connect java to mysql using jdbc on osx
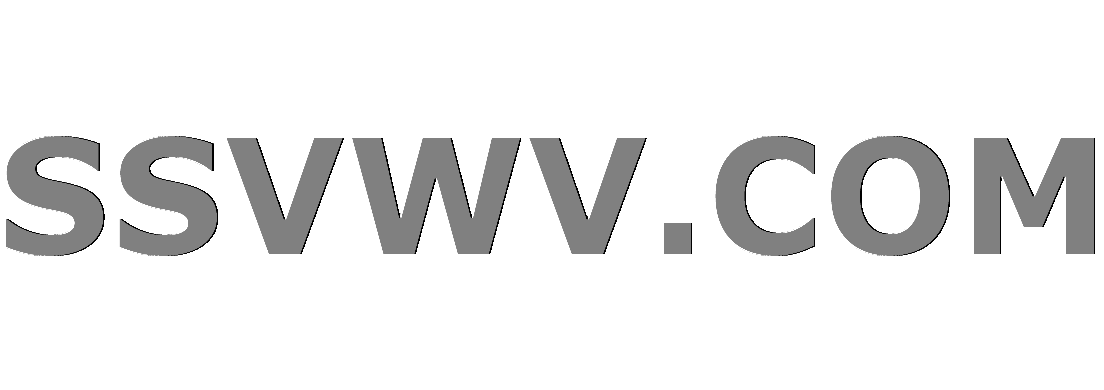
Multi tool use
So I added the MySQL Connector/J 5.1.16 to my project's Build Path. I'm using the default OSX Java package and MAMP Pro 1.9.4 with MySQL 5.1.44 and Eclipse.
I've set up a simple java app with the following function:
private static String dbUrl = "jdbc:mysql://127.0.0.1:3306/mpp";
private static String dbUsername = "root";
private static String dbPassword = "root";
private Statement statement = null;
private void dbConnect() {
try {
Class.forName("com.mysql.jdbc.Driver");
Connection connection = DriverManager.getConnection(dbUrl, dbUsername, dbPassword);
statement = connection.createStatement();
} catch(SQLException e) {
System.err.print(e.getMessage() + " ARGH!");
} catch(Exception e) {
System.err.print(e.getMessage() + " FUUUUUUUUUU!");
}
}
When I run it I get the following error:
Communications link failure
The last packet sent successfully to the server was 0 milliseconds ago. The driver has not received any packets from the server. ARGH!
I googled and searched for about an hour with no success. Any ideas on how to fix this? The JDBC driver should be fine, I kinda tested it.
EDIT
I tried running this through the console
SnowCave:src stefanschipor$ java -cp $CLASSPATH test
I get the same output as above
java mysql jdbc mamp
|
show 1 more comment
So I added the MySQL Connector/J 5.1.16 to my project's Build Path. I'm using the default OSX Java package and MAMP Pro 1.9.4 with MySQL 5.1.44 and Eclipse.
I've set up a simple java app with the following function:
private static String dbUrl = "jdbc:mysql://127.0.0.1:3306/mpp";
private static String dbUsername = "root";
private static String dbPassword = "root";
private Statement statement = null;
private void dbConnect() {
try {
Class.forName("com.mysql.jdbc.Driver");
Connection connection = DriverManager.getConnection(dbUrl, dbUsername, dbPassword);
statement = connection.createStatement();
} catch(SQLException e) {
System.err.print(e.getMessage() + " ARGH!");
} catch(Exception e) {
System.err.print(e.getMessage() + " FUUUUUUUUUU!");
}
}
When I run it I get the following error:
Communications link failure
The last packet sent successfully to the server was 0 milliseconds ago. The driver has not received any packets from the server. ARGH!
I googled and searched for about an hour with no success. Any ideas on how to fix this? The JDBC driver should be fine, I kinda tested it.
EDIT
I tried running this through the console
SnowCave:src stefanschipor$ java -cp $CLASSPATH test
I get the same output as above
java mysql jdbc mamp
Maybe a firewall problem. Is dbUrl correct? Can you connect to MySQL by some client console?
– Farshid Zaker
Jun 4 '11 at 16:14
in your case defaut port localhost:3306
– mKorbel
Jun 4 '11 at 16:18
btw nice error messages:P
– RMT
Jun 4 '11 at 16:19
@Farshid updated the post, same thing :)
– GreenDude
Jun 4 '11 at 16:55
No, I mean connecting to MySQL instance without your code.
