Pyramid is not appearing on the canvas WEB GL
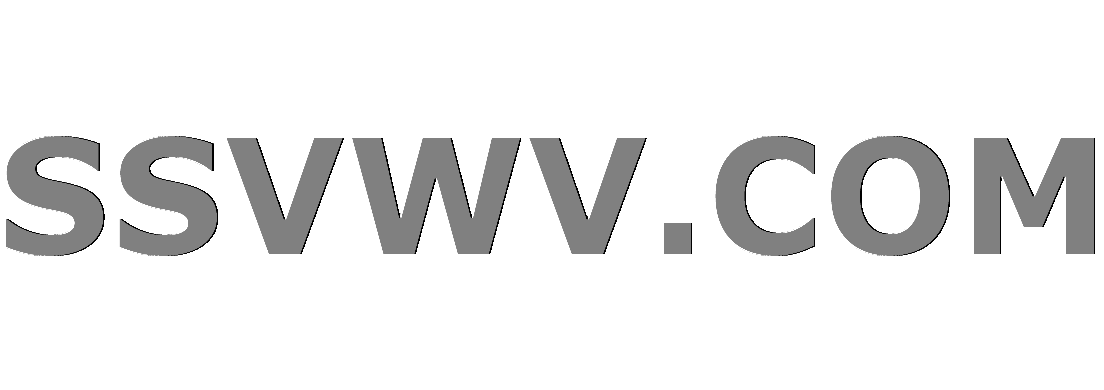
Multi tool use
up vote
1
down vote
favorite
I am trying to create two objects an octagon and a pyramid on the canvas. The octagon is appearing without any problem but the pyramid is not. I am attaching the code of the pyramid.
pyramidPositionBuffer = gl.createBuffer();
gl.bindBuffer(gl.ARRAY_BUFFER, pyramidPositionBuffer);
let pyramidVerts = [
0.0, 0.5, 0.0, //0
-0.5, 0.0, -0.5, //1
0.5, 0.0, -0.5, //2
0.5, 0.0, 0.5, //3
-0.5, 0.0, 0.5 //4
];
pyramidPositionBuffer.itemSize = 3;
pyramidPositionBuffer.numItems = 5;
gl.bufferData(gl.ARRAY_BUFFER, new Float32Array(pyramidVerts), gl.STATIC_DRAW);
pyramidColorBuffer = gl.createBuffer();
gl.bindBuffer(gl.ARRAY_BUFFER, pyramidColorBuffer);
let pyramidColors = [
1.0, 1.0, 1.0, 1.0,
1.0, 0.0, 0.0, 1.0,
1.0, 1.0, 0.0, 1.0,
0.0, 1.0, 0.0, 1.0,
0.0, 0.0, 1.0, 1.0
];
pyramidColorBuffer.itemSize = 4;
pyramidColorBuffer.numItems = 5;
gl.bufferData(gl.ARRAY_BUFFER, new Float32Array(pyramidColorBuffer),gl.STATIC_DRAWS);
pyramidIndexBuffer = gl.createBuffer();
gl.bindBuffer(gl.ELEMENT_ARRAY_BUFFER, pyramidIndexBuffer);
let pyramidIndeces = [
0, 1, 2,
0, 2, 3,
0, 3, 4,
0, 4, 1
];
pyramidIndexBuffer.itemSize = 1;
pyramidIndexBuffer.numItems = 12;
gl.bufferData(gl.ELEMENT_ARRAY_BUFFER, new Uint16Array(pyramidIndeces), gl.STATIC_DRAW);
}
function drawScene() {
gl.viewport(0, 0, gl.viewportWidth, gl.viewportHeight);
gl.clear(gl.COLOR_BUFFER_BIT | gl.DEPTH_BUFFER_BIT);
mat4.perspective(45, gl.viewportWidth / gl.viewportHeight, 0.1, 100.0, pMatrix);
mat4.identity(mvMatrix);
mat4.translate(mvMatrix, [-1.5, 0.0, -6.5]); // We store a translation to our matrix, so when the first object is drawn it will be in that position.
