Using CustomPropertyComparator with java.util.List
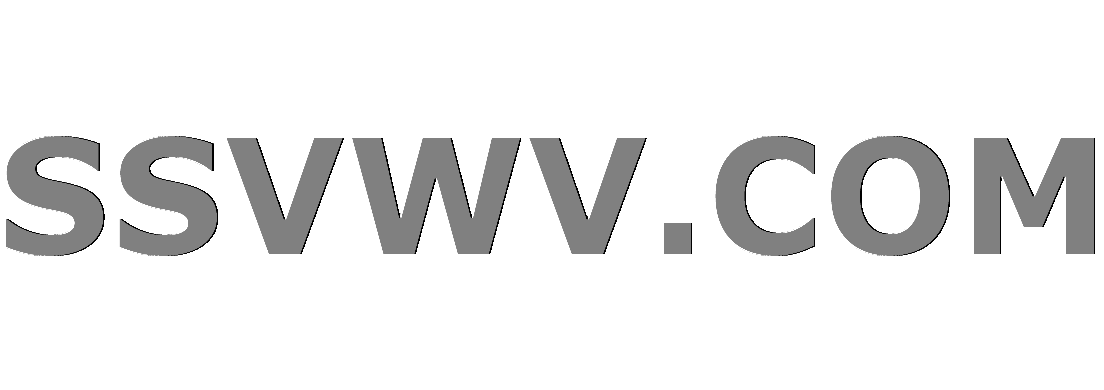
Multi tool use
up vote
0
down vote
favorite
I've created an CustomPropertyComparator to use it to compare Objects which where created by jaxb. So in my case i am not able to use the @DiffIgnore annotation offered by the framework. Also the Collections genereted by jaxb are based on java.util.List and not java.util.Collection. Unfortunatly i couldn't manage to make javers use my CustomPropertyComparator with the List-Interface.
public class Person {
private String name;
private String ignoreThis;
}
public class Company {
private String id;
private Person owner;
private Collection<Person> clients;
private List<Person> partners;
}
Comparator that only compares the name, but ignores the field 'ignoreThis'
public class EntityComparator implements CustomPropertyComparator<Person, ValueChange> {
public ValueChange compare(Person left, Person right, GlobalId affectedId, Property property) {
if (left.getName().equals(right.getName()))
return null;
return new ValueChange(affectedId, "entity/name", left.getName(), right.getName());
}
}
My test-cases looks like this:
This tests works, cause it compares the collection
@Test
public void equalEntityClientTest() {
Person e1 = new Person("james", "ignore this");
Company le1 = new Company("1", null, Arrays.asList(e1), null);
Person e2 = new Person("james", "");
Company le2 = new Company("1", null, Arrays.asList(e2), null);
Diff diff = javers.compare(le1, le2);
System.out.println(diff);
assertEquals(0, diff.getChanges().size());
}
This tests fails, cause it doesn't use my comparator to compare the entity and the diff of the ignored field is true.
@Test
public void equalEntityPartnerTest() {
Person e1 = new Person("james", "ignore this");
Company le1 = new Company("1", e1, null, Arrays.asList(e1));
Person e2 = new Person("james", "");
Company le2 = new Company("1", e2, null, Arrays.asList(e2));
Diff diff = javers.compare(le1, le2);
System.out.println(diff);
assertEquals(0, diff.getChanges().size());
}
In the reference of javers they explain if you have a custom Collection-Interface you need to implement your own comparator, which is okay if you use a collection not based on java.util.Collection. But actually i would expect that java.util.List is supported by the javers Library.
Also i wasn't able to figure out how i can add/create a Comparator for the List-Interface.
Working example can be found under: https://github.com/baumgartner/javerstest
java collections javers
add a comment |
up vote
0
down vote
favorite
I've created an CustomPropertyComparator to use it to compare Objects which where created by jaxb. So in my case i am not able to use the @DiffIgnore annotation offered by the framework. Also the Collections genereted by jaxb are based on java.util.List and not java.util.Collection. Unfortunatly i couldn't manage to make javers use my CustomPropertyComparator with the List-Interface.
public class Person {
private String name;
private String ignoreThis;
}
public class Company {
private String id;
private Person owner;
private Collection<Person> clients;
private List<Person> partners;
}
Comparator that only compares the name, but ignores the field 'ignoreThis'
public class EntityComparator implements CustomPropertyComparator<Person, ValueChange> {
public ValueChange compare(Person left, Person right, GlobalId affectedId, Property property) {
if (left.getName().equals(right.getName()))
return null;
return new ValueChange(affectedId, "entity/name", left.getName(), right.getName());
}
}
My test-cases looks like this:
This tests works, cause it compares the collection
@Test
public void equalEntityClientTest() {
Person e1 = new Person("james", "ignore this");
Company le1 = new Company("1", null, Arrays.asList(e1), null);
Person e2 = new Person("james", "");
Company le2 = new Company("1", null, Arrays.asList(e2), null);
Diff diff = javers.compare(le1, le2);
System.out.println(diff);
assertEquals(0, diff.getChanges().size());
}
This tests fails, cause it doesn't use my comparator to compare the entity and the diff of the ignored field is true.
