What is the cmp.addValueProvider() feature designed for?
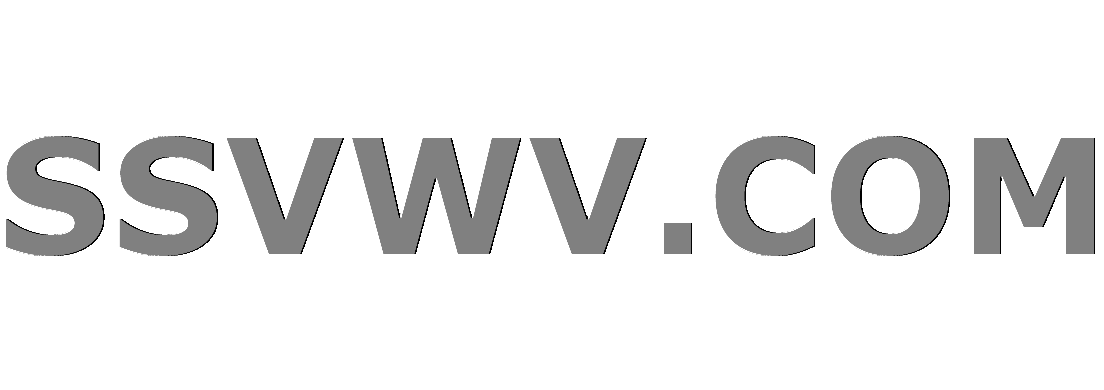
Multi tool use
up vote
7
down vote
favorite
To implement something like a public ValueProvider I implemented a component combining the features out there to have static values available in js and markup. Now I'm having trouble using the addValueProvider Feature (used for the markup side) since there is no documentation out there.
To make static values accessible for any component, I need to reference the value providing component in the concrete component like:
<!-- Value Providers -->
<c:THINGS context="{!this}" />
THINGS.cmp looks like:
<aura:component >
<aura:attribute name="context" type="Map" required="true"/>
<aura:handler name="init" value="{!this}" action="{!c.doInit}" />
</aura:component>
THINGSController.js
({
doInit: function(cmp, evt, hlp) {
const things = { FOO: "Foo", BAR: "Bar"};
cmp.get("v.context").addValueProvider("THINGS", {get: ()=>things});
}
})
Now I should be able to reference FOO
like this:
<!-- Value Providers -->
<c:THINGS context="{!this}" />
<!-- Markup -->
<p>{!THINGS.FOO}</p>
The problem I encountered here is, that THINGS.FOO returns the whole Map, what renders to [object Object]
.
When I discovered this, I started playing around and figured out, that THINGS
behaves really weird.
An init handler on my concrete component looks like this:
<aura:handler name="init" value="{!this}" action="{!c.doInit}" />
ctrl (JSON.parse&stringify to get around the proxy issue):
doInit: function(cmp, evt, hlp) {
JSON.parse(JSON.stringify( cmp.get("THINGS.value") ));
JSON.parse(JSON.stringify( cmp.get("THINGS.FOO") ));
JSON.parse(JSON.stringify( cmp.get("THINGS.x") ));
JSON.parse(JSON.stringify( cmp.get("THINGS.value.FOO") ));
JSON.parse(JSON.stringify( cmp.get("THINGS.asdasd.asdasd.asdd.ads") ));
}
The weird thing here is, that they all returned {FOO: "Foo", BAR: "Bar"}
cmp.get("THINGS")
leads to an error message, not knowing what THINGS means.
Conclusion:
Value providers do only work on single values, (or maybe even lists, when we decide to iterate the result.)
cmp.addValueProvider("FOO", {get: ()=>"Foo"});
I hope you agree that this isn't a value provider, it's just a complex way to store a single value.
Quesion:
How are we supposed to use the setValueProvider feature? all I found was this answer that doesn't seem to be fully accurate.
Is there a chance to store simple key value pairs in these providers? if not, why? And where except the Aura Documentation is it documented? All it states is
Adds Custom ValueProviders to a component
Parameters key : String string by which to identify the
valueProvider. Used in expressions in markup, etc.
valueProvider : Object the object to request data from. Must
implement .get(expression), can implement .set(key,value).
lightning-components documentation value-providers
add a comment |
up vote
7
down vote
favorite
To implement something like a public ValueProvider I implemented a component combining the features out there to have static values available in js and markup. Now I'm having trouble using the addValueProvider Feature (used for the markup side) since there is no documentation out there.
