Nested UserControl event doesn't work with EventTrigger/InvokeCommandAction in MVVM/WPF scenario
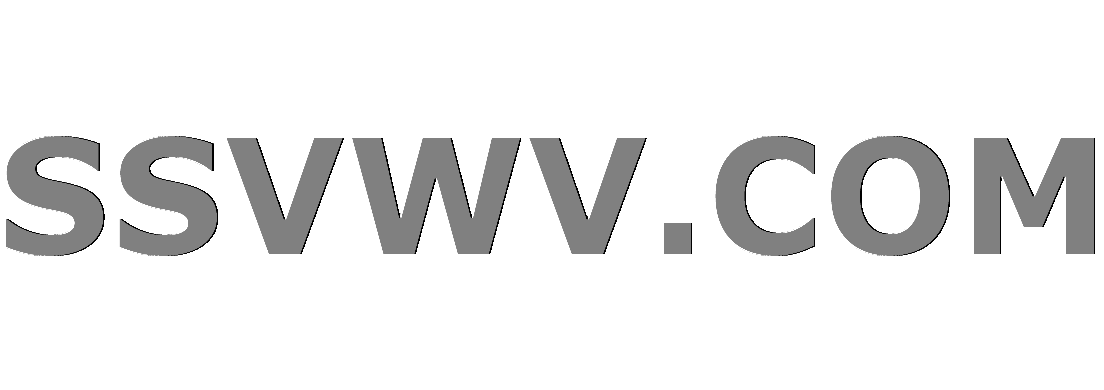
Multi tool use
up vote
2
down vote
favorite
I'm working with WPF with Prism (MVVM), and trying to build an Inspector for a few classes. One of those classes is Vector3:
<Grid x:Name="Vector3Root" Background="White">
<StackPanel Orientation="Horizontal">
<StackPanel Orientation="Horizontal">
<xctk:DoubleUpDown Tag="X" Style="{StaticResource DoubleUpDownStyle}" Value="{Binding X}" ValueChanged="Vector3ValueChanged"/>
</StackPanel>
<StackPanel Orientation="Horizontal">
<xctk:DoubleUpDown Tag="Y" Style="{StaticResource DoubleUpDownStyle}" Value="{Binding Y}" ValueChanged="Vector3ValueChanged"/>
</StackPanel>
<StackPanel Orientation="Horizontal">
<xctk:DoubleUpDown Tag="Z" Style="{StaticResource DoubleUpDownStyle}" Value="{Binding Z}" ValueChanged="Vector3ValueChanged"/>
</StackPanel>
</StackPanel>
</Grid>
And its code-behind
namespace SimROV.WPF.Views{
public partial class Vector3View : UserControl
{
public Vector3View()
{
InitializeComponent();
}
public static readonly RoutedEvent SettingConfirmedEvent =
EventManager.RegisterRoutedEvent("SettingConfirmed", RoutingStrategy.Bubble,
typeof(RoutedEventHandler), typeof(Vector3View));
public event RoutedEventHandler SettingConfirmed
{
add { AddHandler(SettingConfirmedEvent, value); }
remove { RemoveHandler(SettingConfirmedEvent, value); }
}
public void Vector3ValueChanged(object sender, RoutedPropertyChangedEventArgs<object> e)
{
RaiseEvent(new RoutedEventArgs(SettingConfirmedEvent));
}
}}
The problem that I'm struggling with is that I can't catch neither of the fired events (ValueChanged
or SettingConfirmed
) on another UserControl
's ViewModel that is using Vector3View
:
<UserControl
x:Class="SimROV.WPF.Views.TransformView"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:views="clr-namespace:SimROV.WPF.Views"
xmlns:i="http://schemas.microsoft.com/expression/2010/interactivity"
xmlns:ei="http://schemas.microsoft.com/expression/2010/interactions"
xmlns:prism="http://prismlibrary.com/"
mc:Ignorable="d" >
<Grid x:Name="TransformRoot" Background="White">
<StackPanel Orientation="Vertical">
<StackPanel Orientation="Horizontal">
<TextBlock Text="Position" Margin="5"/>
<!--<ContentPresenter ContentTemplate="{StaticResource Vector3Template}"/>-->
<views:Vector3View x:Name="PositionVector3">
<i:Interaction.Triggers>
<i:EventTrigger EventName="SettingConfirmed">
<prism:InvokeCommandAction Command="{Binding PositionValueChangedCommand}"/>
</i:EventTrigger>
</i:Interaction.Triggers>
</views:Vector3View>
</StackPanel>
<StackPanel Orientation="Horizontal">
<TextBlock Text="Rotation" Margin="5"/>
<!--<ContentPresenter ContentTemplate="{StaticResource Vector3Template}"/>-->
<views:Vector3View x:Name="RotationVector3" SettingConfirmed="RotationValueChangedEvent"/>
</StackPanel>
</StackPanel>
</Grid>
At this point I CAN catch SettingConfirmed
