How can I keep my value from an if-statement?
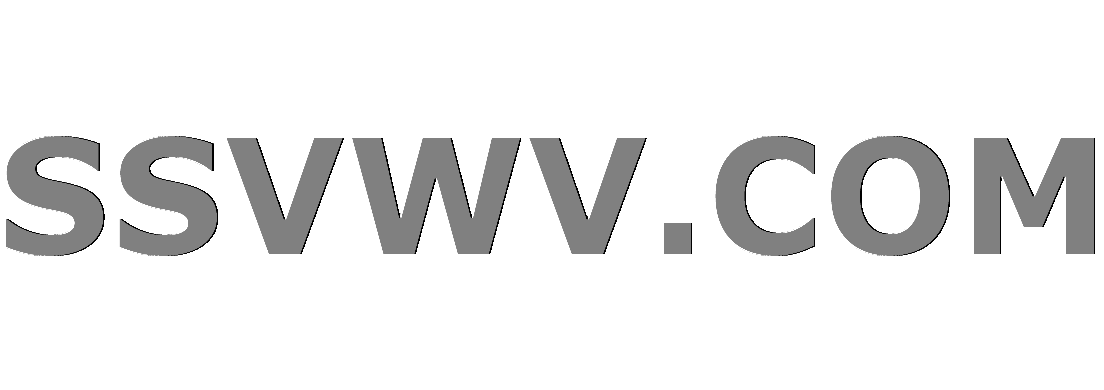
Multi tool use
up vote
2
down vote
favorite
I tried making a very basic calculator, but if I try to output the calculated result I get the error that my variable "result" has not been initialised, even though I did initialise it inside the if-statement at the bottom. When I put the "System.out" line in my if-statement it works, so I have been wondering how can initialise a variable in my statement and keep the value.
But all I found were threads that talked about using "return" to give out a value, but not a variable.
import javax.swing.JOptionPane;
class Calculator{
public static void main(String args)
{
double z1, z2, result;
String input, s;
input = JOptionPane.showInputDialog("Input a number:");
//check whether or not the input is a number
if(isNumber(input) == false)
{
JOptionPane.showMessageDialog(null, "Input a real Number", "Error",JOptionPane.ERROR_MESSAGE);
System.exit(0);
}
z1 = Double.parseDouble(input);
input = JOptionPane.showInputDialog("Input a second number:");
//check whether or not the input is a number
if(isNumber(input) == false)
{
JOptionPane.showMessageDialog(null, "Input a real number", "Error",JOptionPane.ERROR_MESSAGE);
System.exit(0);
}
z2 = Double.parseDouble(input);
s = JOptionPane.showInputDialog("Input an operation(+,-,*,/):");
if(s.equals("+"))
{ result = z1 + z2; }
if(s.equals("-"))
{ result = z1 - z2; }
if(s.equals("*"))
{ result = z1 * z2; }
if(s.equals("/"))
{ result = z1 / z2; }
System.out.println(result);
java if-statement double
add a comment |
up vote
2
down vote
favorite
I tried making a very basic calculator, but if I try to output the calculated result I get the error that my variable "result" has not been initialised, even though I did initialise it inside the if-statement at the bottom. When I put the "System.out" line in my if-statement it works, so I have been wondering how can initialise a variable in my statement and keep the value.
But all I found were threads that talked about using "return" to give out a value, but not a variable.
import javax.swing.JOptionPane;
class Calculator{
public static void main(String args)
{
double z1, z2, result;
String input, s;
input = JOptionPane.showInputDialog("Input a number:");
//check whether or not the input is a number
if(isNumber(input) == false)
{
JOptionPane.showMessageDialog(null, "Input a real Number", "Error",JOptionPane.ERROR_MESSAGE);
System.exit(0);
}
z1 = Double.parseDouble(input);
input = JOptionPane.showInputDialog("Input a second number:");
//check whether or not the input is a number
if(isNumber(input) == false)
{
JOptionPane.showMessageDialog(null, "Input a real number", "Error",JOptionPane.ERROR_MESSAGE);
System.exit(0);
}
z2 = Double.parseDouble(input);
s = JOptionPane.showInputDialog("Input an operation(+,-,*,/):");
if(s.equals("+"))
{ result = z1 + z2; }
if(s.equals("-"))
{ result = z1 - z2; }
if(s.equals("*"))
{ result = z1 * z2; }
if(s.equals("/"))
{ result = z1 / z2; }
System.out.println(result);
java if-statement double
s
might not be +, -, * or /. Ifs
isn't one of those, thenresult
is still undefined.