To make static values accessible for any component, I need to reference the value providing component in the concrete component like:
<!-- Value Providers -->
<c:THINGS context="{!this}" />
THINGS.cmp looks like:
<aura:component >
<aura:attribute name="context" type="Map" required="true"/>
<aura:handler name="init" value="{!this}" action="{!c.doInit}" />
</aura:component>
THINGSController.js
({
doInit: function(cmp, evt, hlp) {
const things = { FOO: "Foo", BAR: "Bar"};
cmp.get("v.context").addValueProvider("THINGS", {get: ()=>things});
}
})
Now I should be able to reference FOO
like this:
<!-- Value Providers -->
<c:THINGS context="{!this}" />
<!-- Markup -->
<p>{!THINGS.FOO}</p>
The problem I encountered here is, that THINGS.FOO returns the whole Map, what renders to [object Object]
.
When I discovered this, I started playing around and figured out, that THINGS
behaves really weird.
An init handler on my concrete component looks like this:
<aura:handler name="init" value="{!this}" action="{!c.doInit}" />
ctrl (JSON.parse&stringify to get around the proxy issue):
doInit: function(cmp, evt, hlp) {
JSON.parse(JSON.stringify( cmp.get("THINGS.value") ));
JSON.parse(JSON.stringify( cmp.get("THINGS.FOO") ));
JSON.parse(JSON.stringify( cmp.get("THINGS.x") ));
JSON.parse(JSON.stringify( cmp.get("THINGS.value.FOO") ));
JSON.parse(JSON.stringify( cmp.get("THINGS.asdasd.asdasd.asdd.ads") ));
}
The weird thing here is, that they all returned {FOO: "Foo", BAR: "Bar"}
cmp.get("THINGS")
leads to an error message, not knowing what THINGS means.
Conclusion:
Value providers do only work on single values, (or maybe even lists, when we decide to iterate the result.)
cmp.addValueProvider("FOO", {get: ()=>"Foo"});
I hope you agree that this isn't a value provider, it's just a complex way to store a single value.
Quesion:
How are we supposed to use the setValueProvider feature? all I found was this answer that doesn't seem to be fully accurate.
Is there a chance to store simple key value pairs in these providers? if not, why? And where except the Aura Documentation is it documented? All it states is
Adds Custom ValueProviders to a component
Parameters key : String string by which to identify the
valueProvider. Used in expressions in markup, etc.
valueProvider : Object the object to request data from. Must
implement .get(expression), can implement .set(key,value).
lightning-components documentation value-providers
add a comment |
up vote
7
down vote
favorite
up vote
7
down vote
favorite
To implement something like a public ValueProvider I implemented a component combining the features out there to have static values available in js and markup. Now I'm having trouble using the addValueProvider Feature (used for the markup side) since there is no documentation out there.
To make static values accessible for any component, I need to reference the value providing component in the concrete component like:
<!-- Value Providers -->
<c:THINGS context="{!this}" />
THINGS.cmp looks like:
<aura:component >
<aura:attribute name="context" type="Map" required="true"/>
<aura:handler name="init" value="{!this}" action="{!c.doInit}" />
</aura:component>
THINGSController.js
({
doInit: function(cmp, evt, hlp) {
const things = { FOO: "Foo", BAR: "Bar"};
cmp.get("v.context").addValueProvider("THINGS", {get: ()=>things});
}
})
Now I should be able to reference FOO
like this:
<!-- Value Providers -->
<c:THINGS context="{!this}" />
<!-- Markup -->
<p>{!THINGS.FOO}</p>
The problem I encountered here is, that THINGS.FOO returns the whole Map, what renders to [object Object]
.
When I discovered this, I started playing around and figured out, that THINGS
behaves really weird.
An init handler on my concrete component looks like this:
<aura:handler name="init" value="{!this}" action="{!c.doInit}" />
ctrl (JSON.parse&stringify to get around the proxy issue):
doInit: function(cmp, evt, hlp) {
JSON.parse(JSON.stringify( cmp.get("THINGS.value") ));
JSON.parse(JSON.stringify( cmp.get("THINGS.FOO") ));
JSON.parse(JSON.stringify( cmp.get("THINGS.x") ));
JSON.parse(JSON.stringify( cmp.get("THINGS.value.FOO") ));
JSON.parse(JSON.stringify( cmp.get("THINGS.asdasd.asdasd.asdd.ads") ));
}
The weird thing here is, that they all returned {FOO: "Foo", BAR: "Bar"}
cmp.get("THINGS")
leads to an error message, not knowing what THINGS means.
