Preserve EXIF after image resize and rotation then save in python
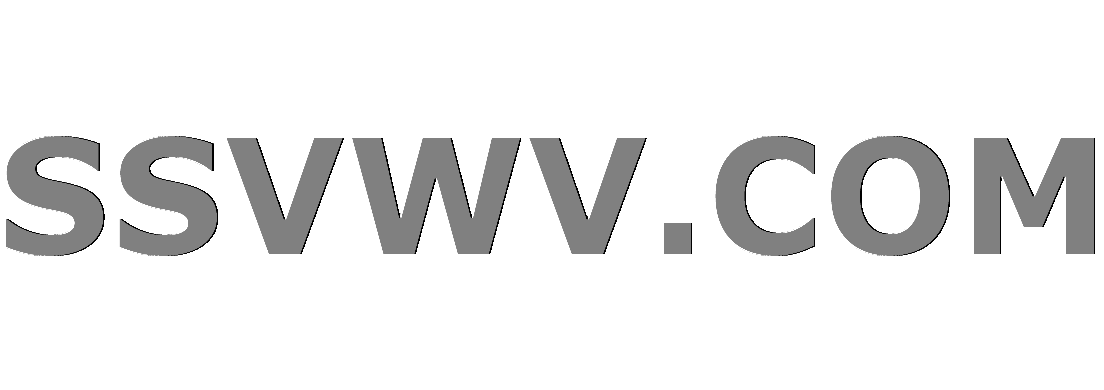
Multi tool use
up vote
0
down vote
favorite
I've read another thread on SO regarding image rotation here:
PIL thumbnail is rotating my image?
and I've read another thread on SO about EXIF preservation here:
Preserve exif data of image with PIL when resize(create thumbnail)
Unfortunately, after implementing the suggestions above, it seems that I can only have either:
1) a saved rotated image without EXIF data
or
2) a non-rotated image with EXIF data
but it seems that I can't have both.
I'm hoping I can get some help to fix what I thought was a real simple problem, but has been turning into a scream-fest against my computer for the past few hours.
Here's the relevant parts of my code:
from PIL import Image, ExifTags
import piexif
currImage = Image.open(inFileName)
exif_dict = piexif.load(currImage.info["exif"])
for orientation in ExifTags.TAGS.keys():
if ExifTags.TAGS[orientation]=='Orientation':
break
exif=dict(currImage._getexif().items())
if exif[orientation] == 3:
currImage=currImage.rotate(180, expand=True)
elif exif[orientation] == 6:
currImage=currImage.rotate(270, expand=True)
elif exif[orientation] == 8:
currImage=currImage.rotate(90, expand=True)
currWidth, currHeight = currImage.size
# Here is where I can only do one or the other. Don't know enough about how to get both
exif_bytes = piexif.dump(exif_dict)
#exif_bytes = piexif.dump(exif)
maxImageDimension = [1280, 640, 360, 160]
for imgDim in maxImageDimension:
thumbRatio = imgDim / max(currWidth, currHeight)
# note that because Python's round function is mathematically incorrect, I have to do the following workaround
newWidth = int(Decimal(str(thumbRatio * currWidth)).quantize(Decimal('0.'), rounding=ROUND_UP))
newHeight = int(Decimal(str(thumbRatio * currHeight)).quantize(Decimal('0.'), rounding=ROUND_UP))
# copy currImage object
newImage = currImage
# note that I have to do resize method because thumbnail method has same rounding problem
newImage = newImage.resize((newWidth, newHeight))
# save the thumbnail
if imgDim == 1280:
outFileName = destinationDir + '\' + file[:len(file)-4] + '.jpg'
else:
outFileName = destinationDir + '\' + file[:len(file)-4] + '-' + str(newWidth) + 'x' + str(newHeight) + '.jpg'
print('Writing: ' + outFileName)
# Here is where I have to choose between exif or exif_bytes when saving but I can only get one or the other desired result
newImage.save(outFileName, exif=exif_bytes)
print('n')
currImage.close()
newImage.close()
Thanks in advance.
python rotation resize python-imaging-library exif
add a comment |
up vote
0
down vote
favorite
I've read another thread on SO regarding image rotation here:
PIL thumbnail is rotating my image?
and I've read another thread on SO about EXIF preservation here:
Preserve exif data of image with PIL when resize(create thumbnail)
Unfortunately, after implementing the suggestions above, it seems that I can only have either:
1) a saved rotated image without EXIF data
or
2) a non-rotated image with EXIF data
but it seems that I can't have both.
