Saving an custom object array that is appended constantly
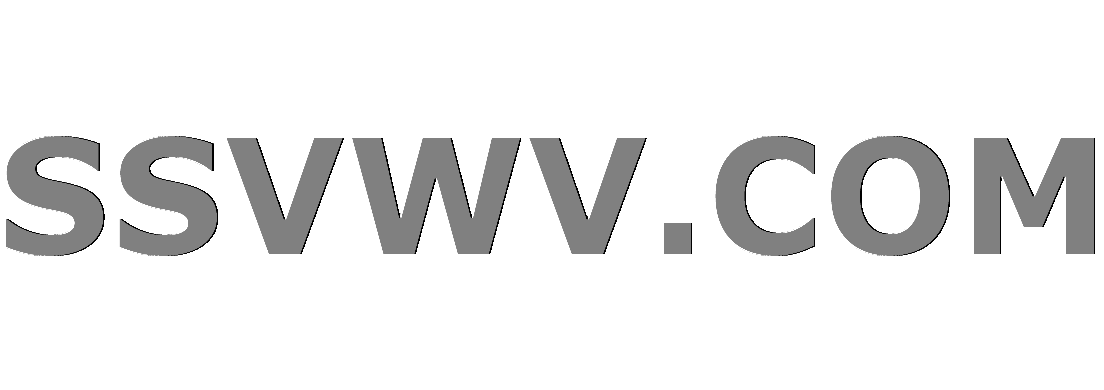
Multi tool use
up vote
0
down vote
favorite
I'm relatively new to Swift and coding in general. I'm trying to hone my skills at the moment but putting together a simple reminder app. I'm trying to get the back end working before I put together the story board but I have the essential story board elements to test if my system will work.
Basically I'm trying to save a array that contains a custom object, but this array is appended to each reminder addition done by the user. This is so that every time the app opens, the array will contain the reminders from last time.
Here is the code I have so far to create and append the list;
func createReminder() {
let reminderAdd = Reminder(chosenReminderDescription: textRetrieve.text!, chosenReminderLength: 1)
reminderList.append(reminderAdd)
dump(reminderList)
}
Here is the object code;
class Reminder {
var reminderDescription = "Require initalisation."
var reminderLength = 1 // in days
init (chosenReminderDescription: String, chosenReminderLength: Int) {
reminderDescription = chosenReminderDescription
reminderLength = chosenReminderLength
}
}
How would I go about saving the array?
EDIT:
This is what i've added so far.
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view, typically from a nib.
let reminderAdd = Reminder(chosenReminderDescription: "Placeholder test", chosenReminderLength: 1)
reminderList.append(reminderAdd)
let appDelegate = UIApplication.shared.delegate as! AppDelegate
let context = appDelegate.persistentContainer.viewContext
let entity = NSEntityDescription.entity(forEntityName: "Tasks", in: context)
let newTask = NSManagedObject(entity: entity!, insertInto: context)
newTask.setValue(reminderList, forKey: "taskName")
do {
try context.save()
} catch {
print("Failed saving")
}
let request = NSFetchRequest<NSFetchRequestResult>(entityName: "Tasks")
//request.predicate = NSPredicate(format: "age = %@", "12")
request.returnsObjectsAsFaults = false
do {
let result = try context.fetch(request)
for data in result as! [NSManagedObject] {
print(data.value(forKey: "taskName"))
}
} catch {
print("Failed")
}
I'm getting crashes and I can't seem to debug it as of yet. I believe this line is causing the crash as when I remove it the app launches fine.
let reminderAdd = Reminder(chosenReminderDescription: "Placeholder test", chosenReminderLength: 1)
reminderList.append(reminderAdd)
Any ideas?
EDIT 2:
datamodel
That is the data model, I'm not entirely sure what you mean to make the object into a codable. Thanks again.
EDIT 3:
ViewDidLoad
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view, typically from a nib
let appDelegate = UIApplication.shared.delegate as! AppDelegate
let context = appDelegate.persistentContainer.viewContext
let entity = NSEntityDescription.entity(forEntityName: "Tasks", in: context)
let newTask = Tasks(entity: entity!, insertInto: context)
newTask.setValue(reminderList, forKey: "taskName")
do {
try context.save()
} catch {
print("Failed saving")
}
let request = NSFetchRequest<NSFetchRequestResult>(entityName: "Tasks")
//request.predicate = NSPredicate(format: "age = %@", "12")
request.returnsObjectsAsFaults = false
do {
let result = try context.fetch(request)
for data in result as! [Tasks] {
print(data.value(forKey: "taskName"))
}
} catch {
print("Failed")
}
dump(reminderList)
}
arrays swift save
add a comment |
up vote
0
down vote
favorite
I'm relatively new to Swift and coding in general. I'm trying to hone my skills at the moment but putting together a simple reminder app. I'm trying to get the back end working before I put together the story board but I have the essential story board elements to test if my system will work.