Conclusion:
Value providers do only work on single values, (or maybe even lists, when we decide to iterate the result.)
cmp.addValueProvider("FOO", {get: ()=>"Foo"});
I hope you agree that this isn't a value provider, it's just a complex way to store a single value.
Quesion:
How are we supposed to use the setValueProvider feature? all I found was this answer that doesn't seem to be fully accurate.
Is there a chance to store simple key value pairs in these providers? if not, why? And where except the Aura Documentation is it documented? All it states is
Adds Custom ValueProviders to a component
Parameters key : String string by which to identify the
valueProvider. Used in expressions in markup, etc.
valueProvider : Object the object to request data from. Must
implement .get(expression), can implement .set(key,value).
lightning-components documentation value-providers
To implement something like a public ValueProvider I implemented a component combining the features out there to have static values available in js and markup. Now I'm having trouble using the addValueProvider Feature (used for the markup side) since there is no documentation out there.
To make static values accessible for any component, I need to reference the value providing component in the concrete component like:
<!-- Value Providers -->
<c:THINGS context="{!this}" />
THINGS.cmp looks like:
<aura:component >
<aura:attribute name="context" type="Map" required="true"/>
<aura:handler name="init" value="{!this}" action="{!c.doInit}" />
</aura:component>
THINGSController.js
({
doInit: function(cmp, evt, hlp) {
const things = { FOO: "Foo", BAR: "Bar"};
cmp.get("v.context").addValueProvider("THINGS", {get: ()=>things});
}
})
Now I should be able to reference FOO
like this:
<!-- Value Providers -->
<c:THINGS context="{!this}" />
<!-- Markup -->
<p>{!THINGS.FOO}</p>
The problem I encountered here is, that THINGS.FOO returns the whole Map, what renders to [object Object]
.
When I discovered this, I started playing around and figured out, that THINGS
behaves really weird.
An init handler on my concrete component looks like this:
<aura:handler name="init" value="{!this}" action="{!c.doInit}" />
ctrl (JSON.parse&stringify to get around the proxy issue):
doInit: function(cmp, evt, hlp) {
JSON.parse(JSON.stringify( cmp.get("THINGS.value") ));
JSON.parse(JSON.stringify( cmp.get("THINGS.FOO") ));
JSON.parse(JSON.stringify( cmp.get("THINGS.x") ));
JSON.parse(JSON.stringify( cmp.get("THINGS.value.FOO") ));
JSON.parse(JSON.stringify( cmp.get("THINGS.asdasd.asdasd.asdd.ads") ));
}
The weird thing here is, that they all returned {FOO: "Foo", BAR: "Bar"}
cmp.get("THINGS")
leads to an error message, not knowing what THINGS means.
Conclusion:
Value providers do only work on single values, (or maybe even lists, when we decide to iterate the result.)
cmp.addValueProvider("FOO", {get: ()=>"Foo"});
I hope you agree that this isn't a value provider, it's just a complex way to store a single value.
Quesion:
How are we supposed to use the setValueProvider feature? all I found was this answer that doesn't seem to be fully accurate.
Is there a chance to store simple key value pairs in these providers? if not, why? And where except the Aura Documentation is it documented? All it states is
Adds Custom ValueProviders to a component
Parameters key : String string by which to identify the
valueProvider. Used in expressions in markup, etc.
valueProvider : Object the object to request data from. Must
implement .get(expression), can implement .set(key,value).
lightning-components documentation value-providers
lightning-components documentation value-providers
edited 3 hours ago
asked 3 hours ago


Basti
3,8961949
3,8961949
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
up vote
7
down vote
accepted
The value provider's get method requires a key to retrieve the value from the map/object. So with your value providers get method returning the entire map you will always get the output of [object Object]
. By changing your get method in the addValueProvider function to .addValueProvider("THINGS", {get: (k)=>things[k]});
,
when retrieving {!THINGS.FOO}
you will get Foo
and likewise with {!THINGS.BAR}
you would get Bar
Additionally if you also want to be able to update or add new values to the value provider you need to also include a set method.