I'm hoping I can get some help to fix what I thought was a real simple problem, but has been turning into a scream-fest against my computer for the past few hours.
Here's the relevant parts of my code:
from PIL import Image, ExifTags
import piexif
currImage = Image.open(inFileName)
exif_dict = piexif.load(currImage.info["exif"])
for orientation in ExifTags.TAGS.keys():
if ExifTags.TAGS[orientation]=='Orientation':
break
exif=dict(currImage._getexif().items())
if exif[orientation] == 3:
currImage=currImage.rotate(180, expand=True)
elif exif[orientation] == 6:
currImage=currImage.rotate(270, expand=True)
elif exif[orientation] == 8:
currImage=currImage.rotate(90, expand=True)
currWidth, currHeight = currImage.size
# Here is where I can only do one or the other. Don't know enough about how to get both
exif_bytes = piexif.dump(exif_dict)
#exif_bytes = piexif.dump(exif)
maxImageDimension = [1280, 640, 360, 160]
for imgDim in maxImageDimension:
thumbRatio = imgDim / max(currWidth, currHeight)
# note that because Python's round function is mathematically incorrect, I have to do the following workaround
newWidth = int(Decimal(str(thumbRatio * currWidth)).quantize(Decimal('0.'), rounding=ROUND_UP))
newHeight = int(Decimal(str(thumbRatio * currHeight)).quantize(Decimal('0.'), rounding=ROUND_UP))
# copy currImage object
newImage = currImage
# note that I have to do resize method because thumbnail method has same rounding problem
newImage = newImage.resize((newWidth, newHeight))
# save the thumbnail
if imgDim == 1280:
outFileName = destinationDir + '\' + file[:len(file)-4] + '.jpg'
else:
outFileName = destinationDir + '\' + file[:len(file)-4] + '-' + str(newWidth) + 'x' + str(newHeight) + '.jpg'
print('Writing: ' + outFileName)
# Here is where I have to choose between exif or exif_bytes when saving but I can only get one or the other desired result
newImage.save(outFileName, exif=exif_bytes)
print('n')
currImage.close()
newImage.close()
Thanks in advance.
python rotation resize python-imaging-library exif
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I've read another thread on SO regarding image rotation here:
PIL thumbnail is rotating my image?
and I've read another thread on SO about EXIF preservation here:
Preserve exif data of image with PIL when resize(create thumbnail)
Unfortunately, after implementing the suggestions above, it seems that I can only have either:
1) a saved rotated image without EXIF data
or
2) a non-rotated image with EXIF data
but it seems that I can't have both.
I'm hoping I can get some help to fix what I thought was a real simple problem, but has been turning into a scream-fest against my computer for the past few hours.
