Match for today's date doesn't match correctly
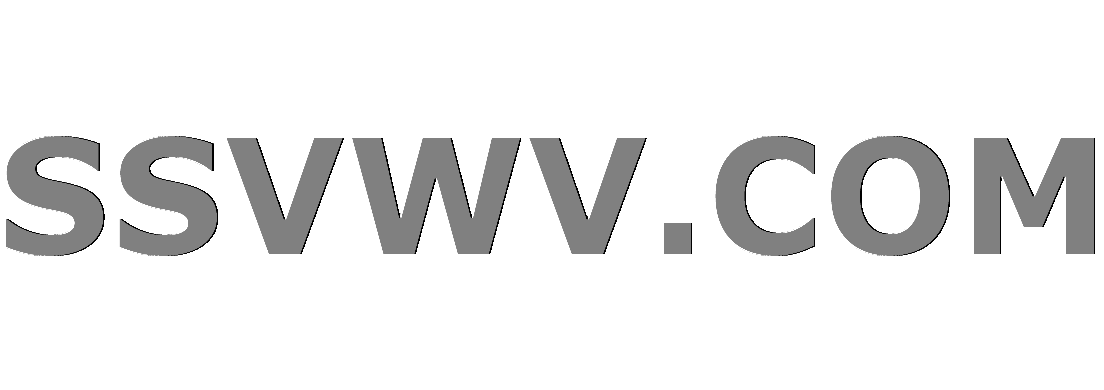
Multi tool use
up vote
0
down vote
favorite
i'm writing a basic Perl script to check if there're files in certain directories their names contain today's date, the script works fine when there are files in the directory and returns TRUE, but doesn't return FALSE when no files withe the given criteria exist
the script is as below:
#!/usr/bin/perl -w
use POSIX qw(strftime);
my $datestring = strftime "%Y%m%d", localtime;
opendir(DIR, 'C:Perl');
@files = grep (/$datestring/,readdir(DIR));
closedir(DIR);
foreach $file (@files) {
my $count = () = $file =~ /$datestring/;
if ($count > 0) {
print ("TRUE");
}
else
{
print ("FALSE");
}
}
the files names are like
export_opportunities_20181111-040005_20181124-040010.csv
export_opportunities_20181111-040005_20181122-040010.csv
perl date
add a comment |
up vote
0
down vote
favorite
i'm writing a basic Perl script to check if there're files in certain directories their names contain today's date, the script works fine when there are files in the directory and returns TRUE, but doesn't return FALSE when no files withe the given criteria exist
the script is as below:
#!/usr/bin/perl -w
use POSIX qw(strftime);
my $datestring = strftime "%Y%m%d", localtime;
opendir(DIR, 'C:Perl');
@files = grep (/$datestring/,readdir(DIR));
closedir(DIR);
foreach $file (@files) {
my $count = () = $file =~ /$datestring/;
if ($count > 0) {
print ("TRUE");
}
else
{
print ("FALSE");
}
}
the files names are like
export_opportunities_20181111-040005_20181124-040010.csv
export_opportunities_20181111-040005_20181122-040010.csv
perl date
2
All files meet the criteria because you already filtered them withgrep
.
