jQuery: How to add div with a dynamic id that increments when button is clicked?
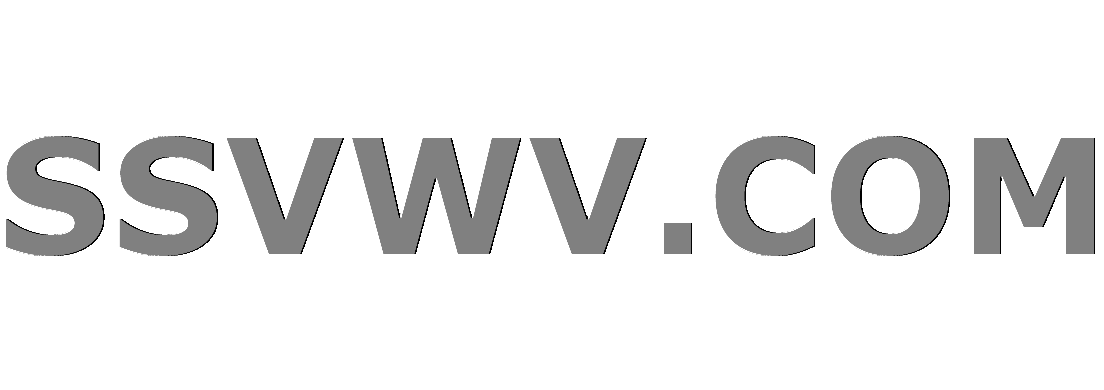
Multi tool use
I have this problem in jQuery:
I have a button that when clicked calls a function to create a div with an incremental id, equal to the number of clicks made. Here's my simplified solution:
$(document).ready(function() {
var count = 0;
});
$("#button").click(function() {
count = count + 1;
addDiv(count);
});
But, if I define the function click
here, the id on my div is NaN
while if I put the function of click
in document.ready()
, it creates two divs. I only want to create a div with incremental Id for each click!
javascript jquery html
add a comment |
I have this problem in jQuery:
I have a button that when clicked calls a function to create a div with an incremental id, equal to the number of clicks made. Here's my simplified solution:
$(document).ready(function() {
var count = 0;
});
$("#button").click(function() {
count = count + 1;
addDiv(count);
});
But, if I define the function click
here, the id on my div is NaN
while if I put the function of click
in document.ready()
, it creates two divs. I only want to create a div with incremental Id for each click!
javascript jquery html
Have a look at whatvar
does and how variables defined withvar
work: developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/…
– Andreas
Nov 22 at 18:45
I think the problem is that you have to change the elements attribute and not just a javascript variable
– tobifasc
Nov 22 at 18:53
The problem is in variable scope check jsfiddle.net/f9ug1dyv
– Mohammad
Nov 22 at 18:57
add a comment |
I have this problem in jQuery:
I have a button that when clicked calls a function to create a div with an incremental id, equal to the number of clicks made. Here's my simplified solution:
$(document).ready(function() {
var count = 0;
});
$("#button").click(function() {
count = count + 1;
addDiv(count);
});
But, if I define the function click
here, the id on my div is NaN
while if I put the function of click
in document.ready()
, it creates two divs. I only want to create a div with incremental Id for each click!
javascript jquery html
I have this problem in jQuery:
I have a button that when clicked calls a function to create a div with an incremental id, equal to the number of clicks made. Here's my simplified solution:
$(document).ready(function() {
var count = 0;
});
$("#button").click(function() {
count = count + 1;
addDiv(count);
});
But, if I define the function click
here, the id on my div is NaN
while if I put the function of click
in document.ready()
, it creates two divs. I only want to create a div with incremental Id for each click!