– Farshid Zaker
Jun 4 '11 at 16:57
|
show 1 more comment
So I added the MySQL Connector/J 5.1.16 to my project's Build Path. I'm using the default OSX Java package and MAMP Pro 1.9.4 with MySQL 5.1.44 and Eclipse.
I've set up a simple java app with the following function:
private static String dbUrl = "jdbc:mysql://127.0.0.1:3306/mpp";
private static String dbUsername = "root";
private static String dbPassword = "root";
private Statement statement = null;
private void dbConnect() {
try {
Class.forName("com.mysql.jdbc.Driver");
Connection connection = DriverManager.getConnection(dbUrl, dbUsername, dbPassword);
statement = connection.createStatement();
} catch(SQLException e) {
System.err.print(e.getMessage() + " ARGH!");
} catch(Exception e) {
System.err.print(e.getMessage() + " FUUUUUUUUUU!");
}
}
When I run it I get the following error:
Communications link failure
The last packet sent successfully to the server was 0 milliseconds ago. The driver has not received any packets from the server. ARGH!
I googled and searched for about an hour with no success. Any ideas on how to fix this? The JDBC driver should be fine, I kinda tested it.
EDIT
I tried running this through the console
SnowCave:src stefanschipor$ java -cp $CLASSPATH test
I get the same output as above
java mysql jdbc mamp
So I added the MySQL Connector/J 5.1.16 to my project's Build Path. I'm using the default OSX Java package and MAMP Pro 1.9.4 with MySQL 5.1.44 and Eclipse.
I've set up a simple java app with the following function:
private static String dbUrl = "jdbc:mysql://127.0.0.1:3306/mpp";
private static String dbUsername = "root";
private static String dbPassword = "root";
private Statement statement = null;
private void dbConnect() {
try {
Class.forName("com.mysql.jdbc.Driver");
Connection connection = DriverManager.getConnection(dbUrl, dbUsername, dbPassword);
statement = connection.createStatement();
} catch(SQLException e) {
System.err.print(e.getMessage() + " ARGH!");
} catch(Exception e) {
System.err.print(e.getMessage() + " FUUUUUUUUUU!");
}
}
When I run it I get the following error:
Communications link failure
The last packet sent successfully to the server was 0 milliseconds ago. The driver has not received any packets from the server. ARGH!
I googled and searched for about an hour with no success. Any ideas on how to fix this? The JDBC driver should be fine, I kinda tested it.
EDIT
I tried running this through the console
SnowCave:src stefanschipor$ java -cp $CLASSPATH test
I get the same output as above
java mysql jdbc mamp
java mysql jdbc mamp
edited Jun 4 '11 at 20:06
jamesallman
31.4k74966
31.4k74966
asked Jun 4 '11 at 16:11
GreenDude
3922414
3922414
Maybe a firewall problem. Is dbUrl correct? Can you connect to MySQL by some client console?
– Farshid Zaker
Jun 4 '11 at 16:14
in your case defaut port localhost:3306
– mKorbel
Jun 4 '11 at 16:18
btw nice error messages:P
– RMT
Jun 4 '11 at 16:19
@Farshid updated the post, same thing :)
– GreenDude
Jun 4 '11 at 16:55
No, I mean connecting to MySQL instance without your code.
– Farshid Zaker
Jun 4 '11 at 16:57
|
show 1 more comment
Maybe a firewall problem. Is dbUrl correct? Can you connect to MySQL by some client console?
– Farshid Zaker
Jun 4 '11 at 16:14
in your case defaut port localhost:3306
– mKorbel
Jun 4 '11 at 16:18
btw nice error messages:P
– RMT
Jun 4 '11 at 16:19
@Farshid updated the post, same thing :)
– GreenDude
Jun 4 '11 at 16:55
No, I mean connecting to MySQL instance without your code.
– Farshid Zaker
Jun 4 '11 at 16:57
Maybe a firewall problem. Is dbUrl correct? Can you connect to MySQL by some client console?
– Farshid Zaker
Jun 4 '11 at 16:14
Maybe a firewall problem. Is dbUrl correct? Can you connect to MySQL by some client console?