– Elliott Frisch
Nov 22 at 16:51
yeah, but when inputting I'm making sure to use a simple "+" or "-"so it shouldn't be a problem. I mean it works if I use system.out.println(result) inside my if-statement
– Awais
Nov 22 at 16:54
Can you set a breakpoint to System.out.println(result) and check what the exact value of result is?
– Lajos Arpad
Nov 22 at 16:55
What are your values and what is the output at the end?
– Lajos Arpad
Nov 22 at 16:56
Sorry, but I'm not using a compiler. I'm just using a text editor and try to compile things through the windows console. I don't think i can set breakpoints there.
– Awais
Nov 22 at 17:00
add a comment |
up vote
2
down vote
favorite
up vote
2
down vote
favorite
I tried making a very basic calculator, but if I try to output the calculated result I get the error that my variable "result" has not been initialised, even though I did initialise it inside the if-statement at the bottom. When I put the "System.out" line in my if-statement it works, so I have been wondering how can initialise a variable in my statement and keep the value.
But all I found were threads that talked about using "return" to give out a value, but not a variable.
import javax.swing.JOptionPane;
class Calculator{
public static void main(String args)
{
double z1, z2, result;
String input, s;
input = JOptionPane.showInputDialog("Input a number:");
//check whether or not the input is a number
if(isNumber(input) == false)
{
JOptionPane.showMessageDialog(null, "Input a real Number", "Error",JOptionPane.ERROR_MESSAGE);
System.exit(0);
}
z1 = Double.parseDouble(input);
input = JOptionPane.showInputDialog("Input a second number:");
//check whether or not the input is a number
if(isNumber(input) == false)
{
JOptionPane.showMessageDialog(null, "Input a real number", "Error",JOptionPane.ERROR_MESSAGE);
System.exit(0);
}
z2 = Double.parseDouble(input);
s = JOptionPane.showInputDialog("Input an operation(+,-,*,/):");
if(s.equals("+"))
{ result = z1 + z2; }
if(s.equals("-"))
{ result = z1 - z2; }
if(s.equals("*"))
{ result = z1 * z2; }
if(s.equals("/"))
{ result = z1 / z2; }
System.out.println(result);
java if-statement double
I tried making a very basic calculator, but if I try to output the calculated result I get the error that my variable "result" has not been initialised, even though I did initialise it inside the if-statement at the bottom. When I put the "System.out" line in my if-statement it works, so I have been wondering how can initialise a variable in my statement and keep the value.
But all I found were threads that talked about using "return" to give out a value, but not a variable.
import javax.swing.JOptionPane;
class Calculator{
public static void main(String args)
{
double z1, z2, result;
String input, s;
input = JOptionPane.showInputDialog("Input a number:");
//check whether or not the input is a number
if(isNumber(input) == false)
{
JOptionPane.showMessageDialog(null, "Input a real Number", "Error",JOptionPane.ERROR_MESSAGE);
System.exit(0);
}
z1 = Double.parseDouble(input);
input = JOptionPane.showInputDialog("Input a second number:");
//check whether or not the input is a number
if(isNumber(input) == false)
{
JOptionPane.showMessageDialog(null, "Input a real number", "Error",JOptionPane.ERROR_MESSAGE);
System.exit(0);
}
z2 = Double.parseDouble(input);
s = JOptionPane.showInputDialog("Input an operation(+,-,*,/):");
if(s.equals("+"))
{ result = z1 + z2; }
if(s.equals("-"))
{ result = z1 - z2; }
if(s.equals("*"))
{ result = z1 * z2; }
if(s.equals("/"))
{ result = z1 / z2; }
System.out.println(result);
java if-statement double
java if-statement double
edited Nov 22 at 17:21
asked Nov 22 at 16:49
Awais
225
225
s
might not be +, -, * or /. Ifs
isn't one of those, thenresult
is still undefined.
– Elliott Frisch
Nov 22 at 16:51
yeah, but when inputting I'm making sure to use a simple "+" or "-"so it shouldn't be a problem. I mean it works if I use system.out.println(result) inside my if-statement
– Awais
Nov 22 at 16:54
Can you set a breakpoint to System.out.println(result) and check what the exact value of result is?
– Lajos Arpad
Nov 22 at 16:55
What are your values and what is the output at the end?
– Lajos Arpad
Nov 22 at 16:56
Sorry, but I'm not using a compiler. I'm just using a text editor and try to compile things through the windows console. I don't think i can set breakpoints there.
– Awais
Nov 22 at 17:00
add a comment |
s
might not be +, -, * or /. Ifs
isn't one of those, thenresult
is still undefined.