with RotationValueChangedEvent
on code-behind, but since I'm following MVVM pattern, that doesn't work for me, which is why I'm using EventTrigger
and InvokeCommandAction
to catch those events on TransformViewModel
, but those never get fired.
Here it's the TransformViewModel
:
namespace SimROV.WPF.ViewModels{
public class TransformViewModel : BindableBase
{
private ICommand _positionCommand;
public ICommand PositionValueChangedCommand => this._positionCommand ?? (this._positionCommand = new DelegateCommand(PositionChanged));
private void PositionChanged()
{
}
public TransformViewModel()
{
}
}}
PositionChanged
just never gets fired and I can't understand why at all.
I don't know if this is relevant, but Transform is an element of an ObservableCollection<IComponent>
at another ViewModel, which is being presented by a ListView
with a ItemContainerStyle
, that has a ContentPresenter
with a ContentTemplateSelector inside.
Can someone point me out on why this is happening and how to fix it?
Thank you.
c# wpf events mvvm prism
add a comment |
up vote
2
down vote
favorite
I'm working with WPF with Prism (MVVM), and trying to build an Inspector for a few classes. One of those classes is Vector3:
<Grid x:Name="Vector3Root" Background="White">
<StackPanel Orientation="Horizontal">
<StackPanel Orientation="Horizontal">
<xctk:DoubleUpDown Tag="X" Style="{StaticResource DoubleUpDownStyle}" Value="{Binding X}" ValueChanged="Vector3ValueChanged"/>
</StackPanel>
<StackPanel Orientation="Horizontal">
<xctk:DoubleUpDown Tag="Y" Style="{StaticResource DoubleUpDownStyle}" Value="{Binding Y}" ValueChanged="Vector3ValueChanged"/>
</StackPanel>
<StackPanel Orientation="Horizontal">
<xctk:DoubleUpDown Tag="Z" Style="{StaticResource DoubleUpDownStyle}" Value="{Binding Z}" ValueChanged="Vector3ValueChanged"/>
</StackPanel>
</StackPanel>
</Grid>
And its code-behind
namespace SimROV.WPF.Views{
public partial class Vector3View : UserControl
{
public Vector3View()
{
InitializeComponent();
}
public static readonly RoutedEvent SettingConfirmedEvent =
EventManager.RegisterRoutedEvent("SettingConfirmed", RoutingStrategy.Bubble,
typeof(RoutedEventHandler), typeof(Vector3View));
public event RoutedEventHandler SettingConfirmed
{
add { AddHandler(SettingConfirmedEvent, value); }
remove { RemoveHandler(SettingConfirmedEvent, value); }
}
public void Vector3ValueChanged(object sender, RoutedPropertyChangedEventArgs<object> e)
{
RaiseEvent(new RoutedEventArgs(SettingConfirmedEvent));
}
}}
The problem that I'm struggling with is that I can't catch neither of the fired events (ValueChanged
or SettingConfirmed
) on another UserControl
's ViewModel that is using Vector3View
:
<UserControl
x:Class="SimROV.WPF.Views.TransformView"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:views="clr-namespace:SimROV.WPF.Views"
xmlns:i="http://schemas.microsoft.com/expression/2010/interactivity"
xmlns:ei="http://schemas.microsoft.com/expression/2010/interactions"
xmlns:prism="http://prismlibrary.com/"
