passing integers in place of file descriptor when calling write command in c
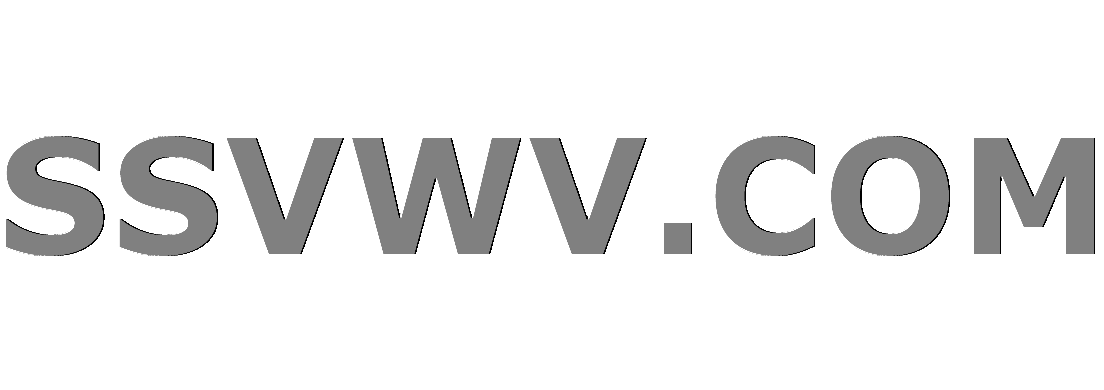
Multi tool use
up vote
0
down vote
favorite
void main()
{
pid_t pid;
int i;
char buf[BUF_SIZE];
fork();
pid = getpid();
for (i = 1; i <= MAX_COUNT; i++) {
sprintf(buf, "This line is from pid %d, value = %dn", pid, i);
write(1, buf, strlen(buf));
// printf("%s",buf);
}
}
I've got the given simple code to show fork process in c. I noticed that when I pass any value greater than 2 in place of 1 in write command the code does not print anything. Why is this happening?
c
add a comment |
up vote
0
down vote
favorite
void main()
{
pid_t pid;
int i;
char buf[BUF_SIZE];
fork();
pid = getpid();
for (i = 1; i <= MAX_COUNT; i++) {
sprintf(buf, "This line is from pid %d, value = %dn", pid, i);
write(1, buf, strlen(buf));
// printf("%s",buf);
}
}
I've got the given simple code to show fork process in c. I noticed that when I pass any value greater than 2 in place of 1 in write command the code does not print anything. Why is this happening?
c
2
You should check thereturn
value ofwrite
for an error.
– Fiddling Bits
Nov 21 at 21:20
1 is for standard output descriptor, 2 is for error. Others aren't open
– Jean-François Fabre
Nov 21 at 21:24
note that thefork
part is irrelevant to the question. The example could be reduced even more to only include awrite
call.
– Jean-François Fabre
Nov 21 at 21:31
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
void main()
{
pid_t pid;
int i;
char buf[BUF_SIZE];
fork();
pid = getpid();
for (i = 1; i <= MAX_COUNT; i++) {
sprintf(buf, "This line is from pid %d, value = %dn", pid, i);
write(1, buf, strlen(buf));
// printf("%s",buf);
}
}
I've got the given simple code to show fork process in c. I noticed that when I pass any value greater than 2 in place of 1 in write command the code does not print anything. Why is this happening?
c
void main()
{
pid_t pid;
int i;
char buf[BUF_SIZE];
fork();
pid = getpid();
for (i = 1; i <= MAX_COUNT; i++) {
sprintf(buf, "This line is from pid %d, value = %dn", pid, i);
write(1, buf, strlen(buf));
// printf("%s",buf);
}
}
I've got the given simple code to show fork process in c. I noticed that when I pass any value greater than 2 in place of 1 in write command the code does not print anything. Why is this happening?
c
c
edited Nov 21 at 21:22


NathanOliver
83k15112173
83k15112173
asked Nov 21 at 21:19
Ashray Sinha
51
51
2
You should check thereturn
value ofwrite
for an error.