– toolic
Nov 22 at 16:13
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
i'm writing a basic Perl script to check if there're files in certain directories their names contain today's date, the script works fine when there are files in the directory and returns TRUE, but doesn't return FALSE when no files withe the given criteria exist
the script is as below:
#!/usr/bin/perl -w
use POSIX qw(strftime);
my $datestring = strftime "%Y%m%d", localtime;
opendir(DIR, 'C:Perl');
@files = grep (/$datestring/,readdir(DIR));
closedir(DIR);
foreach $file (@files) {
my $count = () = $file =~ /$datestring/;
if ($count > 0) {
print ("TRUE");
}
else
{
print ("FALSE");
}
}
the files names are like
export_opportunities_20181111-040005_20181124-040010.csv
export_opportunities_20181111-040005_20181122-040010.csv
perl date
i'm writing a basic Perl script to check if there're files in certain directories their names contain today's date, the script works fine when there are files in the directory and returns TRUE, but doesn't return FALSE when no files withe the given criteria exist
the script is as below:
#!/usr/bin/perl -w
use POSIX qw(strftime);
my $datestring = strftime "%Y%m%d", localtime;
opendir(DIR, 'C:Perl');
@files = grep (/$datestring/,readdir(DIR));
closedir(DIR);
foreach $file (@files) {
my $count = () = $file =~ /$datestring/;
if ($count > 0) {
print ("TRUE");
}
else
{
print ("FALSE");
}
}
the files names are like
export_opportunities_20181111-040005_20181124-040010.csv
export_opportunities_20181111-040005_20181122-040010.csv
perl date
perl date
edited Nov 22 at 16:19
choroba
153k14139201
153k14139201
asked Nov 22 at 16:10
Kareem Hamed
1114
1114
2
All files meet the criteria because you already filtered them withgrep
.
– toolic
Nov 22 at 16:13
add a comment |
2
All files meet the criteria because you already filtered them withgrep
.
– toolic
Nov 22 at 16:13
2
2
All files meet the criteria because you already filtered them with
grep
.– toolic
Nov 22 at 16:13
All files meet the criteria because you already filtered them with
grep
.– toolic
Nov 22 at 16:13
add a comment |
2 Answers
2
active
oldest
votes
up vote
1
down vote
You can use @files
in scalar context to tell if grep
returned any matches from readdir
. In scalar context, @files
is the number of elements in the array.
@files = grep (/$datestring/,readdir(DIR));
# this is more commonly written
# @files = grep {/$datestring/} readdir(DIR);
print @files > 0 ? "TRUE" : "FALSE";
# or
print scalar(@files)." files matched $datestring";
See also List::Util.
use v5.10;
use List::Util qw<all any none>;
###
@files = readdir(DIR);
say 'all match' if all {/$datestring/} @files;
say 'at least one match' if any {/$datestring/} @files;
say 'no matches' if none {/$datestring/} @files;
add a comment |
up vote
1
down vote
As commented by toolic, when you are already removing non-matching files here :
@files = grep (/$datestring/,readdir(DIR));
Hence your for
loop will never see non-matching files.
Here are a few other comments on your code :
always
use strict
anduse warnings
(there are several variables in your code snippet which are not properly declared)always check the return code of system calls such as
opendir
you can use the smartmatch operator in boolean context instead of assigning to the
$count
variableyou don't need to use parentheses around argument to the built-in
print
function
Here is a cleaner version of your code :
#!/usr/bin/perl -w
use strict;
use warnings;
use POSIX qw(strftime);
my $datestring = strftime "%Y%m%d", localtime;
opendir(DIR, 'C:Perl') or die "cannot open dir : $!";
my @files = readdir(DIR);
closedir(DIR);
foreach my $file (@files) {
if ($file =~ /$datestring/) {
print "TRUEn";
} else {
print "FALSEn";
}
}
Thanks fort the feedback, the output really matches for the result but it returns many trues along with each file name, i need only one true if there's one file match and one false if no file matches
– Kareem Hamed
Nov 23 at 11:38
@Kareem ok I edited the answer to produce the desired output
– GMB
Nov 24 at 9:52
add a comment |
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
You can use @files
in scalar context to tell if grep
returned any matches from readdir
. In scalar context, @files
is the number of elements in the array.
@files = grep (/$datestring/,readdir(DIR));
# this is more commonly written
# @files = grep {/$datestring/} readdir(DIR);
print @files > 0 ? "TRUE" : "FALSE";
# or
print scalar(@files)." files matched $datestring";
See also List::Util.
use v5.10;
use List::Util qw<all any none>;
###
@files = readdir(DIR);
say 'all match' if all {/$datestring/} @files;
say 'at least one match' if any {/$datestring/} @files;
say 'no matches' if none {/$datestring/} @files;
add a comment |
up vote
1
down vote
You can use @files
in scalar context to tell if grep
returned any matches from readdir
. In scalar context, @files
is the number of elements in the array.