Basically I'm trying to save a array that contains a custom object, but this array is appended to each reminder addition done by the user. This is so that every time the app opens, the array will contain the reminders from last time.
Here is the code I have so far to create and append the list;
func createReminder() {
let reminderAdd = Reminder(chosenReminderDescription: textRetrieve.text!, chosenReminderLength: 1)
reminderList.append(reminderAdd)
dump(reminderList)
}
Here is the object code;
class Reminder {
var reminderDescription = "Require initalisation."
var reminderLength = 1 // in days
init (chosenReminderDescription: String, chosenReminderLength: Int) {
reminderDescription = chosenReminderDescription
reminderLength = chosenReminderLength
}
}
How would I go about saving the array?
EDIT:
This is what i've added so far.
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view, typically from a nib.
let reminderAdd = Reminder(chosenReminderDescription: "Placeholder test", chosenReminderLength: 1)
reminderList.append(reminderAdd)
let appDelegate = UIApplication.shared.delegate as! AppDelegate
let context = appDelegate.persistentContainer.viewContext
let entity = NSEntityDescription.entity(forEntityName: "Tasks", in: context)
let newTask = NSManagedObject(entity: entity!, insertInto: context)
newTask.setValue(reminderList, forKey: "taskName")
do {
try context.save()
} catch {
print("Failed saving")
}
let request = NSFetchRequest<NSFetchRequestResult>(entityName: "Tasks")
//request.predicate = NSPredicate(format: "age = %@", "12")
request.returnsObjectsAsFaults = false
do {
let result = try context.fetch(request)
for data in result as! [NSManagedObject] {
print(data.value(forKey: "taskName"))
}
} catch {
print("Failed")
}
I'm getting crashes and I can't seem to debug it as of yet. I believe this line is causing the crash as when I remove it the app launches fine.
let reminderAdd = Reminder(chosenReminderDescription: "Placeholder test", chosenReminderLength: 1)
reminderList.append(reminderAdd)
Any ideas?
EDIT 2:
datamodel
That is the data model, I'm not entirely sure what you mean to make the object into a codable. Thanks again.
EDIT 3:
ViewDidLoad
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view, typically from a nib
let appDelegate = UIApplication.shared.delegate as! AppDelegate
let context = appDelegate.persistentContainer.viewContext
let entity = NSEntityDescription.entity(forEntityName: "Tasks", in: context)
let newTask = Tasks(entity: entity!, insertInto: context)
newTask.setValue(reminderList, forKey: "taskName")
do {
try context.save()
} catch {
print("Failed saving")
}
let request = NSFetchRequest<NSFetchRequestResult>(entityName: "Tasks")
//request.predicate = NSPredicate(format: "age = %@", "12")
request.returnsObjectsAsFaults = false
do {
let result = try context.fetch(request)
for data in result as! [Tasks] {
print(data.value(forKey: "taskName"))
}
} catch {
print("Failed")
}
dump(reminderList)
}
arrays swift save
You could save this to a.json
file, asqlite
database,NSUserDefaults
, send it off to a cloud somewhere, or many other persistence alternatives. What have you tried?
– Ian MacDonald
Nov 22 at 15:18
@Ian Don’t use UserDefaults to store app data.
– rmaddy
Nov 22 at 15:42
@rmaddy I mean, I said could, not should, right? :)
– Ian MacDonald
Nov 22 at 15:55
@IanMacDonald I messed around with the UserDefaults but couldn't get it to work. I'll have a play around tonight and see what I can come up with.
– sav
Nov 23 at 4:40
@IanMacDonald I've mucked around with the core data but I'm just came to some more problems. I believe the data is saving and fetching, but now that I have tried to add a value to the array so it can actually save something that isn't just an empty array, it crashes, please refer to my edit. Thanks, Sav.
– sav
Nov 23 at 6:06
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I'm relatively new to Swift and coding in general. I'm trying to hone my skills at the moment but putting together a simple reminder app. I'm trying to get the back end working before I put together the story board but I have the essential story board elements to test if my system will work.
Basically I'm trying to save a array that contains a custom object, but this array is appended to each reminder addition done by the user. This is so that every time the app opens, the array will contain the reminders from last time.
Here is the code I have so far to create and append the list;
func createReminder() {
let reminderAdd = Reminder(chosenReminderDescription: textRetrieve.text!, chosenReminderLength: 1)
reminderList.append(reminderAdd)
dump(reminderList)
}
Here is the object code;
class Reminder {
var reminderDescription = "Require initalisation."
var reminderLength = 1 // in days
init (chosenReminderDescription: String, chosenReminderLength: Int) {
reminderDescription = chosenReminderDescription
reminderLength = chosenReminderLength
}
}
How would I go about saving the array?
EDIT:
This is what i've added so far.
