How to load Assembly at runtime and create class instance?
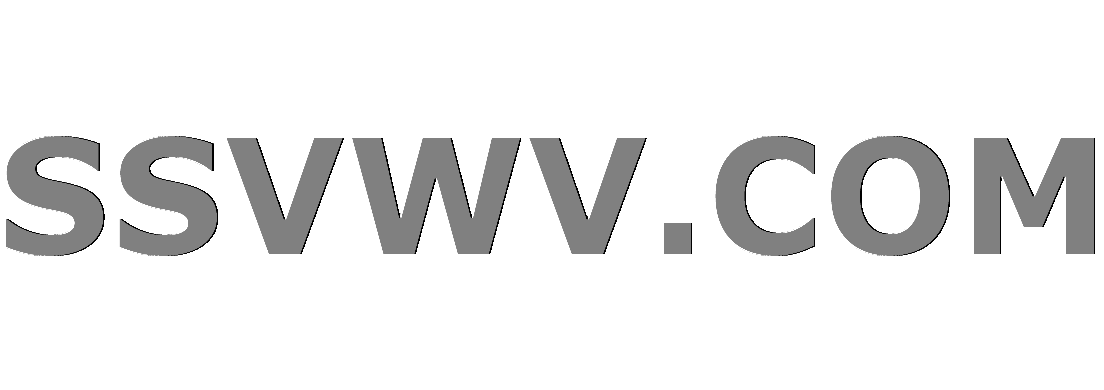
Multi tool use
up vote
17
down vote
favorite
I have a assembly. In this assembly I have a class and interface. I need to load this assembly at runtime and want to create an object of the class and also want to use the interface.
Assembly MyDALL = Assembly.Load("DALL"); // DALL is name of my dll
Type MyLoadClass = MyDALL.GetType("DALL.LoadClass"); // LoadClass is my class
object obj = Activator.CreateInstance(MyLoadClass);
This is my code. How could it be improved?
c# dll reflection load assemblies
add a comment |
up vote
17
down vote
favorite
I have a assembly. In this assembly I have a class and interface. I need to load this assembly at runtime and want to create an object of the class and also want to use the interface.
Assembly MyDALL = Assembly.Load("DALL"); // DALL is name of my dll
Type MyLoadClass = MyDALL.GetType("DALL.LoadClass"); // LoadClass is my class
object obj = Activator.CreateInstance(MyLoadClass);
This is my code. How could it be improved?
c# dll reflection load assemblies
possible duplicate of C# - Correct Way to Load Assembly, Find Class and Call Run() Method
– Alex Angas
Sep 15 '10 at 6:21
One more question, if the dll being loaded needs another dll, could I load multiple dlls the same time?
– NewDTinStackoverflow
Apr 16 '13 at 21:58
add a comment |
up vote
17
down vote
favorite
up vote
17
down vote
favorite
I have a assembly. In this assembly I have a class and interface. I need to load this assembly at runtime and want to create an object of the class and also want to use the interface.
Assembly MyDALL = Assembly.Load("DALL"); // DALL is name of my dll
Type MyLoadClass = MyDALL.GetType("DALL.LoadClass"); // LoadClass is my class
object obj = Activator.CreateInstance(MyLoadClass);
This is my code. How could it be improved?
c# dll reflection load assemblies
I have a assembly. In this assembly I have a class and interface. I need to load this assembly at runtime and want to create an object of the class and also want to use the interface.
Assembly MyDALL = Assembly.Load("DALL"); // DALL is name of my dll
Type MyLoadClass = MyDALL.GetType("DALL.LoadClass"); // LoadClass is my class
object obj = Activator.CreateInstance(MyLoadClass);
This is my code. How could it be improved?
c# dll reflection load assemblies
c# dll reflection load assemblies
edited Mar 8 at 10:50


daniele3004
5,18573647
5,18573647
asked Nov 26 '09 at 13:00
Pankaj
1,760144061
1,760144061
possible duplicate of C# - Correct Way to Load Assembly, Find Class and Call Run() Method
– Alex Angas
Sep 15 '10 at 6:21
One more question, if the dll being loaded needs another dll, could I load multiple dlls the same time?