mc:Ignorable="d" >
<Grid x:Name="TransformRoot" Background="White">
<StackPanel Orientation="Vertical">
<StackPanel Orientation="Horizontal">
<TextBlock Text="Position" Margin="5"/>
<!--<ContentPresenter ContentTemplate="{StaticResource Vector3Template}"/>-->
<views:Vector3View x:Name="PositionVector3">
<i:Interaction.Triggers>
<i:EventTrigger EventName="SettingConfirmed">
<prism:InvokeCommandAction Command="{Binding PositionValueChangedCommand}"/>
</i:EventTrigger>
</i:Interaction.Triggers>
</views:Vector3View>
</StackPanel>
<StackPanel Orientation="Horizontal">
<TextBlock Text="Rotation" Margin="5"/>
<!--<ContentPresenter ContentTemplate="{StaticResource Vector3Template}"/>-->
<views:Vector3View x:Name="RotationVector3" SettingConfirmed="RotationValueChangedEvent"/>
</StackPanel>
</StackPanel>
</Grid>
At this point I CAN catch SettingConfirmed
with RotationValueChangedEvent
on code-behind, but since I'm following MVVM pattern, that doesn't work for me, which is why I'm using EventTrigger
and InvokeCommandAction
to catch those events on TransformViewModel
, but those never get fired.
Here it's the TransformViewModel
:
namespace SimROV.WPF.ViewModels{
public class TransformViewModel : BindableBase
{
private ICommand _positionCommand;
public ICommand PositionValueChangedCommand => this._positionCommand ?? (this._positionCommand = new DelegateCommand(PositionChanged));
private void PositionChanged()
{
}
public TransformViewModel()
{
}
}}
PositionChanged
just never gets fired and I can't understand why at all.
I don't know if this is relevant, but Transform is an element of an ObservableCollection<IComponent>
at another ViewModel, which is being presented by a ListView
with a ItemContainerStyle
, that has a ContentPresenter
with a ContentTemplateSelector inside.
Can someone point me out on why this is happening and how to fix it?
Thank you.
c# wpf events mvvm prism
What's the DataContext of the Vector3View? Probably not a TransformViewModel.
– mm8
Nov 22 at 14:40
Just realized I did forget to add prism:ViewModelLocator.AutoWireViewModel="True" to both TransformView and Vector3View, but that didn't result in anything new. As a matter of fact, Vector3 will just be like a property in many different classes, so I cant hardcode it to Transform. Is it possible to set its DataContext programatically from another UserControl?
– Trmotta
Nov 22 at 15:25
Setting <views:Vector3View x:Name="PositionVector3" DataContext="{Binding RelativeSource={RelativeSource Self}}"> doens't make a difference either
– Trmotta
Nov 22 at 15:32
Why would you set the DataContext of the UserControl to itself? You need to set it to an instance of TransformViewModel for the binding to the command to work.
– mm8
Nov 22 at 15:33
I'm really sorry! I'm still new to WPF/Prism and thus having this kind of silly mistakes. Setting PositionVector3.DataContext to this.DataContext at TransformView.xaml.cs solved my issue. Thank you deeply for your help!