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view, typically from a nib.
let reminderAdd = Reminder(chosenReminderDescription: "Placeholder test", chosenReminderLength: 1)
reminderList.append(reminderAdd)
let appDelegate = UIApplication.shared.delegate as! AppDelegate
let context = appDelegate.persistentContainer.viewContext
let entity = NSEntityDescription.entity(forEntityName: "Tasks", in: context)
let newTask = NSManagedObject(entity: entity!, insertInto: context)
newTask.setValue(reminderList, forKey: "taskName")
do {
try context.save()
} catch {
print("Failed saving")
}
let request = NSFetchRequest<NSFetchRequestResult>(entityName: "Tasks")
//request.predicate = NSPredicate(format: "age = %@", "12")
request.returnsObjectsAsFaults = false
do {
let result = try context.fetch(request)
for data in result as! [NSManagedObject] {
print(data.value(forKey: "taskName"))
}
} catch {
print("Failed")
}
I'm getting crashes and I can't seem to debug it as of yet. I believe this line is causing the crash as when I remove it the app launches fine.
let reminderAdd = Reminder(chosenReminderDescription: "Placeholder test", chosenReminderLength: 1)
reminderList.append(reminderAdd)
Any ideas?
EDIT 2:
datamodel
That is the data model, I'm not entirely sure what you mean to make the object into a codable. Thanks again.
EDIT 3:
ViewDidLoad
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view, typically from a nib
let appDelegate = UIApplication.shared.delegate as! AppDelegate
let context = appDelegate.persistentContainer.viewContext
let entity = NSEntityDescription.entity(forEntityName: "Tasks", in: context)
let newTask = Tasks(entity: entity!, insertInto: context)
newTask.setValue(reminderList, forKey: "taskName")
do {
try context.save()
} catch {
print("Failed saving")
}
let request = NSFetchRequest<NSFetchRequestResult>(entityName: "Tasks")
//request.predicate = NSPredicate(format: "age = %@", "12")
request.returnsObjectsAsFaults = false
do {
let result = try context.fetch(request)
for data in result as! [Tasks] {
print(data.value(forKey: "taskName"))
}
} catch {
print("Failed")
}
dump(reminderList)
}
arrays swift save
I'm relatively new to Swift and coding in general. I'm trying to hone my skills at the moment but putting together a simple reminder app. I'm trying to get the back end working before I put together the story board but I have the essential story board elements to test if my system will work.
Basically I'm trying to save a array that contains a custom object, but this array is appended to each reminder addition done by the user. This is so that every time the app opens, the array will contain the reminders from last time.
Here is the code I have so far to create and append the list;
func createReminder() {
let reminderAdd = Reminder(chosenReminderDescription: textRetrieve.text!, chosenReminderLength: 1)
reminderList.append(reminderAdd)
dump(reminderList)
}
Here is the object code;
class Reminder {
var reminderDescription = "Require initalisation."
var reminderLength = 1 // in days
init (chosenReminderDescription: String, chosenReminderLength: Int) {
reminderDescription = chosenReminderDescription
reminderLength = chosenReminderLength
}
}
How would I go about saving the array?
EDIT:
This is what i've added so far.
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view, typically from a nib.
let reminderAdd = Reminder(chosenReminderDescription: "Placeholder test", chosenReminderLength: 1)
reminderList.append(reminderAdd)
let appDelegate = UIApplication.shared.delegate as! AppDelegate
let context = appDelegate.persistentContainer.viewContext
let entity = NSEntityDescription.entity(forEntityName: "Tasks", in: context)
let newTask = NSManagedObject(entity: entity!, insertInto: context)
newTask.setValue(reminderList, forKey: "taskName")
do {
try context.save()
} catch {
print("Failed saving")
}
let request = NSFetchRequest<NSFetchRequestResult>(entityName: "Tasks")
//request.predicate = NSPredicate(format: "age = %@", "12")
request.returnsObjectsAsFaults = false
do {
let result = try context.fetch(request)
for data in result as! [NSManagedObject] {
print(data.value(forKey: "taskName"))
}
} catch {
print("Failed")
}
I'm getting crashes and I can't seem to debug it as of yet. I believe this line is causing the crash as when I remove it the app launches fine.
let reminderAdd = Reminder(chosenReminderDescription: "Placeholder test", chosenReminderLength: 1)
reminderList.append(reminderAdd)
Any ideas?
EDIT 2:
datamodel
That is the data model, I'm not entirely sure what you mean to make the object into a codable. Thanks again.
EDIT 3:
ViewDidLoad
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view, typically from a nib
let appDelegate = UIApplication.shared.delegate as! AppDelegate
let context = appDelegate.persistentContainer.viewContext
let entity = NSEntityDescription.entity(forEntityName: "Tasks", in: context)
let newTask = Tasks(entity: entity!, insertInto: context)
newTask.setValue(reminderList, forKey: "taskName")
do {
try context.save()
} catch {
print("Failed saving")
}
let request = NSFetchRequest<NSFetchRequestResult>(entityName: "Tasks")
//request.predicate = NSPredicate(format: "age = %@", "12")
request.returnsObjectsAsFaults = false
do {
let result = try context.fetch(request)
for data in result as! [Tasks] {
print(data.value(forKey: "taskName"))
}
} catch {
print("Failed")
}
dump(reminderList)
}
arrays swift save
arrays swift save
edited Nov 23 at 13:44
asked Nov 22 at 15:13


sav
134
134
You could save this to a.json
file, asqlite
database,NSUserDefaults
, send it off to a cloud somewhere, or many other persistence alternatives. What have you tried?