– NewDTinStackoverflow
Apr 16 '13 at 21:58
add a comment |
possible duplicate of C# - Correct Way to Load Assembly, Find Class and Call Run() Method
– Alex Angas
Sep 15 '10 at 6:21
One more question, if the dll being loaded needs another dll, could I load multiple dlls the same time?
– NewDTinStackoverflow
Apr 16 '13 at 21:58
possible duplicate of C# - Correct Way to Load Assembly, Find Class and Call Run() Method
– Alex Angas
Sep 15 '10 at 6:21
possible duplicate of C# - Correct Way to Load Assembly, Find Class and Call Run() Method
– Alex Angas
Sep 15 '10 at 6:21
One more question, if the dll being loaded needs another dll, could I load multiple dlls the same time?
– NewDTinStackoverflow
Apr 16 '13 at 21:58
One more question, if the dll being loaded needs another dll, could I load multiple dlls the same time?
– NewDTinStackoverflow
Apr 16 '13 at 21:58
add a comment |
3 Answers
3
active
oldest
votes
up vote
18
down vote
accepted
If your assembly is in GAC or bin use the assembly name at the end of type name instead of Assembly.Load()
.
object obj = Activator.CreateInstance(Type.GetType("DALL.LoadClass, DALL", true));
add a comment |
up vote
12
down vote
You should Use Dynamic Method with for Improving. its faster than reflection..
Here is a sample code for creating Object using Dynamic Method..
public class ObjectCreateMethod
{
delegate object MethodInvoker();
MethodInvoker methodHandler = null;
public ObjectCreateMethod(Type type)
{
CreateMethod(type.GetConstructor(Type.EmptyTypes));
}
public ObjectCreateMethod(ConstructorInfo target)
{
CreateMethod(target);
}
void CreateMethod(ConstructorInfo target)
{
DynamicMethod dynamic = new DynamicMethod(string.Empty,
typeof(object),
new Type[0],
target.DeclaringType);
ILGenerator il = dynamic.GetILGenerator();
il.DeclareLocal(target.DeclaringType);
il.Emit(OpCodes.Newobj, target);
il.Emit(OpCodes.Stloc_0);
il.Emit(OpCodes.Ldloc_0);
il.Emit(OpCodes.Ret);
methodHandler = (MethodInvoker)dynamic.CreateDelegate(typeof(MethodInvoker));
}
public object CreateInstance()
{
return methodHandler();
}
}
//Use Above class for Object Creation.
ObjectCreateMethod inv = new ObjectCreateMethod(type); //Specify Type
Object obj= inv.CreateInstance();
This method takes only 1/10th time needed by Activator.
Check out http://www.ozcandegirmenci.com/post/2008/02/Create-object-instances-Faster-than-Reflection.aspx
Is there no need to specify the name of the assembly?
– paz
Aug 8 '13 at 18:11
How can this be change to call constructors with parameters such as another object?
– DRobertE
Mar 21 '14 at 16:53
Does this work on Mono?
– jjxtra
Jul 17 '14 at 4:21
add a comment |
up vote
0
down vote
Check out http://www.youtube.com/watch?v=x-KK7bmo1AM
To modify his code to load multiple assemblies use
static Assembly CurrentDomain_AssemblyResolve(object sender, ResolveEventArgs args)
{
string assemblyName = args.Name.Split(',').First();
using (var stream = Assembly.GetExecutingAssembly().GetManifestResourceStream("YourNamespace." + assemblyName + ".dll"))
{
byte assemblyData = new byte[stream.Length];
stream.Read(assemblyData, 0, assemblyData.Length);
return Assembly.Load(assemblyData);
}
}
In your main method put
AppDomain.CurrentDomain.AssemblyResolve += CurrentDomain_AssemblyResolve;
Be sure to add your assemblies to your project and change the build action property to "Embedded Resource".
add a comment |
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
18
down vote
accepted
If your assembly is in GAC or bin use the assembly name at the end of type name instead of Assembly.Load()
.