– Trmotta
Nov 22 at 16:22
add a comment |
up vote
2
down vote
favorite
up vote
2
down vote
favorite
I'm working with WPF with Prism (MVVM), and trying to build an Inspector for a few classes. One of those classes is Vector3:
<Grid x:Name="Vector3Root" Background="White">
<StackPanel Orientation="Horizontal">
<StackPanel Orientation="Horizontal">
<xctk:DoubleUpDown Tag="X" Style="{StaticResource DoubleUpDownStyle}" Value="{Binding X}" ValueChanged="Vector3ValueChanged"/>
</StackPanel>
<StackPanel Orientation="Horizontal">
<xctk:DoubleUpDown Tag="Y" Style="{StaticResource DoubleUpDownStyle}" Value="{Binding Y}" ValueChanged="Vector3ValueChanged"/>
</StackPanel>
<StackPanel Orientation="Horizontal">
<xctk:DoubleUpDown Tag="Z" Style="{StaticResource DoubleUpDownStyle}" Value="{Binding Z}" ValueChanged="Vector3ValueChanged"/>
</StackPanel>
</StackPanel>
</Grid>
And its code-behind
namespace SimROV.WPF.Views{
public partial class Vector3View : UserControl
{
public Vector3View()
{
InitializeComponent();
}
public static readonly RoutedEvent SettingConfirmedEvent =
EventManager.RegisterRoutedEvent("SettingConfirmed", RoutingStrategy.Bubble,
typeof(RoutedEventHandler), typeof(Vector3View));
public event RoutedEventHandler SettingConfirmed
{
add { AddHandler(SettingConfirmedEvent, value); }
remove { RemoveHandler(SettingConfirmedEvent, value); }
}
public void Vector3ValueChanged(object sender, RoutedPropertyChangedEventArgs<object> e)
{
RaiseEvent(new RoutedEventArgs(SettingConfirmedEvent));
}
}}
The problem that I'm struggling with is that I can't catch neither of the fired events (ValueChanged
or SettingConfirmed
) on another UserControl
's ViewModel that is using Vector3View
:
<UserControl
x:Class="SimROV.WPF.Views.TransformView"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:views="clr-namespace:SimROV.WPF.Views"
xmlns:i="http://schemas.microsoft.com/expression/2010/interactivity"
xmlns:ei="http://schemas.microsoft.com/expression/2010/interactions"
xmlns:prism="http://prismlibrary.com/"
mc:Ignorable="d" >
<Grid x:Name="TransformRoot" Background="White">
<StackPanel Orientation="Vertical">
<StackPanel Orientation="Horizontal">
<TextBlock Text="Position" Margin="5"/>
<!--<ContentPresenter ContentTemplate="{StaticResource Vector3Template}"/>-->
<views:Vector3View x:Name="PositionVector3">
<i:Interaction.Triggers>
<i:EventTrigger EventName="SettingConfirmed">
<prism:InvokeCommandAction Command="{Binding PositionValueChangedCommand}"/>
</i:EventTrigger>
</i:Interaction.Triggers>
</views:Vector3View>
</StackPanel>
<StackPanel Orientation="Horizontal">
<TextBlock Text="Rotation" Margin="5"/>
<!--<ContentPresenter ContentTemplate="{StaticResource Vector3Template}"/>-->
<views:Vector3View x:Name="RotationVector3" SettingConfirmed="RotationValueChangedEvent"/>
</StackPanel>
</StackPanel>
</Grid>
At this point I CAN catch SettingConfirmed
with RotationValueChangedEvent
on code-behind, but since I'm following MVVM pattern, that doesn't work for me, which is why I'm using EventTrigger
and InvokeCommandAction
to catch those events on TransformViewModel
, but those never get fired.
Here it's the TransformViewModel
:
namespace SimROV.WPF.ViewModels{
public class TransformViewModel : BindableBase
{
private ICommand _positionCommand;
public ICommand PositionValueChangedCommand => this._positionCommand ?? (this._positionCommand = new DelegateCommand(PositionChanged));
private void PositionChanged()
{
}
public TransformViewModel()
{
}
}}
PositionChanged
just never gets fired and I can't understand why at all.
I don't know if this is relevant, but Transform is an element of an ObservableCollection<IComponent>
at another ViewModel, which is being presented by a ListView
with a ItemContainerStyle
, that has a ContentPresenter
with a ContentTemplateSelector inside.
