Ignoring not void method inside testing method in Mockito
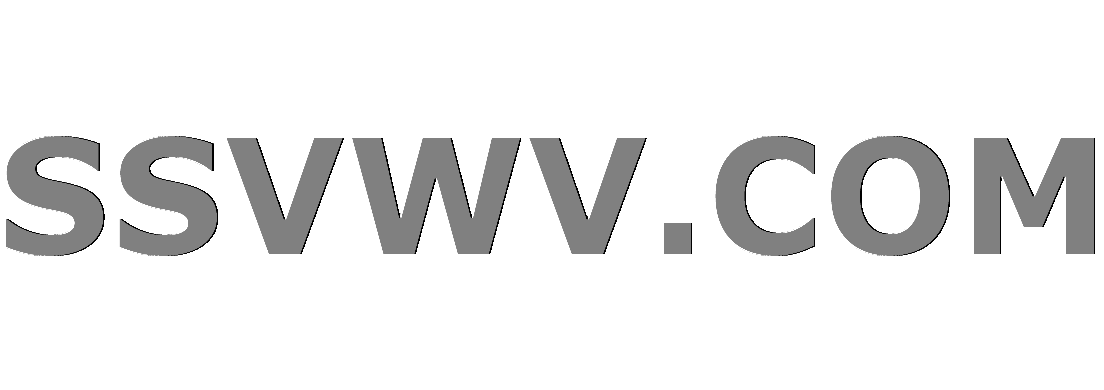
Multi tool use
up vote
0
down vote
favorite
In class Account I have a method public Account reserveA()
which I want to test, inside reserveA
is called a method public Bank DAO.createB()
. Is there a way to call reserveA()
in test method but ignore call DAO.createB()
? Non of these methods are void.
I tried:
doNothing().when(Account).reserveA(param1, param2);
but it's not the proper way.
java junit mockito
add a comment |
up vote
0
down vote
favorite
In class Account I have a method public Account reserveA()
which I want to test, inside reserveA
is called a method public Bank DAO.createB()
. Is there a way to call reserveA()
in test method but ignore call DAO.createB()
? Non of these methods are void.
I tried:
doNothing().when(Account).reserveA(param1, param2);
but it's not the proper way.
java junit mockito
In test method, comment the line where thecreateB()
function is executed
– javimovi
Nov 22 at 14:09
1
It seems you're asking how to use Mockito. Have you read its documentation? It's quite easy and pleasant to read. And you'll learn a lot of stuff. static.javadoc.io/org.mockito/mockito-core/2.23.4/org/mockito/…. If you actually want an answer, then post code.
– JB Nizet
Nov 22 at 14:12
@javimovicreateB
is not even called in test method. JustreserveA
is called and inside thiscreateB
is called.
– Michu93
Nov 22 at 14:12
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
In class Account I have a method public Account reserveA()
which I want to test, inside reserveA
is called a method public Bank DAO.createB()
. Is there a way to call reserveA()
in test method but ignore call DAO.createB()
? Non of these methods are void.
I tried:
doNothing().when(Account).reserveA(param1, param2);
but it's not the proper way.
java junit mockito
In class Account I have a method public Account reserveA()
which I want to test, inside reserveA
is called a method public Bank DAO.createB()
. Is there a way to call reserveA()
in test method but ignore call DAO.createB()
? Non of these methods are void.
I tried:
doNothing().when(Account).reserveA(param1, param2);
but it's not the proper way.
java junit mockito
java junit mockito
edited Nov 22 at 20:31


wojteo
3301522
3301522
asked Nov 22 at 14:03


Michu93
774728
774728
In test method, comment the line where thecreateB()
function is executed
– javimovi
Nov 22 at 14:09
1
It seems you're asking how to use Mockito. Have you read its documentation? It's quite easy and pleasant to read. And you'll learn a lot of stuff. static.javadoc.io/org.mockito/mockito-core/2.23.4/org/mockito/…. If you actually want an answer, then post code.
– JB Nizet
Nov 22 at 14:12
@javimovicreateB
is not even called in test method. JustreserveA
is called and inside thiscreateB
is called.
– Michu93
Nov 22 at 14:12
add a comment |
In test method, comment the line where thecreateB()
function is executed
– javimovi
Nov 22 at 14:09
1
It seems you're asking how to use Mockito. Have you read its documentation? It's quite easy and pleasant to read. And you'll learn a lot of stuff. static.javadoc.io/org.mockito/mockito-core/2.23.4/org/mockito/…. If you actually want an answer, then post code.
– JB Nizet
Nov 22 at 14:12
@javimovicreateB
is not even called in test method. JustreserveA
is called and inside thiscreateB
is called.
– Michu93
Nov 22 at 14:12
In test method, comment the line where the
createB()
function is executed– javimovi
Nov 22 at 14:09
In test method, comment the line where the
createB()
function is executed– javimovi
Nov 22 at 14:09
1
1
It seems you're asking how to use Mockito. Have you read its documentation? It's quite easy and pleasant to read. And you'll learn a lot of stuff. static.javadoc.io/org.mockito/mockito-core/2.23.4/org/mockito/…. If you actually want an answer, then post code.