// Connect the attributes with the shaders and draw...
gl.bindBuffer(gl.ARRAY_BUFFER,octagonPositionBuffer);
gl.vertexAttribPointer(shaderProgram.vertexPositionAttribute, octagonPositionBuffer.itemSize, gl.FLOAT, false, 0, 0);
gl.bindBuffer(gl.ARRAY_BUFFER, octagonColorBuffer);
gl.vertexAttribPointer(shaderProgram.vertexColorAttribute, octagonColorBuffer.itemSize, gl.FLOAT, false, 0, 0);
gl.bindBuffer(gl.ELEMENT_ARRAY_BUFFER, octagonIndexBuffer);
setMatrixUniforms();
gl.drawElements(gl.TRIANGLES, octagonIndexBuffer.numItems, gl.UNSIGNED_SHORT, 0);
mat4.translate(mvMatrix, [2.8, 0.0, 0.0]);
gl.bindBuffer(gl.ARRAY_BUFFER,pyramidPositionBuffer);
gl.vertexAttribPointer(shaderProgram.vertexPositionAttribute, pyramidPositionBuffer.itemSize, gl.FLOAT, false, 0, 0);
gl.bindBuffer(gl.ARRAY_BUFFER, pyramidColorBuffer);
gl.vertexAttribPointer(shaderProgram.vertexColorAttribute, pyramidColorBuffer.itemSize, gl.FLOAT, false, 0, 0);
gl.bindBuffer(gl.ELEMENT_ARRAY_BUFFER, pyramidIndexBuffer);
setMatrixUniforms();
gl.drawElements(gl.TRIANGLES, pyramidIndexBuffer.numItems, gl.UNSIGNED_SHORT, 0);
}
I created the pyramid with the same way I created the octagon but for some reason it is not appearing. If I try to draw the octagon two times it has no problem.
javascript html5 canvas webgl
add a comment |
up vote
1
down vote
favorite
I am trying to create two objects an octagon and a pyramid on the canvas. The octagon is appearing without any problem but the pyramid is not. I am attaching the code of the pyramid.
pyramidPositionBuffer = gl.createBuffer();
gl.bindBuffer(gl.ARRAY_BUFFER, pyramidPositionBuffer);
let pyramidVerts = [
0.0, 0.5, 0.0, //0
-0.5, 0.0, -0.5, //1
0.5, 0.0, -0.5, //2
0.5, 0.0, 0.5, //3
-0.5, 0.0, 0.5 //4
];
pyramidPositionBuffer.itemSize = 3;
pyramidPositionBuffer.numItems = 5;
gl.bufferData(gl.ARRAY_BUFFER, new Float32Array(pyramidVerts), gl.STATIC_DRAW);
pyramidColorBuffer = gl.createBuffer();
gl.bindBuffer(gl.ARRAY_BUFFER, pyramidColorBuffer);
let pyramidColors = [
1.0, 1.0, 1.0, 1.0,
1.0, 0.0, 0.0, 1.0,
1.0, 1.0, 0.0, 1.0,
0.0, 1.0, 0.0, 1.0,
0.0, 0.0, 1.0, 1.0
];
pyramidColorBuffer.itemSize = 4;
pyramidColorBuffer.numItems = 5;
gl.bufferData(gl.ARRAY_BUFFER, new Float32Array(pyramidColorBuffer),gl.STATIC_DRAWS);
pyramidIndexBuffer = gl.createBuffer();
gl.bindBuffer(gl.ELEMENT_ARRAY_BUFFER, pyramidIndexBuffer);
let pyramidIndeces = [
0, 1, 2,
0, 2, 3,
0, 3, 4,
0, 4, 1
];
pyramidIndexBuffer.itemSize = 1;
pyramidIndexBuffer.numItems = 12;
gl.bufferData(gl.ELEMENT_ARRAY_BUFFER, new Uint16Array(pyramidIndeces), gl.STATIC_DRAW);
}
function drawScene() {
gl.viewport(0, 0, gl.viewportWidth, gl.viewportHeight);
gl.clear(gl.COLOR_BUFFER_BIT | gl.DEPTH_BUFFER_BIT);
mat4.perspective(45, gl.viewportWidth / gl.viewportHeight, 0.1, 100.0, pMatrix);
mat4.identity(mvMatrix);
mat4.translate(mvMatrix, [-1.5, 0.0, -6.5]); // We store a translation to our matrix, so when the first object is drawn it will be in that position.