Can someone point me out on why this is happening and how to fix it?
Thank you.
c# wpf events mvvm prism
I'm working with WPF with Prism (MVVM), and trying to build an Inspector for a few classes. One of those classes is Vector3:
<Grid x:Name="Vector3Root" Background="White">
<StackPanel Orientation="Horizontal">
<StackPanel Orientation="Horizontal">
<xctk:DoubleUpDown Tag="X" Style="{StaticResource DoubleUpDownStyle}" Value="{Binding X}" ValueChanged="Vector3ValueChanged"/>
</StackPanel>
<StackPanel Orientation="Horizontal">
<xctk:DoubleUpDown Tag="Y" Style="{StaticResource DoubleUpDownStyle}" Value="{Binding Y}" ValueChanged="Vector3ValueChanged"/>
</StackPanel>
<StackPanel Orientation="Horizontal">
<xctk:DoubleUpDown Tag="Z" Style="{StaticResource DoubleUpDownStyle}" Value="{Binding Z}" ValueChanged="Vector3ValueChanged"/>
</StackPanel>
</StackPanel>
</Grid>
And its code-behind
namespace SimROV.WPF.Views{
public partial class Vector3View : UserControl
{
public Vector3View()
{
InitializeComponent();
}
public static readonly RoutedEvent SettingConfirmedEvent =
EventManager.RegisterRoutedEvent("SettingConfirmed", RoutingStrategy.Bubble,
typeof(RoutedEventHandler), typeof(Vector3View));
public event RoutedEventHandler SettingConfirmed
{
add { AddHandler(SettingConfirmedEvent, value); }
remove { RemoveHandler(SettingConfirmedEvent, value); }
}
public void Vector3ValueChanged(object sender, RoutedPropertyChangedEventArgs<object> e)
{
RaiseEvent(new RoutedEventArgs(SettingConfirmedEvent));
}
}}
The problem that I'm struggling with is that I can't catch neither of the fired events (ValueChanged
or SettingConfirmed
) on another UserControl
's ViewModel that is using Vector3View
:
<UserControl
x:Class="SimROV.WPF.Views.TransformView"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:views="clr-namespace:SimROV.WPF.Views"
xmlns:i="http://schemas.microsoft.com/expression/2010/interactivity"
xmlns:ei="http://schemas.microsoft.com/expression/2010/interactions"
xmlns:prism="http://prismlibrary.com/"
mc:Ignorable="d" >
<Grid x:Name="TransformRoot" Background="White">
<StackPanel Orientation="Vertical">
<StackPanel Orientation="Horizontal">
<TextBlock Text="Position" Margin="5"/>
<!--<ContentPresenter ContentTemplate="{StaticResource Vector3Template}"/>-->
<views:Vector3View x:Name="PositionVector3">
<i:Interaction.Triggers>
<i:EventTrigger EventName="SettingConfirmed">
<prism:InvokeCommandAction Command="{Binding PositionValueChangedCommand}"/>
</i:EventTrigger>
</i:Interaction.Triggers>
</views:Vector3View>
</StackPanel>
<StackPanel Orientation="Horizontal">
<TextBlock Text="Rotation" Margin="5"/>
<!--<ContentPresenter ContentTemplate="{StaticResource Vector3Template}"/>-->
<views:Vector3View x:Name="RotationVector3" SettingConfirmed="RotationValueChangedEvent"/>
</StackPanel>
</StackPanel>
</Grid>
At this point I CAN catch SettingConfirmed
with RotationValueChangedEvent
on code-behind, but since I'm following MVVM pattern, that doesn't work for me, which is why I'm using EventTrigger
and InvokeCommandAction
to catch those events on TransformViewModel
, but those never get fired.