object obj = Activator.CreateInstance(Type.GetType("DALL.LoadClass, DALL", true));
add a comment |
up vote
18
down vote
accepted
If your assembly is in GAC or bin use the assembly name at the end of type name instead of Assembly.Load()
.
object obj = Activator.CreateInstance(Type.GetType("DALL.LoadClass, DALL", true));
add a comment |
up vote
18
down vote
accepted
up vote
18
down vote
accepted
If your assembly is in GAC or bin use the assembly name at the end of type name instead of Assembly.Load()
.
object obj = Activator.CreateInstance(Type.GetType("DALL.LoadClass, DALL", true));
If your assembly is in GAC or bin use the assembly name at the end of type name instead of Assembly.Load()
.
object obj = Activator.CreateInstance(Type.GetType("DALL.LoadClass, DALL", true));
answered Nov 26 '09 at 14:11


Mehdi Golchin
7,26322634
7,26322634
add a comment |
add a comment |
up vote
12
down vote
You should Use Dynamic Method with for Improving. its faster than reflection..
Here is a sample code for creating Object using Dynamic Method..
public class ObjectCreateMethod
{
delegate object MethodInvoker();
MethodInvoker methodHandler = null;
public ObjectCreateMethod(Type type)
{
CreateMethod(type.GetConstructor(Type.EmptyTypes));
}
public ObjectCreateMethod(ConstructorInfo target)
{
CreateMethod(target);
}
void CreateMethod(ConstructorInfo target)
{
DynamicMethod dynamic = new DynamicMethod(string.Empty,
typeof(object),
new Type[0],
target.DeclaringType);
ILGenerator il = dynamic.GetILGenerator();
il.DeclareLocal(target.DeclaringType);
il.Emit(OpCodes.Newobj, target);
il.Emit(OpCodes.Stloc_0);
il.Emit(OpCodes.Ldloc_0);
il.Emit(OpCodes.Ret);
methodHandler = (MethodInvoker)dynamic.CreateDelegate(typeof(MethodInvoker));
}
public object CreateInstance()
{
return methodHandler();
}
}
//Use Above class for Object Creation.
ObjectCreateMethod inv = new ObjectCreateMethod(type); //Specify Type
Object obj= inv.CreateInstance();
This method takes only 1/10th time needed by Activator.
Check out http://www.ozcandegirmenci.com/post/2008/02/Create-object-instances-Faster-than-Reflection.aspx
Is there no need to specify the name of the assembly?
– paz
Aug 8 '13 at 18:11
How can this be change to call constructors with parameters such as another object?
– DRobertE
Mar 21 '14 at 16:53
Does this work on Mono?
– jjxtra
Jul 17 '14 at 4:21
add a comment |
up vote
12
down vote
You should Use Dynamic Method with for Improving. its faster than reflection..
Here is a sample code for creating Object using Dynamic Method..
public class ObjectCreateMethod
{
delegate object MethodInvoker();
MethodInvoker methodHandler = null;
public ObjectCreateMethod(Type type)
{
CreateMethod(type.GetConstructor(Type.EmptyTypes));
}
public ObjectCreateMethod(ConstructorInfo target)
{
CreateMethod(target);
}
void CreateMethod(ConstructorInfo target)
{
DynamicMethod dynamic = new DynamicMethod(string.Empty,
typeof(object),
new Type[0],
target.DeclaringType);
ILGenerator il = dynamic.GetILGenerator();
il.DeclareLocal(target.DeclaringType);
il.Emit(OpCodes.Newobj, target);
il.Emit(OpCodes.Stloc_0);
il.Emit(OpCodes.Ldloc_0);
il.Emit(OpCodes.Ret);
methodHandler = (MethodInvoker)dynamic.CreateDelegate(typeof(MethodInvoker));
}
public object CreateInstance()
{
return methodHandler();
}
}
//Use Above class for Object Creation.
ObjectCreateMethod inv = new ObjectCreateMethod(type); //Specify Type
Object obj= inv.CreateInstance();
This method takes only 1/10th time needed by Activator.
Check out http://www.ozcandegirmenci.com/post/2008/02/Create-object-instances-Faster-than-Reflection.aspx
Is there no need to specify the name of the assembly?
