.e., the local coordinate system of your object is translated by p. In particular, the mesh does not change, only the transformations that the object keeps track of do
– Berthur
Nov 23 at 22:04
Thanks for this reply. So it means while vertices are expressed in object's local coordinate system(v), while object's position(p) is expressed in global coordinate system. So in global coordinate system, a vertice becomes p+v(basic vector algebra rule). Thanks a lot.
– Mapper
Nov 24 at 3:12
I have doubt though. When I do geometry.translate(), why doesn't it change object.position? Shouldn't an object's position be determined by its vertices value?
– Mapper
Nov 24 at 3:19
Ah, I think the main source for confusion here is this: A geometry in THREE.js does not represent an object in the scene. It represents a shape of some sort. You can create a geometry and then assign that same geometry to several objects, all placed in the scene under different translations (or other transformations, e.g. rotation or scale). The underlying geometry will be the same, but the different "copies" may have undergone different transformations.
– Berthur
Nov 24 at 4:30
|
show 3 more comments
THREE.js uses a hierarchical representation of objects and their translation. In particular, the .position
of an Object3D
is not generally, as you say you expected, its middle point in world space, but it can be viewed as a variable that stores the current translation of the object. It is called its local position. This translation is (0,0,0)
on default.
So when you define an object by the vertices of its geometry, the object's vertices will render at these positions. However, if you .translate()
it by a factor (dx, dy, dz)
, then a vertex (vx, vy, vz)
will render at position (vx+dx, vy+dy, vz+dz)
.
Similarly, other transformations are also stored as members of the object. The vertices of the geometry do not change when an object is transformed, but instead the object keeps track of its current local transformations, which are applied, typically as a series of matrix multiplications, to the vertices. Using this logic, you can define a tree of objects inside each other, which have local transformations in relation to its parent, which in turn may be transformed in relation to its parent etc. This sort of representation proves very useful for slightly more complicated scenes.
This should explain your results. For example, in your first test, you are successfully creating an object exactly where you want it, but its position
is still (0,0,0) because it has undergone no transformations.
THREE.js uses a hierarchical representation of objects and their translation. In particular, the .position
of an Object3D
is not generally, as you say you expected, its middle point in world space, but it can be viewed as a variable that stores the current translation of the object. It is called its local position. This translation is (0,0,0)
on default.
So when you define an object by the vertices of its geometry, the object's vertices will render at these positions. However, if you .translate()
it by a factor (dx, dy, dz)
, then a vertex (vx, vy, vz)
will render at position (vx+dx, vy+dy, vz+dz)
.
Similarly, other transformations are also stored as members of the object. The vertices of the geometry do not change when an object is transformed, but instead the object keeps track of its current local transformations, which are applied, typically as a series of matrix multiplications, to the vertices. Using this logic, you can define a tree of objects inside each other, which have local transformations in relation to its parent, which in turn may be transformed in relation to its parent etc. This sort of representation proves very useful for slightly more complicated scenes.
This should explain your results. For example, in your first test, you are successfully creating an object exactly where you want it, but its position
is still (0,0,0) because it has undergone no transformations.
answered Nov 22 at 18:55


Berthur
699211
699211
Which coordinate system are vertices expressed? I made a cube as:
– Mapper
Nov 23 at 19:27
When you define a geometry, its vertices are expressed in a local coordinate system. When you place an object directly in your scene, at position p, then a vertex v in your object will be rendered on position p+v. I.e., the local coordinate system of your object is translated by p. In particular, the mesh does not change, only the transformations that the object keeps track of do
– Berthur
Nov 23 at 22:04
Thanks for this reply. So it means while vertices are expressed in object's local coordinate system(v), while object's position(p) is expressed in global coordinate system. So in global coordinate system, a vertice becomes p+v(basic vector algebra rule). Thanks a lot.
– Mapper
Nov 24 at 3:12
I have doubt though. When I do geometry.translate(), why doesn't it change object.position? Shouldn't an object's position be determined by its vertices value?
– Mapper
Nov 24 at 3:19
Ah, I think the main source for confusion here is this: A geometry in THREE.js does not represent an object in the scene. It represents a shape of some sort. You can create a geometry and then assign that same geometry to several objects, all placed in the scene under different translations (or other transformations, e.g. rotation or scale). The underlying geometry will be the same, but the different "copies" may have undergone different transformations.