Here it's the TransformViewModel
:
namespace SimROV.WPF.ViewModels{
public class TransformViewModel : BindableBase
{
private ICommand _positionCommand;
public ICommand PositionValueChangedCommand => this._positionCommand ?? (this._positionCommand = new DelegateCommand(PositionChanged));
private void PositionChanged()
{
}
public TransformViewModel()
{
}
}}
PositionChanged
just never gets fired and I can't understand why at all.
I don't know if this is relevant, but Transform is an element of an ObservableCollection<IComponent>
at another ViewModel, which is being presented by a ListView
with a ItemContainerStyle
, that has a ContentPresenter
with a ContentTemplateSelector inside.
Can someone point me out on why this is happening and how to fix it?
Thank you.
c# wpf events mvvm prism
c# wpf events mvvm prism
edited Nov 22 at 16:29


mm8
80.2k81831
80.2k81831
asked Nov 22 at 14:09
Trmotta
12829
12829
What's the DataContext of the Vector3View? Probably not a TransformViewModel.
– mm8
Nov 22 at 14:40
Just realized I did forget to add prism:ViewModelLocator.AutoWireViewModel="True" to both TransformView and Vector3View, but that didn't result in anything new. As a matter of fact, Vector3 will just be like a property in many different classes, so I cant hardcode it to Transform. Is it possible to set its DataContext programatically from another UserControl?
– Trmotta
Nov 22 at 15:25
Setting <views:Vector3View x:Name="PositionVector3" DataContext="{Binding RelativeSource={RelativeSource Self}}"> doens't make a difference either
– Trmotta
Nov 22 at 15:32
Why would you set the DataContext of the UserControl to itself? You need to set it to an instance of TransformViewModel for the binding to the command to work.
– mm8
Nov 22 at 15:33
I'm really sorry! I'm still new to WPF/Prism and thus having this kind of silly mistakes. Setting PositionVector3.DataContext to this.DataContext at TransformView.xaml.cs solved my issue. Thank you deeply for your help!
– Trmotta
Nov 22 at 16:22
add a comment |
What's the DataContext of the Vector3View? Probably not a TransformViewModel.
– mm8
Nov 22 at 14:40
Just realized I did forget to add prism:ViewModelLocator.AutoWireViewModel="True" to both TransformView and Vector3View, but that didn't result in anything new. As a matter of fact, Vector3 will just be like a property in many different classes, so I cant hardcode it to Transform. Is it possible to set its DataContext programatically from another UserControl?
– Trmotta
Nov 22 at 15:25
Setting <views:Vector3View x:Name="PositionVector3" DataContext="{Binding RelativeSource={RelativeSource Self}}"> doens't make a difference either
– Trmotta
Nov 22 at 15:32
Why would you set the DataContext of the UserControl to itself? You need to set it to an instance of TransformViewModel for the binding to the command to work.
– mm8
Nov 22 at 15:33
I'm really sorry! I'm still new to WPF/Prism and thus having this kind of silly mistakes. Setting PositionVector3.DataContext to this.DataContext at TransformView.xaml.cs solved my issue. Thank you deeply for your help!
– Trmotta
Nov 22 at 16:22
What's the DataContext of the Vector3View? Probably not a TransformViewModel.
– mm8
Nov 22 at 14:40
What's the DataContext of the Vector3View? Probably not a TransformViewModel.
– mm8
Nov 22 at 14:40
Just realized I did forget to add prism:ViewModelLocator.AutoWireViewModel="True" to both TransformView and Vector3View, but that didn't result in anything new. As a matter of fact, Vector3 will just be like a property in many different classes, so I cant hardcode it to Transform. Is it possible to set its DataContext programatically from another UserControl?
– Trmotta
Nov 22 at 15:25
Just realized I did forget to add prism:ViewModelLocator.AutoWireViewModel="True" to both TransformView and Vector3View, but that didn't result in anything new. As a matter of fact, Vector3 will just be like a property in many different classes, so I cant hardcode it to Transform. Is it possible to set its DataContext programatically from another UserControl?