@files = grep (/$datestring/,readdir(DIR));
# this is more commonly written
# @files = grep {/$datestring/} readdir(DIR);
print @files > 0 ? "TRUE" : "FALSE";
# or
print scalar(@files)." files matched $datestring";
See also List::Util.
use v5.10;
use List::Util qw<all any none>;
###
@files = readdir(DIR);
say 'all match' if all {/$datestring/} @files;
say 'at least one match' if any {/$datestring/} @files;
say 'no matches' if none {/$datestring/} @files;
add a comment |
up vote
1
down vote
up vote
1
down vote
You can use @files
in scalar context to tell if grep
returned any matches from readdir
. In scalar context, @files
is the number of elements in the array.
@files = grep (/$datestring/,readdir(DIR));
# this is more commonly written
# @files = grep {/$datestring/} readdir(DIR);
print @files > 0 ? "TRUE" : "FALSE";
# or
print scalar(@files)." files matched $datestring";
See also List::Util.
use v5.10;
use List::Util qw<all any none>;
###
@files = readdir(DIR);
say 'all match' if all {/$datestring/} @files;
say 'at least one match' if any {/$datestring/} @files;
say 'no matches' if none {/$datestring/} @files;
You can use @files
in scalar context to tell if grep
returned any matches from readdir
. In scalar context, @files
is the number of elements in the array.
@files = grep (/$datestring/,readdir(DIR));
# this is more commonly written
# @files = grep {/$datestring/} readdir(DIR);
print @files > 0 ? "TRUE" : "FALSE";
# or
print scalar(@files)." files matched $datestring";
See also List::Util.
use v5.10;
use List::Util qw<all any none>;
###
@files = readdir(DIR);
say 'all match' if all {/$datestring/} @files;
say 'at least one match' if any {/$datestring/} @files;
say 'no matches' if none {/$datestring/} @files;
answered Nov 23 at 18:02


beasy
923411
923411
add a comment |
add a comment |
up vote
1
down vote
As commented by toolic, when you are already removing non-matching files here :
@files = grep (/$datestring/,readdir(DIR));
Hence your for
loop will never see non-matching files.
Here are a few other comments on your code :
always
use strict
anduse warnings
(there are several variables in your code snippet which are not properly declared)always check the return code of system calls such as
opendir
you can use the smartmatch operator in boolean context instead of assigning to the
$count
variableyou don't need to use parentheses around argument to the built-in
print
function
Here is a cleaner version of your code :
#!/usr/bin/perl -w
use strict;
use warnings;
use POSIX qw(strftime);
my $datestring = strftime "%Y%m%d", localtime;
opendir(DIR, 'C:Perl') or die "cannot open dir : $!";
my @files = readdir(DIR);
closedir(DIR);
foreach my $file (@files) {
if ($file =~ /$datestring/) {
print "TRUEn";
} else {
print "FALSEn";
}
}
Thanks fort the feedback, the output really matches for the result but it returns many trues along with each file name, i need only one true if there's one file match and one false if no file matches
– Kareem Hamed
Nov 23 at 11:38
@Kareem ok I edited the answer to produce the desired output
– GMB
Nov 24 at 9:52
add a comment |
up vote
1
down vote
As commented by toolic, when you are already removing non-matching files here :
@files = grep (/$datestring/,readdir(DIR));
Hence your for
loop will never see non-matching files.