.addValueProvider("THINGS", {get: (k)=>things[k], set: (k, v)=>things[k]=v});
Oh, wow, totally missed thatget(expression)
thing in the documentation. Thank you so much, I already gave up on having a proper value provider, you saved my feature :D
– Basti
57 mins ago
No problem. Unfortunately this happens to us all
– Jonathon Chambers
45 mins ago
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
7
down vote
accepted
The value provider's get method requires a key to retrieve the value from the map/object. So with your value providers get method returning the entire map you will always get the output of [object Object]
. By changing your get method in the addValueProvider function to .addValueProvider("THINGS", {get: (k)=>things[k]});
,
when retrieving {!THINGS.FOO}
you will get Foo
and likewise with {!THINGS.BAR}
you would get Bar
Additionally if you also want to be able to update or add new values to the value provider you need to also include a set method.
.addValueProvider("THINGS", {get: (k)=>things[k], set: (k, v)=>things[k]=v});
Oh, wow, totally missed thatget(expression)
thing in the documentation. Thank you so much, I already gave up on having a proper value provider, you saved my feature :D
– Basti
57 mins ago
No problem. Unfortunately this happens to us all
– Jonathon Chambers
45 mins ago
add a comment |
up vote
7
down vote
accepted
The value provider's get method requires a key to retrieve the value from the map/object. So with your value providers get method returning the entire map you will always get the output of [object Object]
. By changing your get method in the addValueProvider function to .addValueProvider("THINGS", {get: (k)=>things[k]});
,
when retrieving {!THINGS.FOO}
you will get Foo
and likewise with {!THINGS.BAR}
you would get Bar
Additionally if you also want to be able to update or add new values to the value provider you need to also include a set method.
.addValueProvider("THINGS", {get: (k)=>things[k], set: (k, v)=>things[k]=v});
Oh, wow, totally missed thatget(expression)
thing in the documentation. Thank you so much, I already gave up on having a proper value provider, you saved my feature :D
– Basti
57 mins ago
No problem. Unfortunately this happens to us all
– Jonathon Chambers
45 mins ago
add a comment |
up vote
7
down vote
accepted
up vote
7
down vote
accepted
The value provider's get method requires a key to retrieve the value from the map/object. So with your value providers get method returning the entire map you will always get the output of [object Object]
. By changing your get method in the addValueProvider function to .addValueProvider("THINGS", {get: (k)=>things[k]});
,
when retrieving {!THINGS.FOO}
you will get Foo
and likewise with {!THINGS.BAR}
you would get Bar
Additionally if you also want to be able to update or add new values to the value provider you need to also include a set method.
.addValueProvider("THINGS", {get: (k)=>things[k], set: (k, v)=>things[k]=v});
The value provider's get method requires a key to retrieve the value from the map/object. So with your value providers get method returning the entire map you will always get the output of [object Object]
. By changing your get method in the addValueProvider function to .addValueProvider("THINGS", {get: (k)=>things[k]});
,
when retrieving {!THINGS.FOO}
you will get Foo
and likewise with {!THINGS.BAR}
you would get Bar
Additionally if you also want to be able to update or add new values to the value provider you need to also include a set method.
.addValueProvider("THINGS", {get: (k)=>things[k], set: (k, v)=>things[k]=v});
answered 1 hour ago


Jonathon Chambers
1788
1788
Oh, wow, totally missed thatget(expression)
thing in the documentation. Thank you so much, I already gave up on having a proper value provider, you saved my feature :D
– Basti
57 mins ago
No problem. Unfortunately this happens to us all
– Jonathon Chambers
45 mins ago
add a comment |
Oh, wow, totally missed thatget(expression)
thing in the documentation. Thank you so much, I already gave up on having a proper value provider, you saved my feature :D
– Basti
57 mins ago
No problem. Unfortunately this happens to us all
– Jonathon Chambers
45 mins ago
Oh, wow, totally missed that
get(expression)
thing in the documentation. Thank you so much, I already gave up on having a proper value provider, you saved my feature :D– Basti
57 mins ago
Oh, wow, totally missed that
get(expression)
thing in the documentation. Thank you so much, I already gave up on having a proper value provider, you saved my feature :D– Basti
57 mins ago
No problem. Unfortunately this happens to us all
– Jonathon Chambers
45 mins ago
No problem. Unfortunately this happens to us all
– Jonathon Chambers
45 mins ago
add a comment |
Thanks for contributing an answer to Salesforce Stack Exchange!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fsalesforce.stackexchange.com%2fquestions%2f242131%2fwhat-is-the-cmp-addvalueprovider-feature-designed-for%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
hGBlk,9aiCo