– Ian MacDonald
Nov 22 at 15:18
@Ian Don’t use UserDefaults to store app data.
– rmaddy
Nov 22 at 15:42
@rmaddy I mean, I said could, not should, right? :)
– Ian MacDonald
Nov 22 at 15:55
@IanMacDonald I messed around with the UserDefaults but couldn't get it to work. I'll have a play around tonight and see what I can come up with.
– sav
Nov 23 at 4:40
@IanMacDonald I've mucked around with the core data but I'm just came to some more problems. I believe the data is saving and fetching, but now that I have tried to add a value to the array so it can actually save something that isn't just an empty array, it crashes, please refer to my edit. Thanks, Sav.
– sav
Nov 23 at 6:06
add a comment |
You could save this to a.json
file, asqlite
database,NSUserDefaults
, send it off to a cloud somewhere, or many other persistence alternatives. What have you tried?
– Ian MacDonald
Nov 22 at 15:18
@Ian Don’t use UserDefaults to store app data.
– rmaddy
Nov 22 at 15:42
@rmaddy I mean, I said could, not should, right? :)
– Ian MacDonald
Nov 22 at 15:55
@IanMacDonald I messed around with the UserDefaults but couldn't get it to work. I'll have a play around tonight and see what I can come up with.
– sav
Nov 23 at 4:40
@IanMacDonald I've mucked around with the core data but I'm just came to some more problems. I believe the data is saving and fetching, but now that I have tried to add a value to the array so it can actually save something that isn't just an empty array, it crashes, please refer to my edit. Thanks, Sav.
– sav
Nov 23 at 6:06
You could save this to a
.json
file, a sqlite
database, NSUserDefaults
, send it off to a cloud somewhere, or many other persistence alternatives. What have you tried?– Ian MacDonald
Nov 22 at 15:18
You could save this to a
.json
file, a sqlite
database, NSUserDefaults
, send it off to a cloud somewhere, or many other persistence alternatives. What have you tried?– Ian MacDonald
Nov 22 at 15:18
@Ian Don’t use UserDefaults to store app data.
– rmaddy
Nov 22 at 15:42
@Ian Don’t use UserDefaults to store app data.
– rmaddy
Nov 22 at 15:42
@rmaddy I mean, I said could, not should, right? :)
– Ian MacDonald
Nov 22 at 15:55
@rmaddy I mean, I said could, not should, right? :)
– Ian MacDonald
Nov 22 at 15:55
@IanMacDonald I messed around with the UserDefaults but couldn't get it to work. I'll have a play around tonight and see what I can come up with.
– sav
Nov 23 at 4:40
@IanMacDonald I messed around with the UserDefaults but couldn't get it to work. I'll have a play around tonight and see what I can come up with.
– sav
Nov 23 at 4:40
@IanMacDonald I've mucked around with the core data but I'm just came to some more problems. I believe the data is saving and fetching, but now that I have tried to add a value to the array so it can actually save something that isn't just an empty array, it crashes, please refer to my edit. Thanks, Sav.
– sav
Nov 23 at 6:06
@IanMacDonald I've mucked around with the core data but I'm just came to some more problems. I believe the data is saving and fetching, but now that I have tried to add a value to the array so it can actually save something that isn't just an empty array, it crashes, please refer to my edit. Thanks, Sav.
– sav
Nov 23 at 6:06
add a comment |
1 Answer
1
active
oldest
votes
up vote
-1
down vote
accepted
you could create an instance using CoreData and store it like an internal database.
These are some good tutorial to start with that:
https://medium.com/xcblog/core-data-with-swift-4-for-beginners-1fc067cca707
https://www.raywenderlich.com/7569-getting-started-with-core-data-tutorial
EDIT 2
As you can see in this image,
https://ibb.co/f1axcA
my list in coreData is of type [Notifica], so is an array of object Notifica, to implement codable you should do something like this
public class Notifica: NSObject, NSCoding {
public required init?(coder aDecoder: NSCoder) {
self.id = aDecoder.decodeObject(forKey: "id") as? Double
self.type = aDecoder.decodeObject(forKey: "type") as? String
self.idEvent = aDecoder.decodeObject(forKey: "idEvent") as? Int
self.contactPerson = aDecoder.decodeObject(forKey: "contactPerson") as? People
self.title = aDecoder.decodeObject(forKey: "title") as? String
self.date = aDecoder.decodeObject(forKey: "date") as? String
}
public func encode(with aCoder: NSCoder) {
aCoder.encode(id, forKey: "id")
aCoder.encode(type, forKey: "type")
aCoder.encode(idEvent, forKey: "idEvent")
aCoder.encode(contactPerson, forKey: "contactPerson")
aCoder.encode(title, forKey: "title")
aCoder.encode(date, forKey: "date")
}
ecc..