– JB Nizet
Nov 22 at 14:12
It seems you're asking how to use Mockito. Have you read its documentation? It's quite easy and pleasant to read. And you'll learn a lot of stuff. static.javadoc.io/org.mockito/mockito-core/2.23.4/org/mockito/…. If you actually want an answer, then post code.
– JB Nizet
Nov 22 at 14:12
@javimovi
createB
is not even called in test method. Just reserveA
is called and inside this createB
is called.– Michu93
Nov 22 at 14:12
@javimovi
createB
is not even called in test method. Just reserveA
is called and inside this createB
is called.– Michu93
Nov 22 at 14:12
add a comment |
1 Answer
1
active
oldest
votes
up vote
1
down vote
doNothing() is reserved only for void methods.
If your method returns something, then you are required to do as well (or throw exception).
Depending on complexity of your Account.reserveString(), you may need to mock some more than just this one method call if result is used somewhere else.
Trying to use doNothing() on non-void method results in error:
org.mockito.exceptions.base.MockitoException:
Only void methods can doNothing()!
Example of correct use of doNothing():
doNothing().
doThrow(new RuntimeException())
.when(mock).someVoidMethod();
Above means:
someVoidMethod() does nothing the 1st time but throws an exception the 2nd time is called
Consider such classes:
@Component
public class BankDao {
public BankDao() {}
public void createVoid() {
System.out.println("sth - 1");
}
public String createString(){
return "sth - 2";
}
}
@Service
public class Account {
@Autowired
private final BankDao DAO;
public Account(BankDao dao) {
this.DAO = dao;
}
public void reserveVoid() {
System.out.println("before");
DAO.createVoid();
System.out.println("after");
}
public void reserveString() {
System.out.println(DAO.createString());
}
}
For which Test class is made:
@RunWith(MockitoJUnitRunner.class)
public class AccountTest {
@Mock
private BankDao bankDao;
@InjectMocks
private Account account;
@Test
public void reserveVoid_mockBankDaoAndDontUseRealMethod() {
doNothing().when(bankDao).createVoid();
account.reserveVoid();
}
@Test
public void reserveString_mockBankDaoAndDontUseRealMethod() {
when(bankDao.createString()).thenReturn("nothing");
account.reserveString();
}
}
Running such a test will produce:
nothing
before
after
If you change @Mock to @Spy and remove lines with doNothing() and when(), then you'll be calling original methods. Result would be:
sth - 2
before
sth - 1
after
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
doNothing() is reserved only for void methods.
If your method returns something, then you are required to do as well (or throw exception).
Depending on complexity of your Account.reserveString(), you may need to mock some more than just this one method call if result is used somewhere else.
Trying to use doNothing() on non-void method results in error:
org.mockito.exceptions.base.MockitoException:
Only void methods can doNothing()!
Example of correct use of doNothing():
doNothing().
doThrow(new RuntimeException())
.when(mock).someVoidMethod();
Above means:
someVoidMethod() does nothing the 1st time but throws an exception the 2nd time is called
Consider such classes:
@Component
public class BankDao {
public BankDao() {}
public void createVoid() {
System.out.println("sth - 1");
}
public String createString(){
return "sth - 2";
}
}
@Service
public class Account {
@Autowired
private final BankDao DAO;
public Account(BankDao dao) {
this.DAO = dao;
}
public void reserveVoid() {
System.out.println("before");
DAO.createVoid();
System.out.println("after");
}
public void reserveString() {
System.out.println(DAO.createString());
}
}
For which Test class is made:
@RunWith(MockitoJUnitRunner.class)
public class AccountTest {
@Mock
private BankDao bankDao;
@InjectMocks
private Account account;
@Test
public void reserveVoid_mockBankDaoAndDontUseRealMethod() {
doNothing().when(bankDao).createVoid();
account.reserveVoid();
}
@Test
public void reserveString_mockBankDaoAndDontUseRealMethod() {
when(bankDao.createString()).thenReturn("nothing");
account.reserveString();
}
}
Running such a test will produce:
nothing
before
after
If you change @Mock to @Spy and remove lines with doNothing() and when(), then you'll be calling original methods. Result would be:
sth - 2
before
sth - 1
after
add a comment |
up vote
1
down vote
doNothing() is reserved only for void methods.
If your method returns something, then you are required to do as well (or throw exception).
Depending on complexity of your Account.reserveString(), you may need to mock some more than just this one method call if result is used somewhere else.
Trying to use doNothing() on non-void method results in error:
org.mockito.exceptions.base.MockitoException:
Only void methods can doNothing()!