javascript jquery html
javascript jquery html
edited Nov 22 at 19:42
Roy Scheffers
2,15291725
2,15291725
asked Nov 22 at 18:42
user10276822
43
43
Have a look at whatvar
does and how variables defined withvar
work: developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/…
– Andreas
Nov 22 at 18:45
I think the problem is that you have to change the elements attribute and not just a javascript variable
– tobifasc
Nov 22 at 18:53
The problem is in variable scope check jsfiddle.net/f9ug1dyv
– Mohammad
Nov 22 at 18:57
add a comment |
Have a look at whatvar
does and how variables defined withvar
work: developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/…
– Andreas
Nov 22 at 18:45
I think the problem is that you have to change the elements attribute and not just a javascript variable
– tobifasc
Nov 22 at 18:53
The problem is in variable scope check jsfiddle.net/f9ug1dyv
– Mohammad
Nov 22 at 18:57
Have a look at what
var
does and how variables defined with var
work: developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/…– Andreas
Nov 22 at 18:45
Have a look at what
var
does and how variables defined with var
work: developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/…– Andreas
Nov 22 at 18:45
I think the problem is that you have to change the elements attribute and not just a javascript variable
– tobifasc
Nov 22 at 18:53
I think the problem is that you have to change the elements attribute and not just a javascript variable
– tobifasc
Nov 22 at 18:53
The problem is in variable scope check jsfiddle.net/f9ug1dyv
– Mohammad
Nov 22 at 18:57
The problem is in variable scope check jsfiddle.net/f9ug1dyv
– Mohammad
Nov 22 at 18:57
add a comment |
5 Answers
5
active
oldest
votes
try this
var count=0;
$(document).ready(function(){
$("#add-div").click(function(){
count++;
$("body").append('<div id="'+count+'">div number '+count+'</div>');
});
});
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<body>
<button id="add-div">add div</button>
<br>
<br>
</body>
Please, Don't just "Try this". Explain in a couple of paragraph why this works.
– Roko C. Buljan
Nov 22 at 19:02
thanks, but this is a simple code, he will know the error
– abdulsattar-alkhalaf
Nov 22 at 19:04
add a comment |
This was due to scope issue.
Try this code:
$( document ).ready(function() {
var count =0;
console.log(count);
$( "#button" ).click(function() {
count=count+1;
addDiv(count);
});
});
You can remove console.log statement I used this for debugging purpose.
– Jahanzeb Awan
Nov 22 at 19:11
add a comment |
This is because of the scoping issue of count variable. Please note that JavaScript variables are accessible in scoping scenarios (block level scoping or function level scoping). More info here
What's wrong with your code is you have declared the count variable in one function and you are accessing it outside of it which is not allowed. Only to fix your code, I have made the count variable as global and now it will work as expected.
Note: Please note that global variables can be accessed anywhere in the execution context, so can be used with utmost care.
$( document ).ready(function()
{
//var count =0;
});
var count=0;
$('#button').on('click', function(){
count = count + 1;
alert(count);
});
//$( "#button" ).click(function() {
// count=count+1;
// addDiv(count);
//});
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<button id='button'>Click Me</button>
add a comment |
if you define a variable within a function, it is restricted only to this function, if you set it to a global scope, which is what you need in this case, the variable becomes available for all functions that are under this scope, look:
//global scope
var count = 0;
$( "#button" ).click(function() {
//function restrict scope
$('#increment').append(`<div>${count}</div>`)
count++;
});
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<body>
<button id="button">Click Me!</button>
<div id="increment">
</body>
See more at this page
add a comment |
It's a scope problem.
If you don't want to expose variables to the outer scope, you can use a closure.
let addDiv = function(){
let counter = 0;
return function(){
counter++;
let wrapper = $("#someDiv");
wrapper.append(`<div> id: ${counter}</div>`)
}
}();
button.on("click", function(){
addDiv();
});
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53436628%2fjquery-how-to-add-div-with-a-dynamic-id-that-increments-when-button-is-clicked%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
5 Answers
5
active
oldest
votes
5 Answers
5
active
oldest
votes
active
oldest
votes
active
oldest
votes
try this
var count=0;
$(document).ready(function(){
$("#add-div").click(function(){
count++;
$("body").append('<div id="'+count+'">div number '+count+'</div>');
});
});
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<body>
<button id="add-div">add div</button>
<br>
<br>
</body>
Please, Don't just "Try this". Explain in a couple of paragraph why this works.