– Farshid Zaker
Jun 4 '11 at 16:14
in your case defaut port localhost:3306
– mKorbel
Jun 4 '11 at 16:18
in your case defaut port localhost:3306
– mKorbel
Jun 4 '11 at 16:18
btw nice error messages:P
– RMT
Jun 4 '11 at 16:19
btw nice error messages:P
– RMT
Jun 4 '11 at 16:19
@Farshid updated the post, same thing :)
– GreenDude
Jun 4 '11 at 16:55
@Farshid updated the post, same thing :)
– GreenDude
Jun 4 '11 at 16:55
No, I mean connecting to MySQL instance without your code.
– Farshid Zaker
Jun 4 '11 at 16:57
No, I mean connecting to MySQL instance without your code.
– Farshid Zaker
Jun 4 '11 at 16:57
|
show 1 more comment
5 Answers
5
active
oldest
votes
OK, this is just silly. :)
You have open up MAMP, go to Server > MySQL and uncheck "Allow local access only" which is checked by default. This is weird since what I'm doing is local, but anyways...
My program seems to work and the commands suggested by @JamesA also yield the expected output!
Huzzah!
This worked for me. Thanks for posting the solution!
– Ian
Dec 15 '13 at 3:57
1
It could be you are using the wrong port in the JDBC connection. For example, if you are using MAMP, the port could be 8889 instead. This is the exact problem I had, hope it's helpful.
– RHE
May 13 '14 at 14:10
1
On MAMP PRO 3.3, you have to check "Allow network access to MySql" and choose "only from this Mac".
– Emir Memic
Jan 17 '16 at 4:41
add a comment |
Are you sure MySQL is running on port 3306?
If the mysql daemon is listening on port 3306 lsof -i :3306
should return:
COMMAND PID USER FD TYPE DEVICE SIZE/OFF NODE NAME
mysqld 7616 username 10u IPv6 0x1fbf6940 0t0 TCP *:mysql (LISTEN)
A direct connect to the port using nc localhost 3306
should return:
H
5.5.969]G.Mw4??9cfUY?k!^:D&mysql_native_password
where 5.5.969
is the mysql version number.
You could also try a tool like DbVisualizer to test your connection URL.
I had the Firewall turned off, but I enabled it and allowed Java anyway. Still the same :)
– GreenDude
Jun 4 '11 at 16:20
I ran the command in Terminal but I got no output. However, the port should be fine, here's a screenshot of my MAMP config grab.by/ahFv
– GreenDude
Jun 4 '11 at 18:35
What's your output fromlsof -i :3306
?
– jamesallman
Jun 4 '11 at 18:48
No output this time either
– GreenDude
Jun 4 '11 at 19:51
That means MySQL's not listening on port 3306. Check the error log at/Applications/MAMP/logs/mysql_error_log.err
– jamesallman
Jun 4 '11 at 19:58
|
show 4 more comments
you need to use the Port localhost:8889 or whatever port you find in MAMP > Settings > Ports > MySQL-Port.
Then your Connection will succeed!
add a comment |
I also have this mysterious error on OSX and didn't found any solution the first time the error occurred. In my cases it occurs if the database structure was modified or tables were dropped/created during development (MySQL as a Datasource for JBoss). In all cases I can avoid the error if I shut down MySQL after modifications and restart it before JBoss starts.
Restarted MAMP, still no luck :)
– GreenDude
Jun 4 '11 at 16:20
add a comment |
Its just because your mysql
service is not running... just run the mysql
service from system preferences or if you have installed it using brew
run brew services start mysql
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f6237860%2fconnect-java-to-mysql-using-jdbc-on-osx%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
5 Answers
5
active
oldest
votes
5 Answers
5
active
oldest
votes
active
oldest
votes
active
oldest
votes
OK, this is just silly. :)
You have open up MAMP, go to Server > MySQL and uncheck "Allow local access only" which is checked by default. This is weird since what I'm doing is local, but anyways...
My program seems to work and the commands suggested by @JamesA also yield the expected output!
Huzzah!
This worked for me. Thanks for posting the solution!
– Ian
Dec 15 '13 at 3:57
1
It could be you are using the wrong port in the JDBC connection. For example, if you are using MAMP, the port could be 8889 instead. This is the exact problem I had, hope it's helpful.
– RHE
May 13 '14 at 14:10
1
On MAMP PRO 3.3, you have to check "Allow network access to MySql" and choose "only from this Mac".
– Emir Memic
Jan 17 '16 at 4:41
add a comment |
OK, this is just silly. :)
You have open up MAMP, go to Server > MySQL and uncheck "Allow local access only" which is checked by default. This is weird since what I'm doing is local, but anyways...
My program seems to work and the commands suggested by @JamesA also yield the expected output!
