how to mock class in java without creating interface
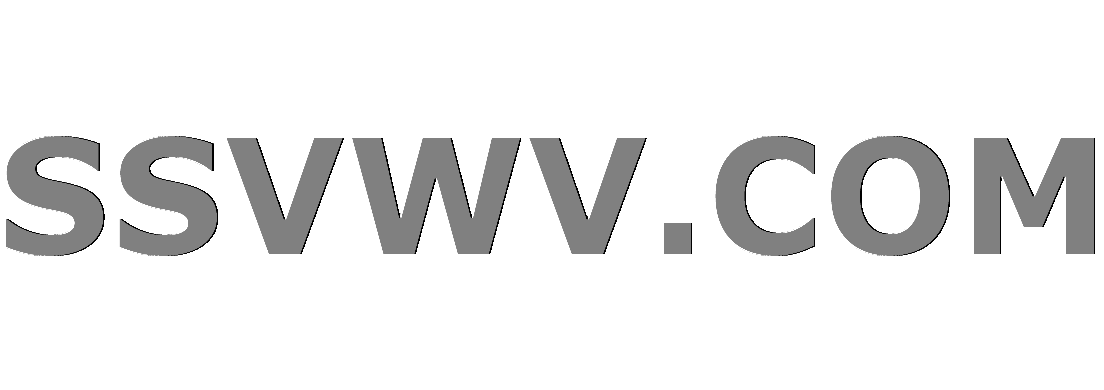
Multi tool use
First, I am not Java developer, I am using php.
I am curious in Java, for example if I have structure like this:
interface Ainterface {
public String method();
}
public class A implements Ainterface {
public String method() {
//do something
}
}
public class B {
public String method(Ainterface a) {
a.method();
//do something
}
}
Now if I want to test B
's method I can mock a
public class Amock implements Ainterface {
public String method() {
//do something
}
}
And inject it into B
's method.
But, if I don't want to create interface and I have situation like this:
public class A {
public String method() {
//do something
}
}
public class B {
public String method(A a) {
a.method();
//do something
}
}
Is there any way to mock a
or test B
's method in other way?
java testing mocking
add a comment |
First, I am not Java developer, I am using php.
I am curious in Java, for example if I have structure like this:
interface Ainterface {
public String method();
}
public class A implements Ainterface {
public String method() {
//do something
}
}
public class B {
public String method(Ainterface a) {
a.method();
//do something
}
}
Now if I want to test B
's method I can mock a
public class Amock implements Ainterface {
public String method() {
//do something
}
}
And inject it into B
's method.
But, if I don't want to create interface and I have situation like this:
public class A {
public String method() {
//do something
}
}
public class B {
public String method(A a) {
a.method();
//do something
}
}
Is there any way to mock a
or test B
's method in other way?
java testing mocking
add a comment |
First, I am not Java developer, I am using php.
I am curious in Java, for example if I have structure like this:
interface Ainterface {
public String method();
}
public class A implements Ainterface {
public String method() {
//do something
}
}
public class B {
public String method(Ainterface a) {
a.method();
//do something
}
}
Now if I want to test B
's method I can mock a
public class Amock implements Ainterface {
public String method() {
//do something
}
}
And inject it into B
's method.
But, if I don't want to create interface and I have situation like this:
public class A {
public String method() {
//do something
}
}
public class B {
public String method(A a) {
a.method();
//do something
}
}
Is there any way to mock a
or test B
's method in other way?
java testing mocking
First, I am not Java developer, I am using php.
I am curious in Java, for example if I have structure like this:
interface Ainterface {
public String method();
}
public class A implements Ainterface {
public String method() {
//do something
}
}
public class B {
public String method(Ainterface a) {
a.method();
//do something
}
}
Now if I want to test B
's method I can mock a
public class Amock implements Ainterface {
public String method() {
//do something
}
}
And inject it into B
's method.
But, if I don't want to create interface and I have situation like this:
public class A {
public String method() {
//do something
}
}
public class B {
public String method(A a) {
a.method();
//do something
}
}
Is there any way to mock a
or test B
's method in other way?
java testing mocking
java testing mocking
asked Nov 22 at 22:38


Nika Khurashvili
71712
71712
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
In Java you can use specific mocking framework like Mockito and use it's specific method - for example
A aMock = Mockito.mock(A.class);
Of course this way you can only create a really simple mock that will do literally nothing but the framework allows you to define what specific method should return with providing when/then
mechanism. The example of such can be
when(aMock.method()).thenReturn("I am just a fake");
The mockito is really powerfull and I cannot explain you whole framework in this answer so please visit Mockito home and reference page to get familiar with this
Also Mockito is one of solutions - it's kind of popular but not only the one so you can look for the solution that fits your requirements best
1
Thanks for answer, I get that, but if I don't want to use some framework is there any native way?