Another thing is to not call NSManagedObject and pass the entity, but you should name that Tasks as you called in dataModel, if you type Tasks on xcode it will fin for you the NSManagedObject created and then you can set the value for taskName
EDIT 3
"<Simple_Reminders.Reminder: 0x60400046da40>
" means that a Reminder object exist! So you saved it! Reminder has two variable:
-reminderDescription and
-reminderLength, so change your code
do {
let result = try context.fetch(request)
for data in result as! [Tasks] {
print(data.value(forKey: "taskName"))
}
} catch {
print("Failed")
}
with this
do {
let result = try context.fetch(request)
for data in result as! [Tasks] {
print(data.value(forKey: "taskName"))
if let reminders = data.value(forKey: "taskName") as? [Reminder] {
for reminder in reminders {
// Now you have your single object Reminder and you can print his variables
print("Your reminder description is (reminder. reminderDescription), and his length is (reminder. reminderLength))"
}
}
}
} catch {
print("Failed")
}
I've mucked around with the core data but I'm just came to some more problems. I believe the data is saving and fetching, but now that I have tried to add a value to the array so it can actually save something that isn't just an empty array, it crashes, please refer to my edit. Thanks, Sav.
– sav
Nov 23 at 6:05
let newTask = NSManagedObject(entity: entity!, insertInto: context) First of all you should use your object "Reminder" instead NSManagedObject, and after that you should modify your object "Reminder" into a codable, can i see how you create your database .xcdatamodeld ?
– Francesco Destino
Nov 23 at 8:35
While this link may answer the question, it is better to include the essential parts of the answer here and provide the link for reference. Link-only answers can become invalid if the linked page changes. - From Review
– Dev_Tandel
Nov 23 at 9:48
@FrancescoDestino I have edited again, showing the data model. How do I go about changing the Reminder object into a codable?
– sav
Nov 23 at 11:26
@FrancescoDestino I believe that has worked but now I'm just getting a crash from the unwrapped optional as xcode wouldn't accept the ?, only the !. I believe this is because there is no values set in the array to begin with.
– sav
Nov 23 at 12:10
|
show 8 more comments
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
-1
down vote
accepted
you could create an instance using CoreData and store it like an internal database.
These are some good tutorial to start with that:
https://medium.com/xcblog/core-data-with-swift-4-for-beginners-1fc067cca707
https://www.raywenderlich.com/7569-getting-started-with-core-data-tutorial
EDIT 2
As you can see in this image,
https://ibb.co/f1axcA
my list in coreData is of type [Notifica], so is an array of object Notifica, to implement codable you should do something like this
public class Notifica: NSObject, NSCoding {
public required init?(coder aDecoder: NSCoder) {
self.id = aDecoder.decodeObject(forKey: "id") as? Double
self.type = aDecoder.decodeObject(forKey: "type") as? String
self.idEvent = aDecoder.decodeObject(forKey: "idEvent") as? Int
self.contactPerson = aDecoder.decodeObject(forKey: "contactPerson") as? People
self.title = aDecoder.decodeObject(forKey: "title") as? String
self.date = aDecoder.decodeObject(forKey: "date") as? String
}
public func encode(with aCoder: NSCoder) {
aCoder.encode(id, forKey: "id")
aCoder.encode(type, forKey: "type")
aCoder.encode(idEvent, forKey: "idEvent")
aCoder.encode(contactPerson, forKey: "contactPerson")
aCoder.encode(title, forKey: "title")
aCoder.encode(date, forKey: "date")
}
ecc..
Another thing is to not call NSManagedObject and pass the entity, but you should name that Tasks as you called in dataModel, if you type Tasks on xcode it will fin for you the NSManagedObject created and then you can set the value for taskName
EDIT 3
"<Simple_Reminders.Reminder: 0x60400046da40>
" means that a Reminder object exist! So you saved it! Reminder has two variable:
-reminderDescription and
-reminderLength, so change your code
do {
let result = try context.fetch(request)
for data in result as! [Tasks] {
print(data.value(forKey: "taskName"))
}
} catch {
print("Failed")
}
with this
do {
let result = try context.fetch(request)
for data in result as! [Tasks] {
print(data.value(forKey: "taskName"))
if let reminders = data.value(forKey: "taskName") as? [Reminder] {
for reminder in reminders {
// Now you have your single object Reminder and you can print his variables
print("Your reminder description is (reminder. reminderDescription), and his length is (reminder. reminderLength))"
}
}
}
} catch {
print("Failed")
}
I've mucked around with the core data but I'm just came to some more problems. I believe the data is saving and fetching, but now that I have tried to add a value to the array so it can actually save something that isn't just an empty array, it crashes, please refer to my edit. Thanks, Sav.