@Test
public void equalEntityPartnerTest() {
Person e1 = new Person("james", "ignore this");
Company le1 = new Company("1", e1, null, Arrays.asList(e1));
Person e2 = new Person("james", "");
Company le2 = new Company("1", e2, null, Arrays.asList(e2));
Diff diff = javers.compare(le1, le2);
System.out.println(diff);
assertEquals(0, diff.getChanges().size());
}
In the reference of javers they explain if you have a custom Collection-Interface you need to implement your own comparator, which is okay if you use a collection not based on java.util.Collection. But actually i would expect that java.util.List is supported by the javers Library.
Also i wasn't able to figure out how i can add/create a Comparator for the List-Interface.
Working example can be found under: https://github.com/baumgartner/javerstest
java collections javers
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I've created an CustomPropertyComparator to use it to compare Objects which where created by jaxb. So in my case i am not able to use the @DiffIgnore annotation offered by the framework. Also the Collections genereted by jaxb are based on java.util.List and not java.util.Collection. Unfortunatly i couldn't manage to make javers use my CustomPropertyComparator with the List-Interface.
public class Person {
private String name;
private String ignoreThis;
}
public class Company {
private String id;
private Person owner;
private Collection<Person> clients;
private List<Person> partners;
}
Comparator that only compares the name, but ignores the field 'ignoreThis'
public class EntityComparator implements CustomPropertyComparator<Person, ValueChange> {
public ValueChange compare(Person left, Person right, GlobalId affectedId, Property property) {
if (left.getName().equals(right.getName()))
return null;
return new ValueChange(affectedId, "entity/name", left.getName(), right.getName());
}
}
My test-cases looks like this:
This tests works, cause it compares the collection
@Test
public void equalEntityClientTest() {
Person e1 = new Person("james", "ignore this");
Company le1 = new Company("1", null, Arrays.asList(e1), null);
Person e2 = new Person("james", "");
Company le2 = new Company("1", null, Arrays.asList(e2), null);
Diff diff = javers.compare(le1, le2);
System.out.println(diff);
assertEquals(0, diff.getChanges().size());
}
This tests fails, cause it doesn't use my comparator to compare the entity and the diff of the ignored field is true.
@Test
public void equalEntityPartnerTest() {
Person e1 = new Person("james", "ignore this");
Company le1 = new Company("1", e1, null, Arrays.asList(e1));
Person e2 = new Person("james", "");
Company le2 = new Company("1", e2, null, Arrays.asList(e2));
Diff diff = javers.compare(le1, le2);
System.out.println(diff);
assertEquals(0, diff.getChanges().size());
}
In the reference of javers they explain if you have a custom Collection-Interface you need to implement your own comparator, which is okay if you use a collection not based on java.util.Collection. But actually i would expect that java.util.List is supported by the javers Library.
Also i wasn't able to figure out how i can add/create a Comparator for the List-Interface.
Working example can be found under: https://github.com/baumgartner/javerstest
java collections javers
I've created an CustomPropertyComparator to use it to compare Objects which where created by jaxb. So in my case i am not able to use the @DiffIgnore annotation offered by the framework. Also the Collections genereted by jaxb are based on java.util.List and not java.util.Collection. Unfortunatly i couldn't manage to make javers use my CustomPropertyComparator with the List-Interface.
public class Person {
private String name;
private String ignoreThis;
}
public class Company {
private String id;
private Person owner;
private Collection<Person> clients;
private List<Person> partners;
}
Comparator that only compares the name, but ignores the field 'ignoreThis'
public class EntityComparator implements CustomPropertyComparator<Person, ValueChange> {
public ValueChange compare(Person left, Person right, GlobalId affectedId, Property property) {
if (left.getName().equals(right.getName()))
return null;
return new ValueChange(affectedId, "entity/name", left.getName(), right.getName());
}
}
My test-cases looks like this:
This tests works, cause it compares the collection
@Test
public void equalEntityClientTest() {
Person e1 = new Person("james", "ignore this");
Company le1 = new Company("1", null, Arrays.asList(e1), null);
Person e2 = new Person("james", "");
Company le2 = new Company("1", null, Arrays.asList(e2), null);
Diff diff = javers.compare(le1, le2);
System.out.println(diff);
assertEquals(0, diff.getChanges().size());
}
This tests fails, cause it doesn't use my comparator to compare the entity and the diff of the ignored field is true.
@Test
public void equalEntityPartnerTest() {
Person e1 = new Person("james", "ignore this");
Company le1 = new Company("1", e1, null, Arrays.asList(e1));
Person e2 = new Person("james", "");
Company le2 = new Company("1", e2, null, Arrays.asList(e2));
Diff diff = javers.compare(le1, le2);
System.out.println(diff);
assertEquals(0, diff.getChanges().size());
}
In the reference of javers they explain if you have a custom Collection-Interface you need to implement your own comparator, which is okay if you use a collection not based on java.util.Collection. But actually i would expect that java.util.List is supported by the javers Library.
Also i wasn't able to figure out how i can add/create a Comparator for the List-Interface.
Working example can be found under: https://github.com/baumgartner/javerstest
java collections javers
java collections javers
edited 2 days ago
asked Nov 21 at 18:31


Martin Baumgartner
2,1261224
2,1261224
add a comment |
add a comment |
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53418466%2fusing-custompropertycomparator-with-java-util-list%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
JTiAda Z045sy6h6s,ykaHtDlP8FJ1yf2xfcx8Igeu9khEMugPKev2FC,Ay