– Roko C. Buljan
Nov 22 at 19:02
thanks, but this is a simple code, he will know the error
– abdulsattar-alkhalaf
Nov 22 at 19:04
add a comment |
try this
var count=0;
$(document).ready(function(){
$("#add-div").click(function(){
count++;
$("body").append('<div id="'+count+'">div number '+count+'</div>');
});
});
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<body>
<button id="add-div">add div</button>
<br>
<br>
</body>
Please, Don't just "Try this". Explain in a couple of paragraph why this works.
– Roko C. Buljan
Nov 22 at 19:02
thanks, but this is a simple code, he will know the error
– abdulsattar-alkhalaf
Nov 22 at 19:04
add a comment |
try this
var count=0;
$(document).ready(function(){
$("#add-div").click(function(){
count++;
$("body").append('<div id="'+count+'">div number '+count+'</div>');
});
});
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<body>
<button id="add-div">add div</button>
<br>
<br>
</body>
try this
var count=0;
$(document).ready(function(){
$("#add-div").click(function(){
count++;
$("body").append('<div id="'+count+'">div number '+count+'</div>');
});
});
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<body>
<button id="add-div">add div</button>
<br>
<br>
</body>
var count=0;
$(document).ready(function(){
$("#add-div").click(function(){
count++;
$("body").append('<div id="'+count+'">div number '+count+'</div>');
});
});
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<body>
<button id="add-div">add div</button>
<br>
<br>
</body>
var count=0;
$(document).ready(function(){
$("#add-div").click(function(){
count++;
$("body").append('<div id="'+count+'">div number '+count+'</div>');
});
});
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<body>
<button id="add-div">add div</button>
<br>
<br>
</body>
answered Nov 22 at 18:57
abdulsattar-alkhalaf
31715
31715
Please, Don't just "Try this". Explain in a couple of paragraph why this works.
– Roko C. Buljan
Nov 22 at 19:02
thanks, but this is a simple code, he will know the error
– abdulsattar-alkhalaf
Nov 22 at 19:04
add a comment |
Please, Don't just "Try this". Explain in a couple of paragraph why this works.
– Roko C. Buljan
Nov 22 at 19:02
thanks, but this is a simple code, he will know the error
– abdulsattar-alkhalaf
Nov 22 at 19:04
Please, Don't just "Try this". Explain in a couple of paragraph why this works.
– Roko C. Buljan
Nov 22 at 19:02
Please, Don't just "Try this". Explain in a couple of paragraph why this works.
– Roko C. Buljan
Nov 22 at 19:02
thanks, but this is a simple code, he will know the error
– abdulsattar-alkhalaf
Nov 22 at 19:04
thanks, but this is a simple code, he will know the error
– abdulsattar-alkhalaf
Nov 22 at 19:04
add a comment |
This was due to scope issue.
Try this code:
$( document ).ready(function() {
var count =0;
console.log(count);
$( "#button" ).click(function() {
count=count+1;
addDiv(count);
});
});
You can remove console.log statement I used this for debugging purpose.
– Jahanzeb Awan
Nov 22 at 19:11
add a comment |
This was due to scope issue.
Try this code:
$( document ).ready(function() {
var count =0;
console.log(count);
$( "#button" ).click(function() {
count=count+1;
addDiv(count);
});
});
You can remove console.log statement I used this for debugging purpose.
– Jahanzeb Awan
Nov 22 at 19:11
add a comment |
This was due to scope issue.
Try this code:
$( document ).ready(function() {
var count =0;
console.log(count);
$( "#button" ).click(function() {
count=count+1;
addDiv(count);
});
});
This was due to scope issue.
Try this code:
$( document ).ready(function() {
var count =0;
console.log(count);
$( "#button" ).click(function() {
count=count+1;
addDiv(count);
});
});
answered Nov 22 at 19:09


Jahanzeb Awan
569
569
You can remove console.log statement I used this for debugging purpose.