– paz
Aug 8 '13 at 18:11
How can this be change to call constructors with parameters such as another object?
– DRobertE
Mar 21 '14 at 16:53
Does this work on Mono?
– jjxtra
Jul 17 '14 at 4:21
add a comment |
up vote
12
down vote
up vote
12
down vote
You should Use Dynamic Method with for Improving. its faster than reflection..
Here is a sample code for creating Object using Dynamic Method..
public class ObjectCreateMethod
{
delegate object MethodInvoker();
MethodInvoker methodHandler = null;
public ObjectCreateMethod(Type type)
{
CreateMethod(type.GetConstructor(Type.EmptyTypes));
}
public ObjectCreateMethod(ConstructorInfo target)
{
CreateMethod(target);
}
void CreateMethod(ConstructorInfo target)
{
DynamicMethod dynamic = new DynamicMethod(string.Empty,
typeof(object),
new Type[0],
target.DeclaringType);
ILGenerator il = dynamic.GetILGenerator();
il.DeclareLocal(target.DeclaringType);
il.Emit(OpCodes.Newobj, target);
il.Emit(OpCodes.Stloc_0);
il.Emit(OpCodes.Ldloc_0);
il.Emit(OpCodes.Ret);
methodHandler = (MethodInvoker)dynamic.CreateDelegate(typeof(MethodInvoker));
}
public object CreateInstance()
{
return methodHandler();
}
}
//Use Above class for Object Creation.
ObjectCreateMethod inv = new ObjectCreateMethod(type); //Specify Type
Object obj= inv.CreateInstance();
This method takes only 1/10th time needed by Activator.
Check out http://www.ozcandegirmenci.com/post/2008/02/Create-object-instances-Faster-than-Reflection.aspx
You should Use Dynamic Method with for Improving. its faster than reflection..
Here is a sample code for creating Object using Dynamic Method..
public class ObjectCreateMethod
{
delegate object MethodInvoker();
MethodInvoker methodHandler = null;
public ObjectCreateMethod(Type type)
{
CreateMethod(type.GetConstructor(Type.EmptyTypes));
}
public ObjectCreateMethod(ConstructorInfo target)
{
CreateMethod(target);
}
void CreateMethod(ConstructorInfo target)
{
DynamicMethod dynamic = new DynamicMethod(string.Empty,
typeof(object),
new Type[0],
target.DeclaringType);
ILGenerator il = dynamic.GetILGenerator();
il.DeclareLocal(target.DeclaringType);
il.Emit(OpCodes.Newobj, target);
il.Emit(OpCodes.Stloc_0);
il.Emit(OpCodes.Ldloc_0);
il.Emit(OpCodes.Ret);
methodHandler = (MethodInvoker)dynamic.CreateDelegate(typeof(MethodInvoker));
}
public object CreateInstance()
{
return methodHandler();
}
}
//Use Above class for Object Creation.
ObjectCreateMethod inv = new ObjectCreateMethod(type); //Specify Type
Object obj= inv.CreateInstance();
This method takes only 1/10th time needed by Activator.
Check out http://www.ozcandegirmenci.com/post/2008/02/Create-object-instances-Faster-than-Reflection.aspx
edited Nov 22 at 13:40
Burcu Co
486
486
answered Nov 26 '09 at 20:57
Sasikumar D.R.
6741516
6741516
Is there no need to specify the name of the assembly?
– paz
Aug 8 '13 at 18:11
How can this be change to call constructors with parameters such as another object?
– DRobertE
Mar 21 '14 at 16:53
Does this work on Mono?
– jjxtra
Jul 17 '14 at 4:21
add a comment |
Is there no need to specify the name of the assembly?
– paz
Aug 8 '13 at 18:11
How can this be change to call constructors with parameters such as another object?
– DRobertE
Mar 21 '14 at 16:53
Does this work on Mono?
– jjxtra
Jul 17 '14 at 4:21
Is there no need to specify the name of the assembly?
– paz
Aug 8 '13 at 18:11
Is there no need to specify the name of the assembly?
– paz
Aug 8 '13 at 18:11
How can this be change to call constructors with parameters such as another object?