– sav
Nov 23 at 6:05
let newTask = NSManagedObject(entity: entity!, insertInto: context) First of all you should use your object "Reminder" instead NSManagedObject, and after that you should modify your object "Reminder" into a codable, can i see how you create your database .xcdatamodeld ?
– Francesco Destino
Nov 23 at 8:35
While this link may answer the question, it is better to include the essential parts of the answer here and provide the link for reference. Link-only answers can become invalid if the linked page changes. - From Review
– Dev_Tandel
Nov 23 at 9:48
@FrancescoDestino I have edited again, showing the data model. How do I go about changing the Reminder object into a codable?
– sav
Nov 23 at 11:26
@FrancescoDestino I believe that has worked but now I'm just getting a crash from the unwrapped optional as xcode wouldn't accept the ?, only the !. I believe this is because there is no values set in the array to begin with.
– sav
Nov 23 at 12:10
|
show 8 more comments
up vote
-1
down vote
accepted
you could create an instance using CoreData and store it like an internal database.
These are some good tutorial to start with that:
https://medium.com/xcblog/core-data-with-swift-4-for-beginners-1fc067cca707
https://www.raywenderlich.com/7569-getting-started-with-core-data-tutorial
EDIT 2
As you can see in this image,
https://ibb.co/f1axcA
my list in coreData is of type [Notifica], so is an array of object Notifica, to implement codable you should do something like this
public class Notifica: NSObject, NSCoding {
public required init?(coder aDecoder: NSCoder) {
self.id = aDecoder.decodeObject(forKey: "id") as? Double
self.type = aDecoder.decodeObject(forKey: "type") as? String
self.idEvent = aDecoder.decodeObject(forKey: "idEvent") as? Int
self.contactPerson = aDecoder.decodeObject(forKey: "contactPerson") as? People
self.title = aDecoder.decodeObject(forKey: "title") as? String
self.date = aDecoder.decodeObject(forKey: "date") as? String
}
public func encode(with aCoder: NSCoder) {
aCoder.encode(id, forKey: "id")
aCoder.encode(type, forKey: "type")
aCoder.encode(idEvent, forKey: "idEvent")
aCoder.encode(contactPerson, forKey: "contactPerson")
aCoder.encode(title, forKey: "title")
aCoder.encode(date, forKey: "date")
}
ecc..
Another thing is to not call NSManagedObject and pass the entity, but you should name that Tasks as you called in dataModel, if you type Tasks on xcode it will fin for you the NSManagedObject created and then you can set the value for taskName
EDIT 3
"<Simple_Reminders.Reminder: 0x60400046da40>
" means that a Reminder object exist! So you saved it! Reminder has two variable:
-reminderDescription and
-reminderLength, so change your code
do {
let result = try context.fetch(request)
for data in result as! [Tasks] {
print(data.value(forKey: "taskName"))
}
} catch {
print("Failed")
}
with this
do {
let result = try context.fetch(request)
for data in result as! [Tasks] {
print(data.value(forKey: "taskName"))
if let reminders = data.value(forKey: "taskName") as? [Reminder] {
for reminder in reminders {
// Now you have your single object Reminder and you can print his variables
print("Your reminder description is (reminder. reminderDescription), and his length is (reminder. reminderLength))"
}
}
}
} catch {
print("Failed")
}
I've mucked around with the core data but I'm just came to some more problems. I believe the data is saving and fetching, but now that I have tried to add a value to the array so it can actually save something that isn't just an empty array, it crashes, please refer to my edit. Thanks, Sav.
– sav
Nov 23 at 6:05
let newTask = NSManagedObject(entity: entity!, insertInto: context) First of all you should use your object "Reminder" instead NSManagedObject, and after that you should modify your object "Reminder" into a codable, can i see how you create your database .xcdatamodeld ?
– Francesco Destino
Nov 23 at 8:35
While this link may answer the question, it is better to include the essential parts of the answer here and provide the link for reference. Link-only answers can become invalid if the linked page changes. - From Review
– Dev_Tandel
Nov 23 at 9:48
@FrancescoDestino I have edited again, showing the data model. How do I go about changing the Reminder object into a codable?
– sav
Nov 23 at 11:26
@FrancescoDestino I believe that has worked but now I'm just getting a crash from the unwrapped optional as xcode wouldn't accept the ?, only the !. I believe this is because there is no values set in the array to begin with.
– sav
Nov 23 at 12:10
|
show 8 more comments
up vote
-1
down vote
accepted
up vote
-1
down vote
accepted
you could create an instance using CoreData and store it like an internal database.