// Connect the attributes with the shaders and draw...
gl.bindBuffer(gl.ARRAY_BUFFER,octagonPositionBuffer);
gl.vertexAttribPointer(shaderProgram.vertexPositionAttribute, octagonPositionBuffer.itemSize, gl.FLOAT, false, 0, 0);
gl.bindBuffer(gl.ARRAY_BUFFER, octagonColorBuffer);
gl.vertexAttribPointer(shaderProgram.vertexColorAttribute, octagonColorBuffer.itemSize, gl.FLOAT, false, 0, 0);
gl.bindBuffer(gl.ELEMENT_ARRAY_BUFFER, octagonIndexBuffer);
setMatrixUniforms();
gl.drawElements(gl.TRIANGLES, octagonIndexBuffer.numItems, gl.UNSIGNED_SHORT, 0);
mat4.translate(mvMatrix, [2.8, 0.0, 0.0]);
gl.bindBuffer(gl.ARRAY_BUFFER,pyramidPositionBuffer);
gl.vertexAttribPointer(shaderProgram.vertexPositionAttribute, pyramidPositionBuffer.itemSize, gl.FLOAT, false, 0, 0);
gl.bindBuffer(gl.ARRAY_BUFFER, pyramidColorBuffer);
gl.vertexAttribPointer(shaderProgram.vertexColorAttribute, pyramidColorBuffer.itemSize, gl.FLOAT, false, 0, 0);
gl.bindBuffer(gl.ELEMENT_ARRAY_BUFFER, pyramidIndexBuffer);
setMatrixUniforms();
gl.drawElements(gl.TRIANGLES, pyramidIndexBuffer.numItems, gl.UNSIGNED_SHORT, 0);
}
I created the pyramid with the same way I created the octagon but for some reason it is not appearing. If I try to draw the octagon two times it has no problem.
javascript html5 canvas webgl
If you take some time to learn about the JavaScript console in your browser it would have told you these errors.
– gman
Nov 23 at 1:51
add a comment |
up vote
1
down vote
favorite
up vote
1
down vote
favorite
I am trying to create two objects an octagon and a pyramid on the canvas. The octagon is appearing without any problem but the pyramid is not. I am attaching the code of the pyramid.
pyramidPositionBuffer = gl.createBuffer();
gl.bindBuffer(gl.ARRAY_BUFFER, pyramidPositionBuffer);
let pyramidVerts = [
0.0, 0.5, 0.0, //0
-0.5, 0.0, -0.5, //1
0.5, 0.0, -0.5, //2
0.5, 0.0, 0.5, //3
-0.5, 0.0, 0.5 //4
];
pyramidPositionBuffer.itemSize = 3;
pyramidPositionBuffer.numItems = 5;
gl.bufferData(gl.ARRAY_BUFFER, new Float32Array(pyramidVerts), gl.STATIC_DRAW);
pyramidColorBuffer = gl.createBuffer();
gl.bindBuffer(gl.ARRAY_BUFFER, pyramidColorBuffer);
let pyramidColors = [
1.0, 1.0, 1.0, 1.0,
1.0, 0.0, 0.0, 1.0,
1.0, 1.0, 0.0, 1.0,
0.0, 1.0, 0.0, 1.0,
0.0, 0.0, 1.0, 1.0
];
pyramidColorBuffer.itemSize = 4;
pyramidColorBuffer.numItems = 5;
gl.bufferData(gl.ARRAY_BUFFER, new Float32Array(pyramidColorBuffer),gl.STATIC_DRAWS);
pyramidIndexBuffer = gl.createBuffer();
gl.bindBuffer(gl.ELEMENT_ARRAY_BUFFER, pyramidIndexBuffer);
let pyramidIndeces = [
0, 1, 2,
0, 2, 3,
0, 3, 4,
0, 4, 1
];
pyramidIndexBuffer.itemSize = 1;
pyramidIndexBuffer.numItems = 12;
gl.bufferData(gl.ELEMENT_ARRAY_BUFFER, new Uint16Array(pyramidIndeces), gl.STATIC_DRAW);
}
function drawScene() {
gl.viewport(0, 0, gl.viewportWidth, gl.viewportHeight);
gl.clear(gl.COLOR_BUFFER_BIT | gl.DEPTH_BUFFER_BIT);
mat4.perspective(45, gl.viewportWidth / gl.viewportHeight, 0.1, 100.0, pMatrix);
mat4.identity(mvMatrix);
mat4.translate(mvMatrix, [-1.5, 0.0, -6.5]); // We store a translation to our matrix, so when the first object is drawn it will be in that position.