Example of correct use of doNothing():
doNothing().
doThrow(new RuntimeException())
.when(mock).someVoidMethod();
Above means:
someVoidMethod() does nothing the 1st time but throws an exception the 2nd time is called
Consider such classes:
@Component
public class BankDao {
public BankDao() {}
public void createVoid() {
System.out.println("sth - 1");
}
public String createString(){
return "sth - 2";
}
}
@Service
public class Account {
@Autowired
private final BankDao DAO;
public Account(BankDao dao) {
this.DAO = dao;
}
public void reserveVoid() {
System.out.println("before");
DAO.createVoid();
System.out.println("after");
}
public void reserveString() {
System.out.println(DAO.createString());
}
}
For which Test class is made:
@RunWith(MockitoJUnitRunner.class)
public class AccountTest {
@Mock
private BankDao bankDao;
@InjectMocks
private Account account;
@Test
public void reserveVoid_mockBankDaoAndDontUseRealMethod() {
doNothing().when(bankDao).createVoid();
account.reserveVoid();
}
@Test
public void reserveString_mockBankDaoAndDontUseRealMethod() {
when(bankDao.createString()).thenReturn("nothing");
account.reserveString();
}
}
Running such a test will produce:
nothing
before
after
If you change @Mock to @Spy and remove lines with doNothing() and when(), then you'll be calling original methods. Result would be:
sth - 2
before
sth - 1
after
add a comment |
up vote
1
down vote
up vote
1
down vote
doNothing() is reserved only for void methods.
If your method returns something, then you are required to do as well (or throw exception).
Depending on complexity of your Account.reserveString(), you may need to mock some more than just this one method call if result is used somewhere else.
Trying to use doNothing() on non-void method results in error:
org.mockito.exceptions.base.MockitoException:
Only void methods can doNothing()!
Example of correct use of doNothing():
doNothing().
doThrow(new RuntimeException())
.when(mock).someVoidMethod();
Above means:
someVoidMethod() does nothing the 1st time but throws an exception the 2nd time is called
Consider such classes:
@Component
public class BankDao {
public BankDao() {}
public void createVoid() {
System.out.println("sth - 1");
}
public String createString(){
return "sth - 2";
}
}
@Service
public class Account {
@Autowired
private final BankDao DAO;
public Account(BankDao dao) {
this.DAO = dao;
}
public void reserveVoid() {
System.out.println("before");
DAO.createVoid();
System.out.println("after");
}
public void reserveString() {
System.out.println(DAO.createString());
}
}
For which Test class is made:
@RunWith(MockitoJUnitRunner.class)
public class AccountTest {
@Mock
private BankDao bankDao;
@InjectMocks
private Account account;
@Test
public void reserveVoid_mockBankDaoAndDontUseRealMethod() {
doNothing().when(bankDao).createVoid();
account.reserveVoid();
}
@Test
public void reserveString_mockBankDaoAndDontUseRealMethod() {
when(bankDao.createString()).thenReturn("nothing");
account.reserveString();
}
}
Running such a test will produce:
nothing
before
after
If you change @Mock to @Spy and remove lines with doNothing() and when(), then you'll be calling original methods. Result would be:
sth - 2
before
sth - 1
after
doNothing() is reserved only for void methods.
If your method returns something, then you are required to do as well (or throw exception).
Depending on complexity of your Account.reserveString(), you may need to mock some more than just this one method call if result is used somewhere else.
Trying to use doNothing() on non-void method results in error:
org.mockito.exceptions.base.MockitoException:
Only void methods can doNothing()!
Example of correct use of doNothing():
doNothing().
doThrow(new RuntimeException())
.when(mock).someVoidMethod();
Above means:
someVoidMethod() does nothing the 1st time but throws an exception the 2nd time is called
Consider such classes:
@Component
public class BankDao {
public BankDao() {}
public void createVoid() {
System.out.println("sth - 1");
}
public String createString(){
return "sth - 2";
}
}
@Service
public class Account {
@Autowired
private final BankDao DAO;
public Account(BankDao dao) {
this.DAO = dao;
}
public void reserveVoid() {
System.out.println("before");
DAO.createVoid();
System.out.println("after");
}
public void reserveString() {
System.out.println(DAO.createString());
}
}
For which Test class is made:
@RunWith(MockitoJUnitRunner.class)
public class AccountTest {
@Mock
private BankDao bankDao;
@InjectMocks
private Account account;
@Test
public void reserveVoid_mockBankDaoAndDontUseRealMethod() {
doNothing().when(bankDao).createVoid();
account.reserveVoid();
}
@Test
public void reserveString_mockBankDaoAndDontUseRealMethod() {
when(bankDao.createString()).thenReturn("nothing");
account.reserveString();
}
}
Running such a test will produce:
nothing
before
after
If you change @Mock to @Spy and remove lines with doNothing() and when(), then you'll be calling original methods. Result would be:
sth - 2
before
sth - 1
after
edited Nov 22 at 20:12
answered Nov 22 at 20:07


wojteo
3301522
3301522
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53432677%2fignoring-not-void-method-inside-testing-method-in-mockito%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
MSZDk7mcK,DLlZnsT
In test method, comment the line where the
createB()
function is executed– javimovi
Nov 22 at 14:09
1
It seems you're asking how to use Mockito. Have you read its documentation? It's quite easy and pleasant to read. And you'll learn a lot of stuff. static.javadoc.io/org.mockito/mockito-core/2.23.4/org/mockito/…. If you actually want an answer, then post code.
– JB Nizet
Nov 22 at 14:12
@javimovi
createB
is not even called in test method. JustreserveA
is called and inside thiscreateB
is called.– Michu93
Nov 22 at 14:12