Here are a few other comments on your code :
always
use strict
anduse warnings
(there are several variables in your code snippet which are not properly declared)always check the return code of system calls such as
opendir
you can use the smartmatch operator in boolean context instead of assigning to the
$count
variableyou don't need to use parentheses around argument to the built-in
print
function
Here is a cleaner version of your code :
#!/usr/bin/perl -w
use strict;
use warnings;
use POSIX qw(strftime);
my $datestring = strftime "%Y%m%d", localtime;
opendir(DIR, 'C:Perl') or die "cannot open dir : $!";
my @files = readdir(DIR);
closedir(DIR);
foreach my $file (@files) {
if ($file =~ /$datestring/) {
print "TRUEn";
} else {
print "FALSEn";
}
}
Thanks fort the feedback, the output really matches for the result but it returns many trues along with each file name, i need only one true if there's one file match and one false if no file matches
– Kareem Hamed
Nov 23 at 11:38
@Kareem ok I edited the answer to produce the desired output
– GMB
Nov 24 at 9:52
add a comment |
up vote
1
down vote
up vote
1
down vote
As commented by toolic, when you are already removing non-matching files here :
@files = grep (/$datestring/,readdir(DIR));
Hence your for
loop will never see non-matching files.
Here are a few other comments on your code :
always
use strict
anduse warnings
(there are several variables in your code snippet which are not properly declared)always check the return code of system calls such as
opendir
you can use the smartmatch operator in boolean context instead of assigning to the
$count
variableyou don't need to use parentheses around argument to the built-in
print
function
Here is a cleaner version of your code :
#!/usr/bin/perl -w
use strict;
use warnings;
use POSIX qw(strftime);
my $datestring = strftime "%Y%m%d", localtime;
opendir(DIR, 'C:Perl') or die "cannot open dir : $!";
my @files = readdir(DIR);
closedir(DIR);
foreach my $file (@files) {
if ($file =~ /$datestring/) {
print "TRUEn";
} else {
print "FALSEn";
}
}
As commented by toolic, when you are already removing non-matching files here :
@files = grep (/$datestring/,readdir(DIR));
Hence your for
loop will never see non-matching files.
Here are a few other comments on your code :
always
use strict
anduse warnings
(there are several variables in your code snippet which are not properly declared)always check the return code of system calls such as
opendir
you can use the smartmatch operator in boolean context instead of assigning to the
$count
variableyou don't need to use parentheses around argument to the built-in
print
function
Here is a cleaner version of your code :
#!/usr/bin/perl -w
use strict;
use warnings;
use POSIX qw(strftime);
my $datestring = strftime "%Y%m%d", localtime;
opendir(DIR, 'C:Perl') or die "cannot open dir : $!";
my @files = readdir(DIR);
closedir(DIR);
foreach my $file (@files) {
if ($file =~ /$datestring/) {
print "TRUEn";
} else {
print "FALSEn";
}
}
edited Nov 24 at 9:51
answered Nov 22 at 21:40


GMB
2,028114
2,028114
Thanks fort the feedback, the output really matches for the result but it returns many trues along with each file name, i need only one true if there's one file match and one false if no file matches
– Kareem Hamed
Nov 23 at 11:38
@Kareem ok I edited the answer to produce the desired output
– GMB
Nov 24 at 9:52
add a comment |
Thanks fort the feedback, the output really matches for the result but it returns many trues along with each file name, i need only one true if there's one file match and one false if no file matches
– Kareem Hamed
Nov 23 at 11:38
@Kareem ok I edited the answer to produce the desired output
– GMB
Nov 24 at 9:52
Thanks fort the feedback, the output really matches for the result but it returns many trues along with each file name, i need only one true if there's one file match and one false if no file matches
– Kareem Hamed
Nov 23 at 11:38
Thanks fort the feedback, the output really matches for the result but it returns many trues along with each file name, i need only one true if there's one file match and one false if no file matches
– Kareem Hamed
Nov 23 at 11:38
@Kareem ok I edited the answer to produce the desired output
– GMB
Nov 24 at 9:52
@Kareem ok I edited the answer to produce the desired output
– GMB
Nov 24 at 9:52
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53434758%2fmatch-for-todays-date-doesnt-match-correctly%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
oP6KAnWx4H4IfLruuDe,67QqTXD9d,AK CTT JrNZN o727oCAb8ba XniqX6mN26WEy,l6 0b1DwRdcUH
2
All files meet the criteria because you already filtered them with
grep
.– toolic
Nov 22 at 16:13