These are some good tutorial to start with that:
https://medium.com/xcblog/core-data-with-swift-4-for-beginners-1fc067cca707
https://www.raywenderlich.com/7569-getting-started-with-core-data-tutorial
EDIT 2
As you can see in this image,
https://ibb.co/f1axcA
my list in coreData is of type [Notifica], so is an array of object Notifica, to implement codable you should do something like this
public class Notifica: NSObject, NSCoding {
public required init?(coder aDecoder: NSCoder) {
self.id = aDecoder.decodeObject(forKey: "id") as? Double
self.type = aDecoder.decodeObject(forKey: "type") as? String
self.idEvent = aDecoder.decodeObject(forKey: "idEvent") as? Int
self.contactPerson = aDecoder.decodeObject(forKey: "contactPerson") as? People
self.title = aDecoder.decodeObject(forKey: "title") as? String
self.date = aDecoder.decodeObject(forKey: "date") as? String
}
public func encode(with aCoder: NSCoder) {
aCoder.encode(id, forKey: "id")
aCoder.encode(type, forKey: "type")
aCoder.encode(idEvent, forKey: "idEvent")
aCoder.encode(contactPerson, forKey: "contactPerson")
aCoder.encode(title, forKey: "title")
aCoder.encode(date, forKey: "date")
}
ecc..
Another thing is to not call NSManagedObject and pass the entity, but you should name that Tasks as you called in dataModel, if you type Tasks on xcode it will fin for you the NSManagedObject created and then you can set the value for taskName
EDIT 3
"<Simple_Reminders.Reminder: 0x60400046da40>
" means that a Reminder object exist! So you saved it! Reminder has two variable:
-reminderDescription and
-reminderLength, so change your code
do {
let result = try context.fetch(request)
for data in result as! [Tasks] {
print(data.value(forKey: "taskName"))
}
} catch {
print("Failed")
}
with this
do {
let result = try context.fetch(request)
for data in result as! [Tasks] {
print(data.value(forKey: "taskName"))
if let reminders = data.value(forKey: "taskName") as? [Reminder] {
for reminder in reminders {
// Now you have your single object Reminder and you can print his variables
print("Your reminder description is (reminder. reminderDescription), and his length is (reminder. reminderLength))"
}
}
}
} catch {
print("Failed")
}
you could create an instance using CoreData and store it like an internal database.
These are some good tutorial to start with that:
https://medium.com/xcblog/core-data-with-swift-4-for-beginners-1fc067cca707
https://www.raywenderlich.com/7569-getting-started-with-core-data-tutorial
EDIT 2
As you can see in this image,
https://ibb.co/f1axcA
my list in coreData is of type [Notifica], so is an array of object Notifica, to implement codable you should do something like this
public class Notifica: NSObject, NSCoding {
public required init?(coder aDecoder: NSCoder) {
self.id = aDecoder.decodeObject(forKey: "id") as? Double
self.type = aDecoder.decodeObject(forKey: "type") as? String
self.idEvent = aDecoder.decodeObject(forKey: "idEvent") as? Int
self.contactPerson = aDecoder.decodeObject(forKey: "contactPerson") as? People
self.title = aDecoder.decodeObject(forKey: "title") as? String
self.date = aDecoder.decodeObject(forKey: "date") as? String
}
public func encode(with aCoder: NSCoder) {
aCoder.encode(id, forKey: "id")
aCoder.encode(type, forKey: "type")
aCoder.encode(idEvent, forKey: "idEvent")
aCoder.encode(contactPerson, forKey: "contactPerson")
aCoder.encode(title, forKey: "title")
aCoder.encode(date, forKey: "date")
}
ecc..
Another thing is to not call NSManagedObject and pass the entity, but you should name that Tasks as you called in dataModel, if you type Tasks on xcode it will fin for you the NSManagedObject created and then you can set the value for taskName
EDIT 3
"<Simple_Reminders.Reminder: 0x60400046da40>
" means that a Reminder object exist! So you saved it! Reminder has two variable:
-reminderDescription and
-reminderLength, so change your code
do {
let result = try context.fetch(request)
for data in result as! [Tasks] {
print(data.value(forKey: "taskName"))
}
} catch {
print("Failed")
}
with this
do {
let result = try context.fetch(request)
for data in result as! [Tasks] {
print(data.value(forKey: "taskName"))
if let reminders = data.value(forKey: "taskName") as? [Reminder] {
for reminder in reminders {
// Now you have your single object Reminder and you can print his variables
print("Your reminder description is (reminder. reminderDescription), and his length is (reminder. reminderLength))"
}
}
}
} catch {
print("Failed")
}
edited Nov 23 at 14:15
answered Nov 22 at 15:35
Francesco Destino
2369
2369
I've mucked around with the core data but I'm just came to some more problems. I believe the data is saving and fetching, but now that I have tried to add a value to the array so it can actually save something that isn't just an empty array, it crashes, please refer to my edit. Thanks, Sav.
– sav
Nov 23 at 6:05
let newTask = NSManagedObject(entity: entity!, insertInto: context) First of all you should use your object "Reminder" instead NSManagedObject, and after that you should modify your object "Reminder" into a codable, can i see how you create your database .xcdatamodeld ?