Huzzah!
This worked for me. Thanks for posting the solution!
– Ian
Dec 15 '13 at 3:57
1
It could be you are using the wrong port in the JDBC connection. For example, if you are using MAMP, the port could be 8889 instead. This is the exact problem I had, hope it's helpful.
– RHE
May 13 '14 at 14:10
1
On MAMP PRO 3.3, you have to check "Allow network access to MySql" and choose "only from this Mac".
– Emir Memic
Jan 17 '16 at 4:41
add a comment |
OK, this is just silly. :)
You have open up MAMP, go to Server > MySQL and uncheck "Allow local access only" which is checked by default. This is weird since what I'm doing is local, but anyways...
My program seems to work and the commands suggested by @JamesA also yield the expected output!
Huzzah!
OK, this is just silly. :)
You have open up MAMP, go to Server > MySQL and uncheck "Allow local access only" which is checked by default. This is weird since what I'm doing is local, but anyways...
My program seems to work and the commands suggested by @JamesA also yield the expected output!
Huzzah!
answered Jun 6 '11 at 1:58
GreenDude
3922414
3922414
This worked for me. Thanks for posting the solution!
– Ian
Dec 15 '13 at 3:57
1
It could be you are using the wrong port in the JDBC connection. For example, if you are using MAMP, the port could be 8889 instead. This is the exact problem I had, hope it's helpful.
– RHE
May 13 '14 at 14:10
1
On MAMP PRO 3.3, you have to check "Allow network access to MySql" and choose "only from this Mac".
– Emir Memic
Jan 17 '16 at 4:41
add a comment |
This worked for me. Thanks for posting the solution!
– Ian
Dec 15 '13 at 3:57
1
It could be you are using the wrong port in the JDBC connection. For example, if you are using MAMP, the port could be 8889 instead. This is the exact problem I had, hope it's helpful.
– RHE
May 13 '14 at 14:10
1
On MAMP PRO 3.3, you have to check "Allow network access to MySql" and choose "only from this Mac".
– Emir Memic
Jan 17 '16 at 4:41
This worked for me. Thanks for posting the solution!
– Ian
Dec 15 '13 at 3:57
This worked for me. Thanks for posting the solution!
– Ian
Dec 15 '13 at 3:57
1
1
It could be you are using the wrong port in the JDBC connection. For example, if you are using MAMP, the port could be 8889 instead. This is the exact problem I had, hope it's helpful.
– RHE
May 13 '14 at 14:10
It could be you are using the wrong port in the JDBC connection. For example, if you are using MAMP, the port could be 8889 instead. This is the exact problem I had, hope it's helpful.
– RHE
May 13 '14 at 14:10
1
1
On MAMP PRO 3.3, you have to check "Allow network access to MySql" and choose "only from this Mac".
– Emir Memic
Jan 17 '16 at 4:41
On MAMP PRO 3.3, you have to check "Allow network access to MySql" and choose "only from this Mac".
– Emir Memic
Jan 17 '16 at 4:41
add a comment |
Are you sure MySQL is running on port 3306?
If the mysql daemon is listening on port 3306 lsof -i :3306
should return:
COMMAND PID USER FD TYPE DEVICE SIZE/OFF NODE NAME
mysqld 7616 username 10u IPv6 0x1fbf6940 0t0 TCP *:mysql (LISTEN)
A direct connect to the port using nc localhost 3306
should return:
H
5.5.969]G.Mw4??9cfUY?k!^:D&mysql_native_password
where 5.5.969
is the mysql version number.
You could also try a tool like DbVisualizer to test your connection URL.
I had the Firewall turned off, but I enabled it and allowed Java anyway. Still the same :)
– GreenDude
Jun 4 '11 at 16:20
I ran the command in Terminal but I got no output. However, the port should be fine, here's a screenshot of my MAMP config grab.by/ahFv
– GreenDude
Jun 4 '11 at 18:35
What's your output fromlsof -i :3306
?
– jamesallman
Jun 4 '11 at 18:48
No output this time either
– GreenDude
Jun 4 '11 at 19:51
That means MySQL's not listening on port 3306. Check the error log at/Applications/MAMP/logs/mysql_error_log.err
– jamesallman
Jun 4 '11 at 19:58
|
show 4 more comments
Are you sure MySQL is running on port 3306?