– Trmotta
Nov 22 at 15:25
Setting <views:Vector3View x:Name="PositionVector3" DataContext="{Binding RelativeSource={RelativeSource Self}}"> doens't make a difference either
– Trmotta
Nov 22 at 15:32
Setting <views:Vector3View x:Name="PositionVector3" DataContext="{Binding RelativeSource={RelativeSource Self}}"> doens't make a difference either
– Trmotta
Nov 22 at 15:32
Why would you set the DataContext of the UserControl to itself? You need to set it to an instance of TransformViewModel for the binding to the command to work.
– mm8
Nov 22 at 15:33
Why would you set the DataContext of the UserControl to itself? You need to set it to an instance of TransformViewModel for the binding to the command to work.
– mm8
Nov 22 at 15:33
I'm really sorry! I'm still new to WPF/Prism and thus having this kind of silly mistakes. Setting PositionVector3.DataContext to this.DataContext at TransformView.xaml.cs solved my issue. Thank you deeply for your help!
– Trmotta
Nov 22 at 16:22
I'm really sorry! I'm still new to WPF/Prism and thus having this kind of silly mistakes. Setting PositionVector3.DataContext to this.DataContext at TransformView.xaml.cs solved my issue. Thank you deeply for your help!
– Trmotta
Nov 22 at 16:22
add a comment |
1 Answer
1
active
oldest
votes
up vote
2
down vote
accepted
Your EventTrigger
and InvokeCommandAction
should work just fine provided that the DataContext
of the Vector3View
actually is a TransformViewModel
so the binding to the PositionValueChangedCommand
property succeeds.
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
2
down vote
accepted
Your EventTrigger
and InvokeCommandAction
should work just fine provided that the DataContext
of the Vector3View
actually is a TransformViewModel
so the binding to the PositionValueChangedCommand
property succeeds.
add a comment |
up vote
2
down vote
accepted
Your EventTrigger
and InvokeCommandAction
should work just fine provided that the DataContext
of the Vector3View
actually is a TransformViewModel
so the binding to the PositionValueChangedCommand
property succeeds.
add a comment |
up vote
2
down vote
accepted
up vote
2
down vote
accepted
Your EventTrigger
and InvokeCommandAction
should work just fine provided that the DataContext
of the Vector3View
actually is a TransformViewModel
so the binding to the PositionValueChangedCommand
property succeeds.
Your EventTrigger
and InvokeCommandAction
should work just fine provided that the DataContext
of the Vector3View
actually is a TransformViewModel
so the binding to the PositionValueChangedCommand
property succeeds.
answered Nov 22 at 16:24


mm8
80.2k81831
80.2k81831
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53432795%2fnested-usercontrol-event-doesnt-work-with-eventtrigger-invokecommandaction-in-m%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
zgmyN1NlIAUlQhLZmrGnCZAmomW UU,Y8,osU OFW2crMNRcE 1liSd6ydv kUev6K6 Oq6M01kOcV
What's the DataContext of the Vector3View? Probably not a TransformViewModel.
– mm8
Nov 22 at 14:40
Just realized I did forget to add prism:ViewModelLocator.AutoWireViewModel="True" to both TransformView and Vector3View, but that didn't result in anything new. As a matter of fact, Vector3 will just be like a property in many different classes, so I cant hardcode it to Transform. Is it possible to set its DataContext programatically from another UserControl?
– Trmotta
Nov 22 at 15:25
Setting <views:Vector3View x:Name="PositionVector3" DataContext="{Binding RelativeSource={RelativeSource Self}}"> doens't make a difference either
– Trmotta
Nov 22 at 15:32
Why would you set the DataContext of the UserControl to itself? You need to set it to an instance of TransformViewModel for the binding to the command to work.
– mm8
Nov 22 at 15:33
I'm really sorry! I'm still new to WPF/Prism and thus having this kind of silly mistakes. Setting PositionVector3.DataContext to this.DataContext at TransformView.xaml.cs solved my issue. Thank you deeply for your help!
– Trmotta
Nov 22 at 16:22