Reverse Sort a stream
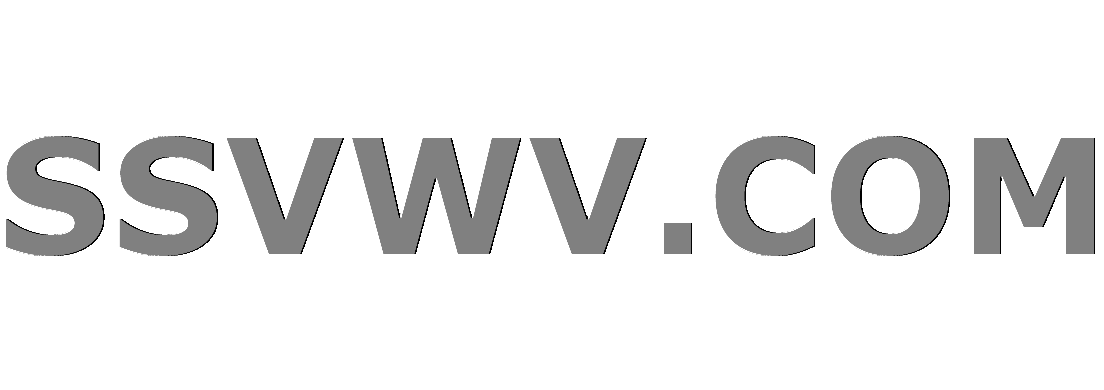
Multi tool use
up vote
7
down vote
favorite
I want to reverse sort a stream such as below but getting compile time error as "The method sorted() in the type IntStream is not applicable for the arguments (( o1, o2) -> {})". Can anyone correct this
IntStream.range(1, 100)
.filter(x -> x%2 != 0)
.sorted((o1,o2) -> -o1.compareTo(o2))
.forEach(System.out::println);
java sorting java-8 java-stream
add a comment |
up vote
7
down vote
favorite
I want to reverse sort a stream such as below but getting compile time error as "The method sorted() in the type IntStream is not applicable for the arguments (( o1, o2) -> {})". Can anyone correct this
IntStream.range(1, 100)
.filter(x -> x%2 != 0)
.sorted((o1,o2) -> -o1.compareTo(o2))
.forEach(System.out::println);
java sorting java-8 java-stream
I'm curious as to why you would use streams for something like this. It would seem to only add a bunch of overhead.
– JimmyJames
7 mins ago
add a comment |
up vote
7
down vote
favorite
up vote
7
down vote
favorite
I want to reverse sort a stream such as below but getting compile time error as "The method sorted() in the type IntStream is not applicable for the arguments (( o1, o2) -> {})". Can anyone correct this
IntStream.range(1, 100)
.filter(x -> x%2 != 0)
.sorted((o1,o2) -> -o1.compareTo(o2))
.forEach(System.out::println);
java sorting java-8 java-stream
I want to reverse sort a stream such as below but getting compile time error as "The method sorted() in the type IntStream is not applicable for the arguments (( o1, o2) -> {})". Can anyone correct this
IntStream.range(1, 100)
.filter(x -> x%2 != 0)
.sorted((o1,o2) -> -o1.compareTo(o2))
.forEach(System.out::println);
java sorting java-8 java-stream
java sorting java-8 java-stream
edited 1 hour ago


Nicholas K
4,85641031
4,85641031
asked 2 hours ago
Sundresh
612
612
I'm curious as to why you would use streams for something like this. It would seem to only add a bunch of overhead.
– JimmyJames
7 mins ago
add a comment |
I'm curious as to why you would use streams for something like this. It would seem to only add a bunch of overhead.
– JimmyJames
7 mins ago
I'm curious as to why you would use streams for something like this. It would seem to only add a bunch of overhead.
– JimmyJames
7 mins ago
I'm curious as to why you would use streams for something like this. It would seem to only add a bunch of overhead.
– JimmyJames
7 mins ago
add a comment |
3 Answers
3
active
oldest
votes
up vote
8
down vote
since you're working with an IntStream
it only has one overload of the sorted method and it's the natural order (which makes sense).
instead, box the stream from IntStream
to Stream<Integer>
then it should suffice:
IntStream.range(1, 100)
.filter(x -> x % 2 != 0)
.boxed() // <--- boxed to Stream<Integer>
.sorted((o1,o2) -> -o1.compareTo(o2)) // now we can call compareTo on Integer
.forEach(System.out::println);
A better approach would be:
IntStream.range(1, 100)
.filter(x -> x % 2 != 0)
.boxed()
.sorted(Comparator.reverseOrder())
.forEachOrdered(System.out::println);
why?