If the mysql daemon is listening on port 3306 lsof -i :3306
should return:
COMMAND PID USER FD TYPE DEVICE SIZE/OFF NODE NAME
mysqld 7616 username 10u IPv6 0x1fbf6940 0t0 TCP *:mysql (LISTEN)
A direct connect to the port using nc localhost 3306
should return:
H
5.5.969]G.Mw4??9cfUY?k!^:D&mysql_native_password
where 5.5.969
is the mysql version number.
You could also try a tool like DbVisualizer to test your connection URL.
I had the Firewall turned off, but I enabled it and allowed Java anyway. Still the same :)
– GreenDude
Jun 4 '11 at 16:20
I ran the command in Terminal but I got no output. However, the port should be fine, here's a screenshot of my MAMP config grab.by/ahFv
– GreenDude
Jun 4 '11 at 18:35
What's your output fromlsof -i :3306
?
– jamesallman
Jun 4 '11 at 18:48
No output this time either
– GreenDude
Jun 4 '11 at 19:51
That means MySQL's not listening on port 3306. Check the error log at/Applications/MAMP/logs/mysql_error_log.err
– jamesallman
Jun 4 '11 at 19:58
|
show 4 more comments
Are you sure MySQL is running on port 3306?
If the mysql daemon is listening on port 3306 lsof -i :3306
should return:
COMMAND PID USER FD TYPE DEVICE SIZE/OFF NODE NAME
mysqld 7616 username 10u IPv6 0x1fbf6940 0t0 TCP *:mysql (LISTEN)
A direct connect to the port using nc localhost 3306
should return:
H
5.5.969]G.Mw4??9cfUY?k!^:D&mysql_native_password
where 5.5.969
is the mysql version number.
You could also try a tool like DbVisualizer to test your connection URL.
Are you sure MySQL is running on port 3306?
If the mysql daemon is listening on port 3306 lsof -i :3306
should return:
COMMAND PID USER FD TYPE DEVICE SIZE/OFF NODE NAME
mysqld 7616 username 10u IPv6 0x1fbf6940 0t0 TCP *:mysql (LISTEN)
A direct connect to the port using nc localhost 3306
should return:
H
5.5.969]G.Mw4??9cfUY?k!^:D&mysql_native_password
where 5.5.969
is the mysql version number.
You could also try a tool like DbVisualizer to test your connection URL.
edited Jun 4 '11 at 21:06
answered Jun 4 '11 at 16:14
jamesallman
31.4k74966
31.4k74966
I had the Firewall turned off, but I enabled it and allowed Java anyway. Still the same :)
– GreenDude
Jun 4 '11 at 16:20
I ran the command in Terminal but I got no output. However, the port should be fine, here's a screenshot of my MAMP config grab.by/ahFv
– GreenDude
Jun 4 '11 at 18:35
What's your output fromlsof -i :3306
?
– jamesallman
Jun 4 '11 at 18:48
No output this time either
– GreenDude
Jun 4 '11 at 19:51
That means MySQL's not listening on port 3306. Check the error log at/Applications/MAMP/logs/mysql_error_log.err
– jamesallman
Jun 4 '11 at 19:58
|
show 4 more comments
I had the Firewall turned off, but I enabled it and allowed Java anyway. Still the same :)
– GreenDude
Jun 4 '11 at 16:20
I ran the command in Terminal but I got no output. However, the port should be fine, here's a screenshot of my MAMP config grab.by/ahFv
– GreenDude
Jun 4 '11 at 18:35
What's your output fromlsof -i :3306
?
– jamesallman
Jun 4 '11 at 18:48
No output this time either
– GreenDude
Jun 4 '11 at 19:51
That means MySQL's not listening on port 3306. Check the error log at/Applications/MAMP/logs/mysql_error_log.err
– jamesallman
Jun 4 '11 at 19:58
I had the Firewall turned off, but I enabled it and allowed Java anyway. Still the same :)
– GreenDude
Jun 4 '11 at 16:20
I had the Firewall turned off, but I enabled it and allowed Java anyway. Still the same :)
– GreenDude
Jun 4 '11 at 16:20
I ran the command in Terminal but I got no output. However, the port should be fine, here's a screenshot of my MAMP config grab.by/ahFv
– GreenDude
Jun 4 '11 at 18:35
I ran the command in Terminal but I got no output. However, the port should be fine, here's a screenshot of my MAMP config grab.by/ahFv
– GreenDude
Jun 4 '11 at 18:35
What's your output from
lsof -i :3306
?– jamesallman
Jun 4 '11 at 18:48
What's your output from
lsof -i :3306
?– jamesallman
Jun 4 '11 at 18:48
No output this time either
– GreenDude
Jun 4 '11 at 19:51
No output this time either
– GreenDude
Jun 4 '11 at 19:51
That means MySQL's not listening on port 3306. Check the error log at
/Applications/MAMP/logs/mysql_error_log.err
– jamesallman
Jun 4 '11 at 19:58
That means MySQL's not listening on port 3306. Check the error log at
/Applications/MAMP/logs/mysql_error_log.err
– jamesallman
Jun 4 '11 at 19:58
|
show 4 more comments
you need to use the Port localhost:8889 or whatever port you find in MAMP > Settings > Ports > MySQL-Port.