– Berthur
Nov 24 at 4:30
|
show 3 more comments
Which coordinate system are vertices expressed? I made a cube as:
– Mapper
Nov 23 at 19:27
When you define a geometry, its vertices are expressed in a local coordinate system. When you place an object directly in your scene, at position p, then a vertex v in your object will be rendered on position p+v. I.e., the local coordinate system of your object is translated by p. In particular, the mesh does not change, only the transformations that the object keeps track of do
– Berthur
Nov 23 at 22:04
Thanks for this reply. So it means while vertices are expressed in object's local coordinate system(v), while object's position(p) is expressed in global coordinate system. So in global coordinate system, a vertice becomes p+v(basic vector algebra rule). Thanks a lot.
– Mapper
Nov 24 at 3:12
I have doubt though. When I do geometry.translate(), why doesn't it change object.position? Shouldn't an object's position be determined by its vertices value?
– Mapper
Nov 24 at 3:19
Ah, I think the main source for confusion here is this: A geometry in THREE.js does not represent an object in the scene. It represents a shape of some sort. You can create a geometry and then assign that same geometry to several objects, all placed in the scene under different translations (or other transformations, e.g. rotation or scale). The underlying geometry will be the same, but the different "copies" may have undergone different transformations.
– Berthur
Nov 24 at 4:30
Which coordinate system are vertices expressed? I made a cube as:
– Mapper
Nov 23 at 19:27
Which coordinate system are vertices expressed? I made a cube as:
– Mapper
Nov 23 at 19:27
When you define a geometry, its vertices are expressed in a local coordinate system. When you place an object directly in your scene, at position p, then a vertex v in your object will be rendered on position p+v. I.e., the local coordinate system of your object is translated by p. In particular, the mesh does not change, only the transformations that the object keeps track of do
– Berthur
Nov 23 at 22:04
When you define a geometry, its vertices are expressed in a local coordinate system. When you place an object directly in your scene, at position p, then a vertex v in your object will be rendered on position p+v. I.e., the local coordinate system of your object is translated by p. In particular, the mesh does not change, only the transformations that the object keeps track of do
– Berthur
Nov 23 at 22:04
Thanks for this reply. So it means while vertices are expressed in object's local coordinate system(v), while object's position(p) is expressed in global coordinate system. So in global coordinate system, a vertice becomes p+v(basic vector algebra rule). Thanks a lot.
– Mapper
Nov 24 at 3:12
Thanks for this reply. So it means while vertices are expressed in object's local coordinate system(v), while object's position(p) is expressed in global coordinate system. So in global coordinate system, a vertice becomes p+v(basic vector algebra rule). Thanks a lot.
– Mapper
Nov 24 at 3:12
I have doubt though. When I do geometry.translate(), why doesn't it change object.position? Shouldn't an object's position be determined by its vertices value?
– Mapper
Nov 24 at 3:19
I have doubt though. When I do geometry.translate(), why doesn't it change object.position? Shouldn't an object's position be determined by its vertices value?
– Mapper
Nov 24 at 3:19
Ah, I think the main source for confusion here is this: A geometry in THREE.js does not represent an object in the scene. It represents a shape of some sort. You can create a geometry and then assign that same geometry to several objects, all placed in the scene under different translations (or other transformations, e.g. rotation or scale). The underlying geometry will be the same, but the different "copies" may have undergone different transformations.
– Berthur
Nov 24 at 4:30
Ah, I think the main source for confusion here is this: A geometry in THREE.js does not represent an object in the scene. It represents a shape of some sort. You can create a geometry and then assign that same geometry to several objects, all placed in the scene under different translations (or other transformations, e.g. rotation or scale). The underlying geometry will be the same, but the different "copies" may have undergone different transformations.
– Berthur
Nov 24 at 4:30
|
show 3 more comments
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53435879%2fproblem-in-understanding-three-js-coordinate-and-axes-system%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
CNOF c0WGR F vmou9kbtYJoksmvMVmj7S1APXFiE2tb7KXgdrg4UHC,8Bd AtuG,16E1FgzZT7e,GJGz