- There's already a built-in comparator to perform reverse order as shown above.
- if you want to guarantee that the elements are to be seen in the sorted order when printing then utilise
forEachOrdered
(big shout out to @Holger for always reminding me)
btw, in JDK9 you can simplify this to:
IntStream.iterate(1, i -> i <= 99, i -> i + 2)
.boxed()
.sorted(Comparator.reverseOrder())
.forEachOrdered(System.out::println);
With this approach, we can avoid the filter intermediate operation and increment in 2's.
and finally you could simplify it even further with:
IntStream.iterate(99, i -> i > 0 , i -> i - 2)
.forEachOrdered(System.out::println);
With this approach, we can avoid filter
, boxed
,sorted
et al.
When we are at usual reminders, never use a comparator function like(o1,o2) -> -o1.compareTo(o2)
. It is perfectly legal for acompareTo
implementation to returnInteger.MIN_VALUE
, in which case negation will fail and produce inconsistent results. A valid reverse comparator would be(o1,o2) -> o2.compareTo(o1)
, or justComparator.reverseOrder()
as you have shown, which does already the job right. By the way, I wouldn’t useiterate
but ratherIntStream.range(0, 50).map(i -> 99-i*2)
which is more efficient in a lot of cases.
– Holger
7 mins ago
add a comment |
up vote
4
down vote
If that is your real code, then it may be more efficient to use IntStream.iterate
and generate numbers from 99
to 0
:
IntStream.iterate(99, i -> i - 1)
.limit(100)
.filter(x -> x % 2 != 0)
.forEachOrdered(System.out::println);
add a comment |
up vote
2
down vote
How about simple util such as :
private IntStream reverseSort(int from, int to) {
return IntStream.range(from, to)
.filter(x -> x % 2 != 0)
.sorted()
.map(i -> to - i + from - 1);
}
Credits: Stuart Marks for the reverse util.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53764661%2freverse-sort-a-stream%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
8
down vote
since you're working with an IntStream
it only has one overload of the sorted method and it's the natural order (which makes sense).
instead, box the stream from IntStream
to Stream<Integer>
then it should suffice:
IntStream.range(1, 100)
.filter(x -> x % 2 != 0)
.boxed() // <--- boxed to Stream<Integer>
.sorted((o1,o2) -> -o1.compareTo(o2)) // now we can call compareTo on Integer
.forEach(System.out::println);
A better approach would be:
IntStream.range(1, 100)
.filter(x -> x % 2 != 0)
.boxed()
.sorted(Comparator.reverseOrder())
.forEachOrdered(System.out::println);
why?
- There's already a built-in comparator to perform reverse order as shown above.
- if you want to guarantee that the elements are to be seen in the sorted order when printing then utilise
forEachOrdered
(big shout out to @Holger for always reminding me)
btw, in JDK9 you can simplify this to:
IntStream.iterate(1, i -> i <= 99, i -> i + 2)
.boxed()
.sorted(Comparator.reverseOrder())
.forEachOrdered(System.out::println);
With this approach, we can avoid the filter intermediate operation and increment in 2's.
and finally you could simplify it even further with:
IntStream.iterate(99, i -> i > 0 , i -> i - 2)
.forEachOrdered(System.out::println);
With this approach, we can avoid filter
, boxed
,sorted
et al.
When we are at usual reminders, never use a comparator function like(o1,o2) -> -o1.compareTo(o2)
. It is perfectly legal for acompareTo
implementation to returnInteger.MIN_VALUE
, in which case negation will fail and produce inconsistent results. A valid reverse comparator would be(o1,o2) -> o2.compareTo(o1)
, or justComparator.reverseOrder()
as you have shown, which does already the job right. By the way, I wouldn’t useiterate
but ratherIntStream.range(0, 50).map(i -> 99-i*2)
which is more efficient in a lot of cases.
– Holger
7 mins ago
add a comment |
up vote
8
down vote
since you're working with an IntStream
it only has one overload of the sorted method and it's the natural order (which makes sense).
instead, box the stream from IntStream
to Stream<Integer>
then it should suffice:
IntStream.range(1, 100)
.filter(x -> x % 2 != 0)
.boxed() // <--- boxed to Stream<Integer>
.sorted((o1,o2) -> -o1.compareTo(o2)) // now we can call compareTo on Integer
.forEach(System.out::println);
A better approach would be:
IntStream.range(1, 100)
.filter(x -> x % 2 != 0)
.boxed()
.sorted(Comparator.reverseOrder())
.forEachOrdered(System.out::println);
why?
- There's already a built-in comparator to perform reverse order as shown above.