– Nika Khurashvili
Nov 22 at 22:45
1
nooooooooope :)
– m.antkowicz
Nov 22 at 22:47
Yes, but that would be reinventing the wheel, producing code to do something similar that mockito does. (Building your own mocking framework, for example with Proxies)
– Pascal Ludwig
Nov 22 at 23:17
Building your own mocking framework is not a native way
– m.antkowicz
Nov 23 at 7:37
add a comment |
@m.antkowicz's answer shows the correct way of doing it, by using a mocking framework. In the comments you asked for a way without using external framework, so this answer tries to address that.
Just like you created Amock
by implementing Ainterface
, you can create a child class that extends A
.
So you would have a class like class AmockClassBased extends A
and then you can override method()
to make it do what you were doing in Amock
class's method()
.
So your Amock
will be changed to:-
public class AmockClassBased extends A {
@Override
public String method() {
//do something
}
}
Then you can pass an instance of this class to B
's method(A a)
.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53438731%2fhow-to-mock-class-in-java-without-creating-interface%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
In Java you can use specific mocking framework like Mockito and use it's specific method - for example
A aMock = Mockito.mock(A.class);
Of course this way you can only create a really simple mock that will do literally nothing but the framework allows you to define what specific method should return with providing when/then
mechanism. The example of such can be
when(aMock.method()).thenReturn("I am just a fake");
The mockito is really powerfull and I cannot explain you whole framework in this answer so please visit Mockito home and reference page to get familiar with this
Also Mockito is one of solutions - it's kind of popular but not only the one so you can look for the solution that fits your requirements best
1
Thanks for answer, I get that, but if I don't want to use some framework is there any native way?
– Nika Khurashvili
Nov 22 at 22:45
1
nooooooooope :)
– m.antkowicz
Nov 22 at 22:47
Yes, but that would be reinventing the wheel, producing code to do something similar that mockito does. (Building your own mocking framework, for example with Proxies)
– Pascal Ludwig
Nov 22 at 23:17
Building your own mocking framework is not a native way
– m.antkowicz
Nov 23 at 7:37
add a comment |
In Java you can use specific mocking framework like Mockito and use it's specific method - for example
A aMock = Mockito.mock(A.class);
Of course this way you can only create a really simple mock that will do literally nothing but the framework allows you to define what specific method should return with providing when/then
mechanism. The example of such can be
when(aMock.method()).thenReturn("I am just a fake");
The mockito is really powerfull and I cannot explain you whole framework in this answer so please visit Mockito home and reference page to get familiar with this
Also Mockito is one of solutions - it's kind of popular but not only the one so you can look for the solution that fits your requirements best
1
Thanks for answer, I get that, but if I don't want to use some framework is there any native way?
– Nika Khurashvili
Nov 22 at 22:45
1
nooooooooope :)
– m.antkowicz
Nov 22 at 22:47
Yes, but that would be reinventing the wheel, producing code to do something similar that mockito does. (Building your own mocking framework, for example with Proxies)
– Pascal Ludwig
Nov 22 at 23:17
Building your own mocking framework is not a native way
– m.antkowicz
Nov 23 at 7:37
add a comment |
In Java you can use specific mocking framework like Mockito and use it's specific method - for example
A aMock = Mockito.mock(A.class);
Of course this way you can only create a really simple mock that will do literally nothing but the framework allows you to define what specific method should return with providing when/then
mechanism. The example of such can be
when(aMock.method()).thenReturn("I am just a fake");
The mockito is really powerfull and I cannot explain you whole framework in this answer so please visit Mockito home and reference page to get familiar with this
Also Mockito is one of solutions - it's kind of popular but not only the one so you can look for the solution that fits your requirements best
In Java you can use specific mocking framework like Mockito and use it's specific method - for example
A aMock = Mockito.mock(A.class);
Of course this way you can only create a really simple mock that will do literally nothing but the framework allows you to define what specific method should return with providing when/then
mechanism. The example of such can be
when(aMock.method()).thenReturn("I am just a fake");
The mockito is really powerfull and I cannot explain you whole framework in this answer so please visit Mockito home and reference page to get familiar with this
Also Mockito is one of solutions - it's kind of popular but not only the one so you can look for the solution that fits your requirements best
edited Nov 22 at 22:48
answered Nov 22 at 22:40
m.antkowicz
8,542928
8,542928
1
Thanks for answer, I get that, but if I don't want to use some framework is there any native way?