– Jahanzeb Awan
Nov 22 at 19:11
add a comment |
You can remove console.log statement I used this for debugging purpose.
– Jahanzeb Awan
Nov 22 at 19:11
You can remove console.log statement I used this for debugging purpose.
– Jahanzeb Awan
Nov 22 at 19:11
You can remove console.log statement I used this for debugging purpose.
– Jahanzeb Awan
Nov 22 at 19:11
add a comment |
This is because of the scoping issue of count variable. Please note that JavaScript variables are accessible in scoping scenarios (block level scoping or function level scoping). More info here
What's wrong with your code is you have declared the count variable in one function and you are accessing it outside of it which is not allowed. Only to fix your code, I have made the count variable as global and now it will work as expected.
Note: Please note that global variables can be accessed anywhere in the execution context, so can be used with utmost care.
$( document ).ready(function()
{
//var count =0;
});
var count=0;
$('#button').on('click', function(){
count = count + 1;
alert(count);
});
//$( "#button" ).click(function() {
// count=count+1;
// addDiv(count);
//});
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<button id='button'>Click Me</button>
add a comment |
This is because of the scoping issue of count variable. Please note that JavaScript variables are accessible in scoping scenarios (block level scoping or function level scoping). More info here
What's wrong with your code is you have declared the count variable in one function and you are accessing it outside of it which is not allowed. Only to fix your code, I have made the count variable as global and now it will work as expected.
Note: Please note that global variables can be accessed anywhere in the execution context, so can be used with utmost care.
$( document ).ready(function()
{
//var count =0;
});
var count=0;
$('#button').on('click', function(){
count = count + 1;
alert(count);
});
//$( "#button" ).click(function() {
// count=count+1;
// addDiv(count);
//});
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<button id='button'>Click Me</button>
add a comment |
This is because of the scoping issue of count variable. Please note that JavaScript variables are accessible in scoping scenarios (block level scoping or function level scoping). More info here
What's wrong with your code is you have declared the count variable in one function and you are accessing it outside of it which is not allowed. Only to fix your code, I have made the count variable as global and now it will work as expected.
Note: Please note that global variables can be accessed anywhere in the execution context, so can be used with utmost care.
$( document ).ready(function()
{
//var count =0;
});
var count=0;
$('#button').on('click', function(){
count = count + 1;
alert(count);
});
//$( "#button" ).click(function() {
// count=count+1;
// addDiv(count);
//});
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<button id='button'>Click Me</button>
This is because of the scoping issue of count variable. Please note that JavaScript variables are accessible in scoping scenarios (block level scoping or function level scoping). More info here
What's wrong with your code is you have declared the count variable in one function and you are accessing it outside of it which is not allowed. Only to fix your code, I have made the count variable as global and now it will work as expected.
Note: Please note that global variables can be accessed anywhere in the execution context, so can be used with utmost care.