// Connect the attributes with the shaders and draw...
gl.bindBuffer(gl.ARRAY_BUFFER,octagonPositionBuffer);
gl.vertexAttribPointer(shaderProgram.vertexPositionAttribute, octagonPositionBuffer.itemSize, gl.FLOAT, false, 0, 0);
gl.bindBuffer(gl.ARRAY_BUFFER, octagonColorBuffer);
gl.vertexAttribPointer(shaderProgram.vertexColorAttribute, octagonColorBuffer.itemSize, gl.FLOAT, false, 0, 0);
gl.bindBuffer(gl.ELEMENT_ARRAY_BUFFER, octagonIndexBuffer);
setMatrixUniforms();
gl.drawElements(gl.TRIANGLES, octagonIndexBuffer.numItems, gl.UNSIGNED_SHORT, 0);
mat4.translate(mvMatrix, [2.8, 0.0, 0.0]);
gl.bindBuffer(gl.ARRAY_BUFFER,pyramidPositionBuffer);
gl.vertexAttribPointer(shaderProgram.vertexPositionAttribute, pyramidPositionBuffer.itemSize, gl.FLOAT, false, 0, 0);
gl.bindBuffer(gl.ARRAY_BUFFER, pyramidColorBuffer);
gl.vertexAttribPointer(shaderProgram.vertexColorAttribute, pyramidColorBuffer.itemSize, gl.FLOAT, false, 0, 0);
gl.bindBuffer(gl.ELEMENT_ARRAY_BUFFER, pyramidIndexBuffer);
setMatrixUniforms();
gl.drawElements(gl.TRIANGLES, pyramidIndexBuffer.numItems, gl.UNSIGNED_SHORT, 0);
}
I created the pyramid with the same way I created the octagon but for some reason it is not appearing. If I try to draw the octagon two times it has no problem.
javascript html5 canvas webgl
I am trying to create two objects an octagon and a pyramid on the canvas. The octagon is appearing without any problem but the pyramid is not. I am attaching the code of the pyramid.
pyramidPositionBuffer = gl.createBuffer();
gl.bindBuffer(gl.ARRAY_BUFFER, pyramidPositionBuffer);
let pyramidVerts = [
0.0, 0.5, 0.0, //0
-0.5, 0.0, -0.5, //1
0.5, 0.0, -0.5, //2
0.5, 0.0, 0.5, //3
-0.5, 0.0, 0.5 //4
];
pyramidPositionBuffer.itemSize = 3;
pyramidPositionBuffer.numItems = 5;
gl.bufferData(gl.ARRAY_BUFFER, new Float32Array(pyramidVerts), gl.STATIC_DRAW);
pyramidColorBuffer = gl.createBuffer();
gl.bindBuffer(gl.ARRAY_BUFFER, pyramidColorBuffer);
let pyramidColors = [
1.0, 1.0, 1.0, 1.0,
1.0, 0.0, 0.0, 1.0,
1.0, 1.0, 0.0, 1.0,
0.0, 1.0, 0.0, 1.0,
0.0, 0.0, 1.0, 1.0
];
pyramidColorBuffer.itemSize = 4;
pyramidColorBuffer.numItems = 5;
gl.bufferData(gl.ARRAY_BUFFER, new Float32Array(pyramidColorBuffer),gl.STATIC_DRAWS);
pyramidIndexBuffer = gl.createBuffer();
gl.bindBuffer(gl.ELEMENT_ARRAY_BUFFER, pyramidIndexBuffer);
let pyramidIndeces = [
0, 1, 2,
0, 2, 3,
0, 3, 4,
0, 4, 1
];
pyramidIndexBuffer.itemSize = 1;
pyramidIndexBuffer.numItems = 12;
gl.bufferData(gl.ELEMENT_ARRAY_BUFFER, new Uint16Array(pyramidIndeces), gl.STATIC_DRAW);
}
function drawScene() {
gl.viewport(0, 0, gl.viewportWidth, gl.viewportHeight);
gl.clear(gl.COLOR_BUFFER_BIT | gl.DEPTH_BUFFER_BIT);
mat4.perspective(45, gl.viewportWidth / gl.viewportHeight, 0.1, 100.0, pMatrix);
mat4.identity(mvMatrix);
mat4.translate(mvMatrix, [-1.5, 0.0, -6.5]); // We store a translation to our matrix, so when the first object is drawn it will be in that position.