– Nika Khurashvili
Nov 22 at 22:45
1
nooooooooope :)
– m.antkowicz
Nov 22 at 22:47
Yes, but that would be reinventing the wheel, producing code to do something similar that mockito does. (Building your own mocking framework, for example with Proxies)
– Pascal Ludwig
Nov 22 at 23:17
Building your own mocking framework is not a native way
– m.antkowicz
Nov 23 at 7:37
add a comment |
1
Thanks for answer, I get that, but if I don't want to use some framework is there any native way?
– Nika Khurashvili
Nov 22 at 22:45
1
nooooooooope :)
– m.antkowicz
Nov 22 at 22:47
Yes, but that would be reinventing the wheel, producing code to do something similar that mockito does. (Building your own mocking framework, for example with Proxies)
– Pascal Ludwig
Nov 22 at 23:17
Building your own mocking framework is not a native way
– m.antkowicz
Nov 23 at 7:37
1
1
Thanks for answer, I get that, but if I don't want to use some framework is there any native way?
– Nika Khurashvili
Nov 22 at 22:45
Thanks for answer, I get that, but if I don't want to use some framework is there any native way?
– Nika Khurashvili
Nov 22 at 22:45
1
1
nooooooooope :)
– m.antkowicz
Nov 22 at 22:47
nooooooooope :)
– m.antkowicz
Nov 22 at 22:47
Yes, but that would be reinventing the wheel, producing code to do something similar that mockito does. (Building your own mocking framework, for example with Proxies)
– Pascal Ludwig
Nov 22 at 23:17
Yes, but that would be reinventing the wheel, producing code to do something similar that mockito does. (Building your own mocking framework, for example with Proxies)
– Pascal Ludwig
Nov 22 at 23:17
Building your own mocking framework is not a native way
– m.antkowicz
Nov 23 at 7:37
Building your own mocking framework is not a native way
– m.antkowicz
Nov 23 at 7:37
add a comment |
@m.antkowicz's answer shows the correct way of doing it, by using a mocking framework. In the comments you asked for a way without using external framework, so this answer tries to address that.
Just like you created Amock
by implementing Ainterface
, you can create a child class that extends A
.
So you would have a class like class AmockClassBased extends A
and then you can override method()
to make it do what you were doing in Amock
class's method()
.
So your Amock
will be changed to:-
public class AmockClassBased extends A {
@Override
public String method() {
//do something
}
}
Then you can pass an instance of this class to B
's method(A a)
.
add a comment |
@m.antkowicz's answer shows the correct way of doing it, by using a mocking framework. In the comments you asked for a way without using external framework, so this answer tries to address that.
Just like you created Amock
by implementing Ainterface
, you can create a child class that extends A
.
So you would have a class like class AmockClassBased extends A
and then you can override method()
to make it do what you were doing in Amock
class's method()
.
So your Amock
will be changed to:-
public class AmockClassBased extends A {
@Override
public String method() {
//do something
}
}
Then you can pass an instance of this class to B
's method(A a)
.
add a comment |
@m.antkowicz's answer shows the correct way of doing it, by using a mocking framework. In the comments you asked for a way without using external framework, so this answer tries to address that.
Just like you created Amock
by implementing Ainterface
, you can create a child class that extends A
.
So you would have a class like class AmockClassBased extends A
and then you can override method()
to make it do what you were doing in Amock
class's method()
.
So your Amock
will be changed to:-
public class AmockClassBased extends A {
@Override
public String method() {
//do something
}
}
Then you can pass an instance of this class to B
's method(A a)
.
@m.antkowicz's answer shows the correct way of doing it, by using a mocking framework. In the comments you asked for a way without using external framework, so this answer tries to address that.
Just like you created Amock
by implementing Ainterface
, you can create a child class that extends A
.
So you would have a class like class AmockClassBased extends A
and then you can override method()
to make it do what you were doing in Amock
class's method()
.
So your Amock
will be changed to:-
public class AmockClassBased extends A {
@Override
public String method() {
//do something
}
}
Then you can pass an instance of this class to B
's method(A a)
.
answered Nov 22 at 23:21


Kartik
2,51731331
2,51731331
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53438731%2fhow-to-mock-class-in-java-without-creating-interface%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
LCJEr,iMYqcASACVT9jWONm7ayB,VB6d375Mxk,FV1 dXbKD