$( document ).ready(function()
{
//var count =0;
});
var count=0;
$('#button').on('click', function(){
count = count + 1;
alert(count);
});
//$( "#button" ).click(function() {
// count=count+1;
// addDiv(count);
//});
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<button id='button'>Click Me</button>
$( document ).ready(function()
{
//var count =0;
});
var count=0;
$('#button').on('click', function(){
count = count + 1;
alert(count);
});
//$( "#button" ).click(function() {
// count=count+1;
// addDiv(count);
//});
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<button id='button'>Click Me</button>
$( document ).ready(function()
{
//var count =0;
});
var count=0;
$('#button').on('click', function(){
count = count + 1;
alert(count);
});
//$( "#button" ).click(function() {
// count=count+1;
// addDiv(count);
//});
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<button id='button'>Click Me</button>
answered Nov 22 at 19:00


Sahil Sharma
795619
795619
add a comment |
add a comment |
if you define a variable within a function, it is restricted only to this function, if you set it to a global scope, which is what you need in this case, the variable becomes available for all functions that are under this scope, look:
//global scope
var count = 0;
$( "#button" ).click(function() {
//function restrict scope
$('#increment').append(`<div>${count}</div>`)
count++;
});
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<body>
<button id="button">Click Me!</button>
<div id="increment">
</body>
See more at this page
add a comment |
if you define a variable within a function, it is restricted only to this function, if you set it to a global scope, which is what you need in this case, the variable becomes available for all functions that are under this scope, look:
//global scope
var count = 0;
$( "#button" ).click(function() {
//function restrict scope
$('#increment').append(`<div>${count}</div>`)
count++;
});
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<body>
<button id="button">Click Me!</button>
<div id="increment">
</body>
See more at this page
add a comment |
if you define a variable within a function, it is restricted only to this function, if you set it to a global scope, which is what you need in this case, the variable becomes available for all functions that are under this scope, look:
//global scope
var count = 0;
$( "#button" ).click(function() {
//function restrict scope
$('#increment').append(`<div>${count}</div>`)
count++;
});
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<body>
<button id="button">Click Me!</button>
<div id="increment">
</body>
See more at this page
if you define a variable within a function, it is restricted only to this function, if you set it to a global scope, which is what you need in this case, the variable becomes available for all functions that are under this scope, look:
//global scope
var count = 0;
$( "#button" ).click(function() {
//function restrict scope
$('#increment').append(`<div>${count}</div>`)
count++;
});
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<body>
<button id="button">Click Me!</button>
<div id="increment">
</body>
See more at this page
//global scope
var count = 0;
$( "#button" ).click(function() {
//function restrict scope
$('#increment').append(`<div>${count}</div>`)
count++;
});
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<body>
<button id="button">Click Me!</button>
<div id="increment">
</body>
//global scope
var count = 0;
$( "#button" ).click(function() {
//function restrict scope
$('#increment').append(`<div>${count}</div>`)
count++;
});
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<body>
<button id="button">Click Me!</button>
<div id="increment">
</body>
answered Nov 22 at 19:05


Demian
1637
1637
add a comment |
add a comment |
It's a scope problem.
If you don't want to expose variables to the outer scope, you can use a closure.
let addDiv = function(){
let counter = 0;
return function(){
counter++;
let wrapper = $("#someDiv");
wrapper.append(`<div> id: ${counter}</div>`)
}
}();
button.on("click", function(){
addDiv();
});
add a comment |
It's a scope problem.
If you don't want to expose variables to the outer scope, you can use a closure.
let addDiv = function(){
let counter = 0;
return function(){
counter++;
let wrapper = $("#someDiv");
wrapper.append(`<div> id: ${counter}</div>`)
}
}();
button.on("click", function(){
addDiv();
});
add a comment |
It's a scope problem.
If you don't want to expose variables to the outer scope, you can use a closure.
let addDiv = function(){
let counter = 0;
return function(){
counter++;
let wrapper = $("#someDiv");
wrapper.append(`<div> id: ${counter}</div>`)
}
}();
button.on("click", function(){
addDiv();
});
It's a scope problem.
If you don't want to expose variables to the outer scope, you can use a closure.
let addDiv = function(){
let counter = 0;
return function(){
counter++;
let wrapper = $("#someDiv");
wrapper.append(`<div> id: ${counter}</div>`)
}
}();
button.on("click", function(){
addDiv();
});
answered Nov 22 at 19:22
Dmitry Shevchenko
1
1
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53436628%2fjquery-how-to-add-div-with-a-dynamic-id-that-increments-when-button-is-clicked%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
uw,Q5np1c,fZt3orZ5Q gjnPSL I,PzRExhUiLj6kd3Jms
Have a look at what
var
does and how variables defined withvar
work: developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/…– Andreas
Nov 22 at 18:45
I think the problem is that you have to change the elements attribute and not just a javascript variable
– tobifasc
Nov 22 at 18:53
The problem is in variable scope check jsfiddle.net/f9ug1dyv
– Mohammad
Nov 22 at 18:57