- if you want to guarantee that the elements are to be seen in the sorted order when printing then utilise
forEachOrdered
(big shout out to @Holger for always reminding me)
btw, in JDK9 you can simplify this to:
IntStream.iterate(1, i -> i <= 99, i -> i + 2)
.boxed()
.sorted(Comparator.reverseOrder())
.forEachOrdered(System.out::println);
With this approach, we can avoid the filter intermediate operation and increment in 2's.
and finally you could simplify it even further with:
IntStream.iterate(99, i -> i > 0 , i -> i - 2)
.forEachOrdered(System.out::println);
With this approach, we can avoid filter
, boxed
,sorted
et al.
When we are at usual reminders, never use a comparator function like(o1,o2) -> -o1.compareTo(o2)
. It is perfectly legal for acompareTo
implementation to returnInteger.MIN_VALUE
, in which case negation will fail and produce inconsistent results. A valid reverse comparator would be(o1,o2) -> o2.compareTo(o1)
, or justComparator.reverseOrder()
as you have shown, which does already the job right. By the way, I wouldn’t useiterate
but ratherIntStream.range(0, 50).map(i -> 99-i*2)
which is more efficient in a lot of cases.
– Holger
7 mins ago
add a comment |
up vote
8
down vote
up vote
8
down vote
since you're working with an IntStream
it only has one overload of the sorted method and it's the natural order (which makes sense).
instead, box the stream from IntStream
to Stream<Integer>
then it should suffice:
IntStream.range(1, 100)
.filter(x -> x % 2 != 0)
.boxed() // <--- boxed to Stream<Integer>
.sorted((o1,o2) -> -o1.compareTo(o2)) // now we can call compareTo on Integer
.forEach(System.out::println);
A better approach would be:
IntStream.range(1, 100)
.filter(x -> x % 2 != 0)
.boxed()
.sorted(Comparator.reverseOrder())
.forEachOrdered(System.out::println);
why?
- There's already a built-in comparator to perform reverse order as shown above.
- if you want to guarantee that the elements are to be seen in the sorted order when printing then utilise
forEachOrdered
(big shout out to @Holger for always reminding me)
btw, in JDK9 you can simplify this to:
IntStream.iterate(1, i -> i <= 99, i -> i + 2)
.boxed()
.sorted(Comparator.reverseOrder())
.forEachOrdered(System.out::println);
With this approach, we can avoid the filter intermediate operation and increment in 2's.
and finally you could simplify it even further with:
IntStream.iterate(99, i -> i > 0 , i -> i - 2)
.forEachOrdered(System.out::println);
With this approach, we can avoid filter
, boxed
,sorted
et al.
since you're working with an IntStream
it only has one overload of the sorted method and it's the natural order (which makes sense).
instead, box the stream from IntStream
to Stream<Integer>
then it should suffice:
IntStream.range(1, 100)
.filter(x -> x % 2 != 0)
.boxed() // <--- boxed to Stream<Integer>
.sorted((o1,o2) -> -o1.compareTo(o2)) // now we can call compareTo on Integer
.forEach(System.out::println);
A better approach would be:
IntStream.range(1, 100)
.filter(x -> x % 2 != 0)
.boxed()
.sorted(Comparator.reverseOrder())
.forEachOrdered(System.out::println);
why?
- There's already a built-in comparator to perform reverse order as shown above.
- if you want to guarantee that the elements are to be seen in the sorted order when printing then utilise
forEachOrdered
(big shout out to @Holger for always reminding me)
btw, in JDK9 you can simplify this to:
IntStream.iterate(1, i -> i <= 99, i -> i + 2)
.boxed()
.sorted(Comparator.reverseOrder())
.forEachOrdered(System.out::println);
With this approach, we can avoid the filter intermediate operation and increment in 2's.
and finally you could simplify it even further with:
IntStream.iterate(99, i -> i > 0 , i -> i - 2)
.forEachOrdered(System.out::println);
With this approach, we can avoid filter
, boxed
,sorted
et al.
edited 1 hour ago
answered 2 hours ago


Aomine
36k62960
36k62960
When we are at usual reminders, never use a comparator function like(o1,o2) -> -o1.compareTo(o2)
. It is perfectly legal for acompareTo
implementation to returnInteger.MIN_VALUE
, in which case negation will fail and produce inconsistent results. A valid reverse comparator would be(o1,o2) -> o2.compareTo(o1)
, or justComparator.reverseOrder()
as you have shown, which does already the job right. By the way, I wouldn’t useiterate
but ratherIntStream.range(0, 50).map(i -> 99-i*2)
which is more efficient in a lot of cases.