– Elliott Frisch
Nov 22 at 16:51
yeah, but when inputting I'm making sure to use a simple "+" or "-"so it shouldn't be a problem. I mean it works if I use system.out.println(result) inside my if-statement
– Awais
Nov 22 at 16:54
Can you set a breakpoint to System.out.println(result) and check what the exact value of result is?
– Lajos Arpad
Nov 22 at 16:55
What are your values and what is the output at the end?
– Lajos Arpad
Nov 22 at 16:56
Sorry, but I'm not using a compiler. I'm just using a text editor and try to compile things through the windows console. I don't think i can set breakpoints there.
– Awais
Nov 22 at 17:00
s
might not be +, -, * or /. If s
isn't one of those, then result
is still undefined.– Elliott Frisch
Nov 22 at 16:51
s
might not be +, -, * or /. If s
isn't one of those, then result
is still undefined.– Elliott Frisch
Nov 22 at 16:51
yeah, but when inputting I'm making sure to use a simple "+" or "-"so it shouldn't be a problem. I mean it works if I use system.out.println(result) inside my if-statement
– Awais
Nov 22 at 16:54
yeah, but when inputting I'm making sure to use a simple "+" or "-"so it shouldn't be a problem. I mean it works if I use system.out.println(result) inside my if-statement
– Awais
Nov 22 at 16:54
Can you set a breakpoint to System.out.println(result) and check what the exact value of result is?
– Lajos Arpad
Nov 22 at 16:55
Can you set a breakpoint to System.out.println(result) and check what the exact value of result is?
– Lajos Arpad
Nov 22 at 16:55
What are your values and what is the output at the end?
– Lajos Arpad
Nov 22 at 16:56
What are your values and what is the output at the end?
– Lajos Arpad
Nov 22 at 16:56
Sorry, but I'm not using a compiler. I'm just using a text editor and try to compile things through the windows console. I don't think i can set breakpoints there.
– Awais
Nov 22 at 17:00
Sorry, but I'm not using a compiler. I'm just using a text editor and try to compile things through the windows console. I don't think i can set breakpoints there.
– Awais
Nov 22 at 17:00
add a comment |
2 Answers
2
active
oldest
votes
up vote
3
down vote
accepted
Since this code:
if(s.equals("+"))
{ result = z1 + z2; }
if(s.equals("-"))
{ result = z1 - z2; }
if(s.equals("*"))
{ result = z1 * z2; }
if(s.equals("/"))
{ result = z1 / z2; }
does not have a final else
statement it is not guaranteed that result
will be initialized.
One way to overcome this problem:
if (s.equals("+")) {
result = z1 + z2;
} else if (s.equals("-")) {
result = z1 - z2;
} else if (s.equals("*")) {
result = z1 * z2;
} else if (s.equals("/")) {
result = z1 / z2;
} else {
result = 0.0;
}
or in the definition:
double result = 0.0;
add a comment |
up vote
1
down vote
The problem is that the environment checks whether everything is okay and sees that you have a few ifs where the variable might be initialized and finds that result
is not guaranteed to be initialized. As a result you get this error. Try to initialize it at the start:
double z1, z2, result = 0;
@Awais if this answer solved your problem, then you might consider accepting it as the correct answer. The other answer is correct as well.
– Lajos Arpad
Nov 22 at 17:04
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53435360%2fhow-can-i-keep-my-value-from-an-if-statement%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
3
down vote
accepted
Since this code:
if(s.equals("+"))
{ result = z1 + z2; }
if(s.equals("-"))
{ result = z1 - z2; }
if(s.equals("*"))
{ result = z1 * z2; }
if(s.equals("/"))
{ result = z1 / z2; }
does not have a final else
statement it is not guaranteed that result
will be initialized.
One way to overcome this problem:
if (s.equals("+")) {
result = z1 + z2;
} else if (s.equals("-")) {
result = z1 - z2;
} else if (s.equals("*")) {
result = z1 * z2;
} else if (s.equals("/")) {
result = z1 / z2;
} else {
result = 0.0;
}
or in the definition:
double result = 0.0;
add a comment |
up vote
3
down vote
accepted
Since this code:
if(s.equals("+"))
{ result = z1 + z2; }
if(s.equals("-"))
{ result = z1 - z2; }
if(s.equals("*"))
{ result = z1 * z2; }
if(s.equals("/"))
{ result = z1 / z2; }
does not have a final else
statement it is not guaranteed that result
will be initialized.