– Francesco Destino
Nov 23 at 8:35
While this link may answer the question, it is better to include the essential parts of the answer here and provide the link for reference. Link-only answers can become invalid if the linked page changes. - From Review
– Dev_Tandel
Nov 23 at 9:48
@FrancescoDestino I have edited again, showing the data model. How do I go about changing the Reminder object into a codable?
– sav
Nov 23 at 11:26
@FrancescoDestino I believe that has worked but now I'm just getting a crash from the unwrapped optional as xcode wouldn't accept the ?, only the !. I believe this is because there is no values set in the array to begin with.
– sav
Nov 23 at 12:10
|
show 8 more comments
I've mucked around with the core data but I'm just came to some more problems. I believe the data is saving and fetching, but now that I have tried to add a value to the array so it can actually save something that isn't just an empty array, it crashes, please refer to my edit. Thanks, Sav.
– sav
Nov 23 at 6:05
let newTask = NSManagedObject(entity: entity!, insertInto: context) First of all you should use your object "Reminder" instead NSManagedObject, and after that you should modify your object "Reminder" into a codable, can i see how you create your database .xcdatamodeld ?
– Francesco Destino
Nov 23 at 8:35
While this link may answer the question, it is better to include the essential parts of the answer here and provide the link for reference. Link-only answers can become invalid if the linked page changes. - From Review
– Dev_Tandel
Nov 23 at 9:48
@FrancescoDestino I have edited again, showing the data model. How do I go about changing the Reminder object into a codable?
– sav
Nov 23 at 11:26
@FrancescoDestino I believe that has worked but now I'm just getting a crash from the unwrapped optional as xcode wouldn't accept the ?, only the !. I believe this is because there is no values set in the array to begin with.
– sav
Nov 23 at 12:10
I've mucked around with the core data but I'm just came to some more problems. I believe the data is saving and fetching, but now that I have tried to add a value to the array so it can actually save something that isn't just an empty array, it crashes, please refer to my edit. Thanks, Sav.
– sav
Nov 23 at 6:05
I've mucked around with the core data but I'm just came to some more problems. I believe the data is saving and fetching, but now that I have tried to add a value to the array so it can actually save something that isn't just an empty array, it crashes, please refer to my edit. Thanks, Sav.
– sav
Nov 23 at 6:05
let newTask = NSManagedObject(entity: entity!, insertInto: context) First of all you should use your object "Reminder" instead NSManagedObject, and after that you should modify your object "Reminder" into a codable, can i see how you create your database .xcdatamodeld ?
– Francesco Destino
Nov 23 at 8:35
let newTask = NSManagedObject(entity: entity!, insertInto: context) First of all you should use your object "Reminder" instead NSManagedObject, and after that you should modify your object "Reminder" into a codable, can i see how you create your database .xcdatamodeld ?
– Francesco Destino
Nov 23 at 8:35
While this link may answer the question, it is better to include the essential parts of the answer here and provide the link for reference. Link-only answers can become invalid if the linked page changes. - From Review
– Dev_Tandel
Nov 23 at 9:48
While this link may answer the question, it is better to include the essential parts of the answer here and provide the link for reference. Link-only answers can become invalid if the linked page changes. - From Review
– Dev_Tandel
Nov 23 at 9:48
@FrancescoDestino I have edited again, showing the data model. How do I go about changing the Reminder object into a codable?
– sav
Nov 23 at 11:26
@FrancescoDestino I have edited again, showing the data model. How do I go about changing the Reminder object into a codable?
– sav
Nov 23 at 11:26
@FrancescoDestino I believe that has worked but now I'm just getting a crash from the unwrapped optional as xcode wouldn't accept the ?, only the !. I believe this is because there is no values set in the array to begin with.
– sav
Nov 23 at 12:10
@FrancescoDestino I believe that has worked but now I'm just getting a crash from the unwrapped optional as xcode wouldn't accept the ?, only the !. I believe this is because there is no values set in the array to begin with.
– sav
Nov 23 at 12:10
|
show 8 more comments
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53433865%2fsaving-an-custom-object-array-that-is-appended-constantly%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
OFWRA7sIIX3DwxI0guqNiiKoWadRa9RbRomcW8zlCJU 27
You could save this to a
.json
file, asqlite
database,NSUserDefaults
, send it off to a cloud somewhere, or many other persistence alternatives. What have you tried?– Ian MacDonald
Nov 22 at 15:18
@Ian Don’t use UserDefaults to store app data.
– rmaddy
Nov 22 at 15:42
@rmaddy I mean, I said could, not should, right? :)
– Ian MacDonald
Nov 22 at 15:55
@IanMacDonald I messed around with the UserDefaults but couldn't get it to work. I'll have a play around tonight and see what I can come up with.
– sav
Nov 23 at 4:40
@IanMacDonald I've mucked around with the core data but I'm just came to some more problems. I believe the data is saving and fetching, but now that I have tried to add a value to the array so it can actually save something that isn't just an empty array, it crashes, please refer to my edit. Thanks, Sav.
– sav
Nov 23 at 6:06