– Holger
7 mins ago
add a comment |
When we are at usual reminders, never use a comparator function like(o1,o2) -> -o1.compareTo(o2)
. It is perfectly legal for acompareTo
implementation to returnInteger.MIN_VALUE
, in which case negation will fail and produce inconsistent results. A valid reverse comparator would be(o1,o2) -> o2.compareTo(o1)
, or justComparator.reverseOrder()
as you have shown, which does already the job right. By the way, I wouldn’t useiterate
but ratherIntStream.range(0, 50).map(i -> 99-i*2)
which is more efficient in a lot of cases.
– Holger
7 mins ago
When we are at usual reminders, never use a comparator function like
(o1,o2) -> -o1.compareTo(o2)
. It is perfectly legal for a compareTo
implementation to return Integer.MIN_VALUE
, in which case negation will fail and produce inconsistent results. A valid reverse comparator would be (o1,o2) -> o2.compareTo(o1)
, or just Comparator.reverseOrder()
as you have shown, which does already the job right. By the way, I wouldn’t use iterate
but rather IntStream.range(0, 50).map(i -> 99-i*2)
which is more efficient in a lot of cases.– Holger
7 mins ago
When we are at usual reminders, never use a comparator function like
(o1,o2) -> -o1.compareTo(o2)
. It is perfectly legal for a compareTo
implementation to return Integer.MIN_VALUE
, in which case negation will fail and produce inconsistent results. A valid reverse comparator would be (o1,o2) -> o2.compareTo(o1)
, or just Comparator.reverseOrder()
as you have shown, which does already the job right. By the way, I wouldn’t use iterate
but rather IntStream.range(0, 50).map(i -> 99-i*2)
which is more efficient in a lot of cases.– Holger
7 mins ago
add a comment |
up vote
4
down vote
If that is your real code, then it may be more efficient to use IntStream.iterate
and generate numbers from 99
to 0
:
IntStream.iterate(99, i -> i - 1)
.limit(100)
.filter(x -> x % 2 != 0)
.forEachOrdered(System.out::println);
add a comment |
up vote
4
down vote
If that is your real code, then it may be more efficient to use IntStream.iterate
and generate numbers from 99
to 0
:
IntStream.iterate(99, i -> i - 1)
.limit(100)
.filter(x -> x % 2 != 0)
.forEachOrdered(System.out::println);
add a comment |
up vote
4
down vote
up vote
4
down vote
If that is your real code, then it may be more efficient to use IntStream.iterate
and generate numbers from 99
to 0
:
IntStream.iterate(99, i -> i - 1)
.limit(100)
.filter(x -> x % 2 != 0)
.forEachOrdered(System.out::println);
If that is your real code, then it may be more efficient to use IntStream.iterate
and generate numbers from 99
to 0
:
IntStream.iterate(99, i -> i - 1)
.limit(100)
.filter(x -> x % 2 != 0)
.forEachOrdered(System.out::println);
answered 2 hours ago
ernest_k
18.8k41838
18.8k41838
add a comment |
add a comment |
up vote
2
down vote
How about simple util such as :
private IntStream reverseSort(int from, int to) {
return IntStream.range(from, to)
.filter(x -> x % 2 != 0)
.sorted()
.map(i -> to - i + from - 1);
}
Credits: Stuart Marks for the reverse util.
add a comment |
up vote
2
down vote
How about simple util such as :
private IntStream reverseSort(int from, int to) {
return IntStream.range(from, to)
.filter(x -> x % 2 != 0)
.sorted()
.map(i -> to - i + from - 1);
}
Credits: Stuart Marks for the reverse util.
add a comment |
up vote
2
down vote
up vote
2
down vote
How about simple util such as :
private IntStream reverseSort(int from, int to) {
return IntStream.range(from, to)
.filter(x -> x % 2 != 0)
.sorted()
.map(i -> to - i + from - 1);
}
Credits: Stuart Marks for the reverse util.
How about simple util such as :
private IntStream reverseSort(int from, int to) {
return IntStream.range(from, to)
.filter(x -> x % 2 != 0)
.sorted()
.map(i -> to - i + from - 1);
}
Credits: Stuart Marks for the reverse util.
edited 1 hour ago
answered 1 hour ago


nullpointer
38.3k1073146
38.3k1073146
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53764661%2freverse-sort-a-stream%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
LyXpWC1 cOgA4kNe7ht,KIPp,ZRyMGNZBOma4Ua,sIROJilWcKcYfIFfZjhMF,U4os,MENEgyiO,s
I'm curious as to why you would use streams for something like this. It would seem to only add a bunch of overhead.
– JimmyJames
7 mins ago