One way to overcome this problem:
if (s.equals("+")) {
result = z1 + z2;
} else if (s.equals("-")) {
result = z1 - z2;
} else if (s.equals("*")) {
result = z1 * z2;
} else if (s.equals("/")) {
result = z1 / z2;
} else {
result = 0.0;
}
or in the definition:
double result = 0.0;
add a comment |
up vote
3
down vote
accepted
up vote
3
down vote
accepted
Since this code:
if(s.equals("+"))
{ result = z1 + z2; }
if(s.equals("-"))
{ result = z1 - z2; }
if(s.equals("*"))
{ result = z1 * z2; }
if(s.equals("/"))
{ result = z1 / z2; }
does not have a final else
statement it is not guaranteed that result
will be initialized.
One way to overcome this problem:
if (s.equals("+")) {
result = z1 + z2;
} else if (s.equals("-")) {
result = z1 - z2;
} else if (s.equals("*")) {
result = z1 * z2;
} else if (s.equals("/")) {
result = z1 / z2;
} else {
result = 0.0;
}
or in the definition:
double result = 0.0;
Since this code:
if(s.equals("+"))
{ result = z1 + z2; }
if(s.equals("-"))
{ result = z1 - z2; }
if(s.equals("*"))
{ result = z1 * z2; }
if(s.equals("/"))
{ result = z1 / z2; }
does not have a final else
statement it is not guaranteed that result
will be initialized.
One way to overcome this problem:
if (s.equals("+")) {
result = z1 + z2;
} else if (s.equals("-")) {
result = z1 - z2;
} else if (s.equals("*")) {
result = z1 * z2;
} else if (s.equals("/")) {
result = z1 / z2;
} else {
result = 0.0;
}
or in the definition:
double result = 0.0;
edited Nov 22 at 17:03
answered Nov 22 at 17:02
forpas
6,3131218
6,3131218
add a comment |
add a comment |
up vote
1
down vote
The problem is that the environment checks whether everything is okay and sees that you have a few ifs where the variable might be initialized and finds that result
is not guaranteed to be initialized. As a result you get this error. Try to initialize it at the start:
double z1, z2, result = 0;
@Awais if this answer solved your problem, then you might consider accepting it as the correct answer. The other answer is correct as well.
– Lajos Arpad
Nov 22 at 17:04
add a comment |
up vote
1
down vote
The problem is that the environment checks whether everything is okay and sees that you have a few ifs where the variable might be initialized and finds that result
is not guaranteed to be initialized. As a result you get this error. Try to initialize it at the start:
double z1, z2, result = 0;
@Awais if this answer solved your problem, then you might consider accepting it as the correct answer. The other answer is correct as well.
– Lajos Arpad
Nov 22 at 17:04
add a comment |
up vote
1
down vote
up vote
1
down vote
The problem is that the environment checks whether everything is okay and sees that you have a few ifs where the variable might be initialized and finds that result
is not guaranteed to be initialized. As a result you get this error. Try to initialize it at the start:
double z1, z2, result = 0;
The problem is that the environment checks whether everything is okay and sees that you have a few ifs where the variable might be initialized and finds that result
is not guaranteed to be initialized. As a result you get this error. Try to initialize it at the start:
double z1, z2, result = 0;
answered Nov 22 at 16:59


Lajos Arpad
27.2k1861116
27.2k1861116
@Awais if this answer solved your problem, then you might consider accepting it as the correct answer. The other answer is correct as well.
– Lajos Arpad
Nov 22 at 17:04
add a comment |
@Awais if this answer solved your problem, then you might consider accepting it as the correct answer. The other answer is correct as well.
– Lajos Arpad
Nov 22 at 17:04
@Awais if this answer solved your problem, then you might consider accepting it as the correct answer. The other answer is correct as well.
– Lajos Arpad
Nov 22 at 17:04
@Awais if this answer solved your problem, then you might consider accepting it as the correct answer. The other answer is correct as well.
– Lajos Arpad
Nov 22 at 17:04
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53435360%2fhow-can-i-keep-my-value-from-an-if-statement%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
AalIYsfzGVo
s
might not be +, -, * or /. Ifs
isn't one of those, thenresult
is still undefined.– Elliott Frisch
Nov 22 at 16:51
yeah, but when inputting I'm making sure to use a simple "+" or "-"so it shouldn't be a problem. I mean it works if I use system.out.println(result) inside my if-statement
– Awais
Nov 22 at 16:54
Can you set a breakpoint to System.out.println(result) and check what the exact value of result is?
– Lajos Arpad
Nov 22 at 16:55
What are your values and what is the output at the end?
– Lajos Arpad
Nov 22 at 16:56
Sorry, but I'm not using a compiler. I'm just using a text editor and try to compile things through the windows console. I don't think i can set breakpoints there.
– Awais
Nov 22 at 17:00