How to set id of spring repository entry to exact value?
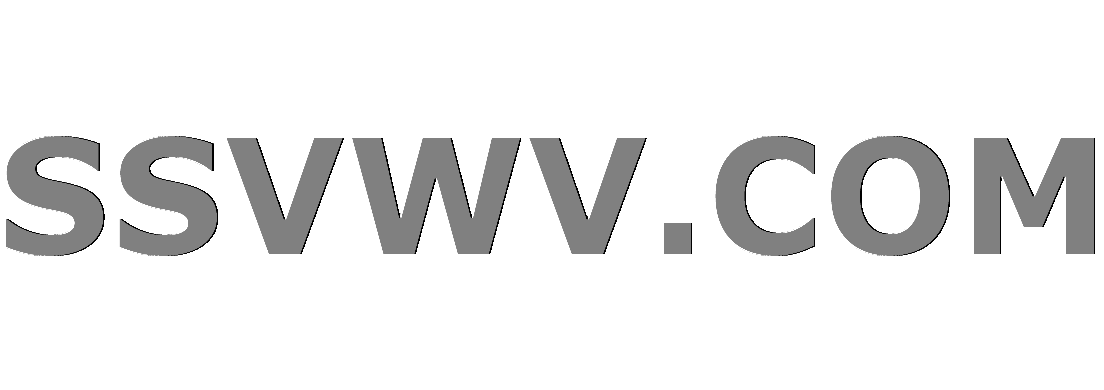
Multi tool use
up vote
1
down vote
favorite
I have a entity class like this:
@Getter
@Setter
@Entity
@AllArgsConstructor
public class Employee{
@Id
private Long id;
private String name;
}
and repository:
public interface EmployeeRepository extends CrudRepository<Employee, Long>{}
Now, in my service class I have:
EmployeeRepository employeeRepository;
public void addEmployee(EmployeeForm employeeForm){
//EmployeeForm is simple inteface
Employee employee = new Employee(employeeForm.getId(), employeeForm.getName());
employeeRepository.save(employee);
}
This will assign some id to the employee, ignoring id I got from EmployeeForm
.
Because I need to import list of employees from some other place, and there is already id assigned to each employee, how can I make EmployeeRepository
to register each employee with id I gave it?
java spring
add a comment |
up vote
1
down vote
favorite
I have a entity class like this:
@Getter
@Setter
@Entity
@AllArgsConstructor
public class Employee{
@Id
private Long id;
private String name;
}
and repository:
public interface EmployeeRepository extends CrudRepository<Employee, Long>{}
Now, in my service class I have:
EmployeeRepository employeeRepository;
public void addEmployee(EmployeeForm employeeForm){
//EmployeeForm is simple inteface
Employee employee = new Employee(employeeForm.getId(), employeeForm.getName());
employeeRepository.save(employee);
}
This will assign some id to the employee, ignoring id I got from EmployeeForm
.
Because I need to import list of employees from some other place, and there is already id assigned to each employee, how can I make EmployeeRepository
to register each employee with id I gave it?
java spring
If you're going to set the Id yourself, you've already done enough, just the @Id annotation is ok.
– Essex Boy
Nov 22 at 15:51
I was missing@NoArgsConstructor
, and in Postman I was supposed to fully match "id" as it was inEmployeeForm
.employeeRepository.save(employee)
works fine with user set ID, it doesn't override it.
– pajkeki
Nov 22 at 16:29
add a comment |
up vote
1
down vote
favorite
up vote
1
down vote
favorite
I have a entity class like this:
@Getter
@Setter
@Entity
@AllArgsConstructor
public class Employee{
@Id
private Long id;
private String name;
}
and repository:
public interface EmployeeRepository extends CrudRepository<Employee, Long>{}
Now, in my service class I have:
EmployeeRepository employeeRepository;
public void addEmployee(EmployeeForm employeeForm){
//EmployeeForm is simple inteface
Employee employee = new Employee(employeeForm.getId(), employeeForm.getName());
employeeRepository.save(employee);
}
This will assign some id to the employee, ignoring id I got from EmployeeForm
.
Because I need to import list of employees from some other place, and there is already id assigned to each employee, how can I make EmployeeRepository
to register each employee with id I gave it?
java spring
I have a entity class like this:
@Getter
@Setter
@Entity
@AllArgsConstructor
public class Employee{
@Id
private Long id;
private String name;
}
and repository:
public interface EmployeeRepository extends CrudRepository<Employee, Long>{}
Now, in my service class I have:
EmployeeRepository employeeRepository;
public void addEmployee(EmployeeForm employeeForm){
//EmployeeForm is simple inteface
Employee employee = new Employee(employeeForm.getId(), employeeForm.getName());
employeeRepository.save(employee);
}
This will assign some id to the employee, ignoring id I got from EmployeeForm
.
Because I need to import list of employees from some other place, and there is already id assigned to each employee, how can I make EmployeeRepository
to register each employee with id I gave it?
java spring
java spring
edited Nov 22 at 16:04
asked Nov 22 at 15:45
pajkeki
104
104
If you're going to set the Id yourself, you've already done enough, just the @Id annotation is ok.
– Essex Boy
Nov 22 at 15:51
I was missing@NoArgsConstructor
, and in Postman I was supposed to fully match "id" as it was inEmployeeForm
.employeeRepository.save(employee)
works fine with user set ID, it doesn't override it.
– pajkeki
Nov 22 at 16:29
add a comment |
If you're going to set the Id yourself, you've already done enough, just the @Id annotation is ok.