– Fiddling Bits
Nov 21 at 21:20
1 is for standard output descriptor, 2 is for error. Others aren't open
– Jean-François Fabre
Nov 21 at 21:24
note that thefork
part is irrelevant to the question. The example could be reduced even more to only include awrite
call.
– Jean-François Fabre
Nov 21 at 21:31
add a comment |
2
You should check thereturn
value ofwrite
for an error.
– Fiddling Bits
Nov 21 at 21:20
1 is for standard output descriptor, 2 is for error. Others aren't open
– Jean-François Fabre
Nov 21 at 21:24
note that thefork
part is irrelevant to the question. The example could be reduced even more to only include awrite
call.
– Jean-François Fabre
Nov 21 at 21:31
2
2
You should check the
return
value of write
for an error.– Fiddling Bits
Nov 21 at 21:20
You should check the
return
value of write
for an error.– Fiddling Bits
Nov 21 at 21:20
1 is for standard output descriptor, 2 is for error. Others aren't open
– Jean-François Fabre
Nov 21 at 21:24
1 is for standard output descriptor, 2 is for error. Others aren't open
– Jean-François Fabre
Nov 21 at 21:24
note that the
fork
part is irrelevant to the question. The example could be reduced even more to only include a write
call.– Jean-François Fabre
Nov 21 at 21:31
note that the
fork
part is irrelevant to the question. The example could be reduced even more to only include a write
call.– Jean-François Fabre
Nov 21 at 21:31
add a comment |
1 Answer
1
active
oldest
votes
up vote
1
down vote
accepted
you're performing a raw write
call where at the start of your program:
- 1 is the standard output descriptor
- 2 is the standard error descriptor
Both write to the console by default (and 0 is the standard input, cannot be written to)
Other descriptors are invalid unless they've been returned by an open
call. Since you don't check the return value of write
, you can't see that an error code is returned (probably EBADF
, bad file descriptor)
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
accepted
you're performing a raw write
call where at the start of your program:
- 1 is the standard output descriptor
- 2 is the standard error descriptor
Both write to the console by default (and 0 is the standard input, cannot be written to)
Other descriptors are invalid unless they've been returned by an open
call. Since you don't check the return value of write
, you can't see that an error code is returned (probably EBADF
, bad file descriptor)
add a comment |
up vote
1
down vote
accepted
you're performing a raw write
call where at the start of your program:
- 1 is the standard output descriptor
- 2 is the standard error descriptor
Both write to the console by default (and 0 is the standard input, cannot be written to)
Other descriptors are invalid unless they've been returned by an open
call. Since you don't check the return value of write
, you can't see that an error code is returned (probably EBADF
, bad file descriptor)
add a comment |
up vote
1
down vote
accepted
up vote
1
down vote
accepted
you're performing a raw write
call where at the start of your program:
- 1 is the standard output descriptor
- 2 is the standard error descriptor
Both write to the console by default (and 0 is the standard input, cannot be written to)
Other descriptors are invalid unless they've been returned by an open
call. Since you don't check the return value of write
, you can't see that an error code is returned (probably EBADF
, bad file descriptor)
you're performing a raw write
call where at the start of your program:
- 1 is the standard output descriptor
- 2 is the standard error descriptor
Both write to the console by default (and 0 is the standard input, cannot be written to)
Other descriptors are invalid unless they've been returned by an open
call. Since you don't check the return value of write
, you can't see that an error code is returned (probably EBADF
, bad file descriptor)
edited Nov 21 at 21:30
answered Nov 21 at 21:25


Jean-François Fabre
97.9k950107
97.9k950107
add a comment |
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53420606%2fpassing-integers-in-place-of-file-descriptor-when-calling-write-command-in-c%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
0GFNQ4,H1,Tfp UO0voJZH4l,CbKLtfXDV8,vTUwXVRyti
2
You should check the
return
value ofwrite
for an error.– Fiddling Bits
Nov 21 at 21:20
1 is for standard output descriptor, 2 is for error. Others aren't open
– Jean-François Fabre
Nov 21 at 21:24
note that the
fork
part is irrelevant to the question. The example could be reduced even more to only include awrite
call.– Jean-François Fabre
Nov 21 at 21:31