// Connect the attributes with the shaders and draw...
gl.bindBuffer(gl.ARRAY_BUFFER,octagonPositionBuffer);
gl.vertexAttribPointer(shaderProgram.vertexPositionAttribute, octagonPositionBuffer.itemSize, gl.FLOAT, false, 0, 0);
gl.bindBuffer(gl.ARRAY_BUFFER, octagonColorBuffer);
gl.vertexAttribPointer(shaderProgram.vertexColorAttribute, octagonColorBuffer.itemSize, gl.FLOAT, false, 0, 0);
gl.bindBuffer(gl.ELEMENT_ARRAY_BUFFER, octagonIndexBuffer);
setMatrixUniforms();
gl.drawElements(gl.TRIANGLES, octagonIndexBuffer.numItems, gl.UNSIGNED_SHORT, 0);
mat4.translate(mvMatrix, [2.8, 0.0, 0.0]);
gl.bindBuffer(gl.ARRAY_BUFFER,pyramidPositionBuffer);
gl.vertexAttribPointer(shaderProgram.vertexPositionAttribute, pyramidPositionBuffer.itemSize, gl.FLOAT, false, 0, 0);
gl.bindBuffer(gl.ARRAY_BUFFER, pyramidColorBuffer);
gl.vertexAttribPointer(shaderProgram.vertexColorAttribute, pyramidColorBuffer.itemSize, gl.FLOAT, false, 0, 0);
gl.bindBuffer(gl.ELEMENT_ARRAY_BUFFER, pyramidIndexBuffer);
setMatrixUniforms();
gl.drawElements(gl.TRIANGLES, pyramidIndexBuffer.numItems, gl.UNSIGNED_SHORT, 0);
}
I created the pyramid with the same way I created the octagon but for some reason it is not appearing. If I try to draw the octagon two times it has no problem.
javascript html5 canvas webgl
javascript html5 canvas webgl
asked Nov 22 at 14:17
Jane
134
134
If you take some time to learn about the JavaScript console in your browser it would have told you these errors.
– gman
Nov 23 at 1:51
add a comment |
If you take some time to learn about the JavaScript console in your browser it would have told you these errors.
– gman
Nov 23 at 1:51
If you take some time to learn about the JavaScript console in your browser it would have told you these errors.
– gman
Nov 23 at 1:51
If you take some time to learn about the JavaScript console in your browser it would have told you these errors.
– gman
Nov 23 at 1:51
add a comment |
1 Answer
1
active
oldest
votes
up vote
1
down vote
accepted
There are 2 issues in the line
gl.bufferData(gl.ARRAY_BUFFER, new Float32Array(pyramidColorBuffer),gl.STATIC_DRAWS);
STATIC_DRAWS
is not a valid enumeration constant, the correct name is STATIC_DRAW
. In this case you should get an INVALID_ENUM error.
pyramidColorBuffer
is the buffer object (pyramidColorBuffer = gl.createBuffer()
), but not the array for the generic vertex attributes data. The name of the array is pyramidColors
. In this case you should get an INVALID_OPERATION error.
Change the line to
gl.bufferData(gl.ARRAY_BUFFER, new Float32Array(pyramidColors), gl.STATIC_DRAW);
to solve the issue.
Enable of the generic vertex attribute arrays is missing in your code:
gl.enableVertexAttribArray( shaderProgram.vertexPositionAttribute );
gl.enableVertexAttribArray( shaderProgram.vertexColorAttribute );
But possibly this is only missing in the code snippet which you have posted in your question.
Thank you very much. I changed what you said and now it is finally working. Thanks again!
– Jane
Nov 22 at 20:00
@Jane You're welcome
– Rabbid76
Nov 22 at 20:01
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
accepted
There are 2 issues in the line
gl.bufferData(gl.ARRAY_BUFFER, new Float32Array(pyramidColorBuffer),gl.STATIC_DRAWS);
STATIC_DRAWS
is not a valid enumeration constant, the correct name is STATIC_DRAW
. In this case you should get an INVALID_ENUM error.
pyramidColorBuffer
is the buffer object (pyramidColorBuffer = gl.createBuffer()
), but not the array for the generic vertex attributes data. The name of the array is pyramidColors
. In this case you should get an INVALID_OPERATION error.
Change the line to
gl.bufferData(gl.ARRAY_BUFFER, new Float32Array(pyramidColors), gl.STATIC_DRAW);
to solve the issue.
Enable of the generic vertex attribute arrays is missing in your code:
gl.enableVertexAttribArray( shaderProgram.vertexPositionAttribute );
gl.enableVertexAttribArray( shaderProgram.vertexColorAttribute );
But possibly this is only missing in the code snippet which you have posted in your question.
Thank you very much. I changed what you said and now it is finally working. Thanks again!