– DRobertE
Mar 21 '14 at 16:53
How can this be change to call constructors with parameters such as another object?
– DRobertE
Mar 21 '14 at 16:53
Does this work on Mono?
– jjxtra
Jul 17 '14 at 4:21
Does this work on Mono?
– jjxtra
Jul 17 '14 at 4:21
add a comment |
up vote
0
down vote
Check out http://www.youtube.com/watch?v=x-KK7bmo1AM
To modify his code to load multiple assemblies use
static Assembly CurrentDomain_AssemblyResolve(object sender, ResolveEventArgs args)
{
string assemblyName = args.Name.Split(',').First();
using (var stream = Assembly.GetExecutingAssembly().GetManifestResourceStream("YourNamespace." + assemblyName + ".dll"))
{
byte assemblyData = new byte[stream.Length];
stream.Read(assemblyData, 0, assemblyData.Length);
return Assembly.Load(assemblyData);
}
}
In your main method put
AppDomain.CurrentDomain.AssemblyResolve += CurrentDomain_AssemblyResolve;
Be sure to add your assemblies to your project and change the build action property to "Embedded Resource".
add a comment |
up vote
0
down vote
Check out http://www.youtube.com/watch?v=x-KK7bmo1AM
To modify his code to load multiple assemblies use
static Assembly CurrentDomain_AssemblyResolve(object sender, ResolveEventArgs args)
{
string assemblyName = args.Name.Split(',').First();
using (var stream = Assembly.GetExecutingAssembly().GetManifestResourceStream("YourNamespace." + assemblyName + ".dll"))
{
byte assemblyData = new byte[stream.Length];
stream.Read(assemblyData, 0, assemblyData.Length);
return Assembly.Load(assemblyData);
}
}
In your main method put
AppDomain.CurrentDomain.AssemblyResolve += CurrentDomain_AssemblyResolve;
Be sure to add your assemblies to your project and change the build action property to "Embedded Resource".
add a comment |
up vote
0
down vote
up vote
0
down vote
Check out http://www.youtube.com/watch?v=x-KK7bmo1AM
To modify his code to load multiple assemblies use
static Assembly CurrentDomain_AssemblyResolve(object sender, ResolveEventArgs args)
{
string assemblyName = args.Name.Split(',').First();
using (var stream = Assembly.GetExecutingAssembly().GetManifestResourceStream("YourNamespace." + assemblyName + ".dll"))
{
byte assemblyData = new byte[stream.Length];
stream.Read(assemblyData, 0, assemblyData.Length);
return Assembly.Load(assemblyData);
}
}
In your main method put
AppDomain.CurrentDomain.AssemblyResolve += CurrentDomain_AssemblyResolve;
Be sure to add your assemblies to your project and change the build action property to "Embedded Resource".
Check out http://www.youtube.com/watch?v=x-KK7bmo1AM
To modify his code to load multiple assemblies use
static Assembly CurrentDomain_AssemblyResolve(object sender, ResolveEventArgs args)
{
string assemblyName = args.Name.Split(',').First();
using (var stream = Assembly.GetExecutingAssembly().GetManifestResourceStream("YourNamespace." + assemblyName + ".dll"))
{
byte assemblyData = new byte[stream.Length];
stream.Read(assemblyData, 0, assemblyData.Length);
return Assembly.Load(assemblyData);
}
}
In your main method put
AppDomain.CurrentDomain.AssemblyResolve += CurrentDomain_AssemblyResolve;
Be sure to add your assemblies to your project and change the build action property to "Embedded Resource".
edited May 24 '13 at 22:24
answered May 24 '13 at 22:13


reggaeguitar
1,1621836
1,1621836
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f1803540%2fhow-to-load-assembly-at-runtime-and-create-class-instance%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
S5,px,v0
possible duplicate of C# - Correct Way to Load Assembly, Find Class and Call Run() Method
– Alex Angas
Sep 15 '10 at 6:21
One more question, if the dll being loaded needs another dll, could I load multiple dlls the same time?
– NewDTinStackoverflow
Apr 16 '13 at 21:58