Here's the relevant parts of my code:
from PIL import Image, ExifTags
import piexif
currImage = Image.open(inFileName)
exif_dict = piexif.load(currImage.info["exif"])
for orientation in ExifTags.TAGS.keys():
if ExifTags.TAGS[orientation]=='Orientation':
break
exif=dict(currImage._getexif().items())
if exif[orientation] == 3:
currImage=currImage.rotate(180, expand=True)
elif exif[orientation] == 6:
currImage=currImage.rotate(270, expand=True)
elif exif[orientation] == 8:
currImage=currImage.rotate(90, expand=True)
currWidth, currHeight = currImage.size
# Here is where I can only do one or the other. Don't know enough about how to get both
exif_bytes = piexif.dump(exif_dict)
#exif_bytes = piexif.dump(exif)
maxImageDimension = [1280, 640, 360, 160]
for imgDim in maxImageDimension:
thumbRatio = imgDim / max(currWidth, currHeight)
# note that because Python's round function is mathematically incorrect, I have to do the following workaround
newWidth = int(Decimal(str(thumbRatio * currWidth)).quantize(Decimal('0.'), rounding=ROUND_UP))
newHeight = int(Decimal(str(thumbRatio * currHeight)).quantize(Decimal('0.'), rounding=ROUND_UP))
# copy currImage object
newImage = currImage
# note that I have to do resize method because thumbnail method has same rounding problem
newImage = newImage.resize((newWidth, newHeight))
# save the thumbnail
if imgDim == 1280:
outFileName = destinationDir + '\' + file[:len(file)-4] + '.jpg'
else:
outFileName = destinationDir + '\' + file[:len(file)-4] + '-' + str(newWidth) + 'x' + str(newHeight) + '.jpg'
print('Writing: ' + outFileName)
# Here is where I have to choose between exif or exif_bytes when saving but I can only get one or the other desired result
newImage.save(outFileName, exif=exif_bytes)
print('n')
currImage.close()
newImage.close()
Thanks in advance.
python rotation resize python-imaging-library exif
I've read another thread on SO regarding image rotation here:
PIL thumbnail is rotating my image?
and I've read another thread on SO about EXIF preservation here:
Preserve exif data of image with PIL when resize(create thumbnail)
Unfortunately, after implementing the suggestions above, it seems that I can only have either:
1) a saved rotated image without EXIF data
or
2) a non-rotated image with EXIF data
but it seems that I can't have both.
I'm hoping I can get some help to fix what I thought was a real simple problem, but has been turning into a scream-fest against my computer for the past few hours.
Here's the relevant parts of my code:
from PIL import Image, ExifTags
import piexif
currImage = Image.open(inFileName)
exif_dict = piexif.load(currImage.info["exif"])
for orientation in ExifTags.TAGS.keys():
if ExifTags.TAGS[orientation]=='Orientation':
break
exif=dict(currImage._getexif().items())
if exif[orientation] == 3:
currImage=currImage.rotate(180, expand=True)
elif exif[orientation] == 6:
currImage=currImage.rotate(270, expand=True)
elif exif[orientation] == 8:
currImage=currImage.rotate(90, expand=True)
currWidth, currHeight = currImage.size
# Here is where I can only do one or the other. Don't know enough about how to get both
exif_bytes = piexif.dump(exif_dict)
#exif_bytes = piexif.dump(exif)
maxImageDimension = [1280, 640, 360, 160]
for imgDim in maxImageDimension:
thumbRatio = imgDim / max(currWidth, currHeight)
# note that because Python's round function is mathematically incorrect, I have to do the following workaround
newWidth = int(Decimal(str(thumbRatio * currWidth)).quantize(Decimal('0.'), rounding=ROUND_UP))
newHeight = int(Decimal(str(thumbRatio * currHeight)).quantize(Decimal('0.'), rounding=ROUND_UP))
# copy currImage object
newImage = currImage
# note that I have to do resize method because thumbnail method has same rounding problem
newImage = newImage.resize((newWidth, newHeight))
# save the thumbnail
if imgDim == 1280:
outFileName = destinationDir + '\' + file[:len(file)-4] + '.jpg'
else:
outFileName = destinationDir + '\' + file[:len(file)-4] + '-' + str(newWidth) + 'x' + str(newHeight) + '.jpg'
print('Writing: ' + outFileName)
# Here is where I have to choose between exif or exif_bytes when saving but I can only get one or the other desired result
newImage.save(outFileName, exif=exif_bytes)
print('n')
currImage.close()
newImage.close()
Thanks in advance.
python rotation resize python-imaging-library exif
python rotation resize python-imaging-library exif
asked Nov 21 at 23:49


Johnny Cheng
12
12
add a comment |
add a comment |
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53422044%2fpreserve-exif-after-image-resize-and-rotation-then-save-in-python%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
QuZlyzKOPnH7Lgu8i50zfu an3Oc5358aQ,d7y5ps1