– Essex Boy
Nov 22 at 15:51
I was missing@NoArgsConstructor
, and in Postman I was supposed to fully match "id" as it was inEmployeeForm
.employeeRepository.save(employee)
works fine with user set ID, it doesn't override it.
– pajkeki
Nov 22 at 16:29
If you're going to set the Id yourself, you've already done enough, just the @Id annotation is ok.
– Essex Boy
Nov 22 at 15:51
If you're going to set the Id yourself, you've already done enough, just the @Id annotation is ok.
– Essex Boy
Nov 22 at 15:51
I was missing
@NoArgsConstructor
, and in Postman I was supposed to fully match "id" as it was in EmployeeForm
. employeeRepository.save(employee)
works fine with user set ID, it doesn't override it.– pajkeki
Nov 22 at 16:29
I was missing
@NoArgsConstructor
, and in Postman I was supposed to fully match "id" as it was in EmployeeForm
. employeeRepository.save(employee)
works fine with user set ID, it doesn't override it.– pajkeki
Nov 22 at 16:29
add a comment |
2 Answers
2
active
oldest
votes
up vote
1
down vote
accepted
I think that you are missing: @Entity on your Employee Entity, the other should be done by it self.
You can check extra for a difference between long and Long, but this should not cause a problem as it is.
Hope this helps..
Actually, I've already added @Entity, but when you mentioned Long, I remembered that I should be using it instead of long when I'm dealing with repositories.
– pajkeki
Nov 22 at 16:03
is it working now?
– flopcoder
Nov 22 at 16:36
add a comment |
up vote
0
down vote
I was missing @NoArgsConstructor
, and in Postman I was supposed to fully match "id" as it was in EmployeeForm
. employeeRepository.save(employee)
works fine with user set ID, it doesn't override it.
add a comment |
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
accepted
I think that you are missing: @Entity on your Employee Entity, the other should be done by it self.
You can check extra for a difference between long and Long, but this should not cause a problem as it is.
Hope this helps..
Actually, I've already added @Entity, but when you mentioned Long, I remembered that I should be using it instead of long when I'm dealing with repositories.
– pajkeki
Nov 22 at 16:03
is it working now?
– flopcoder
Nov 22 at 16:36
add a comment |
up vote
1
down vote
accepted
I think that you are missing: @Entity on your Employee Entity, the other should be done by it self.
You can check extra for a difference between long and Long, but this should not cause a problem as it is.
Hope this helps..
Actually, I've already added @Entity, but when you mentioned Long, I remembered that I should be using it instead of long when I'm dealing with repositories.
– pajkeki
Nov 22 at 16:03
is it working now?
– flopcoder
Nov 22 at 16:36
add a comment |
up vote
1
down vote
accepted
up vote
1
down vote
accepted
I think that you are missing: @Entity on your Employee Entity, the other should be done by it self.
You can check extra for a difference between long and Long, but this should not cause a problem as it is.
Hope this helps..
I think that you are missing: @Entity on your Employee Entity, the other should be done by it self.
You can check extra for a difference between long and Long, but this should not cause a problem as it is.
Hope this helps..
answered Nov 22 at 15:58


Nenad Vichentikj
719
719
Actually, I've already added @Entity, but when you mentioned Long, I remembered that I should be using it instead of long when I'm dealing with repositories.
– pajkeki
Nov 22 at 16:03
is it working now?
– flopcoder
Nov 22 at 16:36
add a comment |
Actually, I've already added @Entity, but when you mentioned Long, I remembered that I should be using it instead of long when I'm dealing with repositories.
– pajkeki
Nov 22 at 16:03
is it working now?
– flopcoder
Nov 22 at 16:36
Actually, I've already added @Entity, but when you mentioned Long, I remembered that I should be using it instead of long when I'm dealing with repositories.
– pajkeki
Nov 22 at 16:03
Actually, I've already added @Entity, but when you mentioned Long, I remembered that I should be using it instead of long when I'm dealing with repositories.
– pajkeki
Nov 22 at 16:03
is it working now?
– flopcoder
Nov 22 at 16:36
is it working now?
– flopcoder
Nov 22 at 16:36
add a comment |
up vote
0
down vote
I was missing @NoArgsConstructor
, and in Postman I was supposed to fully match "id" as it was in EmployeeForm
. employeeRepository.save(employee)
works fine with user set ID, it doesn't override it.
add a comment |
up vote
0
down vote
I was missing @NoArgsConstructor
, and in Postman I was supposed to fully match "id" as it was in EmployeeForm
. employeeRepository.save(employee)
works fine with user set ID, it doesn't override it.
add a comment |
up vote
0
down vote
up vote
0
down vote
I was missing @NoArgsConstructor
, and in Postman I was supposed to fully match "id" as it was in EmployeeForm
. employeeRepository.save(employee)
works fine with user set ID, it doesn't override it.
I was missing @NoArgsConstructor
, and in Postman I was supposed to fully match "id" as it was in EmployeeForm
. employeeRepository.save(employee)
works fine with user set ID, it doesn't override it.
answered Nov 22 at 16:51
pajkeki
104
104
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53434386%2fhow-to-set-id-of-spring-repository-entry-to-exact-value%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
4 ScWEnYNHMxhtdErU,kGa5,cj0JOhUeogp
If you're going to set the Id yourself, you've already done enough, just the @Id annotation is ok.
– Essex Boy
Nov 22 at 15:51
I was missing
@NoArgsConstructor
, and in Postman I was supposed to fully match "id" as it was inEmployeeForm
.employeeRepository.save(employee)
works fine with user set ID, it doesn't override it.– pajkeki
Nov 22 at 16:29