Then your Connection will succeed!
add a comment |
you need to use the Port localhost:8889 or whatever port you find in MAMP > Settings > Ports > MySQL-Port.
Then your Connection will succeed!
add a comment |
you need to use the Port localhost:8889 or whatever port you find in MAMP > Settings > Ports > MySQL-Port.
Then your Connection will succeed!
you need to use the Port localhost:8889 or whatever port you find in MAMP > Settings > Ports > MySQL-Port.
Then your Connection will succeed!
answered Nov 22 '17 at 10:37
heinrich.osudio
111
111
add a comment |
add a comment |
I also have this mysterious error on OSX and didn't found any solution the first time the error occurred. In my cases it occurs if the database structure was modified or tables were dropped/created during development (MySQL as a Datasource for JBoss). In all cases I can avoid the error if I shut down MySQL after modifications and restart it before JBoss starts.
Restarted MAMP, still no luck :)
– GreenDude
Jun 4 '11 at 16:20
add a comment |
I also have this mysterious error on OSX and didn't found any solution the first time the error occurred. In my cases it occurs if the database structure was modified or tables were dropped/created during development (MySQL as a Datasource for JBoss). In all cases I can avoid the error if I shut down MySQL after modifications and restart it before JBoss starts.
Restarted MAMP, still no luck :)
– GreenDude
Jun 4 '11 at 16:20
add a comment |
I also have this mysterious error on OSX and didn't found any solution the first time the error occurred. In my cases it occurs if the database structure was modified or tables were dropped/created during development (MySQL as a Datasource for JBoss). In all cases I can avoid the error if I shut down MySQL after modifications and restart it before JBoss starts.
I also have this mysterious error on OSX and didn't found any solution the first time the error occurred. In my cases it occurs if the database structure was modified or tables were dropped/created during development (MySQL as a Datasource for JBoss). In all cases I can avoid the error if I shut down MySQL after modifications and restart it before JBoss starts.
answered Jun 4 '11 at 16:15
Thor
4,231125390
4,231125390
Restarted MAMP, still no luck :)
– GreenDude
Jun 4 '11 at 16:20
add a comment |
Restarted MAMP, still no luck :)
– GreenDude
Jun 4 '11 at 16:20
Restarted MAMP, still no luck :)
– GreenDude
Jun 4 '11 at 16:20
Restarted MAMP, still no luck :)
– GreenDude
Jun 4 '11 at 16:20
add a comment |
Its just because your mysql
service is not running... just run the mysql
service from system preferences or if you have installed it using brew
run brew services start mysql
add a comment |
Its just because your mysql
service is not running... just run the mysql
service from system preferences or if you have installed it using brew
run brew services start mysql
add a comment |
Its just because your mysql
service is not running... just run the mysql
service from system preferences or if you have installed it using brew
run brew services start mysql
Its just because your mysql
service is not running... just run the mysql
service from system preferences or if you have installed it using brew
run brew services start mysql
edited Nov 22 at 21:20


komron
1,0871923
1,0871923
answered Nov 22 at 18:23


Neeraj Pandey
11
11
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f6237860%2fconnect-java-to-mysql-using-jdbc-on-osx%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Kj,Cjn6X0mET LmjHUkQkLv,i cJD6
Maybe a firewall problem. Is dbUrl correct? Can you connect to MySQL by some client console?
– Farshid Zaker
Jun 4 '11 at 16:14
in your case defaut port localhost:3306
– mKorbel
Jun 4 '11 at 16:18
btw nice error messages:P
– RMT
Jun 4 '11 at 16:19
@Farshid updated the post, same thing :)
– GreenDude
Jun 4 '11 at 16:55
No, I mean connecting to MySQL instance without your code.
– Farshid Zaker
Jun 4 '11 at 16:57