– Jane
Nov 22 at 20:00
@Jane You're welcome
– Rabbid76
Nov 22 at 20:01
add a comment |
up vote
1
down vote
accepted
There are 2 issues in the line
gl.bufferData(gl.ARRAY_BUFFER, new Float32Array(pyramidColorBuffer),gl.STATIC_DRAWS);
STATIC_DRAWS
is not a valid enumeration constant, the correct name is STATIC_DRAW
. In this case you should get an INVALID_ENUM error.
pyramidColorBuffer
is the buffer object (pyramidColorBuffer = gl.createBuffer()
), but not the array for the generic vertex attributes data. The name of the array is pyramidColors
. In this case you should get an INVALID_OPERATION error.
Change the line to
gl.bufferData(gl.ARRAY_BUFFER, new Float32Array(pyramidColors), gl.STATIC_DRAW);
to solve the issue.
Enable of the generic vertex attribute arrays is missing in your code:
gl.enableVertexAttribArray( shaderProgram.vertexPositionAttribute );
gl.enableVertexAttribArray( shaderProgram.vertexColorAttribute );
But possibly this is only missing in the code snippet which you have posted in your question.
Thank you very much. I changed what you said and now it is finally working. Thanks again!
– Jane
Nov 22 at 20:00
@Jane You're welcome
– Rabbid76
Nov 22 at 20:01
add a comment |
up vote
1
down vote
accepted
up vote
1
down vote
accepted
There are 2 issues in the line
gl.bufferData(gl.ARRAY_BUFFER, new Float32Array(pyramidColorBuffer),gl.STATIC_DRAWS);
STATIC_DRAWS
is not a valid enumeration constant, the correct name is STATIC_DRAW
. In this case you should get an INVALID_ENUM error.
pyramidColorBuffer
is the buffer object (pyramidColorBuffer = gl.createBuffer()
), but not the array for the generic vertex attributes data. The name of the array is pyramidColors
. In this case you should get an INVALID_OPERATION error.
Change the line to
gl.bufferData(gl.ARRAY_BUFFER, new Float32Array(pyramidColors), gl.STATIC_DRAW);
to solve the issue.
Enable of the generic vertex attribute arrays is missing in your code:
gl.enableVertexAttribArray( shaderProgram.vertexPositionAttribute );
gl.enableVertexAttribArray( shaderProgram.vertexColorAttribute );
But possibly this is only missing in the code snippet which you have posted in your question.
There are 2 issues in the line
gl.bufferData(gl.ARRAY_BUFFER, new Float32Array(pyramidColorBuffer),gl.STATIC_DRAWS);
STATIC_DRAWS
is not a valid enumeration constant, the correct name is STATIC_DRAW
. In this case you should get an INVALID_ENUM error.
pyramidColorBuffer
is the buffer object (pyramidColorBuffer = gl.createBuffer()
), but not the array for the generic vertex attributes data. The name of the array is pyramidColors
. In this case you should get an INVALID_OPERATION error.
Change the line to
gl.bufferData(gl.ARRAY_BUFFER, new Float32Array(pyramidColors), gl.STATIC_DRAW);
to solve the issue.
Enable of the generic vertex attribute arrays is missing in your code:
gl.enableVertexAttribArray( shaderProgram.vertexPositionAttribute );
gl.enableVertexAttribArray( shaderProgram.vertexColorAttribute );
But possibly this is only missing in the code snippet which you have posted in your question.
edited Nov 22 at 18:31
answered Nov 22 at 18:19


Rabbid76
31.4k112842
31.4k112842
Thank you very much. I changed what you said and now it is finally working. Thanks again!
– Jane
Nov 22 at 20:00
@Jane You're welcome
– Rabbid76
Nov 22 at 20:01
add a comment |
Thank you very much. I changed what you said and now it is finally working. Thanks again!
– Jane
Nov 22 at 20:00
@Jane You're welcome
– Rabbid76
Nov 22 at 20:01
Thank you very much. I changed what you said and now it is finally working. Thanks again!
– Jane
Nov 22 at 20:00
Thank you very much. I changed what you said and now it is finally working. Thanks again!
– Jane
Nov 22 at 20:00
@Jane You're welcome
– Rabbid76
Nov 22 at 20:01
@Jane You're welcome
– Rabbid76
Nov 22 at 20:01
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53432916%2fpyramid-is-not-appearing-on-the-canvas-web-gl%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
O,KTaH0Dj7O4kTy SBe4Lp4E3hF1wT09C9vCMTbSsobLUPzN GBp,38TvX8qu
If you take some time to learn about the JavaScript console in your browser it would have told you these errors.
– gman
Nov 23 at 1:51